


Introduction to the differences between JS special functions (Function() constructor, function literal)_Basic knowledge
A function is declared in this way: keyword function, function name, a set of parameters, and the code to be executed in parentheses.
There are three types of construction syntax for functions:
Js code
1.function functionName(arg0, arg1, ... argN) { statements }//function statement
2.var function_name = new Function(arg1, arg2, ..., argN, function_body); //Function() constructor
3.var func = function(arg0, arg1, ... argN) { statements };//Function direct quantity
Example:
Js Code
1.function f(x){return x* x};//function statement
2.var f = new Function("x","return x*x;");//Function() constructor
3.var f = function(x) {return x*x;};//Function literal
If the function has no clear return value, or calls a return statement without parameters, then the value it actually returns is undefined.
Function() constructor
Function is actually a fully functional object. The Function class can represent any function defined by the developer. The syntax for creating a function directly using the Function class is as follows:
var function_name = new function(arg1, arg2, ..., argN, function_body)
In the above form, each arg is a parameter, and the last one The argument is the body of the function (the code to be executed). These parameters must be strings.
var sayHi = new Function("sName", "sMessage", "alert('Hello ' sName sMessage);");
sayHi("jzj,", "Hello!");//Hello jzj, hello!
The function name is just a variable pointing to the function, so can the function be passed as a parameter to another function? The answer is yes, please see:
Js code
function callAnotherFunc(fnFunction, vArgument) {
fnFunction(vArgument);
}
var doAdd = new Function("iNum", "alert(iNum 10)");
callAnotherFunc(doAdd, 10); //Output "20"
Note: Although you can use the Function constructor to create functions, it is best not to use it because defining functions with it is much slower than using the traditional way. However, all functions should be considered instances of the Function class.
If the function you define has no parameters, you can just pass a string (that is, the body of the function) to the constructor.
Note: None of the parameters passed to the constructor Function() specify the name of the function it is creating. Unnamed functions created with the Function() constructor are sometimes called "anonymous functions". The
Function() function allows us to dynamically build and compile a function without limiting us to the precompiled function body of the function statement.
Function literal
A function literal is an expression that can define an anonymous function. The syntax of a function literal is very similar to that of a function statement, except that it is used as an expression rather than a statement, and there is no need to specify a function name. Syntax:
Js code
var func = function( arg0, arg1, ... argN) { statements };//Function literal
Although function literal creates an unnamed function, its syntax also stipulates that it can specify a function name. This is very useful when writing recursive functions that call themselves, for example:
Js code
var f = function fact(x) {
if (x <= 1) {
return 1;
} else {
return x * fact(x - 1);
}
};
Note: It does not actually create a function named fact(), it just allows the function body to refer to itself by this name. Versions of JavaScript prior to 1.5 did not implement this named function literal correctly.
•Function reference
The function name has no real meaning. It is just the name of the variable used to save the function. You can assign this function to other variables and it will still start in the same way. Function:
Js code
function square(x){return x*x;}
var a = square;
var b = a(5);//b is 25
This is a bit like a function pointer in C.
The difference between Function() constructor and function literal
The difference between Function() constructor and function literal is that the function created using constructor Function() does not use Lexical scope, on the contrary, they are always compiled by top-level functions, such as:
Js code
var y = "global";
function constructFunction() {
var y = "local";
//Function() constructor
return new Function("return y;");//Do not use local scope
}
function constFunction() {
var y = "local";
//Function literal
var f = function () {
return y;//Use local scope
};
return f;
}
//Display global because the Function() constructor returns The function does not use the local scope
alert(constructFunction()());
//Display global, because the function returns the function directly and uses the local scope
alert(constFunction()()) ;

