


Examples of encapsulation of touch events in javascript mobile device web development_javascript skills
On touch screen devices, some basic gestures require secondary encapsulation of touch events.
zepto is a highly used class library on mobile terminals, but some events simulated by its touch module have some compatibility issues. For example, tap events have event penetration bugs on some Android devices, and other types There are also more or less compatibility issues in the events.
So, I simply encapsulated these commonly used gesture events myself. Since there are not many real devices to test, there may be some compatibility issues. The following code is only for iOS 7 and Andorid 4. The test passes in some common browsers.
tap event
The tap event is equivalent to the click effect in PC browsers. Although the click event is still available on touch screen devices, on many devices, there will be some delay in the click. If you want a quick response to the "click" event, you need This is achieved with the help of touch events.
var startTx, startTy;
element.addEventListener( 'touchstart', function( e ){
var touches = e.touches[0];
startTx = touches.clientX;
startTy = touches.clientY;
}, false );
element.addEventListener( 'touchend', function( e ){
var touches = e.changedTouches[0],
endTx = touches.clientX,
endTy = touches.clientY;
// On some devices, touch events are more sensitive, causing the event coordinates to change slightly when pressing and releasing your finger.
if( Math.abs(startTx - endTx) < 6 && Math.abs (startTy - endTy) < 6 ){
console.log( 'fire tap event' );
}
}, false );
doubleTap event
The doubleTap event is an event triggered when a finger taps the screen twice within the same position range and within a very short period of time. In some browsers, the doubleTap event will select text. If you do not want to select text, you can add the user-select:none css attribute to the element.
var isTouchEnd = false,
lastTime = 0,
lastTx = null,
lastTy = null,
firstTouchEnd = true,
body = document.body,
dTapTimer, startTx, startTy, startTime;
element.addEventListener( 'touchstart', function( e ){
if( dTapTimer ){
clearTimeout( dTapTimer );
dTapTimer = null;
}
var touches = e.touches[0];
startTx = touches.clientX;
startTy = touches.clientY;
}, false );
element.addEventListener( 'touchend', function( e ){
var touches = e.changedTouches[0],
endTx = touches.clientX,
endTy = touches.clientY,
now = Date.now(),
duration = now - lastTime;
// First make sure that a single tap event can be triggered
if( Math.abs(startTx - endTx) < 6 && Math.abs(startTx - endTx) < 6 ){
// The interval between two taps must be within 500 milliseconds
if( duration < 301 ){
// A certain range of error is allowed between this tap position and the previous tap position
if( lastTx !== null &&
Math.abs(lastTx - endTx) < 45 &&
Math.abs(lastTy - endTy) < 45 ){
firstTouchEnd = true;
lastTx = lastTy = null;
console.log( 'fire double tap event' );
}
}
else{
lastTx = endTx ;
lastTy = endTy;
}
}
else{
firstTouchEnd = true;
lastTx = lastTy = null;
}
lastTime = now;
}, false );
// On iOS Safari, if your finger taps the screen too fast,
// there is a certain chance that the touchstart and touchend events will not respond the second time
// At the same time, the finger taps for a long time touch does not trigger click
if( ~navigator.userAgent.toLowerCase().indexOf('iphone os') ){
body.addEventListener( 'touchstart', function( e ){
startTime = Date.now();
}, true );
body.addEventListener( 'touchend', function( e ){
var noLongTap = Date.now() - startTime < 501;
if( firstTouchEnd ){
firstTouchEnd = false;
if( noLongTap && e.target === element ){
dTapTimer = setTimeout(function(){
firstTouchEnd = true;
lastTx = lastTy = null; }
else{
firstTouchEnd = true ;
}
}, true );
// On iOS, the click event will not be triggered when the finger taps the screen multiple times too fast
element.addEventListener( 'click', function( e ){
if( dTapTimer ){
dTapTimer = null;
firstTouchEnd = true;
}
}, false );
}
longTap event
The longTap event is an event triggered when a finger presses the screen for a long time without moving.
Copy code
var startTx, startTy, lTapTimer;
element.addEventListener( 'touchstart', function( e ){
if( lTapTimer ){
clearTimeout( lTapTimer );
lTapTimer = null;
}
var touches = e.touches[0];
startTx = touches.clientX;
startTy = touches.clientY;
lTapTimer = setTimeout(function(){
console.log( 'fire long tap event' );
}, 1000 );
e.preventDefault();
}, false );
element.addEventListener( 'touchmove', function( e ){
var touches = e.touches[0],
endTx = touches.clientX,
endTy = touches.clientY;
if( lTapTimer && (Math.abs(endTx - startTx) > 5 || Math.abs(endTy - startTy) > 5) ){
clearTimeout( lTapTimer );
lTapTimer = null;
}
}, false );
element.addEventListener( 'touchend', function( e ){
if( lTapTimer ){
clearTimeout( lTapTimer );
lTapTimer = null;
}
}, false );
swipe事件
swipe 事件是当手指在屏幕上滑动后触发的事件,根据手指滑动的方向又分为 swipeLeft (向左)、swipeRight (向右)、swipeUp (向上)、swipeDown (向下)。
var isTouchMove, startTx, startTy;
element.addEventListener( 'touchstart', function( e ){
var touches = e.touches[0];
startTx = touches.clientX;
startTy = touches.clientY;
isTouchMove = false;
}, false );
element.addEventListener( 'touchmove', function( e ){
isTouchMove = true;
e.preventDefault();
}, false );
element.addEventListener( 'touchend', function( e ){
if( !isTouchMove ){
return;
}
var touches = e.changedTouches[0],
endTx = touches.clientX,
endTy = touches.clientY,
distanceX = startTx - endTx
distanceY = startTy - endTy,
isSwipe = false;
if( Math.abs(distanceX) >= Math.abs(distanceY) ){
if( distanceX > 20 ){
console.log( 'fire swipe left event' );
isSwipe = true;
}
else if( distanceX < -20 ){
console.log( 'fire swipe right event' );
isSwipe = true;
}
}
else{
if( distanceY > 20 ){
console.log( 'fire swipe up event' );
isSwipe = true;
}
else if( distanceY < -20 ){
console.log( 'fire swipe down event' );
isSwipe = true;
}
}
if( isSwipe ){
console.log( 'fire swipe event' );
}
}, false );
上面模拟的事件都封装在 MonoEvent 中了。完整代码地址:https://github.com/chenmnkken/monoevent,需要的朋友看看吧~

