


activity switching animation and page switching animation_html/css_WEB-ITnose
Activity switching animation
To achieve Activity switching animation, you need to rely on overridePendingTransition. There are two parameters in it: the animation when entering the Activity and the animation when leaving the Activity.
It should be noted that it must be called immediately after StartActivity() or finish()
For example, if there is a Button in MainActivity, the code to jump to OtherActivity after clicking the Button is as follows:
Intent intent = new Intent(this, OtherActivity.class); startActivity(intent); this.overridePendingTransition(R.anim.enteralpha, R.anim.exitalpha);
Interface switching animation
Interface switching animation relies on ViewFlipper to implement
<ViewFlipper android:id="@+id/view_flipper" android:layout_width="match_parent" android:layout_height="match_parent" > <!-- 第一页 --> <LinearLayout android:layout_width="match_parent" android:layout_height="match_parent" android:background="#009900" android:orientation="vertical" > <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:text="第一页" /> </LinearLayout> <!-- 第二页 --> <LinearLayout android:layout_width="match_parent" android:layout_height="match_parent" android:background="#ffff00" android:orientation="vertical" > <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:text="第二页" /> </LinearLayout> </ViewFlipper>
Then determine the finger Is it sliding left or right?
@Override public boolean onTouchEvent(MotionEvent event) { // TODO Auto-generated method stub if (event.getAction() == MotionEvent.ACTION_DOWN) { startX = event.getX(); } else if (event.getAction() == MotionEvent.ACTION_UP) { float endX = event.getX(); if (endX > startX ) { flipper.showNext();// 显示下一页 } else if (endX<startX) { flipper.showPrevious();// 显示前一页 } return true; } return super.onTouchEvent(event); }
This way you can switch pages when sliding your finger left or right.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










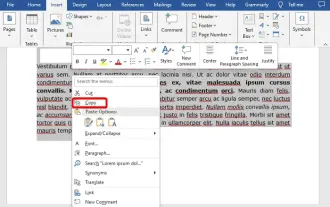
Want to copy a page in Microsoft Word and keep the formatting intact? This is a smart idea because duplicating pages in Word can be a useful time-saving technique when you want to create multiple copies of a specific document layout or format. This guide will walk you through the step-by-step process of copying pages in Word, whether you are creating a template or copying a specific page in a document. These simple instructions are designed to help you easily recreate your page without having to start from scratch. Why copy pages in Microsoft Word? There are several reasons why copying pages in Word is very beneficial: When you have a document with a specific layout or format that you want to copy. Unlike recreating the entire page from scratch
![Animation not working in PowerPoint [Fixed]](https://img.php.cn/upload/article/000/887/227/170831232982910.jpg?x-oss-process=image/resize,m_fill,h_207,w_330)
Are you trying to create a presentation but can't add animation? If animations are not working in PowerPoint on your Windows PC, then this article will help you. This is a common problem that many people complain about. For example, animations may stop working during presentations in Microsoft Teams or during screen recordings. In this guide, we will explore various troubleshooting techniques to help you fix animations not working in PowerPoint on Windows. Why aren't my PowerPoint animations working? We have noticed that some possible reasons that may cause the animation in PowerPoint not working issue on Windows are as follows: Due to personal

