


Share an example tutorial on connecting Java to MongoDB for addition, deletion, modification and query
This article mainly introduces the relevant information about Java connection MongoDB to perform additions, deletions, modifications and queries. Friends in need can refer to
Java connection to MongoDB for additions, deletions, modifications and queries. Operations
1. Create a database connection and perform addition, deletion, modification and query
(interface and implementation class respectively)
package com.dao; import java.util.List; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.data.mongodb.core.MongoTemplate; import org.springframework.data.mongodb.core.query.Criteria; import org.springframework.data.mongodb.core.query.Query; import org.springframework.stereotype.Repository; import com.bean.Company; @Repository public class RepositoryImpl implements AbstractRepository { @Autowired private MongoTemplate mongoTemplate; // 查询所有数据 public List<?> findAll(Class<?> entity) { return mongoTemplate.findAll(entity); } // 更新数据 public Company findOne(String id, Class<?> entity) { return (Company) mongoTemplate.findOne(new Query(Criteria.where("id") .is(id)), entity); } // 添加到数据库 public void updateEntity(Company company) { mongoTemplate.save(company); } // 删除选中的数据 public void delete(String id, Class<Company> class1) { Criteria criteria = Criteria.where("id").in(id); if (criteria != null) { Query query = new Query(criteria); if (query != null && mongoTemplate.findOne(query, class1) != null) mongoTemplate.remove(mongoTemplate.findOne(query, class1)); } } //增加到数据库 public void insert(Company company) { mongoTemplate.insert(company); } }
package com.dao; import java.util.List; import com.bean.Company; public interface AbstractRepository { public List<?> findAll(Class<?> entity); public Company findOne(String id,Class<?> entity); public void updateEntity(Company company); public void delete(String id, Class<Company> class1); public void insert(Company company); }
Summary: It is the same as the connection of relational database, there is no difference.
【Related Recommendations】
1. Notes on MongoDB Java connection pool
2. MongoDB (6) java Operate mongodb add, delete, modify and query
3. Share an example tutorial on using Spring Boot to develop Restful programs
4. Detailed explanation of examples of using Elasticsearch in spring Tutorial
The above is the detailed content of Share an example tutorial on connecting Java to MongoDB for addition, deletion, modification and query. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics











AI can help optimize the use of Composer. Specific methods include: 1. Dependency management optimization: AI analyzes dependencies, recommends the best version combination, and reduces conflicts. 2. Automated code generation: AI generates composer.json files that conform to best practices. 3. Improve code quality: AI detects potential problems, provides optimization suggestions, and improves code quality. These methods are implemented through machine learning and natural language processing technologies to help developers improve efficiency and code quality.
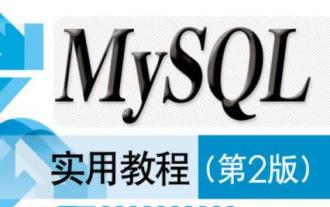
MySQL functions can be used for data processing and calculation. 1. Basic usage includes string processing, date calculation and mathematical operations. 2. Advanced usage involves combining multiple functions to implement complex operations. 3. Performance optimization requires avoiding the use of functions in the WHERE clause and using GROUPBY and temporary tables.
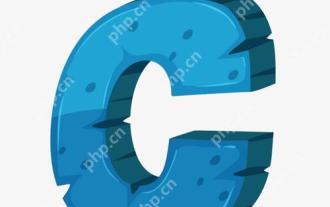
typetraits are used in C for compile-time type checking and operation, improving code flexibility and type safety. 1) Type judgment is performed through std::is_integral and std::is_floating_point to achieve efficient type checking and output. 2) Use std::is_trivially_copyable to optimize vector copy and select different copy strategies according to the type. 3) Pay attention to compile-time decision-making, type safety, performance optimization and code complexity. Reasonable use of typetraits can greatly improve code quality.
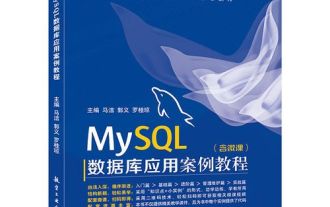
Methods for configuring character sets and collations in MySQL include: 1. Setting the character sets and collations at the server level: SETNAMES'utf8'; SETCHARACTERSETutf8; SETCOLLATION_CONNECTION='utf8_general_ci'; 2. Create a database that uses specific character sets and collations: CREATEDATABASEexample_dbCHARACTERSETutf8COLLATEutf8_general_ci; 3. Specify character sets and collations when creating a table: CREATETABLEexample_table(idINT
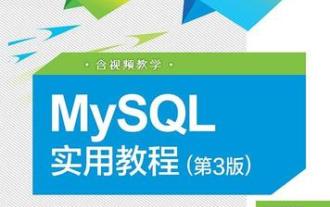
Renaming a database in MySQL requires indirect methods. The steps are as follows: 1. Create a new database; 2. Use mysqldump to export the old database; 3. Import the data into the new database; 4. Delete the old database.
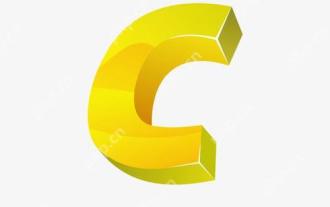
Implementing singleton pattern in C can ensure that there is only one instance of the class through static member variables and static member functions. The specific steps include: 1. Use a private constructor and delete the copy constructor and assignment operator to prevent external direct instantiation. 2. Provide a global access point through the static method getInstance to ensure that only one instance is created. 3. For thread safety, double check lock mode can be used. 4. Use smart pointers such as std::shared_ptr to avoid memory leakage. 5. For high-performance requirements, static local variables can be implemented. It should be noted that singleton pattern can lead to abuse of global state, and it is recommended to use it with caution and consider alternatives.

Java is suitable for developing cross-server web applications. 1) Java's "write once, run everywhere" philosophy makes its code run on any platform that supports JVM. 2) Java has a rich ecosystem, including tools such as Spring and Hibernate, to simplify the development process. 3) Java performs excellently in performance and security, providing efficient memory management and strong security guarantees.
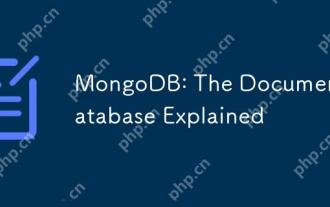
MongoDB is a NoSQL database that is suitable for handling large amounts of unstructured data. 1) It uses documents and collections to store data. Documents are similar to JSON objects and collections are similar to SQL tables. 2) MongoDB realizes efficient data operations through B-tree indexing and sharding. 3) Basic operations include connecting, inserting and querying documents; advanced operations such as aggregated pipelines can perform complex data processing. 4) Common errors include improper handling of ObjectId and improper use of indexes. 5) Performance optimization includes index optimization, sharding, read-write separation and data modeling.
