import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import java.util.Map.Entry;
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import android.os.Bundle;
import android.os.Handler;
import android.os.Message;
import android.app.Activity;
import android.view.Menu;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
public class MainActivity extends Activity {
private MyHandler handler;
private Map urlMap;
private Map idMap;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// final Map urlMap = new HashMap();
urlMap = new HashMap();
urlMap.put("tv_q", "http://xw.qq.com/");
urlMap.put("tv_taobao", "http://m.taobao.com/");
urlMap.put("tv_baidu", "http://m.baidu.com/");
urlMap.put("tv_sina", "http://sina.cn/");
urlMap.put("tv_google", "http://www.google.com.hk/");
urlMap.put("tv_apple", "http://www.apple.com/");
idMap = new HashMap();
idMap.put("tv_q", R.id.tv_q);
idMap.put("tv_taobao", R.id.tv_taobao);
idMap.put("tv_baidu", R.id.tv_baidu);
idMap.put("tv_sina", R.id.tv_sina);
idMap.put("tv_google", R.id.tv_google);
idMap.put("tv_apple", R.id.tv_apple);
Button button = (Button) findViewById(R.id.button1);
handler = new MyHandler();
// 设置监听
button.setOnClickListener(new Button.OnClickListener() {
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
Iterator> it = urlMap.entrySet()
.iterator();
while (it.hasNext()) {
String speed = "正在检测..";
Entry e = it.next();
String id = e.getKey();
String url = e.getValue();
TextView tv = (TextView) MainActivity.this
.findViewById(idMap.get(id));
tv.setText(speed);
}
new MyThread().start();
}
});
}
class MyHandler extends Handler {
@Override
public void handleMessage(Message msg) {
super.handleMessage(msg);
TextView tv = (TextView) findViewById(msg.what);
tv.setText(msg.getData().getString("speed"));
}
}
class MyThread extends Thread {
@Override
public void run() {
Iterator> it = urlMap.entrySet().iterator();
while (it.hasNext()) {
String speed = "访问失败";
Entry e = it.next();
String id = e.getKey();
String url = e.getValue();
long time = 0;
String result = "";
try {
long start = System.currentTimeMillis();
Document doc = Jsoup.connect(url).get();
long end = System.currentTimeMillis();
time = end - start;
result = doc.body().html();
} catch (Exception ex) {
ex.printStackTrace();
}
if (result.length() > 0) {
long len = result.getBytes().length;
speed = speed(time, len);
}
Message message = new Message();
Bundle bundle = new Bundle();
bundle.putString("speed", speed);
message.setData(bundle);
message.what = idMap.get(id);
handler.sendMessage(message);
}
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
private String speed(long time, long len) {
String speed = "";
if (time > 0) {
long s = len * 1000 / time; // B/ms
speed = s + "B/s";
if (s > 1024) {
s = s / 1024;
speed = s + "KB/s";
}
if (s > 1024) {
s = s / 1024;
speed = s + "MB/s";
}
if (s > 1024) {
s = s / 1024;
speed = s + "GB/s";
}
String size = len + "B";
if (len > 1024) {
len = len / 1024;
size = len + "KB";
}
if (len > 1024) {
len = len / 1024;
size = len + "MB";
}
String t = time + "ms";
if (time > 1000) {
time = time / 1000;
t = time + "sec";
if (time > 60) {
time = time / 60;
t = time + "min";
}
}
speed = speed + "(" + size + ", " + t + ")";
}
return speed;
}
}
Copy after login
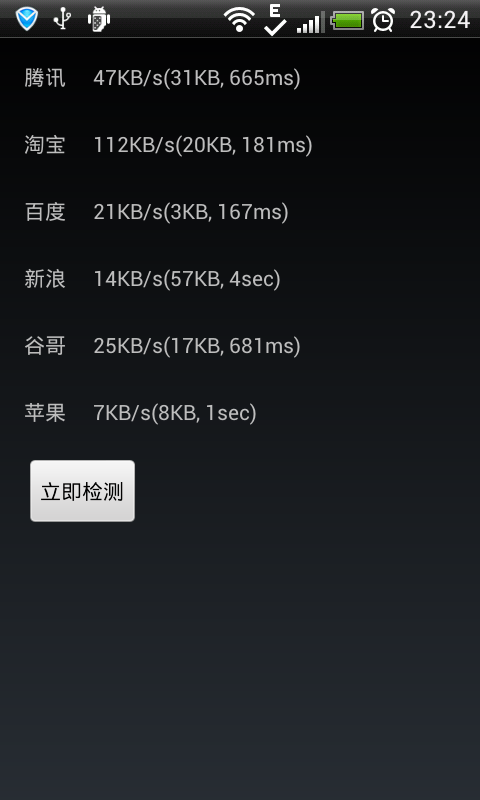