Must-see efficient and clean CSS code principles
1. Use global reset
Different browser elements have different default attributes. Use Reset to reset some default attributes of browser elements to achieve browser compatibility. But it should be noted that please do not use global Reset:
*{ margin:0; padding:0; }
This is not only because it is a slow and inefficient method, but also leads to some unnecessary The element also has its margins and padding reset. It is recommended to refer to the practices of YUI Reset and Eric Meyer. Reset is not static. It needs to be modified appropriately according to the different needs of the project to achieve browser compatibility and operational convenience. The Reset I use is as follows:
/**Clear inner and outer margins **/
body, h1, h2, h3, h4, h5, h6, hr, p,
blockquote,
dl, dt, dd, ul, ol, li,
pre,
form, fieldset, legend, button, input, textarea,
th, td,
img{
border:medium none;
margin: 0;
padding: 0;
}
/**Set default font **/
body,button, input, select, textarea {
font: 12px/1.5 ,tahoma , Srial, helvetica, sans-serif;
}
h1, h2, h3, h4, h5, h6 { font-size: 100%; }
em{font-style:normal;}
/**Reset list elements **/
ul, ol { list-style: none; }
/**Reset hyperlink element **/
a { text-decoration: none; color:#333; }
a:hover { text-decoration: underline; color:#F40; }
/**Reset image elements **/
img{ border:0px;}
/**Reset table elements * */
table { border-collapse: collapse; border-spacing: 0; }
2. Good naming habits
No doubt messy or unsemantically named code will drive anyone crazy. Like this code:
.aaabb{margin:2px;color:red;}
I think even a beginner would not name a class like this in an actual project, but have you ever thought about it? The code is also very problematic:
My name is Wiky
If the original red font is changed to blue, then the style will become:
.red{color:bule;}
Such naming will be very confusing. The same name is .leftBar. It will also be troublesome if the sidebar needs to be modified to the right sidebar. Therefore, please do not use the characteristics of the element (color, position, size, etc.) to name a class or id. You can choose meaningful naming such as: #navigation{...}, .sidebar{...}, .postwrap{ ...}
In this way, no matter how you modify the styles that define these classes or ids, the connection between them and HTML elements will not be affected.
There is another situation. Some fixed styles will not be modified after they are defined. Then you don’t have to worry about the situation just mentioned when naming, such as
.alignleft{float:left;margin- right:20px;}
.alignright{float:right;text-align:right;margin-left:20px;}
.clear{clear:both;text-indent:-9999px;}
Then For such a paragraph
I am a paragraph!
If you need to change this paragraph from the original left alignment to right alignment, then you only need to modify its className to alignright.
3. Code abbreviation
CSS code abbreviation can improve the speed of writing code and simplify the amount of code. There are many properties that can be abbreviated in CSS, including margin, padding, border, font, background and color values, etc. If you learn the code abbreviation, the original code would be like this:
li{
font-family: Arial, Helvetica, sans-serif;
font-size: 1.2em;
line-height: 1.4em;
padding-top:5px;
padding-bottom:10px;
padding -left:5px;
}
can be abbreviated as:
li{
font: 1.2em/1.4em Arial, Helvetica, sans-serif;
padding:5px 0 10px 5px;
}
If you want to know more about how to abbreviate these attributes, you can refer to "Common CSS Abbreviation Syntax Summary".
4. Use CSS inheritance
If multiple child elements of the parent element on the page use the same style, it is best to define their same styles on their parent elements and let them inherit these CSS styles. This way you can maintain your code well and reduce the amount of code. So the original code is like this:
#container li{ font-family:Georgia, serif; }
#container p{ font-family:Georgia, serif; }
#container h1{font-family:Georgia , serif; }
can be abbreviated as:
#container{ font-family:Georgia, serif; }
5. Using multiple selectors
You can merge multiple CSS selectors into one. If they have a common style. Doing so not only keeps the code concise but also saves you time and space. For example:
h1{ font-family:Arial, Helvetica, sans-serif; font-weight:normal; }
h2{ font-family:Arial, Helvetica, sans-serif; font-weight:normal; }
h3{ font-family:Arial, Helvetica, sans-serif; font-weight:normal; }
can be combined into
h1, h2, h3{ font-family:Arial, Helvetica, sans-serif ; font-weight:normal; }
6. Appropriate code comments
Code comments can make it easier for others to understand your code, and reasonable organization of code comments can make the structure clearer. You can choose to add a directory at the beginning of the style sheet:
This way the structure of your code will be clear at a glance, and you can easily find and modify the code.
