Detailed explanation of second-level cache in java
This article mainly introduces the detailed explanation of hibernate second-level cache in Java. The editor thinks it is quite good. Now I will share it with you and give it as a reference. Let’s follow the editor to take a look
Hibernate’s second-level cache
1. Cache overview
Cache ): A very common concept in the computer field. It is located between the application and the permanent data storage source (such as a file or database on the hard disk). Its function is to reduce the frequency of the application directly reading and writing the permanent data storage source, thereby improving the application's running performance. The data in the cache is a copy of the data in the data storage source. The physical medium of cache is usually memory
hibernate provides two levels of cache
The first level of cache isSession Level cache, which is a transaction-wide cache. This level of cache is managed by hibernate, and generally no intervention is required
The second level of cache is the SessionFactory level cache, which is a process-wide cache
Hibernate's cache can be divided into two categories:
Built-in cache: Hibernate comes with it and cannot be uninstalled. Usually during the initialization phase of Hibernate, Hibernate will store the mapping metadata and predefined SQL statements are placed in the SessionFactory cache. The mapping metadata is a copy of the data in the mapping file, and the predefined SQL statements are pushed out by Hibernate based on the mapping metadata. The built-in cache is read-only.
External cache (second-level cache): A configurable cache plug-in. By default, SessionFactory will not enable this cache plug-in. The data in the external cache is a copy of the database data, external The physical medium of the cache can be memory or hard disk
2. Understand the concurrent access strategy of the second-level cache
3. Configure the process-wide second-level cache (configuring ehcache cache)
1 Copy ehcache-1.5.0.jar to the lib directory of the current project
Depend on backport-util-concurrent and commons-logging
2 Turn on the second level cache
<property name="hibernate.cache.use_second_level_cache">true</property>
3 To specify the cache Supplier
<property name="hibernate.cache.provider_class"> org.hibernate.cache.EhCacheProvider</property>
4 Specify the class that uses the second-level cache
Method 1 Use the *.hbm.xml configuration of the class
Select what you need to use The persistence class of the second-level cache sets the concurrent access policy of its second-level cache. The cache sub-element of the
<!-- 指定使用二级缓存的类 放在maping下面 --> <!-- 配置类级别的二级缓存 --> <class-cache class="com.sihai.c3p0.Customer" usage="read-write"/> <class-cache class="com.sihai.c3p0.Order" usage="read-write"/> <!-- 配置集合级别的二级缓存 --> <collection-cache collection="com.sihai.c3p0.Customer.orders" usage="read-write"/>
Configuration file ehcache.xml (fixed name) (placed in the class path)
<ehcache xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="../config/ehcache.xsd"> <diskStore path="c:/ehcache"/> <defaultCache maxElementsInMemory="5" eternal="false" timeToIdleSeconds="120" timeToLiveSeconds="120" overflowToDisk="true" maxElementsOnDisk="10000000" diskPersistent="false" diskExpiryThreadIntervalSeconds="120" memoryStoreEvictionPolicy="LRU" /> </ehcache>
4. Test
package com.sihai.hibernate3.test; import java.util.Iterator; import java.util.List; import org.hibernate.Query; import org.hibernate.Session; import org.hibernate.Transaction; import org.junit.Test; import com.sihai.hibernate3.demo1.Customer; import com.sihai.hibernate3.demo1.Order; import com.sihai.utils.HibernateUtils; public class HibernateTest6 { @Test // 查询缓存的测试 public void demo9(){ Session session = HibernateUtils.getCurrentSession(); Transaction tx = session.beginTransaction(); Query query = session.createQuery("select c.cname from Customer c"); // 使用查询缓存: query.setCacheable(true); query.list(); tx.commit(); session = HibernateUtils.getCurrentSession(); tx = session.beginTransaction(); query = session.createQuery("select c.cname from Customer c"); query.setCacheable(true); query.list(); tx.commit(); } @SuppressWarnings("unused") @Test // 更新时间戳 public void demo8(){ Session session = HibernateUtils.getCurrentSession(); Transaction tx = session.beginTransaction(); Customer customer = (Customer) session.get(Customer.class, 2); session.createQuery("update Customer set cname = '奶茶' where cid = 2").executeUpdate(); tx.commit(); session = HibernateUtils.getCurrentSession(); tx = session.beginTransaction(); Customer customer2 = (Customer) session.get(Customer.class, 