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










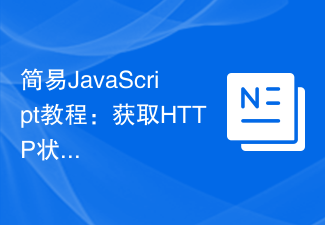
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest
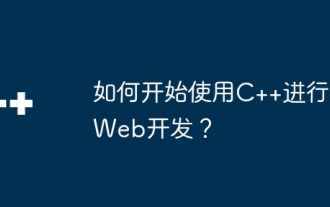
To use C++ for web development, you need to use frameworks that support C++ web application development, such as Boost.ASIO, Beast, and cpp-netlib. In the development environment, you need to install a C++ compiler, text editor or IDE, and web framework. Create a web server, for example using Boost.ASIO. Handle user requests, including parsing HTTP requests, generating responses, and sending them back to the client. HTTP requests can be parsed using the Beast library. Finally, a simple web application can be developed, such as using the cpp-netlib library to create a REST API, implementing endpoints that handle HTTP GET and POST requests, and using J
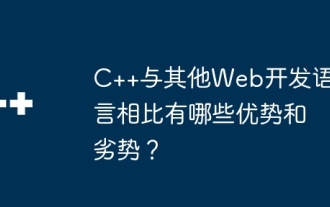
The advantages of C++ in web development include speed, performance, and low-level access, while limitations include a steep learning curve and memory management requirements. When choosing a web development language, developers should consider the advantages and limitations of C++ based on application needs.
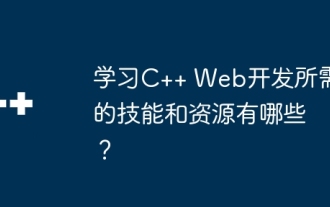
C++ Web development requires mastering the basics of C++ programming, network protocols, and database knowledge. Necessary resources include web frameworks such as cppcms and Pistache, database connectors such as cppdb and pqxx, and auxiliary tools such as CMake, g++, and Wireshark. By learning practical cases, such as creating a simple HTTP server, you can start your C++ Web development journey.

HTML, CSS and JavaScript are the three pillars of web development. 1. HTML defines the web page structure and uses tags such as, etc. 2. CSS controls the web page style, using selectors and attributes such as color, font-size, etc. 3. JavaScript realizes dynamic effects and interaction, through event monitoring and DOM operations.

PHP remains important in modern web development, especially in content management and e-commerce platforms. 1) PHP has a rich ecosystem and strong framework support, such as Laravel and Symfony. 2) Performance optimization can be achieved through OPcache and Nginx. 3) PHP8.0 introduces JIT compiler to improve performance. 4) Cloud-native applications are deployed through Docker and Kubernetes to improve flexibility and scalability.
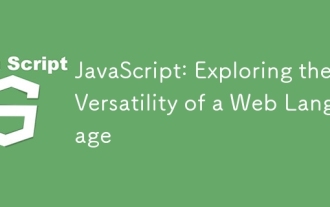
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.

Bootstrap is an open source front-end framework, and its main function is to help developers quickly build responsive websites. 1) It provides predefined CSS classes and JavaScript plug-ins to facilitate the implementation of complex UI effects. 2) The working principle of Bootstrap relies on its CSS and JavaScript components to realize responsive design through media queries. 3) Examples of usage include basic usage, such as creating buttons, and advanced usage, such as custom styles. 4) Common errors include misspelling of class names and incorrectly introducing files. It is recommended to use browser developer tools to debug. 5) Performance optimization can be achieved through custom build tools, best practices include predefined using semantic HTML and Bootstrap
