Java Improvement (8) ----- Implementing Multiple Inheritance
Multiple inheritance means that a class can inherit behaviors and characteristics from more than one parent class at the same time. However, we know that Java only allows single inheritance in order to ensure data security. Sometimes we think that if multiple inheritance needs to be used in the system, it is often a bad design. At this time, what we often need to think about is not how to use multiple inheritance, but whether there are problems with your design. But sometimes we do need to implement multiple inheritance. Inheritance, and this situation really exists in real life, such as inheritance: we inherit both the behavior and characteristics of our father and the behavior and characteristics of our mother. Fortunately, Java is very kind and understanding of us. It provides two ways for us to implement multiple inheritance: interfaces and inner classes.
1. Interface
When introducing interfaces and abstract classes, we learned that subclasses can only inherit one parent class, which means that there can only be a single Inheritance, but can implement multiple interfaces, which paves the way for us to implement multiple inheritance.
For interfaces, sometimes it represents more than just a more pure abstract class. The interface does not have any specific implementation, that is to say, there is no storage related to the interface, so it cannot Combination of multiple interfaces is blocked.
interface CanFight { void fight(); } interface CanSwim { void swim(); } interface CanFly { void fly(); } public class ActionCharacter { public void fight(){ } } public class Hero extends ActionCharacter implements CanFight,CanFly,CanSwim{ public void fly() { } public void swim() { } /** * 对于fight()方法,继承父类的,所以不需要显示声明 */ }
2. Internal classes
The above use of interfaces to implement multiple inheritance is a more feasible and common way. When introducing internal classes, we talked about the changes in the implementation of multiple inheritance made by internal classes. It is more perfect, and it also makes it clear that if the parent class is an abstract class or a concrete class, then I can only implement multiple inheritance through inner classes. How to use internal classes to achieve multiple inheritance, please see the following example: how a son uses multiple inheritance to inherit the excellent genes of his father and mother.
First, Father and Mother:
public class Father { public int strong(){ return 9; } } public class Mother { public int kind(){ return 8; } }
The highlight is here, Son:
public class Son { /** * 内部类继承Father类 */ class Father_1 extends Father{ public int strong(){ return super.strong() + 1; } } class Mother_1 extends Mother{ public int kind(){ return super.kind() - 2; } } public int getStrong(){ return new Father_1().strong(); } public int getKind(){ return new Mother_1().kind(); } }
Test procedure:
public class Test1 { public static void main(String[] args) { Son son = new Son(); System.out.println("Son 的Strong:" + son.getStrong()); System.out.println("Son 的kind:" + son.getKind()); } } ---------------------------------------- Output: Son 的Strong:10 Son 的kind:6
The son inherited his father and became stronger than his father. He also inherited his mother, but his gentleness index dropped. Two inner classes are defined here. They inherit the Father class and the Mother class respectively, and both can obtain the behavior of their respective parent classes very naturally. This is an important feature of the inner class: the inner class can inherit one from the outer class. Irrelevant classes ensure the independence of internal classes. It is based on this that multiple inheritance becomes possible.
The above is the content of java improvement (eight)-----implementing multiple inheritance. For more related content, please pay attention to the PHP Chinese website (www.php.cn) !

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
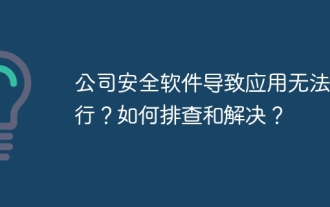
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
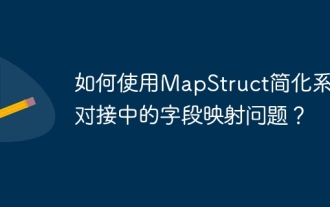
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
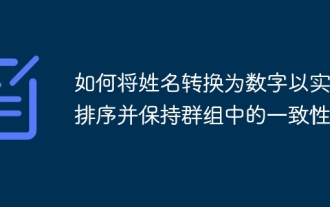
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
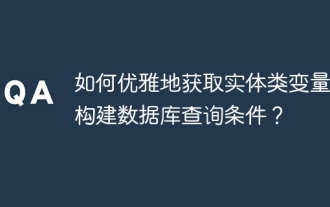
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
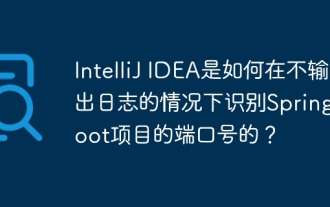
Start Spring using IntelliJIDEAUltimate version...
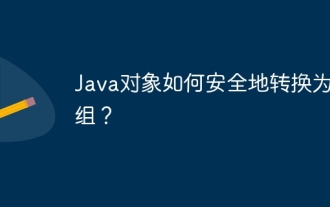
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
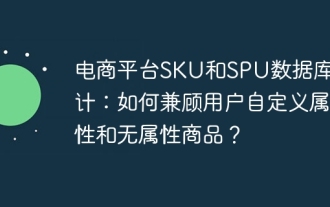
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
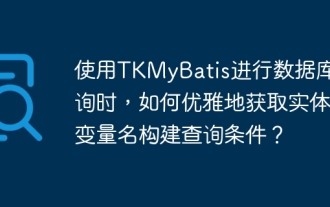
When using TKMyBatis for database queries, how to gracefully get entity class variable names to build query conditions is a common problem. This article will pin...