2); tx.commit(); } @SuppressWarnings("all") @Test // 将内存中的数据写到硬盘 public void demo7(){ Session session = HibernateUtils.getCurrentSession(); Transaction tx = session.beginTransaction(); List<Order> list = session.createQuery("from Order").list(); tx.commit(); } @Test // 一级缓存的更新会同步到二级缓存: public void demo6(){ Session session = HibernateUtils.getCurrentSession(); Transaction tx = session.beginTransaction(); Customer customer = (Customer) session.get(Customer.class, 1); customer.setCname("芙蓉"); tx.commit(); session = HibernateUtils.getCurrentSession(); tx = session.beginTransaction(); Customer customer2 = (Customer) session.get(Customer.class, 1); tx.commit(); } @SuppressWarnings("unchecked") @Test // iterate()方法可以查询所有信息. // iterate方法会发送N+1条SQL查询.但是会使用二级缓存的数据 public void demo5(){ Session session = HibernateUtils.getCurrentSession(); Transaction tx = session.beginTransaction(); // N+1条SQL去查询. Iterator<Customer> iterator = session.createQuery("from Customer").iterate(); while(iterator.hasNext()){ Customer customer = iterator.next(); System.out.println(customer); } tx.commit(); session = HibernateUtils.getCurrentSession(); tx = session.beginTransaction(); iterator = session.createQuery("from Customer").iterate(); while(iterator.hasNext()){ Customer customer = iterator.next(); System.out.println(customer); } tx.commit(); } @SuppressWarnings("unchecked") @Test // 查询所有.Query接口的list()方法. // list()方法会向二级缓存中放数据,但是不会使用二级缓存中的数据. public void demo4(){ Session session = HibernateUtils.getCurrentSession(); Transaction tx = session.beginTransaction(); // 查询所有客户: // list方法会向二级缓存中放入数据的. List<Customer> list = session.createQuery("from Customer").list(); for (Customer customer : list) { System.out.println(customer.getCname()); } tx.commit(); session = HibernateUtils.getCurrentSession(); tx = session.beginTransaction(); // Customer customer = (Customer) session.get(Customer.class, 1);// 没有发生SQL ,从二级缓存获取的数据. // list()方法没有使用二级缓存的数据. list = session.createQuery("from Customer").list(); for (Customer customer : list) { System.out.println(customer.getCname()); } tx.commit(); } @Test // 二级缓存的集合缓冲区特点: public void demo3(){ Session session = HibernateUtils.getCurrentSession(); Transaction tx = session.beginTransaction(); Customer customer = (Customer) session.get(Customer.class, 1); // 查询客户的订单. System.out.println("订单的数量:"+customer.getOrders().size()); tx.commit(); session = HibernateUtils.getCurrentSession(); tx = session.beginTransaction(); Customer customer2 = (Customer) session.get(Customer.class, 1); // 查询客户的订单. System.out.println("订单的数量:"+customer2.getOrders().size()); tx.commit(); } @SuppressWarnings("unused") @Test // 配置二级缓存的情况 public void demo2(){ Session session = HibernateUtils.getCurrentSession(); Transaction tx = session.beginTransaction(); Customer customer1 = (Customer) session.get(Customer.class, 1);// 发送SQL. Customer customer2 = (Customer) session.get(Customer.class, 1);// 不发送SQL. System.out.println(customer1 == customer2); tx.commit(); session = HibernateUtils.getCurrentSession(); tx = session.beginTransaction(); Customer customer3 = (Customer) session.get(Customer.class, 1);// 不发送SQL. Customer customer4 = (Customer) session.get(Customer.class, 1);// 不发送SQL. System.out.println(customer3 == customer4); tx.commit(); } @SuppressWarnings("unused") @Test // 没有配置二级缓存的情况 public void demo1(){ Session session = HibernateUtils.getCurrentSession(); Transaction tx = session.beginTransaction(); Customer customer1 = (Customer) session.get(Customer.class, 1);// 发送SQL. Customer customer2 = (Customer) session.get(Customer.class, 1);// 不发送SQL. tx.commit(); session = HibernateUtils.getCurrentSession(); tx = session.beginTransaction(); Customer customer3 = (Customer) session.get(Customer.class, 1);// 发送SQL. tx.commit(); } }
Special recommendation:"php programmer toolbox ”V0.1 version download
2. 3.The above is the detailed content of Detailed explanation of second-level cache in java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










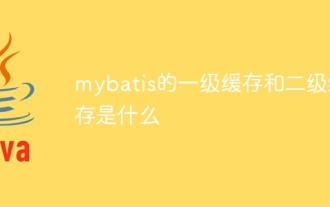
Mybatis's first-level cache is enabled by default and is at the SqlSession level. This means that multiple queries in the same SqlSession will take advantage of this level of caching. The first-level cache mainly stores query results. When executing a query operation, MyBatis will store the mapping relationship between the mapping statement and the query result, as well as the query result data in the cache. The second-level cache of mybatis is different from the first-level cache. The second-level cache is shared throughout the application, unlike the first-level cache in each SqlSession and so on.

