C# parses Json into DateTable
C# Parse Json into DateTable
#region 将 Json 解析成 DateTable /// <summary> /// 将 Json 解析成 DateTable。 /// Json 数据格式如: /// {table:[{column1:1,column2:2,column3:3},{column1:1,column2:2,column3:3}]} /// </summary> /// <param name="strJson">要解析的 Json 字符串</param> /// <returns>返回 DateTable</returns> public DataTable JsonToDataTable(string strJson) { // 取出表名 var rg = new Regex(@"(?<={)[^:]+(?=:\[)", RegexOptions.IgnoreCase); string strName = rg.Match(strJson).Value; DataTable tb = null; //数据为空返回 if (strJson.Trim().Length == 0) { return tb; } // 检查strJson是否是json字符串 if (!JsonSplit.IsJson(strJson)) { return tb; } try { // 去除表名 strJson = strJson.Substring(strJson.IndexOf("[") + 1); strJson = strJson.Substring(0, strJson.IndexOf("]")); // 获取数据 rg = new Regex(@"(?<={)[^}]+(?=})"); MatchCollection mc = rg.Matches(strJson); for (int i = 0; i < mc.Count; i++) { string strRow = mc[i].Value; string[] strRows = strRow.Split(','); // 创建表 if (tb == null) { tb = new DataTable(); tb.TableName = strName; foreach (string str in strRows) { var dc = new DataColumn(); string[] strCell = str.Split(':'); dc.ColumnName = strCell[0].Replace("\"", ""); tb.Columns.Add(dc); } tb.AcceptChanges(); } // 增加内容 DataRow dr = tb.NewRow(); for (int j = 0; j < strRows.Length; j++) { dr[j] = strRows[j].Split(':')[1].Replace("\"", ""); } tb.Rows.Add(dr); tb.AcceptChanges(); } } catch (Exception ee) { MessageBox.Show(ee.ToString()); } return tb; } #endregion
Identify whether a string is in Json format: http://www.php.cn/csharp-article-352536.html
The format is as follows:
{ "table": [ { "column1": 1, "column2": 2, "column3": 3 }, { "column1": 1, "column2": 2, "column3": 3 } ] }
For example:
[{"Code":"MetaDataId","Name":"MetaDataId"},{"Code":"MetadataCode","Name":"编号"},{"Code":"SolutionName","Name":"名称"}]
After formatting:
[ { "Code": "MetaDataId", "Name": "MetaDataId" }, { "Code": "MetadataCode", "Name": "编号" }, { "Code": "SolutionName", "Name": "名称" } ]
Code formatting tool: http://tool.oschina.net/codeformat/xml
The result after conversion is as follows:

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










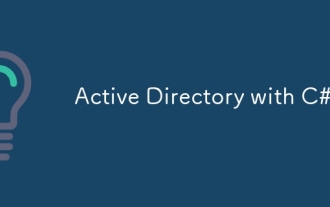
Guide to Active Directory with C#. Here we discuss the introduction and how Active Directory works in C# along with the syntax and example.
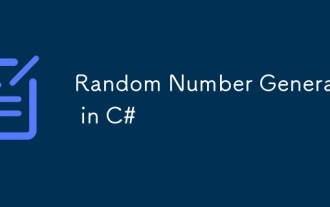
Guide to Random Number Generator in C#. Here we discuss how Random Number Generator work, concept of pseudo-random and secure numbers.
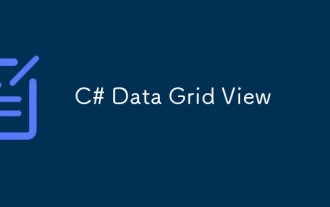
Guide to C# Data Grid View. Here we discuss the examples of how a data grid view can be loaded and exported from the SQL database or an excel file.
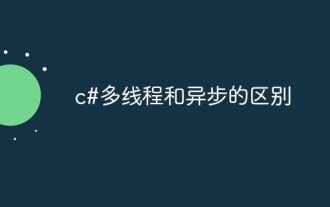
The difference between multithreading and asynchronous is that multithreading executes multiple threads at the same time, while asynchronously performs operations without blocking the current thread. Multithreading is used for compute-intensive tasks, while asynchronously is used for user interaction. The advantage of multi-threading is to improve computing performance, while the advantage of asynchronous is to not block UI threads. Choosing multithreading or asynchronous depends on the nature of the task: Computation-intensive tasks use multithreading, tasks that interact with external resources and need to keep UI responsiveness use asynchronous.
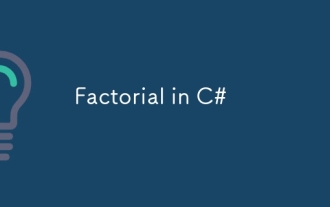
Guide to Factorial in C#. Here we discuss the introduction to factorial in c# along with different examples and code implementation.
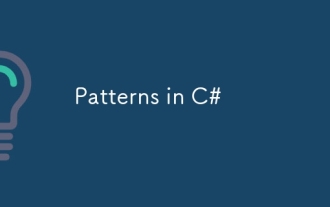
Guide to Patterns in C#. Here we discuss the introduction and top 3 types of Patterns in C# along with its examples and code implementation.
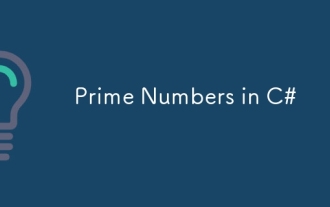
Guide to Prime Numbers in C#. Here we discuss the introduction and examples of prime numbers in c# along with code implementation.

Guide to Queue in C#. Here we discuss the introduction, working with different examples, constructors and function of queue in C#.