The main content of the code should also be divided appropriately, and the code should even be commented where necessary, which is also conducive to team development:
/*** Header ***/
#header{ height:145px; position:relative; }
#header h1{ width:324px; margin:45px 0 0 20px; float:left; height:72px;}
/*** Content *** /
#content{ background:#fff; width:650px; float:left; min-height:600px; overflow:hidden;}
#content h1{color:#F00}
#content .posts { overflow:hidden; }
#content .recent{ margin-bottom:20px; border-bottom:1px solid #f3f3f3; position:relative; overflow:hidden; }
/*** Footer ***/
#footer{ clear:both; padding:50px 5px 0; overflow:hidden;}
#footer h4{ color:#b99d7f; font-family:Arial, Helvetica, sans-serif; font-size:1.1em ; }
7. Sort your CSS code
If the properties in the code can be sorted alphabetically, it will be faster to find modifications:
/*** Style properties are sorted alphabetically ***/
div{
background-color:#3399cc;
color:#666;
font:1.2em/1.4em Arial, Helvetica, sans-serif;
height:300px;
margin :10px 5px;
padding:5px 0 10px 5px;
width:30%;
z-index:10;
}
8. Keep CSS readable
Writing Readable CSS will make it easier to find and modify styles. I think it's self-evident which of the following two cases is more readable.
/*** Write one line for each style attribute ***/
div{
background-color:#3399cc;
color:#666;
font: 1.2em/1.4em Arial, Helvetica, sans- serif;
height:300px;
margin:10px 5px;
padding:5px 0 10px 5px;
width:30%;
z-index:10;
}
/*** All style attributes are written on the same line ***/
div{ background-color:#3399cc; color:#666; font: 1.2em/1.4em Arial, Helvetica, sans-serif; height:300px; margin:10px 5px; padding:5px 0 10px 5px; width:30%; z-index:10; }
When it comes to some selectors with fewer style attributes, I will write a line:
/*** Selector attributes with fewer attributes are written on the same line ***/
div{ background-color:#3399cc; color:#666;}
This rule is not hard and fast, but no matter which way you write it, my suggestion is to always keep the code consistent. If there are many attributes, write them in separate lines. If there are less than 3 attributes, you can write one line.
9. Choose better style attribute values
Some attributes in CSS use different attribute values. Although the effects are similar, there are differences in performance, such as
The difference is that border:0 changes border Set to 0px, although it is not visible on the page, but according to the default value of border, the browser still renders border-width/border-color, that is, the memory value has been occupied.
And border:none sets the border to "none", which means there is no border. When the browser parses "none", it will not perform rendering actions, that is, it will not consume memory values. Therefore, it is recommended to use border:none;
Similarly, display:none hides the object browser and does not render it and does not occupy memory. And visibility:hidden will.
10. Use instead of @import
First of all, @import does not belong to the XHTML tag and is not part of the Web standard. It is not very compatible with earlier browsers and has a negative impact on the performance of the website. some negative effects. For details, please refer to "High-Performance Website Design: Don't Use @import". So, please avoid using @import
11. Use external style sheets
This principle is always a good design practice. Not only is it easier to maintain and modify, but more importantly, using external files can improve page speed, because CSS files can be cached in the browser. CSS built into the HTML document will be re-downloaded with the HTML document on each request. Therefore, in practical applications, there is no need to build CSS code into HTML documents:
or
Instead use< link>Import external style sheets:
12. Avoid using CSS expressions (Expression)
CSS expressions are a powerful (but dangerous) way to dynamically set CSS properties. Internet Explorer supports CSS expressions starting with version 5. In the following example, a CSS expression can be used to switch the background color every hour:
background-color: expression( (new Date()).getHours()%2 ? "#B8D4FF" : "#F08A00" );
As shown above, JavaScript expression is used in expression. CSS properties are set based on the evaluation of JavaScript expressions.