Analysis of MyBatis' caching mechanism: The difference and application of first-level cache and second-level cache In the MyBatis framework, caching is a very important feature that can effectively improve the performance of database operations. Among them, first-level cache and second-level cache are two commonly used caching mechanisms in MyBatis. This article will analyze the differences and applications of first-level cache and second-level cache in detail, and provide specific code examples to illustrate. 1. Level 1 Cache Level 1 cache is also called local cache. It is enabled by default and cannot be turned off. The first level cache is SqlSes
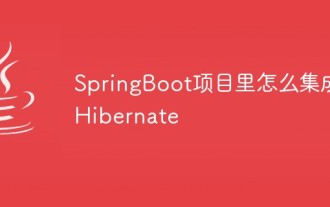
Integrating Hibernate in SpringBoot Project Preface Hibernate is a popular ORM (Object Relational Mapping) framework that can map Java objects to database tables to facilitate persistence operations. In the SpringBoot project, integrating Hibernate can help us perform database operations more easily. This article will introduce how to integrate Hibernate in the SpringBoot project and provide corresponding examples. 1.Introduce dependenciesIntroduce the following dependencies in the pom.xml file: org.springframework.bootspring-boot-starter-data-jpam
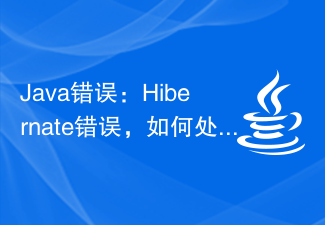
Java is an object-oriented programming language that is widely used in the field of software development. Hibernate is a popular Java persistence framework that provides a simple and efficient way to manage the persistence of Java objects. However, Hibernate errors are often encountered during the development process, and these errors may cause the program to terminate abnormally or become unstable. How to handle and avoid Hibernate errors has become a skill that Java developers must master. This article will introduce some common Hib
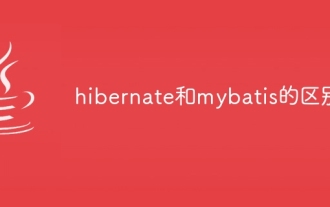
The differences between hibernate and mybatis: 1. Implementation method; 2. Performance; 3. Comparison of object management; 4. Caching mechanism. Detailed introduction: 1. Implementation method, Hibernate is a complete object/relational mapping solution that maps objects to database tables, while MyBatis requires developers to manually write SQL statements and ResultMap; 2. Performance, Hibernate is possible in terms of development speed Faster than MyBatis because Hibernate simplifies the DAO layer and so on.
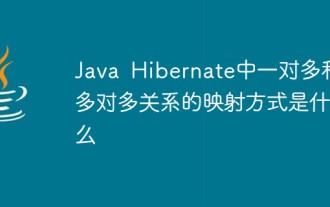
Hibernate's one-to-many and many-to-many Hibernate is an excellent ORM framework that simplifies data access between Java applications and relational databases. In Hibernate, we can use one-to-many and many-to-many relationships to handle complex data models. Hibernate's one-to-many In Hibernate, a one-to-many relationship means that one entity class corresponds to multiple other entity classes. For example, an order can correspond to multiple order items (OrderItem), and a user (User) can correspond to multiple orders (Order). To implement a one-to-many relationship in Hibernate, you need to define a collection attribute in the entity class to store
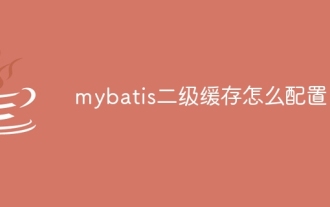
The steps for mybatis secondary cache configuration: 1. Enable the secondary cache; 2. Configure the secondary cache; 3. Specify the concurrency level of the cache; 4. Use the secondary cache; 5. Clear the secondary cache. MyBatis provides a second-level cache function to improve query performance. The second-level cache is a cache that spans multiple SQL Sessions. It can reduce the number of accesses to the database and improve application performance. When using the second-level cache, you need to pay attention to thread safety issues to ensure that multiple threads do not modify the same data at the same time.
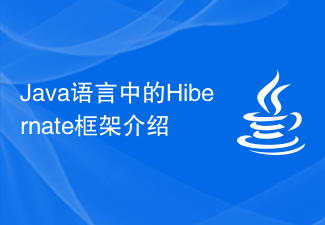
Hibernate is an open source ORM framework that binds the data mapping between relational databases and Java programs to each other, making it easier for developers to access data in the database. Using the Hibernate framework can greatly reduce the work of writing SQL statements and improve the development efficiency and reusability of applications. Let's introduce the Hibernate framework from the following aspects. 1. Advantages of the Hibernate framework: object-relational mapping, hiding database access details, making development
