


How to create a horizontal sliding module? Example code for sliding events of web page elements
We still need to complete a few things before we can figure out this method. Currently, we have implemented touch gestures and mouse drag.
function addSlide(element, options) { // Validate arguments... // Resolve element... // Touch supports... // Mouse supports... // ToDo: Implement it. return null; }
Now let’s deal with the return value. This will be an object containing methods capable of unregistering swipe events.
return { dispose: function () { // Remove touch events. ele.removeEventListener("touchstart", touchStart, false); if (!!touchMove) ele.removeEventListener("touchmove", touchMove, false); ele.removeEventListener("touchend", touchEnd, false); // Remove mouse event. ele.removeEventListener("mousedown", mouseDown, false); } };
Now, you’re done! Let's take a look at the final code.
/** * Adds gesture handlers. * @param element the target element. * @param options the options. */ function addSlide(element, options) { if (!options || !element) return { dispose: function () { } }; // Get the element. var ele = !!element && typeof element === "string" ? document.getElementById(element) : element; if (!ele) return { dispose: function () { } }; // Get the minimum moving distances. var minX = options.minX; var minY = options.minY; if (minX == null || minX < 0) minX = 1; minX = Math.abs(minX); if (minY == null || minY < 0) minY = 1; minY = Math.abs(minY); // The handler occured after moving. var moved = function (x, y) { var isX = !y || (Math.abs(x) / Math.abs(y) > (minX + 0.01) / (minY + 0.01)); if (isX) { if (x > minX && !!options.turnLeft) options.turnLeft(ele, x); else if (x < -minX && !!options.turnRight) options.turnRight(ele, -x); } else { if (y > minY && !!options.turnUp) options.turnUp(ele, y); else if (y < -minY && !!options.turnDown) options.turnDown(ele, -y); } if (!!options.moveEnd) options.moveEnd(ele, x, y); }; // Touch starting event handler. var start = null; var touchStart = function (ev) { start = { x: ev.targetTouches[0].pageX, y: ev.targetTouches[0].pageY }; if (!!options.moveStart) options.moveStart(ele); }; ele.addEventListener("touchstart", touchStart, false); // Touch moving event handler. var touchMove = !!options.moving ? function (ev) { var point = ev.touches ? ev.touches[0] : ev; if (!point) return; var coor = { x: point.pageX - start.x, y: point.pageY - start.y }; options.moving(ele, coor.x, coor.y); } : null; if (!!touchMove) ele.addEventListener("touchmove", touchMove, false); // Touch ending event handler. var touchEnd = function (ev) { if (start == null) return; var x = ev.changedTouches[0].pageX - start.x; var y = ev.changedTouches[0].pageY - start.y; start = null; moved(x, y); }; ele.addEventListener("touchend", touchEnd, false); // Mouse event handler. var mouseDown = function (ev) { // Record current mouse position // when mouse down. var mStartP = getMousePosition(); // Mouse moving event handler. var mouseMove = function (ev) { var mCurP = getMousePosition(); var x = mCurP.x - mStartP.x; var y = mCurP.y - mStartP.y; options.moving(ele, x, y); }; document.body.addEventListener("mousemove", mouseMove, false); // Mouse up event handler. // Need remove all mouse event handlers. var mouseUpHandlers = []; var mouseUp = function (ev) { mouseUpHandlers.forEach(function (h, hi, ha) { h(ev); }); }; mouseUpHandlers.push( function () { document.body.removeEventListener("mousemove", mouseMove, false); }, function () { document.body.removeEventListener("mouseup", mouseUp, false); }, function (ev) { var mCurP = getMousePosition(); var x = mCurP.x - mStartP.x; var y = mCurP.y - mStartP.y; moved(x, y); } ); document.body.addEventListener("mouseup", mouseUp, false); }; ele.addEventListener("mousedown", mouseDown, false); // Return a disposable object // for removing all event handlers. return { dispose: function () { // Remove touch events. ele.removeEventListener("touchstart", touchStart, false); if (!!touchMove) ele.removeEventListener("touchmove", touchMove, false); ele.removeEventListener("touchend", touchEnd, false); // Remove mouse event. ele.removeEventListener("mousedown", mouseDown, false); } }; }
Now let’s do a test. Suppose there is a DOM element like this.
<p id="demo_gesture"> <p id="demo_gesture_fore"> </p> </p>
The style is as follows.
#demo_gesture { max-width: 800px; height: 20px; background-color: #EEE; border: 1px solid #CCC; border-radius: 10px; position: relative; overflow: hidden; } #demo_gesture > #demo_gesture_fore { color: #CCC; text-align: center; width: 20px; height: 20px; background-color: #CCC; border-radius: 10px; position: absolute; top: 0; left: 0; overflow: hidden; cursor: pointer; }
After the document element is loaded, we execute the following code.
var adjustPosition = function (ele, x) { x = (ele.position || 0) + x; if (x < 0) x = 0; else if (x > ele.parentElement.offsetWidth - ele.offsetWidth) x = ele.parentElement.offsetWidth - ele.offsetWidth; ele.style.left = x + "px"; return x; }; addSlide("demo_gesture_fore", { moving: function (ele, pos) { adjustPosition(ele, pos.x); }, moveEnd: function (ele, pos) { ele.position = adjustPosition(ele, pos.x); } });
Students who need to learn CSS please pay attention to the php Chinese websiteCSS video tutorial. Many css online video tutorials can be watched for free!
The above is the detailed content of How to create a horizontal sliding module? Example code for sliding events of web page elements. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
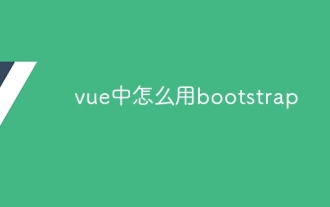
Using Bootstrap in Vue.js is divided into five steps: Install Bootstrap. Import Bootstrap in main.js. Use the Bootstrap component directly in the template. Optional: Custom style. Optional: Use plug-ins.

