04.Java Basics - Classes
Basic concepts
#In the object-oriented concept, we know that all objects are represented by classes.
In Java, classes are defined by the keyword class.
Similarly, classes in Java have many forms, including ordinary classes, abstract classes, and inner classes.
Inner classes include: member inner classes, local inner classes, anonymous inner classes, and static inner classes.
Normal category
There is nothing to say about this.
public class Demo { }
Abstract class
1. Basic concept
All objects mentioned above are passed through classes To describe, but not all classes are used to describe objects.
If a class does not contain enough information to describe a specific object (we can understand it as an incomplete class), such a class is Abstract class.
Abstract classes are defined through the keyword absract. It can contain abstract methods and non-abstract methods.
2. Example study
Abstract class definition
public abstract class Parent { // 1.成员变量,与普通类无差别 private String word="aa"; // 2.非抽象方法,与普通类无差 private void talk(){ System.out.println("Parent is talking"); } // 3.抽象方法,访问权限只能是 public 和 protected abstract void print(); }
Abstract class inheritance
// 1.抽象类继承抽象类,抽象子类不用实现父类的方法public abstract class Son extends Parent { } // 2.普通类继承抽象类,普通子类必须实现父类的所有抽象方法public class Grandson extends Son { @Override void print() { System.out.println("I am Grandson"); } }
Abstract class call
// 错误,抽象类不允许被实例化//Parent parent = new Parent();
Through the above code, we can summarize the following points:
Abstract classes and abstract methods cannot be modified by private and must use keywords abstract definition.
If the subclass is not an abstract class, it must implement all abstract methods of the parent class.
Abstract class is not allowed to be instantiated, compilation error.
Abstract classes can also contain ordinary methods and member variables.
Member inner class
1. Basic concepts
Located inside a class The class is called a member inner class.
Member inner classes have the following characteristics: all properties of their outer classes can be accessed without any special conditions.
2. Example study
Member internal class definition:
public class Outter { private int a = 10; static int b = 20; int c = 30; // 内部类 class Inner { void print(int d) { System.out.println(a + "-" +b+ "-" +c+ "-" + "-" +d; } } // 取得内部类 Inner getInner() { return new Inner(); } }
Member internal class call
Outter out = new Outter();// 创建内部类的两种方式: Outter.Inner inner = out.new Inner();Outter.Inner inner2 = out.getInner();inner.print(20);
3. Principle analysis
By decompiling the class file, the command is as follows:
javap -v Outter
After executing the command, two class files are obtained: Oututter.Class and Oututter$Inner.Class.
Explanation: For virtual machines, internal classes are actually the same as regular classes. So Inner is still compiled into an independent class, rather than a certain field of the Outter class.
But because the member inner class looks like a member of the outer class, it can have the same access rights as the member.
Local internal classes
1. Basic concepts
There are two types of local internal classes:
Class within the method.
Classes within the scope.
It can be regarded as an internal local variable of the method or scope, so its access rights are limited to the method or the scope.
Like local variables, it cannot be modified by the public, protected, private, and static keywords.
2. Example study
public class Man { public Object getWoman() { // 注意:三个变量都相互不受影响 int age = 30; // 1.方法内的类 class Woman { int age = 20; } // 2.作用域内的类,此时作用域为 if if(true){ class Son{ int age = 10; } } return new Woman(); } }
Anonymous inner class
1.Basic concepts
Anonymous inner classes are the only classes without a constructor. Because of this feature, the scope of use of anonymous inner classes is very limited, and most of them are used for interface callbacks.
It has the following characteristics:
The anonymous inner class is automatically named Oututter$1.class by the system during compilation.
Anonymous inner classes are generally used to inherit other classes or implement interfaces. There is no need to add additional methods, but only the implementation or rewriting of inherited methods.
Anonymous inner classes cannot access local variables in outer class methods unless the variables are declared as final type
2. Example study
public class Test { // a 属于全局变量 int a =100; public static void main(String[] args) { Test t = new Test(); t.test(200); } // b 属于局部变量 public void test(final int b) { // c 属于局部变量 final int c = 300; // 匿名内部类 new Thread() { int d = 400; public void run() { System.out.println(a+"-"+b+"-"+c+"-"+d); }; }.start(); } }
3. Principle analysis
You can get two class files through the decompile command: Outter.class and Oututter$1.class.
About the life cycle of local variables:
When the method is called, local variables are created on the stack. When the method ends, it is popped off the stack and the local variables die.
About the declaration cycle of inner class objects:
Create an anonymous inner class object, and the system allocates memory for the object until no variables are referenced. Points to the memory allocated to the object before it will be processed by GC.
So there is a situation:
The member method has been called and the local variable has died, but the object of the anonymous inner class is still alive.
