A small example of generating and parsing QR code images using ZXing in Java
Overview
ZXing is an open source Java class library for parsing 1D/2D barcodes in multiple formats. The goal is to be able to decode 1D barcodes of QR encoding, Data Matrix, and UPC. It provides clients on multiple platforms including: J2ME, J2SE and Android.
Practical
This example demonstrates how to use ZXing to generate and parse QR code images in a non-android Java project.
Installation
The maven project only needs to introduce dependencies:
<dependency> <groupId>com.google.zxing</groupId> <artifactId>core</artifactId> <version>3.3.0</version> </dependency> <dependency> <groupId>com.google.zxing</groupId> <artifactId>javase</artifactId> <version>3.3.0</version> </dependency>
Generate QR code images
ZXing has the following steps to generate QR code images:
1.com.google.zxing.MultiFormatWriter generates an image 2D matrix based on the content and image encoding parameters.
2. com.google.zxing.client.j2se.MatrixToImageWriter generates image files or image cache BufferedImage based on the image matrix.
public void encode(String content, String filepath) throws WriterException, IOException { int width = 100; int height = 100; Map<EncodeHintType, Object> encodeHints = new HashMap<EncodeHintType, Object>(); encodeHints.put(EncodeHintType.CHARACTER_SET, "UTF-8"); BitMatrix bitMatrix = new MultiFormatWriter().encode(content, BarcodeFormat.QR_CODE, width, height, encodeHints); Path path = FileSystems.getDefault().getPath(filepath); MatrixToImageWriter.writeToPath(bitMatrix, "png", path); }
Parsing QR code images
ZXing has the following steps to parse QR code images:
1. Use javax.imageio.ImageIO to read Image files are stored as a java.awt.image.BufferedImage object.
2. Convert java.awt.image.BufferedImage into a com.google.zxing.BinaryBitmap object that ZXing can recognize.
3.com.google.zxing.MultiFormatReader Parses com.google.zxing.BinaryBitmap based on image decoding parameters.
public String decode(String filepath) throws IOException, NotFoundException { BufferedImage bufferedImage = ImageIO.read(new FileInputStream(filepath)); LuminanceSource source = new BufferedImageLuminanceSource(bufferedImage); Binarizer binarizer = new HybridBinarizer(source); BinaryBitmap bitmap = new BinaryBitmap(binarizer); HashMap<DecodeHintType, Object> decodeHints = new HashMap<DecodeHintType, Object>(); decodeHints.put(DecodeHintType.CHARACTER_SET, "UTF-8"); Result result = new MultiFormatReader().decode(bitmap, decodeHints); return result.getText(); }
The following is an example of a generated QR code image:
The above is the entire article The content, I hope it will be helpful to everyone's learning, and I also hope that everyone will support the PHP Chinese website.
For more related articles on small examples of ZXing generating and parsing QR code images in Java, please pay attention to the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










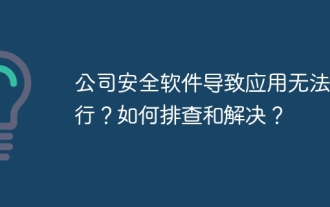
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
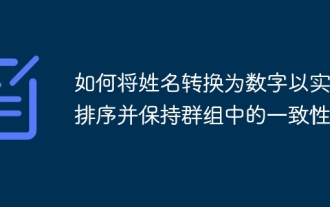
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
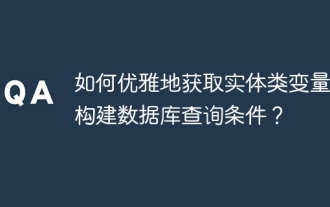
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
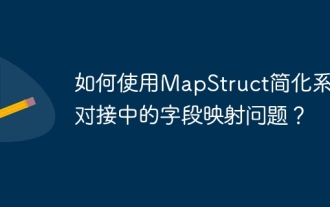
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
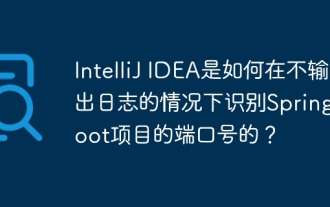
Start Spring using IntelliJIDEAUltimate version...
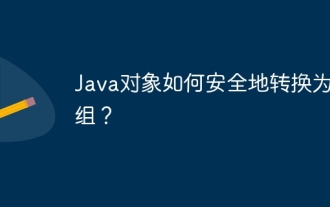
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
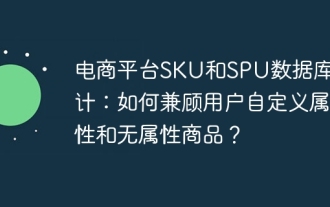
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
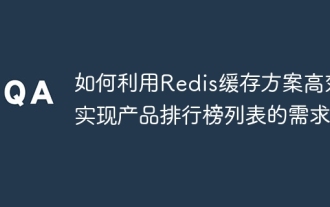
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
