Java method to obtain method parameter name information in code
Preface
Everyone knows that with the use of java8, a new object Parameter has been added to the corresponding method signature, which is used to represent specific parameter information. The corresponding parameter name can be obtained through its getName. .That is, if it is written in the code, if it is named username, then when passing parameters in the front desk, there is no need to write annotations such as @Parameter("username"), and mapping by name can be performed directly.
The following code reference is shown:
public class T { private interface T2 { void method(String username, String password); } public static void main(String[] args) throws Exception { System.out.println(T.class.getMethod("main", String[].class).getParameters()[0].getName()); System.out.println(T2.class.getMethod("method", String.class, String.class).getParameters()[0].getName()); System.out.println(T2.class.getMethod("method", String.class, String.class).getParameters()[1].getName()); } }
Before pressing java8, you can also get the parameter name information through some means, but the method is different. For example, through ParameterMethodNameResolver in spring mvc can also work normally in previous versions. However, it requires special compilation. What works here is LocalVariableTable and MethodParameters, which are compiled into local variable tables and method parameter tables in Chinese.
LocalVariableTable local variable table
According to the jvm specification, the local variable table exists in the Code attribute, and the Code attribute is an attribute of methodInfo. It can be understood that when a method has a method body, it will The corresponding Code attribute appears, and in the code attribute, in addition to the specific execution code, there will also be other information. Such as LineNumberTable (used to describe the location of each line of code).
The local variable table belongs to It is part of the debugging information in the method, so this information will not be generated in the class file by default. You need to turn on the -g or -g:vars switch. Fortunately, for IDE or maven compilation, these switches are It is enabled by default. In IDE, you can control it by setting (generate debugging info for idea) (default tick). In maven, you can control whether to output by debug or debugLevel in the plug-in maven-compiler-plugin (default The value is true).
The local variable table is after javap, as shown below:
//非静态方法 LocalVariableTable: Start Length Slot Name Signature 0 1 0 this LT; 0 1 1 count J 0 1 3 name Ljava/lang/String; //静态方法 LocalVariableTable: Start Length Slot Name Signature 0 101 0 args [Ljava/lang/String;
The local variable table not only saves parameter information, but also saves it in the entire method Temporary variables that may be used in the body, such as declared int i, etc. And as shown above, the difference between the expression method and the non-static method is the this variable in the first place. Therefore, you can read the number of parameters (method .getParameterCount), and then read the specified number of parameter information in the local variable table according to the method signature.
It should be noted that in the above figure, if the parameter is long or double , its slot occupies 2 slots. When obtaining parameter information through slots, you need to consider the type information of the parameters.
Since the interface method does not have a code attribute, there is no local variable table. Get an interface Method definition, the corresponding parameter name cannot be obtained through the local variable table
MethodParameters Method parameter table
The method parameter table was introduced after 1.8, so it only uses the class compiled and generated by jdk8 Only files have this information. Unlike the local variable table, it belongs to the MethodInfo attribute, that is, it is at the same level as the Code attribute. Whether it is an interface method or an ordinary method, it has this attribute. Therefore, even if it is an interface Method, you can also get the corresponding parameter information.
By default, there is no such information in the class. You need to use special compilation parameters - parameters to generate it, and in ide and maven, it is not available by default. Generate this information. In idea, you need to add this compilation parameter in java additional line parameters. In maven, you also need to add this parameter in the compilerArgs parameter of maven-compiler-plugin.
Method parameters The table is in the following form after javap:
//非静态方法 MethodParameters: Name Flags count name //静态方法 MethodParameters: Name Flags args
It can be seen that no matter whether it is static or not, in the parameter table, only the information used to describe the parameters will appear. The following Flags parameter Used in some special scenarios, such as final parameters for method rewriting, etc.
Some tools that can be used
In addition to using the native api and spring tool kit, there are other tools You can get parameter name information. In the spring system, the interface used to describe parameter names is ParameterNameDiscoverer. Through it, you can obtain the corresponding parameter name information. In addition, com.thoughtworks.paranamer:paranamer in this toolkit Paranamer can also process corresponding information. However, the support for jdk8's methodParameters is not very high. Users can achieve their own goals by extending it.
Summary
The above is a summary of how to obtain method parameter name information in the code in Java. I hope it will be helpful to everyone in learning or using Java. If you have any questions, you can leave a message to communicate.
For more articles related to Java’s method of obtaining method parameter name information in the code, please pay attention to the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
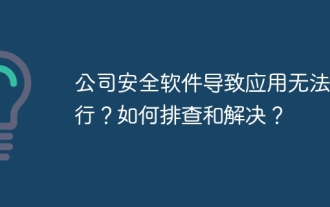
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
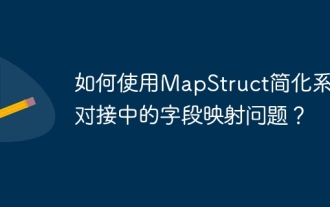
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
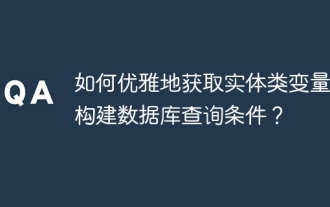
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
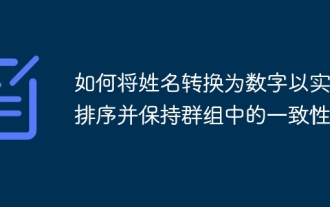
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
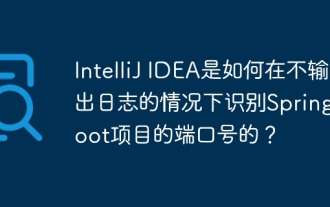
Start Spring using IntelliJIDEAUltimate version...
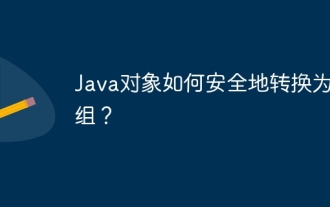
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
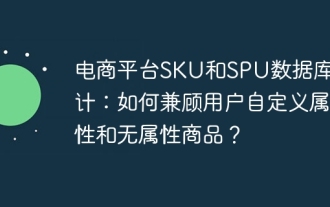
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
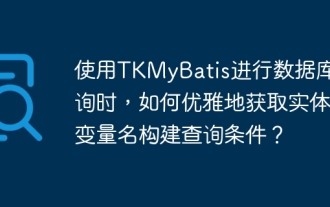
When using TKMyBatis for database queries, how to gracefully get entity class variable names to build query conditions is a common problem. This article will pin...
