Introduction to for..in loop traps in JavaScript_javascript tips
Everyone knows that JavaScript provides two ways to iterate objects:
(1) for loop;
(2) for..in loop;
Use for loop to iterate array objects. I think everyone has already It's commonplace. However, when using for..in loops, everyone should pay attention. Why do you say this? Everyone, listen to me...
Javascript provides a special loop (that is, the for..in loop), which is used to iterate the properties of an object or each element of an array, for...in The loop counter in the loop is a string, not a number. It contains the name of the current property or the index of the current array element.
Case 1:
//Use for.. in loop traverses the object attributes
varperson={
name: "Admin",
age: 21,
address: "shandong"
};
for(vari in person){
console.log(i);
}
The execution result is:
name
age
address
When traversing an object, the variable i means that the loop counter is the attribute name of the object
// Use for..in loop to traverse the array
vararray = ["admin","manager","db"]
for(vari in array){
console.log(i);
}
Execution result:
0
1
2
When traversing an array, the variable i, which is the loop counter, is the index of the current array element
Case 2:
However, it seems that the for.. in loop is quite useful now, but don’t be too happy too early. Take a look at the following example:
var array =["admin","manager","db"];
//Add a prototype to Array name attribute
Array.prototype.name= "zhangsan";
for(var i in array){
alert(array[i]);
}
Running results:
admin
manager
db
zhangsan
Hey, it’s a wonder, how come zhangsan appears for no reason
Now, let’s see what happens when using a for loop?
vararray = ["admin","manager"," db"];
//Add a name attribute to the prototype of Array
Array.prototype.name = "zhangsan";
for(var i =0 ; i
};
Run result:
admin
manager
db
Oh, now I understand, for. The .in loop will traverse the methods and properties in the prototype of a certain type, so this may cause unexpected errors in the code. In order to avoid this problem, we can use the object's hasOwnProperty() method to avoid this problem. If the object's properties or methods are non-inherited, then the hasOwnProperty() method returns true. That is, the check here does not involve properties and methods inherited from other objects, but only properties created directly in the specific object itself.
Case 3:
vararray = ["admin" ,"manager","db"];
Array.prototype.name= "zhangshan";
for(vari in array){
//If it is not an attribute directly created by the object itself (that is, The property // is an attribute in the prototype), then skip the display
if(!array.hasOwnProperty(i)){
continue;
}
alert(array[i]);
}
Run results:
admin
manager
db
Everything is as good as before. Hey, I don’t know, what do comrades feel after reading this? Doesn’t it feel like “the clouds and mist are parted to see the sunny sky”, haha

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
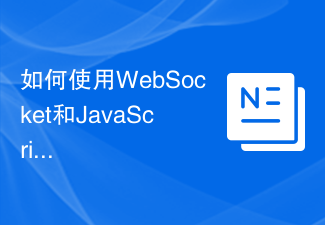
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
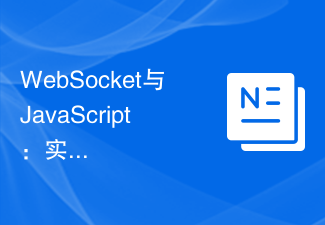
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
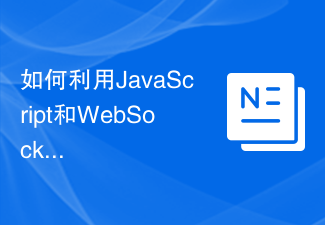
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
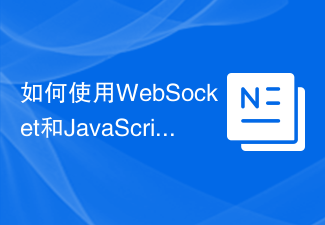
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
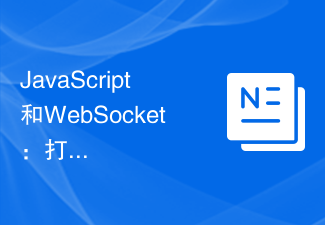
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
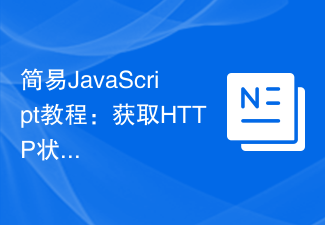
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
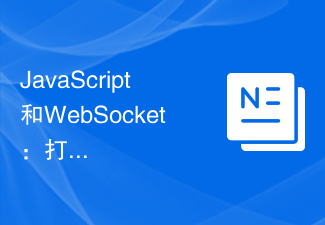
JavaScript is a programming language widely used in web development, while WebSocket is a network protocol used for real-time communication. Combining the powerful functions of the two, we can create an efficient real-time image processing system. This article will introduce how to implement this system using JavaScript and WebSocket, and provide specific code examples. First, we need to clarify the requirements and goals of the real-time image processing system. Suppose we have a camera device that can collect real-time image data
