


Method to directly convert Json string to object in Java (including multi-layer List collection)
Class used: net.sf.json.JSONObject
When using JSON, in addition to importing the json-lib-2.2-jdk15.jar package downloaded from the JSON website, there must be other Several dependency packages: commons-beanutils.jar, commons-httpclient.jar, commons-lang.jar, ezmorph.jar, morph-1.0.1.jar
The following is an example code:
// JSON转换 JSONObject jsonObj = JSONObject.fromObject(jsonStrBody); Map<String, Class> classMap = new HashMap<String, Class>(); classMap.put("results", WeatherBean_Baidu_City.class); classMap.put("index", WeatherBean_Baidu_City_Index.class); classMap.put("weather_data", WeatherBean_Baidu_City_Weatherdata.class); // 将JSON转换成WeatherBean_Baidu WeatherBean_Baidu weather = (WeatherBean_Baidu) JSONObject.toBean(jsonObj, WeatherBean_Baidu.class, classMap); System.out.println(weather.getResults());
Several JAVA class codes used:
package com.lenovo.conference.entity.vo; import java.io.Serializable; import java.util.List; /** * 天气Bean * * @author SHANHY * */ @SuppressWarnings("serial") public class WeatherBean_Baidu implements Serializable { private String error;//错误号 private String status;//状态值 private String date;//日期 private List<WeatherBean_Baidu_City> results;//城市天气预报集合(因为一次可以查询多个城市) public WeatherBean_Baidu() { super(); } public String getError() { return error; } public void setError(String error) { this.error = error; } public String getStatus() { return status; } public void setStatus(String status) { this.status = status; } public String getDate() { return date; } public void setDate(String date) { this.date = date; } public List<WeatherBean_Baidu_City> getResults() { return results; } public void setResults(List<WeatherBean_Baidu_City> results) { this.results = results; } }
package com.lenovo.conference.entity.vo; import java.io.Serializable; import java.util.List; /** * 天气Bean * * @author SHANHY * */ @SuppressWarnings("serial") public class WeatherBean_Baidu_City implements Serializable { private String currentCity;//城市名称 private String pm25;//pm2.5值 private List<WeatherBean_Baidu_City_Index> index;//指数集合 private List<WeatherBean_Baidu_City_Weatherdata> weather_data;//几天的天气集合 public WeatherBean_Baidu_City() { super(); } public String getCurrentCity() { return currentCity; } public void setCurrentCity(String currentCity) { this.currentCity = currentCity; } public String getPm25() { return pm25; } public void setPm25(String pm25) { this.pm25 = pm25; } public List<WeatherBean_Baidu_City_Index> getIndex() { return index; } public void setIndex(List<WeatherBean_Baidu_City_Index> index) { this.index = index; } public List<WeatherBean_Baidu_City_Weatherdata> getWeather_data() { return weather_data; } public void setWeather_data( List<WeatherBean_Baidu_City_Weatherdata> weather_data) { this.weather_data = weather_data; } }
