The Collections tool class provides four static method operations
The Collections tool class provides a large number of operations for Collection/Map. This article mainly introduces the Collections tool class_Compiled by the Power Node Java Academy. Friends in need can refer to
The Collections tool class provides a large number of operations for Collection/Map. It can be divided into four categories, all of which are Static (static) method:
1. Sorting operation (mainly related to List interface)
- ## reverse( List list): Reverse the order of the elements in the specified List collection
- shuffle(List list): Randomly sort (shuffle) the elements in the List
- sort(List list): Sort the elements in the List according to natural ascending order
- sort(List list, Comparator c): Sort with a custom comparator
- swap(List list, int i, int j): Swap the element at i and the element out of j in the specified List collection
- rotate(List list, int distance): Shift all elements to the right by the specified length. If distance is equal to size, the result remains unchanged
public void testSort() { System.out.println("原始顺序:" + list); Collections.reverse(list); System.out.println("reverse后顺序:" + list); Collections.shuffle(list); System.out.println("shuffle后顺序:" + list); Collections.swap(list, 1, 3); System.out.println("swap后顺序:" + list); Collections.sort(list); System.out.println("sort后顺序:" + list); Collections.rotate(list, 1); System.out.println("rotate后顺序:" + list); }
Reverse order: [c Zhao Wu, e Qian Qi, a Li Si, d Sun Liu, b Zhang San]
The order after shuffle: [b Zhang San, c Zhao Wu, d Sun Liu, e Qian Qi, a Li Si]
The order after swap: [b Zhang San, e Qian Qi, d Sun Six, c Zhao Wu, a Li Si]
The order after sorting: [a Li Si, b Zhang San, c Zhao Wu, d Sun Liu, e Qian Qi]
The order after rotating: [e Qian Qi, a Li Si, b Zhang San, c Zhao Wu, d Sun Liu]
2. Search and replace (mainly related to Collection interface)
- binarySearch(List list, Object key): Use binary search method to obtain the index of the specified object in the List, provided that the collection has been sorted ## max( Collection coll): Returns the maximum element
- max(Collection coll, Comparator comp): Returns the maximum element
- based on the custom comparator min(Collection coll): Returns the smallest element
- min(Collection coll, Comparator comp): Returns the smallest element
- based on the custom comparator fill(List list, Object obj): Fill with the specified object
- frequency(Collection Object o): Return the number of times the specified object appears in the specified collection
- replaceAll(List list, Object old, Object new): Replace
public void testSearch() { System.out.println("给定的list:" + list); System.out.println("max:" + Collections.max(list)); System.out.println("min:" + Collections.min(list)); System.out.println("frequency:" + Collections.frequency(list, "a李四")); Collections.replaceAll(list, "a李四", "aa李四"); System.out.println("replaceAll之后:" + list); // 如果binarySearch的对象没有排序的话,搜索结果是不确定的 System.out.println("binarySearch在sort之前:" + Collections.binarySearch(list, "c赵五")); Collections.sort(list); // sort之后,结果出来了 System.out.println("binarySearch在sort之后:" + Collections.binarySearch(list, "c赵五")); Collections.fill(list, "A"); System.out.println("fill:" + list); }
Copy after loginOutput
The given list: [b Zhang San, d Sun Liu, a Li Si, e Qian Qi, c Zhao Wu]
min:a Li Si
frequency: 1
After replaceAll: [ b Zhang San, d Sun Liu, aa Li Si, e Qian Qi, c Zhao Wu]
binarySearch before sort: -4
binarySearch after sort: 2
fill: [A, A, A , A, A]
Collections tool class provides multiple syn
onizedXxx Method, this method returns the synchronization object corresponding to the specified collection object, thereby solving the security problem of threads when multiple threads concurrently access the collection. HashSet, ArrayList, and HashMap are all thread-unsafe. If synchronization needs to be considered, use these methods. These methods mainly include: synchronizedSet, synchronizedSortedSet, synchronizedList, synchronizedMap, synchronizedSortedMap.
It should be pointed out in particular that when using the iterative method to traverse the collection, you need to manually synchronize the returned collection.
Map m = Collections.synchronizedMap(new HashMap()); ... Set s = m.keySet(); // Needn't be in synchronized block ... synchronized (m) { // Synchronizing on m, not s! Iterator i = s.iterator(); // Must be in synchronized block while (i.hasNext()) foo(i.next()); }
Collections has three types of methods that can return an immutable collection:
1. emptyXxx(): returns an Empty immutable collection object
2. singletonXxx(): Returns an immutable collection object that only contains the specified object.
3. unmodifiableXxx(): Returns the immutable value of the specified collection object
View5.Others1. disjoint(Collection> c1, Collection> c2) - Returns true if there are no identical elements in the two specified collections.