HTML defines the web structure, CSS is responsible for style and layout, and JavaScript gives dynamic interaction. The three perform their duties in web development and jointly build a colorful website.

WebdevelopmentreliesonHTML,CSS,andJavaScript:1)HTMLstructurescontent,2)CSSstylesit,and3)JavaScriptaddsinteractivity,formingthebasisofmodernwebexperiences.
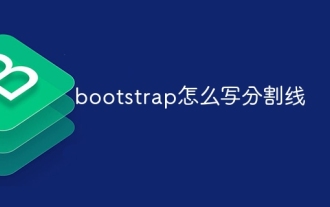
There are two ways to create a Bootstrap split line: using the tag, which creates a horizontal split line. Use the CSS border property to create custom style split lines.
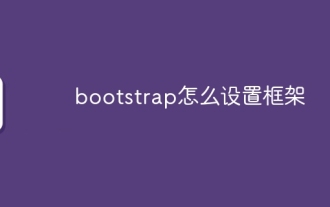
To set up the Bootstrap framework, you need to follow these steps: 1. Reference the Bootstrap file via CDN; 2. Download and host the file on your own server; 3. Include the Bootstrap file in HTML; 4. Compile Sass/Less as needed; 5. Import a custom file (optional). Once setup is complete, you can use Bootstrap's grid systems, components, and styles to create responsive websites and applications.
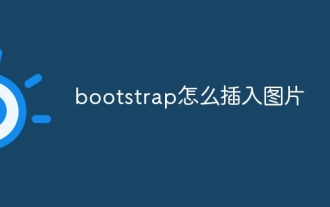
There are several ways to insert images in Bootstrap: insert images directly, using the HTML img tag. With the Bootstrap image component, you can provide responsive images and more styles. Set the image size, use the img-fluid class to make the image adaptable. Set the border, using the img-bordered class. Set the rounded corners and use the img-rounded class. Set the shadow, use the shadow class. Resize and position the image, using CSS style. Using the background image, use the background-image CSS property.
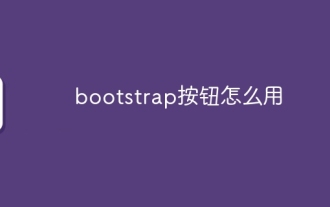
How to use the Bootstrap button? Introduce Bootstrap CSS to create button elements and add Bootstrap button class to add button text
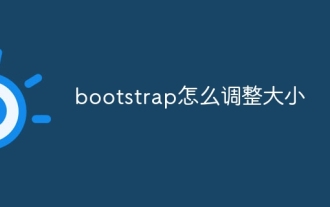
To adjust the size of elements in Bootstrap, you can use the dimension class, which includes: adjusting width: .col-, .w-, .mw-adjust height: .h-, .min-h-, .max-h-