package com.lenovo.conference.entity.vo; import java.io.Serializable; /** * 天气Bean * * @author SHANHY * */ @SuppressWarnings("serial") public class WeatherBean_Baidu_City_Weatherdata implements Serializable { private String date;// 日期 private String dayPictureUrl;// 白天的天气图片 private String nightPictureUrl;// 晚上的天气图片 private String weather;// 天气 private String wind;// 风向 private String temperature;// 温度 public WeatherBean_Baidu_City_Weatherdata() { super(); } public String getDate() { return date; } public void setDate(String date) { this.date = date; } public String getDayPictureUrl() { return dayPictureUrl; } public void setDayPictureUrl(String dayPictureUrl) { this.dayPictureUrl = dayPictureUrl; } public String getNightPictureUrl() { return nightPictureUrl; } public void setNightPictureUrl(String nightPictureUrl) { this.nightPictureUrl = nightPictureUrl; } public String getWeather() { return weather; } public void setWeather(String weather) { this.weather = weather; } public String getWind() { return wind; } public void setWind(String wind) { this.wind = wind; } public String getTemperature() { return temperature; } public void setTemperature(String temperature) { this.temperature = temperature; } }
package com.lenovo.conference.entity.vo; import java.io.Serializable; /** * 天气Bean * * @author SHANHY * */ @SuppressWarnings("serial") public class WeatherBean_Baidu_City_Index implements Serializable { private String title;//标题 private String zs;//舒适度 private String tipt;//指数简述 private String des;//指数概述 public WeatherBean_Baidu_City_Index() { super(); } public String getTitle() { return title; } public void setTitle(String title) { this.title = title; } public String getZs() { return zs; } public void setZs(String zs) { this.zs = zs; } public String getTipt() { return tipt; } public void setTipt(String tipt) { this.tipt = tipt; } public String getDes() { return des; } public void setDes(String des) { this.des = des; } }
The corresponding JSON string parsed in the example
{"error":0,"status":"success","date":"2015-01-15","results":[{"currentCity":"南京","pm25":"83","index":[{"title":"穿衣","zs":"较冷","tipt":"穿衣指数","des":"建议着厚外套加毛衣等服装。年老体弱者宜着大衣、呢外套加羊毛衫。"},{"title":"洗车","zs":"较适宜","tipt":"洗车指数","des":"较适宜洗车,未来一天无雨,风力较小,擦洗一新的汽车至少能保持一天。"},{"title":"旅游","zs":"适宜","tipt":"旅游指数","des":"天气较好,气温稍低,会感觉稍微有点凉,不过也是个好天气哦。适宜旅游,可不要错过机会呦!"},{"title":"感冒","zs":"少发","tipt":"感冒指数","des":"各项气象条件适宜,无明显降温过程,发生感冒机率较低。"},{"title":"运动","zs":"较不宜","tipt":"运动指数","des":"阴天,且天气寒冷,推荐您在室内进行低强度运动;若坚持户外运动,请选择合适的运动并注意保暖。"},{"title":"紫外线强度","zs":"最弱","tipt":"紫外线强度指数","des":"属弱紫外线辐射天气,无需特别防护。若长期在户外,建议涂擦SPF在8-12之间的防晒护肤品。"}],"weather_data":[{"date":"周四 01月15日 (实时:6℃)","dayPictureUrl":"http://api.map.baidu.com/images/weather/day/yin.png","nightPictureUrl":"http://api.map.baidu.com/images/weather/night/duoyun.png","weather":"阴转多云","wind":"北风微风","temperature":"8 ~ 4℃"},{"date":"周五","dayPictureUrl":"http://api.map.baidu.com/images/weather/day/duoyun.png","nightPictureUrl":"http://api.map.baidu.com/images/weather/night/qing.png","weather":"多云转晴","wind":"西北风3-4级","temperature":"12 ~ 0℃"},{"date":"周六","dayPictureUrl":"http://api.map.baidu.com/images/weather/day/qing.png","nightPictureUrl":"http://api.map.baidu.com/images/weather/night/duoyun.png","weather":"晴转多云","wind":"东北风3-4级","temperature":"8 ~ 0℃"},{"date":"周日","dayPictureUrl":"http://api.map.baidu.com/images/weather/day/qing.png","nightPictureUrl":"http://api.map.baidu.com/images/weather/night/qing.png","weather":"晴","wind":"西风微风","temperature":"10 ~ -1℃"}]},{"currentCity":"徐州","pm25":"154","index":[{"title":"穿衣","zs":"较冷","tipt":"穿衣指数","des":"建议着厚外套加毛衣等服装。