


require.js in-depth understanding of require.js features_javascript class library
Now, Require.js is my favorite way to program Javascript. It breaks the code into smaller pieces and makes it easier to manage. The Require.js Optimizer can help us disperse a larger application into multiple smaller applications, connect them through dependencies, and finally merge them during compilation and packaging. These reasons lead us to use require.js.
So, let’s take a look at the awesome features of require.js!
Compatible with CommonJS
AMD (Asynchronous Module Definition Specification) emerged from the CommonJS working group. CommonJS aims to create a Javascript ecosystem. An important part of CommonJS is transport/c, the predecessor of AMD, and require.js is an implementation of this specification.
The syntax difference between the CommonJS module and the AMD module is mainly due to the fact that AMD needs to support the asynchronous features of the browser. The CommonJS module needs to be executed synchronously, for example:
var someModule = require( "someModule" );
var anotherModule = require( "anotherModule" );
exports.asplode = function() {
SomeModule.doTehAwesome();
AnotherModule.doMoarAwesome();
};
AMD modules load modules asynchronously, so the module definition requires an array as the first parameter, and the callback function after the module is loaded is passed in as the second parameter.
define( [ "someModule"], function( someModule ) {
Return {
asplode: function() {
someModule.doTehAwesome();
// This will be executed asynchronously
require( [ "anotherModule" ], function( anotherModule ) {
anotherModule.doMoarAwesome();
});
}
};
});
However, AMD is also compatible with CommonJS syntax in require.js. By wrapping the CommonJS module with AMD's define function, you can also have a CommonJS module in AMD, for example:
define(function(require, exports, module)
var someModule = require( "someModule" );
var anotherModule = require( "anotherModule" );
SomeModule.doTehAwesome();
AnotherModule.doMoarAwesome();
exports.asplode = function() {
someModule.doTehAwesome();
anotherModule.doMoarAwesome();
};
});
In fact, require.js interprets the module content of the callback function through the function .toString, finds its correct dependencies, and turns it into a normal AMD module. It should be noted that if you write a module in this way, it may be incompatible with other AMD loaders, because it violates the AMD specification, but it can understand the writing of this format very well.
What's happening here is that require.js actually does the function.toString callback function to parse the contents of the module and find the correct dependencies, just like it would if it were a normal AMD module. It's important to note that if you choose to write modules this way, they will most likely be incompatible with other AMD module loaders, as this goes against AMD specifications, but it's good to know that this format exists!
CDN fallback
Another hidden gem of require.js is that it supports falling back to loading the local corresponding library when the CDN is not loaded correctly. We can achieve this through require.config:
requirejs.config({
Paths: {
jquery: [
'//cdnjs.cloudflare.com/ajax/libs/jquery/2.0.0/jquery.min.js',
'lib/jquery'
]
}
});
No dependencies? Object literal? no problem!
When you write a module without any dependencies and just return an object containing some functional functions, then we can use a simple syntax:
define({
forceChoke: function() {
},
forceLighting: function() {
},
forceRun: function() {
}
});
Very simple and useful if the module is just a collection of functions, or just a data package.
Circular dependencies
In some cases, we may need module moduleA and the functions in moduleA need to depend on some applications. This is circular dependency.
// js/app/moduleA.js
define([ "require", "app/app"],
function(require, app) {
return {
foo: function(title) {
var app = require( "app/app" );
return app.something();
}
}
}
);
Get the module’s address
If you need to get the address of the module, you can do this...
var path = require.toUrl("./style.css");
BaseUrl
Usually, when performing unit testing, your source code may be placed in a folder similar to src, and at the same time, your tests may be placed in a folder similar to tests. This can be difficult to get the test configuration right.
For example, we have an index.html file in the tests folder and need to load tests/spec/*.js locally. And assuming, all source code is in src/js/*.js, and there is a main.js in that folder.
In index.html, do not set data-main when loading require.js.
<script><br> require( [ "../src/js/main.js" ], function() {<br> require.config({<br> baseUrl: "../src/js/"<br> });<br> <br> require([ <br> "./spec/test.spec.js",<br> "./spec/moar.spec.js"<br> ], function() {<br> // start your test framework<br> });<br> });<br> </script>
You can find main.js loaded. However, since data-main is not set, we need to specify a baseUrl. When using data-main, baseUrl will be automatically set according to the file it is set to.
Here, you can see main.js being loaded. However, since it does not load data in the main script tag, you must specify a base. When the data is primarily for baseURL it is inferred from the location in the main file. By customizing baseUrl we can easily store test code and application code separately.
JSONP
We can handle JSONP terminal like this:
require( [
"http://someapi.com/foo?callback=define"
], function (data) {
console.log(data);
});
For non-AMD libraries, use shim to solve
Under many requests, we need to use non-AMD libraries. For example, Backbone and Underscore are not adapted to AMD specifications. And jQuery actually just defines itself as a global variable named jQuery, so there is nothing to do with jQuery.
Fortunately, we can use shim configuration to solve this problem.
require.config({
Paths: {
"backbone": "vendor/backbone",
"underscore": "vendor/underscore"
},
shim: {
“backbone”: {
deps: [ "underscore" ],
Exports: "Backbone"
},
"underscore": {
Exports: "_"
}
}
});

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
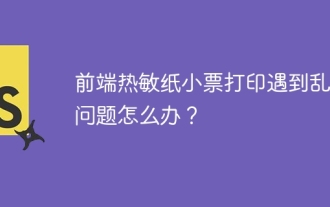
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
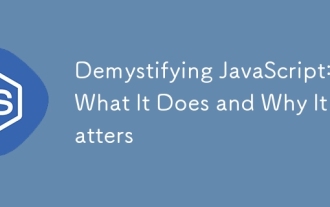
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
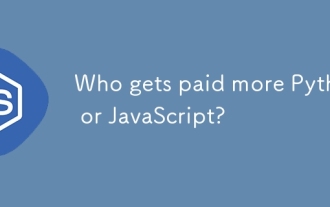
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
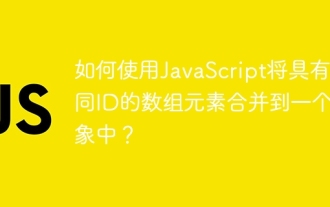
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
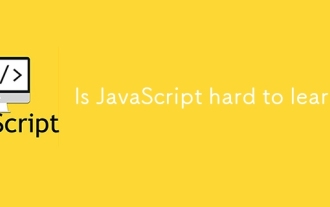
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
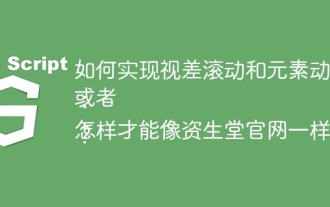
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
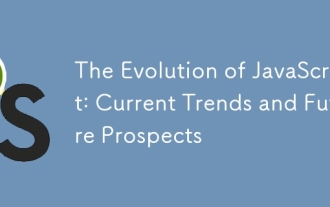
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
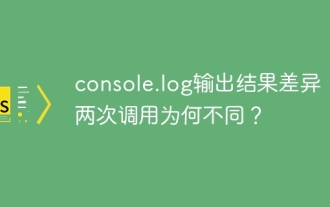
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