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



Function means function. It is a reusable code block with specific functions. It is one of the basic components of a program. It can accept input parameters, perform specific operations, and return results. Its purpose is to encapsulate a reusable block of code. code to improve code reusability and maintainability.
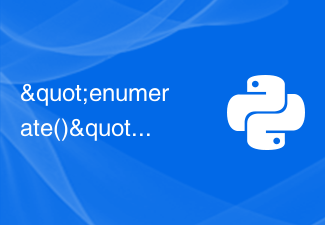
In this article, we will learn about enumerate() function and the purpose of “enumerate()” function in Python. What is the enumerate() function? Python's enumerate() function accepts a data collection as a parameter and returns an enumeration object. Enumeration objects are returned as key-value pairs. The key is the index corresponding to each item, and the value is the items. Syntax enumerate(iterable,start) Parameters iterable - The passed in data collection can be returned as an enumeration object, called iterablestart - As the name suggests, the starting index of the enumeration object is defined by start. if we ignore
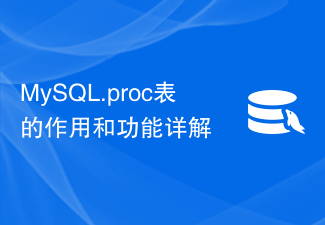
Detailed explanation of the role and function of the MySQL.proc table. MySQL is a popular relational database management system. When developers use MySQL, they often involve the creation and management of stored procedures (StoredProcedure). The MySQL.proc table is a very important system table. It stores information related to all stored procedures in the database, including the name, definition, parameters, etc. of the stored procedures. In this article, we will explain in detail the role and functionality of the MySQL.proc table
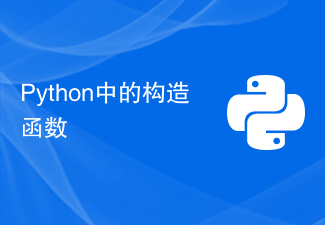
In Python, every class has a constructor, which is a special method specified inside the class. The constructor/initializer will be called automatically when a new object is created for the class. When an object is initialized, the constructor assigns values to data members in the class. There is no need to define the constructor explicitly. But in order to create a constructor, we need to follow the following rules - For a class, it is allowed to have only one constructor. The constructor name must be __init__. Constructors must be defined using instance properties (just specify the self keyword as the first argument). It cannot return any value except None. Syntax classA():def__init__(self):pass Example Consider the following example and
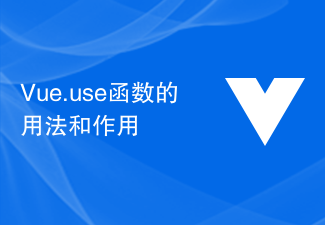
Usage and Function of Vue.use Function Vue is a popular front-end framework that provides many useful features and functions. One of them is the Vue.use function, which allows us to use plugins in Vue applications. This article will introduce the usage and function of the Vue.use function and provide some code examples. The basic usage of the Vue.use function is very simple, just call it before Vue is instantiated, passing in the plugin you want to use as a parameter. Here is a simple example: //Introduce and use the plug-in

The file_exists method checks whether a file or directory exists. It accepts as argument the path of the file or directory to be checked. Here's what it's used for - it's useful when you need to know if a file exists before processing it. This way, when creating a new file, you can use this function to know if the file already exists. Syntax file_exists($file_path) Parameters file_path - Set the path of the file or directory to be checked for existence. Required. Return file_exists() method returns. Returns TrueFalse if the file or directory exists, if the file or directory does not exist Example let us see a check for "candidate.txt" file and even if the file
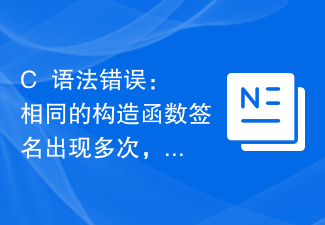
C++ is a powerful programming language, but it is inevitable to encounter various problems during use. Among them, the same constructor signature appearing multiple times is a common syntax error. This article explains the causes and solutions to this error. 1. Cause of the error In C++, the constructor is used to initialize the data members of the object when creating the object. However, if the same constructor signature is defined in the same class (that is, the parameter types and order are the same), the compiler cannot determine which constructor to call, causing a compilation error. For example,
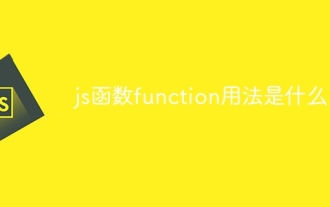
The usage of js function function is: 1. Declare function; 2. Call function; 3. Function parameters; 4. Function return value; 5. Anonymous function; 6. Function as parameter; 7. Function scope; 8. Recursive function.
