C# Learning Diary 26---Interface Type
An interface contains the definition of a set of related functions that a class or structure can implement. For example, using an interface can include behavior from multiple sources in a class. Since the C# language does not support multiple inheritance, multiple inheritance can be achieved through interfaces. In short, the interface only contains the declaration of members (properties, events, indexers). How the definition of a member is implemented is determined by its derived class.
Declare an interface:
The interface is declared using the interface keyword, which is similar to the declaration of a class. Interface declarations are public by default, and interfaces cannot contain constants, fields, operators, instance constructors, destructors, or types. Interface members automatically become public members and cannot contain any access modifiers. Members cannot be static members either. For example:
public interface person //Define an interface
{ void setname();} //Declare a method
An instance of an interface:
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace Test1 {//定义一个person接口 public interface person {//只声明方法 void setname(string name); void setsex(char sex); void setage(uint age); void getinformation(); } //people继承于person class people:person { private string name; private char sex; private uint age; //实现接口中的方法 public void setname(string name) { this.name = name; } public void setsex(char sex) { this.sex = sex; } public void setage(uint age) { this.age = age; } public void getinformation() { Console.WriteLine("姓名:\t"+name); Console.WriteLine("性别:\t"+sex); Console.WriteLine("年龄:\t"+age); } } //多重继承 class student :people,person { } class Program { static void Main(string[] args) { people peo = new people(); peo.setname("HC666"); peo.setsex('男'); peo.setage(18); peo.getinformation(); student stu = new student(); stu.setname("HC555"); stu.setsex('男'); stu.setage(19); stu.getinformation(); } } }
Result:
My interface seems to have no effect. If it is removed, it can still run.
If we look at it from a design perspective. There are several classes that need to be written in the project. Since these classes are relatively complex and the workload is relatively large, each class requires a staff member to write. For example, programmer A will define the Dog class, and programmer B will write the Cat class. These two classes have nothing to do with each other originally, but because the user needs them to both implement a method about "calling", this requires a constraint on them. Let them all inherit from the IBark interface, in order to facilitate unified management. In addition, One is the convenience of calling. Of course, the purpose can be achieved without using an interface. But in this case, the constraint is not so obvious. If there are such classes and Duck classes, etc., it is inevitable that someone will miss such a method. .So it is more reliable and more binding through the interface.
The above is the content of C# Learning Diary 26---interface type. For more related content, please pay attention to the PHP Chinese website (www .php.cn)!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










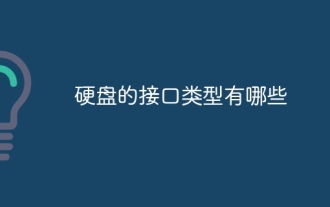
Hard drive interface types: 1. SATA interface, serial hard drive; 2. IDE interface, electronic integrated drive; 3. SCSI interface, small computer system interface; 4. SAS interface, computer hub technology; 5. M.2 interface, host computer Interface solution; 6. Fiber Channel hard disk interface, an interface specially designed for the network.
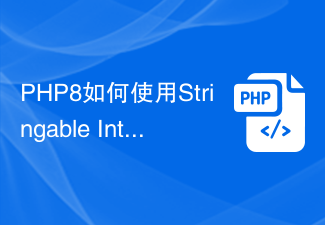
How does PHP8 use StringableInterface to handle various types of strings? PHP8 introduces a new interface Stringable, which can help developers process various types of strings more conveniently. In the past, we usually used the is_string() function to determine whether a variable is of string type, and then perform corresponding operations. Now, with the Stringable interface, we can handle strings more intuitively without having to determine their type.
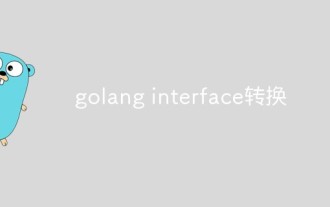
The interface in Go language is a very special type that can abstract any data type with certain characteristics and can perform type conversion. This feature makes interface play a very important role in the Go language, but interface type conversion is also a relatively complex issue that requires us to carefully discuss and understand.
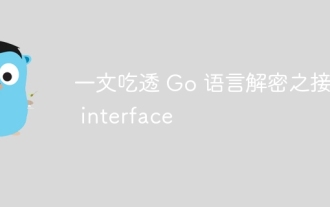
In the semantics of the Go language, as long as a type implements a defined set of methods, it is considered to be the same type and the same thing. People often call it duck typing because it is relatively consistent with the definition of duck typing.

Common interface types include VGA interface, HDMI interface, DP interface, DVI interface, USB interface, RJ45 interface, HDMI/MHL interface, Micro USB interface, Type-C interface, 3.5mm headphone interface, etc. Detailed introduction: 1. VGA interface: used to connect to a monitor, which is an analog signal interface; 2. HDMI interface: used to connect to high-definition multimedia equipment, which is a digital signal interface; 3. DP interface: the abbreviation of DisplayPort, which is a digital video interface standards and so on.
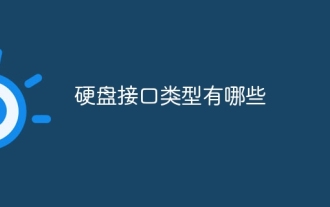
Hard drive interface types include IDE, SATA, SCSI, Fiber Channel, USB, eSATA, mSATA, PCIe, etc. Detailed introduction: 1. The IDE interface is a parallel interface, mainly used to connect devices such as hard drives and optical drives. It mainly has two types: ATA and ATAPI. The IDE interface has gradually been replaced by the SATA interface; 2. The SATA interface is a serial interface. Compared with the IDE interface, it has higher transmission speed, lower power consumption and smaller size; 3. SCSI interface, etc.
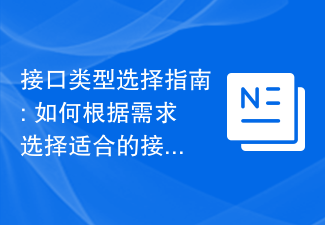
Interface type selection guide: How to choose a suitable interface type according to your needs, specific code examples are required. Introduction: In developing software, interfaces are an indispensable component. Choosing the right interface type is critical to software functionality and performance. This article will introduce several common interface types and provide code examples to help readers choose based on actual needs. 1. Synchronous interface: Synchronous interface is one of the most common interface types. It waits for a response to be received after sending a request before continuing to execute. Synchronous interfaces are typically used when real-time feedback is required
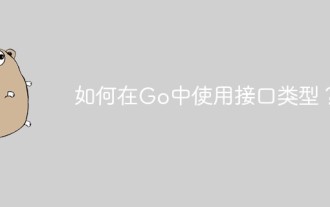
Go language is a statically typed programming language that supports the concept of interface types. An interface type is a convention that defines the set of methods a component should have. This convention can make the code more flexible and reusable, and can help us achieve better code organization. This article will introduce how to use interface types in Go, including tips for defining, implementing, and using interface types. 1. Define the interface type. Defining an interface type in Go is very simple. You only need to declare a set of methods. For example: typeWriterin