So the final keyword + copy method is used in Java to solve the problem:
final 关键字:因为它的特性是一旦变量被赋值后,就不能被修改。
复制:在匿名内部类中直接复制了一个与局部变量值的数,让它变成自己的局部变量。
所以当局部变量的生命周期结束后,匿名内部类照样可以访问 final 类型的局部变量,因为它自己拷贝了一份,且与原局部变量的值始终一致。
下面针对上面的代码来分析:
当 test 方法执行完毕之后,变量 b、c 的生命周期就结束了。然而 Thread 对象的生命周期很可能还没有结束。因此要将 b、c 设置为 final 。
a 之所以不采用 final 修饰,因为它是全局变量,生命周期是随着类的结束而结束。而类的生命周期肯定大于匿名内部类。
静态内部类
1.基本概念
静态内部类也是定义在一个类里面的类,只不过在类的前面多了一个关键字static。
静态内部类和静态成员变量其实具有相同的特点:
它只有类有关,与对象无关。因此可以在没有外部类对象情况下,创建静态内部类。
不能访问外部类的非静态成员或方法
2.实例探究
内部静态类定义:
public class Outter { int a = 5; static int b = 500; // 静态内部类 static class Inner { public Inner() { // 只能访问外部类的静态成员 System.out.println(b); } } }
静态内部类调用:
// 静态内部类的调用不依赖外部类对象Outter.Inner inner= new Outter.Inner();
接口内部类
1.基本概念
接口内部类,顾名思义就是在接口内定义的类。
2.实例探究
接口内部类定义:
public interface People{ class Man { public void print() { System.out.println("man.print()..."); } } }
接口内部类调用:
People.Man man = new People.Man();
以上就是04.Java 基础 - 类的内容,更多相关内容请关注PHP中文网(www.php.cn)!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










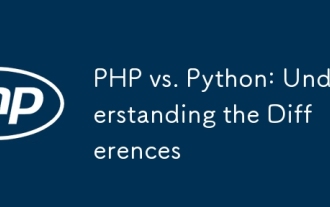
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
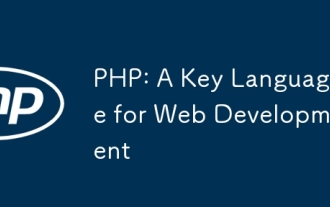
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
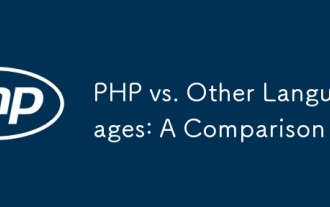
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
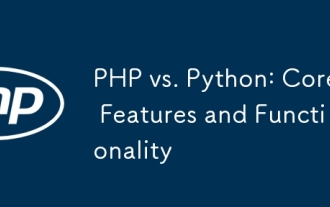
PHP and Python each have their own advantages and are suitable for different scenarios. 1.PHP is suitable for web development and provides built-in web servers and rich function libraries. 2. Python is suitable for data science and machine learning, with concise syntax and a powerful standard library. When choosing, it should be decided based on project requirements.
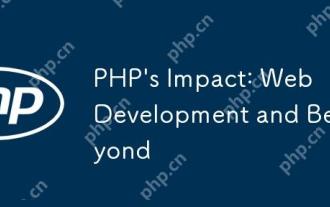
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
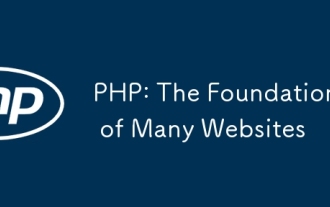
The reasons why PHP is the preferred technology stack for many websites include its ease of use, strong community support, and widespread use. 1) Easy to learn and use, suitable for beginners. 2) Have a huge developer community and rich resources. 3) Widely used in WordPress, Drupal and other platforms. 4) Integrate tightly with web servers to simplify development deployment.
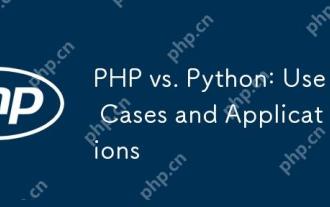
PHP is suitable for web development and content management systems, and Python is suitable for data science, machine learning and automation scripts. 1.PHP performs well in building fast and scalable websites and applications and is commonly used in CMS such as WordPress. 2. Python has performed outstandingly in the fields of data science and machine learning, with rich libraries such as NumPy and TensorFlow.

The tools and frameworks that need to be mastered in H5 development include Vue.js, React and Webpack. 1.Vue.js is suitable for building user interfaces and supports component development. 2.React optimizes page rendering through virtual DOM, suitable for complex applications. 3.Webpack is used for module packaging and optimize resource loading.