年老体弱者宜着大衣、呢外套加羊毛衫。"},{"title":"洗车","zs":"较适宜","tipt":"洗车指数","des":"较适宜洗车,未来一天无雨,风力较小,擦洗一新的汽车至少能保持一天。"},{"title":"旅游","zs":"适宜","tipt":"旅游指数","des":"天气较好,但丝毫不会影响您出行的心情。温度适宜又有微风相伴,适宜旅游。"},{"title":"感冒","zs":"较易发","tipt":"感冒指数","des":"天气较凉,较易发生感冒,请适当增加衣服。体质较弱的朋友尤其应该注意防护。"},{"title":"运动","zs":"较不宜","tipt":"运动指数","des":"天气较好,但考虑天气寒冷,推荐您进行各种室内运动,若在户外运动请注意保暖并做好准备活动。"},{"title":"紫外线强度","zs":"最弱","tipt":"紫外线强度指数","des":"属弱紫外线辐射天气,无需特别防护。若长期在户外,建议涂擦SPF在8-12之间的防晒护肤品。"}],"weather_data":[{"date":"周四 01月15日 (实时:6℃)","dayPictureUrl":"http://api.map.baidu.com/images/weather/day/duoyun.png","nightPictureUrl":"http://api.map.baidu.com/images/weather/night/duoyun.png","weather":"多云","wind":"南风微风","temperature":"10 ~ 3℃"},{"date":"周五","dayPictureUrl":"http://api.map.baidu.com/images/weather/day/duoyun.png","nightPictureUrl":"http://api.map.baidu.com/images/weather/night/duoyun.png","weather":"多云","wind":"北风3-4级","temperature":"11 ~ -4℃"},{"date":"周六","dayPictureUrl":"http://api.map.baidu.com/images/weather/day/duoyun.png","nightPictureUrl":"http://api.map.baidu.com/images/weather/night/duoyun.png","weather":"多云","wind":"东风微风","temperature":"6 ~ -4℃"},{"date":"周日","dayPictureUrl":"http://api.map.baidu.com/images/weather/day/duoyun.png","nightPictureUrl":"http://api.map.baidu.com/images/weather/night/duoyun.png","weather":"多云","wind":"西风3-4级","temperature":"11 ~ -1℃"}]}]}
The above method of directly converting Json strings into objects in Java (including multi-layer List collections) is all the content shared by the editor. I hope it can be useful to everyone. A reference, and I hope everyone will support the PHP Chinese website.
For more related articles on methods for directly converting Json strings into objects in Java (including multi-layer List collections), please pay attention to the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










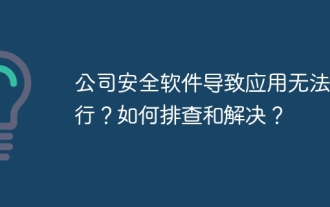
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
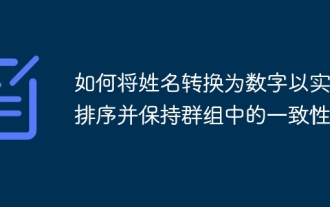
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
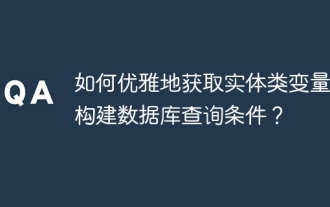
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
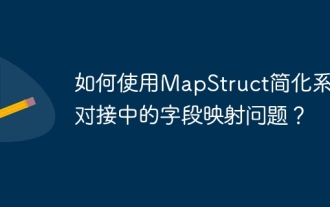
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
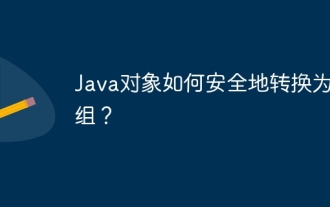
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
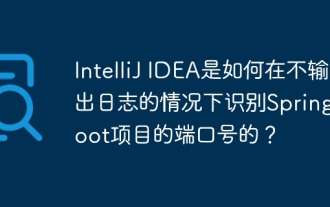
Start Spring using IntelliJIDEAUltimate version...
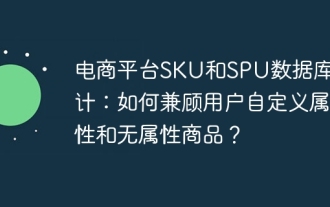
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
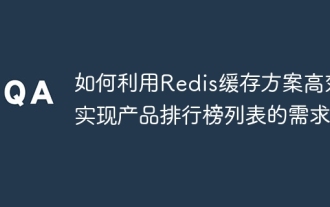
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
