A detailed introduction to the Composite pattern in Java
Class diagram
##
/** * 树 整体 * * @author stone * */ public class Tree { private TreeNode root; //根节点 public Tree(String name) { this.root = new TreeNode(name); } public TreeNode getRoot() { return root; } }
/** * 树节点 部份 * 也可以自身代表树:一堆节点组成了一颗树 * * @author stone * */ public class TreeNode { private String name; private TreeNode parent; private List<TreeNode> children; public TreeNode(String name) { this.name = name; this.children = new ArrayList<TreeNode>(); } public void setName(String name) { this.name = name; } public String getName() { return name; } public void setParent(TreeNode parent) { this.parent = parent; } public TreeNode getParent() { return parent; } public List<TreeNode> getChildren() { return children; } public void add(TreeNode node) { this.children.add(node); } public void remove(TreeNode node) { this.children.remove(node); } }
/* * 组合(Composite)模式 又叫做部分-整体模式 * 它使我们层级、树形结构的问题中,模糊了简单元素和复杂元素的概念,客户程序可以像处理简单元素一样来处理复杂元素,从而使得客户程序与复杂元素的内部结构解耦 * 以下情况下适用Composite模式: 1.你想表示对象的部分-整体层次结构 2.你希望用户忽略组合对象与单个对象的不同,用户将统一地使用组合结构中的所有对象。 */ public class Test { public static void main(String[] args) { // Tree treeA = new Tree("A"); // treeA.getRoot().add(new TreeNode("B")); // treeA.getRoot().add(new TreeNode("C")); // TreeNode treeNodeD = new TreeNode("D"); // treeNodeD.add(new TreeNode("D1")); // treeNodeD.add(new TreeNode("D2")); // treeA.getRoot().add(treeNodeD); // print(treeA.getRoot()); /* * 上面使用了Tree对象, * 下面只使用TreeNode对象,符合组合模式的定义,既代表部分也代表整体 */ TreeNode treeA = new TreeNode("A"); treeA.add(new TreeNode("B")); treeA.add(new TreeNode("C")); TreeNode treeNodeD = new TreeNode("D"); treeNodeD.add(new TreeNode("D1")); treeNodeD.add(new TreeNode("D2")); treeA.add(treeNodeD); print(treeA); /* * 其他示例:文件系统{目录、文件}, 类似这种可用递归遍历的结构, * 用一个对象就能表示部分与整体,都可以用组合模式 */ } public static void print(TreeNode root) { if (root == null) return; LinkedList<TreeNode> linkedList = new LinkedList<TreeNode>(); linkedList.add(root); while (!linkedList.isEmpty()) { TreeNode node = linkedList.removeFirst(); System.out.println(node.getName()); List<TreeNode> children = node.getChildren(); for (int i = 0; i < children.size(); i++) { TreeNode next = children.get(i); List<TreeNode> children2 = next.getChildren(); if (!children2.isEmpty()) { linkedList.add(next); } else { System.out.println(next.getName()); } } } } }
A B C D D1 D2
The above is the detailed content of A detailed introduction to the Composite pattern in Java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics











AI can help optimize the use of Composer. Specific methods include: 1. Dependency management optimization: AI analyzes dependencies, recommends the best version combination, and reduces conflicts. 2. Automated code generation: AI generates composer.json files that conform to best practices. 3. Improve code quality: AI detects potential problems, provides optimization suggestions, and improves code quality. These methods are implemented through machine learning and natural language processing technologies to help developers improve efficiency and code quality.

HTML5 brings five key improvements: 1. Semantic tags improve code clarity and SEO effects; 2. Multimedia support simplifies video and audio embedding; 3. Form enhancement simplifies verification; 4. Offline and local storage improves user experience; 5. Canvas and graphics functions enhance the visualization of web pages.
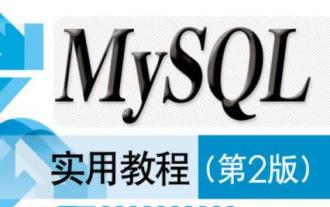
MySQL functions can be used for data processing and calculation. 1. Basic usage includes string processing, date calculation and mathematical operations. 2. Advanced usage involves combining multiple functions to implement complex operations. 3. Performance optimization requires avoiding the use of functions in the WHERE clause and using GROUPBY and temporary tables.
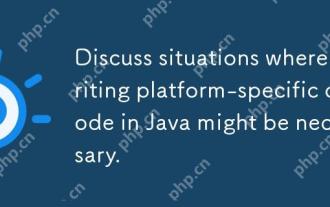
Reasons for writing platform-specific code in Java include access to specific operating system features, interacting with specific hardware, and optimizing performance. 1) Use JNA or JNI to access the Windows registry; 2) Interact with Linux-specific hardware drivers through JNI; 3) Use Metal to optimize gaming performance on macOS through JNI. Nevertheless, writing platform-specific code can affect the portability of the code, increase complexity, and potentially pose performance overhead and security risks.
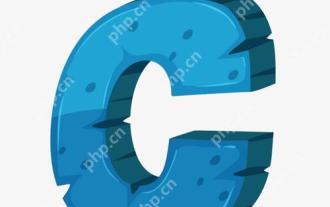
typetraits are used in C for compile-time type checking and operation, improving code flexibility and type safety. 1) Type judgment is performed through std::is_integral and std::is_floating_point to achieve efficient type checking and output. 2) Use std::is_trivially_copyable to optimize vector copy and select different copy strategies according to the type. 3) Pay attention to compile-time decision-making, type safety, performance optimization and code complexity. Reasonable use of typetraits can greatly improve code quality.
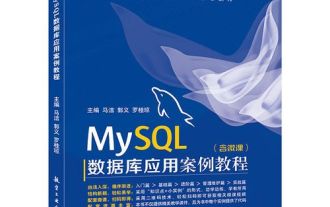
Methods for configuring character sets and collations in MySQL include: 1. Setting the character sets and collations at the server level: SETNAMES'utf8'; SETCHARACTERSETutf8; SETCOLLATION_CONNECTION='utf8_general_ci'; 2. Create a database that uses specific character sets and collations: CREATEDATABASEexample_dbCHARACTERSETutf8COLLATEutf8_general_ci; 3. Specify character sets and collations when creating a table: CREATETABLEexample_table(idINT
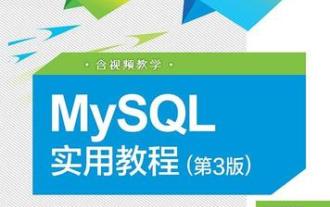
Renaming a database in MySQL requires indirect methods. The steps are as follows: 1. Create a new database; 2. Use mysqldump to export the old database; 3. Import the data into the new database; 4. Delete the old database.
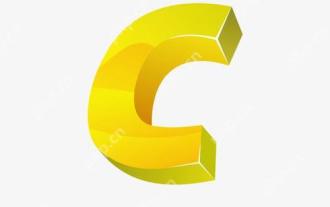
Implementing singleton pattern in C can ensure that there is only one instance of the class through static member variables and static member functions. The specific steps include: 1. Use a private constructor and delete the copy constructor and assignment operator to prevent external direct instantiation. 2. Provide a global access point through the static method getInstance to ensure that only one instance is created. 3. For thread safety, double check lock mode can be used. 4. Use smart pointers such as std::shared_ptr to avoid memory leakage. 5. For high-performance requirements, static local variables can be implemented. It should be noted that singleton pattern can lead to abuse of global state, and it is recommended to use it with caution and consider alternatives.