2. addAll(Collection super T> c, T... a) - A convenient way to add all specified elements to the specified collection. Demonstration:
3. Comparator
public void testOther() { List<String> list1 = new ArrayList<String>(); List<String> list2 = new ArrayList<String>(); // addAll增加变长参数 Collections.addAll(list1, "大家好", "你好","我也好"); Collections.addAll(list2, "大家好", "a李四","我也好"); // disjoint检查两个Collection是否的交集 boolean b1 = Collections.disjoint(list, list1); boolean b2 = Collections.disjoint(list, list2); System.out.println(b1 + "\t" + b2); // 利用reverseOrder倒序 Collections.sort(list1, Collections.reverseOrder()); System.out.println(list1); }
输出
true false
[我也好, 大家好, 你好]
6. 完整代码
package com.bjpowernode.test; import java.util.*; import org.junit.Before; import org.junit.Test; public class CollectionsTest { private Listlist = new ArrayList (); @Before public void init() { // 准备测试数据 list.add("b张三"); list.add("d孙六"); list.add("a李四"); list.add("e钱七"); list.add("c赵五"); } @Test public void testUnmodifiable() { System.out.println("给定的list:" + list); List<String> unmodList = Collections.unmodifiableList(list); unmodList.add("再加个试试!"); // 抛出:java.lang.UnsupportedOperationException // 这一行不会执行了 System.out.println("新的unmodList:" + unmodList); } @Test public void testSort() { System.out.println("原始顺序:" + list); Collections.reverse(list); System.out.println("reverse后顺序:" + list); Collections.shuffle(list); System.out.println("shuffle后顺序:" + list); Collections.swap(list, 1, 3); System.out.println("swap后顺序:" + list); Collections.sort(list); System.out.println("sort后顺序:" + list); Collections.rotate(list, 1); System.out.println("rotate后顺序:" + list); } @Test public void testSearch() { System.out.println("给定的list:" + list); System.out.println("max:" + Collections.max(list)); System.out.println("min:" + Collections.min(list)); System.out.println("frequency:" + Collections.frequency(list, "a李四")); Collections.replaceAll(list, "a李四", "aa李四"); System.out.println("replaceAll之后:" + list); // 如果binarySearch的对象没有排序的话,搜索结果是不确定的 System.out.println("binarySearch在sort之前:" + Collections.binarySearch(list, "c赵五")); Collections.sort(list); // sort之后,结果出来了 System.out.println("binarySearch在sort之后:" + Collections.binarySearch(list, "c赵五")); Collections.fill(list, "A"); System.out.println("fill:" + list); } @Test public void testOther() { List<String> list1 = new ArrayList<String>(); List<String> list2 = new ArrayList<String>(); // addAll增加变长参数 Collections.addAll(list1, "大家好", "你好","我也好"); Collections.addAll(list2, "大家好", "a李四","我也好"); // disjoint检查两个Collection是否的交集 boolean b1 = Collections.disjoint(list, list1); boolean b2 = Collections.disjoint(list, list2); System.out.println(b1 + "\t" + b2); // 利用reverseOrder倒序 Collections.sort(list1, Collections.reverseOrder()); System.out.println(list1); } }
【相关推荐】
1. Java免费视频教程
2. YMP在线手册
3. 全面解析Java注解
The above is the detailed content of The Collections tool class provides four static method operations. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










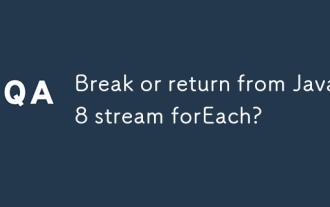
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
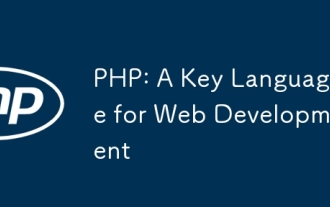
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
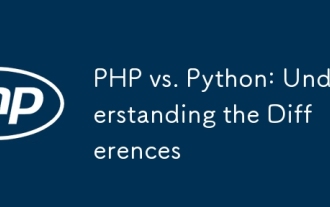
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
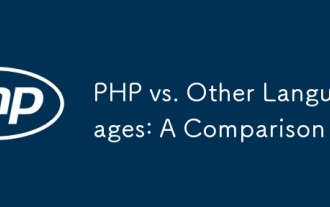
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
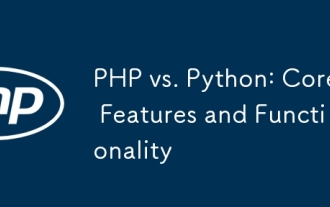
PHP and Python each have their own advantages and are suitable for different scenarios. 1.PHP is suitable for web development and provides built-in web servers and rich function libraries. 2. Python is suitable for data science and machine learning, with concise syntax and a powerful standard library. When choosing, it should be decided based on project requirements.
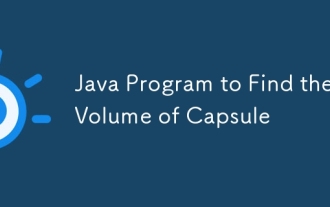
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
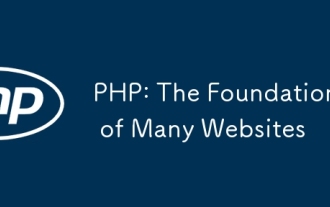
The reasons why PHP is the preferred technology stack for many websites include its ease of use, strong community support, and widespread use. 1) Easy to learn and use, suitable for beginners. 2) Have a huge developer community and rich resources. 3) Widely used in WordPress, Drupal and other platforms. 4) Integrate tightly with web servers to simplify development deployment.
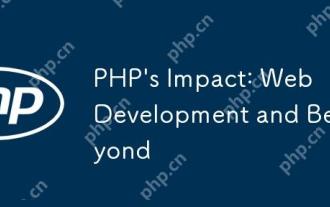
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