The problem with expressions is that they are evaluated more often than we think. Not only when the page is displayed and zoomed, but also when the page is scrolled and even when the mouse is moved, it will be recalculated. Add a counter to a CSS expression to track how often the expression is evaluated. You can easily achieve more than 10,000 calculations by simply moving the mouse on the page.
If you must use CSS expressions, be sure to remember that they are evaluated thousands of times and may have an impact on the performance of your page. So, avoid using CSS expressions unless absolutely necessary.
13. Code Compression
When you decide to deploy your website project to the Internet, you must consider compressing the CSS to remove comments and spaces to make the web page load faster. To compress your code, you can use some tools, such as YUI Compressor
, which can streamline CSS code and reduce file size for higher loading speed.
The above is the detailed content of Must-see efficient and clean CSS code principles. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
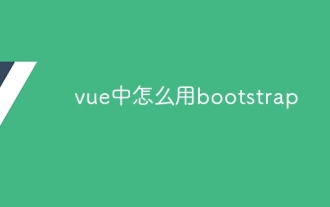
Using Bootstrap in Vue.js is divided into five steps: Install Bootstrap. Import Bootstrap in main.js. Use the Bootstrap component directly in the template. Optional: Custom style. Optional: Use plug-ins.

HTML defines the web structure, CSS is responsible for style and layout, and JavaScript gives dynamic interaction. The three perform their duties in web development and jointly build a colorful website.

WebdevelopmentreliesonHTML,CSS,andJavaScript:1)HTMLstructurescontent,2)CSSstylesit,and3)JavaScriptaddsinteractivity,formingthebasisofmodernwebexperiences.
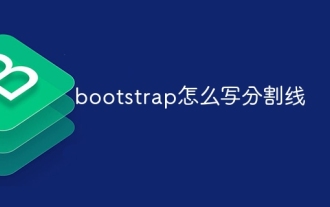
There are two ways to create a Bootstrap split line: using the tag, which creates a horizontal split line. Use the CSS border property to create custom style split lines.
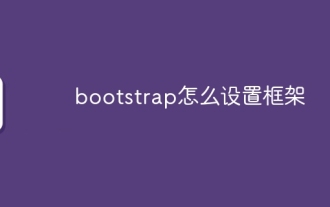
To set up the Bootstrap framework, you need to follow these steps: 1. Reference the Bootstrap file via CDN; 2. Download and host the file on your own server; 3. Include the Bootstrap file in HTML; 4. Compile Sass/Less as needed; 5. Import a custom file (optional). Once setup is complete, you can use Bootstrap's grid systems, components, and styles to create responsive websites and applications.
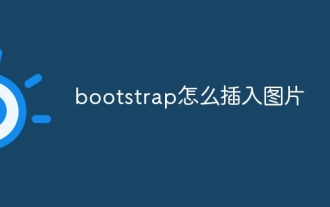
There are several ways to insert images in Bootstrap: insert images directly, using the HTML img tag. With the Bootstrap image component, you can provide responsive images and more styles. Set the image size, use the img-fluid class to make the image adaptable. Set the border, using the img-bordered class. Set the rounded corners and use the img-rounded class. Set the shadow, use the shadow class. Resize and position the image, using CSS style. Using the background image, use the background-image CSS property.
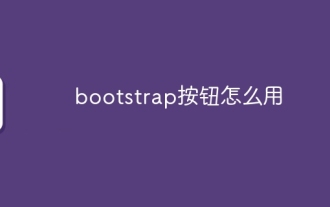
How to use the Bootstrap button? Introduce Bootstrap CSS to create button elements and add Bootstrap button class to add button text
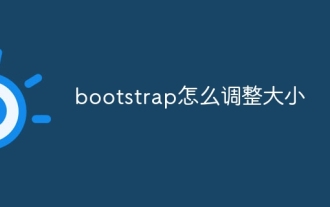
To adjust the size of elements in Bootstrap, you can use the dimension class, which includes: adjusting width: .col-, .w-, .mw-adjust height: .h-, .min-h-, .max-h-