CSS animation: How to achieve the flash effect of elements, specific code examples are needed. In web design, animation effects can sometimes bring a good user experience to the page. The glitter effect is a common animation effect that can make elements more eye-catching. The following will introduce how to use CSS to achieve the flash effect of elements. 1. Basic implementation of flash First, we need to use the animation property of CSS to achieve the flash effect. The value of the animation attribute needs to specify the animation name, animation execution time, and animation delay time
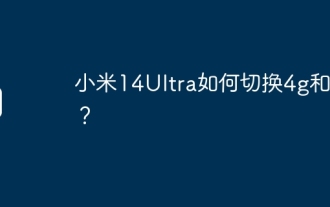
Xiaomi 14Ultra is one of the most popular Xiaomi models this year. Xiaomi 14Ultra not only upgrades the processor and various configurations, but also brings many new functional applications to users. This can be seen from the sales of Xiaomi 14Ultra mobile phones. It is very popular, but there are some commonly used functions that you may not know yet. So how does Xiaomi 14Ultra switch between 4g and 5g? Let me introduce the specific content to you below! How to switch between 4g and 5g on Xiaomi 14Ultra? 1. Open the settings menu of your phone. 2. Find and select the "Network" and "Mobile Network" options in the settings menu. 3. In the mobile network settings, you will see the "Preferred network type" option. 4. Click or select this option and you will see
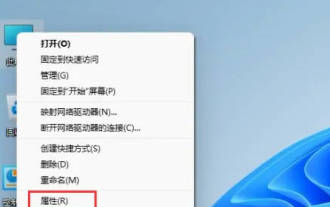
How to convert Win11 Home Edition to Win11 Professional Edition? In Win11 system, it is divided into Home Edition, Professional Edition, Enterprise Edition, etc., and most Win11 notebooks are pre-installed with Win11 Home Edition system. Today, the editor will show you the steps to switch from win11 home version to professional version! 1. First, right-click on this computer on the win11 desktop and properties. 2. Click Change Product Key or Upgrade Windows. 3. Then click Change Product Key after entering. 4. Enter the activation key: 8G7XN-V7YWC-W8RPC-V73KB-YWRDB and select Next. 5. Then it will prompt success, so you can upgrade win11 home version to win11 professional version.
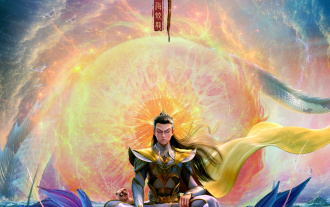
This website reported on January 26 that the domestic 3D animated film "Er Lang Shen: The Deep Sea Dragon" released a set of latest stills and officially announced that it will be released on July 13. It is understood that "Er Lang Shen: The Deep Sea Dragon" is produced by Mihuxing (Beijing) Animation Co., Ltd., Horgos Zhonghe Qiancheng Film Co., Ltd., Zhejiang Hengdian Film Co., Ltd., Zhejiang Gongying Film Co., Ltd., Chengdu The animated film produced by Tianhuo Technology Co., Ltd. and Huawen Image (Beijing) Film Co., Ltd. and directed by Wang Jun was originally scheduled to be released in mainland China on July 22, 2022. Synopsis of the plot of this site: After the Battle of the Conferred Gods, Jiang Ziya took the "Conferred Gods List" to divide the gods, and then the Conferred Gods List was sealed by the Heavenly Court under the deep sea of Kyushu Secret Realm. In fact, in addition to conferring divine positions, there are also many powerful evil spirits sealed in the Conferred Gods List.
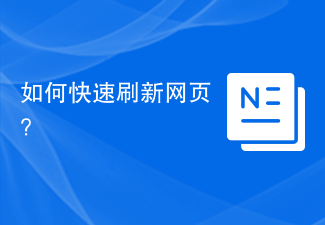
Page refresh is very common in our daily network use. When we visit a web page, we sometimes encounter some problems, such as the web page not loading or displaying abnormally, etc. At this time, we usually choose to refresh the page to solve the problem, so how to refresh the page quickly? Let’s discuss the shortcut keys for page refresh. The page refresh shortcut key is a method to quickly refresh the current web page through keyboard operations. In different operating systems and browsers, the shortcut keys for page refresh may be different. Below we use the common W
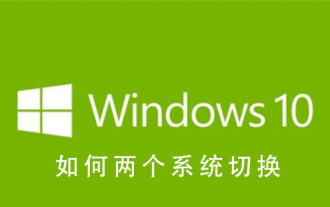
Many friends may not be used to the win system when they first come into contact with it. There are dual systems in the computer. At this time, you can actually switch between the two systems. Let's take a look at the detailed steps for switching between the two systems. How to switch between two systems in win10 system 1. Shortcut key switching 1. Press the "win" + "R" keys to open Run 2. Enter "msconfig" in the run box and click "OK" 3. In the open "System Configuration" In the interface, select the system you need and click "Set as Default". After completion, "Restart" can complete the switch. Method 2. Select switch when booting 1. When you have dual systems, a selection operation interface will appear when booting. You can use the keyboard " Up and down keys to select the system
