Detailed Example of JS Singleton Pattern_Basic Knowledge
What is a singleton?
A singleton requires a class to have one and only one instance, providing a global access point. Therefore, it has to bypass the regular controller so that it can only have one instance for the user to use, and the user does not care about how many instances there are, so this is the designer's responsibility
In JavaScript, Singletons serve as a shared resource namespace which isolate implementation code from the global namespace so as to provide a single point of access for functions.
In JavaScript, a singleton is treated as a global namespace, providing a point of access to the object.
Usage scenarios
In practice, the Singleton pattern is useful when exactly one object is needed to coordinate others across a system.
Analogy
A single case is somewhat similar to the team leader of a group. There is only one team leader for a period of time, and the team leader designates the work of the team members, assigns and coordinates the work of the team members.
Example 1: This is the simplest singleton, which stores attributes and methods in the form of key and value
B:function(el){
},
C:function(el){
},
D:function(el ){
},
E:function(el){
}
}
var instance;
function init() {
//Private methods and variables
function privateMethod(){
console.log( "I am private" );}
var privateVariable = "Im also private";
// Shared methods and variables
publicMethod: function () {
console.log( "The public can see me!" ); },
publicProperty: "I am also public"
};
};
// If the instance does not exist, create one
getInstance: function () {
if ( !instance ) {
instance = init();
return instance;
}
};
var singleA = mySingleton;
var singleB = mySingleton;
console.log( singleA === singleB ); // true

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
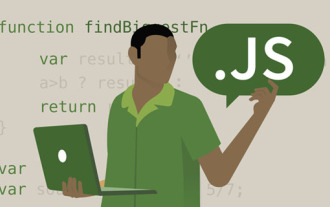
The JS singleton pattern is a commonly used design pattern that ensures that a class has only one instance. This mode is mainly used to manage global variables to avoid naming conflicts and repeated loading. It can also reduce memory usage and improve code maintainability and scalability.
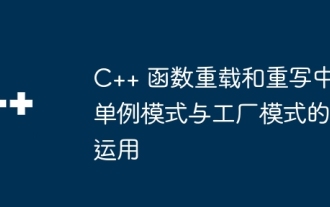
Singleton pattern: Provide singleton instances with different parameters through function overloading. Factory pattern: Create different types of objects through function rewriting to decouple the creation process from specific product classes.
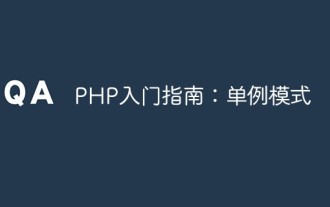
In software development, we often encounter situations where multiple objects need to access the same resource. In order to avoid resource conflicts and improve program efficiency, we can use design patterns. Among them, the singleton pattern is a commonly used way to create objects, which ensures that a class has only one instance and provides global access. This article will introduce how to use PHP to implement the singleton pattern and provide some best practice suggestions. 1. What is the singleton mode? The singleton mode is a commonly used way to create objects. Its characteristic is to ensure that a class has only one instance and provides
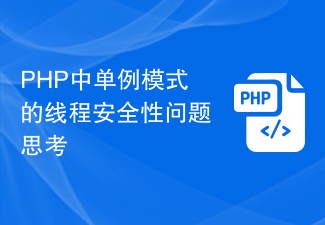
Thinking about thread safety issues of singleton mode in PHP In PHP programming, singleton mode is a commonly used design pattern. It can ensure that a class has only one instance and provide a global access point to access this instance. However, when using the singleton pattern in a multi-threaded environment, thread safety issues need to be considered. The most basic implementation of the singleton pattern includes a private constructor, a private static variable, and a public static method. The specific code is as follows: classSingleton{pr
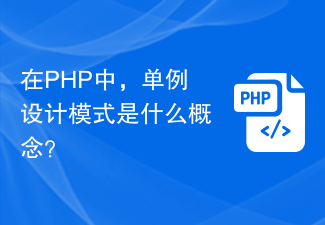
The Singleton pattern ensures that a class has only one instance and provides a global access point. It ensures that only one object is available and under control in the application. The Singleton pattern provides a way to access its unique object directly without instantiating the object of the class. Example<?php classdatabase{ publicstatic$connection; privatefunc
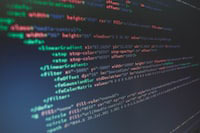
Introduction PHP design patterns are a set of proven solutions to common challenges in software development. By following these patterns, developers can create elegant, robust, and maintainable code. They help developers follow SOLID principles (single responsibility, open-closed, Liskov replacement, interface isolation and dependency inversion), thereby improving code readability, maintainability and scalability. Types of Design Patterns There are many different design patterns, each with its own unique purpose and advantages. Here are some of the most commonly used PHP design patterns: Singleton pattern: Ensures that a class has only one instance and provides a way to access this instance globally. Factory Pattern: Creates an object without specifying its exact class. It allows developers to conditionally
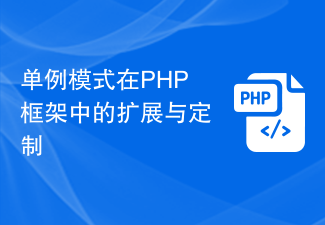
Extension and customization of singleton mode in PHP framework [Introduction] Singleton mode is a common design pattern, which ensures that a class can only be instantiated once in the entire application. In PHP development, the singleton pattern is widely used, especially in the development and expansion of frameworks. This article will introduce how to extend and customize the singleton pattern in the PHP framework and provide specific code examples. [What is the singleton pattern] The singleton pattern means that a class can only have one object instance and provides a global access point for external use. In PHP development, pass
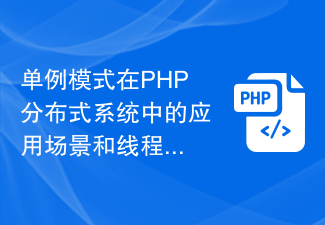
Application scenarios and thread safety processes of singleton mode in PHP distributed systems Introduction: With the rapid development of the Internet, distributed systems have become a hot topic in modern software development. In distributed systems, thread safety has always been an important issue. In PHP development, the singleton pattern is a commonly used design pattern, which can effectively solve the problems of resource sharing and thread safety. This article will focus on the application scenarios and thread safety processes of the singleton pattern in PHP distributed systems, and provide specific code examples. 1. Singleton mode
