var dataObjCloned=JSON.parse(JSON.stringify( dataObj ))
I saw this on Dacheng Xiaopang’s Weibo last night. I was very interested and marked it.
Today I sorted out the information and analyzed why deep cloning of pure data json objects can be achieved in one sentence.
1.JSON.stringify function
Convert JavaScript value to JavaScript Object Notation (Json) string.
JSON.stringify(value [, replacer] [, space])
Parameters
value
Required. The JavaScript value to convert (usually an object or array).
replacer
Optional. A function or array to convert the result.
If replacer is a function, JSON.stringify will call the function, passing in the key and value of each member. Use the return value instead of the original value. If this function returns undefined, the member is excluded. The key of the root object is an empty string: "".
If replacer is an array, only members with key values in the array will be converted. Members are converted in the same order as the keys in the array. When the value argument is also an array, the replacer array is ignored.
space
Optional. Adds indentation, whitespace, and newlines to the return value JSON text to make it easier to read.
If space is omitted, the return value text will be generated without any extra whitespace.
If space is a number, the return value text is indented by the specified number of spaces at each level. If space is greater than 10, the text is indented by 10 spaces.
If space is a non-empty string (e.g. " "), the return value text is the number of characters in the string to be indented at each level.
If space is a string longer than 10 characters, the first 10 characters are used.
Return value
A string containing JSON text.
From the above introduction, you can see that this function converts an object or array into a json string.
2.JSON.parse function
Convert JavaScript Object Notation (Json) string to object.
JSON.parse(text [, reviver])
Parameters
text
Required. A valid JSON string.
reviver
Optional. A function that converts the result. This function will be called for each member of the object. If a member contains nested objects, the nested objects are converted before the parent object. For each member, the following happens:
• If reviver returns a valid value, the member value is replaced with the converted value.
• If the reviver returns the same value it received, the member value is not modified.
• If reviver returns null or undefined, delete the member.
Return value
An object or array.
As can be seen from the above introduction, this function converts a json string into an object or array.
3. Example Clone of array:
var obj = [1,2,[3,4,5]];
var objCloned = JSON.parse(JSON.stringify(obj));
console.log(obj );
console.log(JSON.stringify(obj));
console.log(objCloned);
objCloned[0] = 6;
console.log(obj);
console.log(objCloned);
Experimental results:
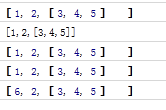
From the above results, we found that an array was indeed deeply cloned.
Clone of object:
var obj = {name:'rey',info:{location:'beijing',age:'28'}};
var objCloned = JSON.parse(JSON.stringify(obj));
console .log(obj);
console.log(JSON.stringify(obj));
console.log(objCloned);
console.log(JSON.stringify(objCloned));
objCloned .name = 'luopan';
console.log(obj);
console.log(JSON.stringify(obj));
console.log(objCloned);
console.log(JSON .stringify(objCloned));
Experimental results:
From the above experiment, we found that this method can also clone objects.
4. But all the above experiments are for pure data, that is to say, this method is only effective in arrays or object clones of pure data.
Experiments with impure data:
var obj = {name:'rey',info:{location:'beijing',age:'28'},hello:function(){console.log('hello world!');}};
var objCloned = JSON.parse(JSON.stringify(obj));
console.log(obj);
console.log(JSON.stringify(obj));
console.log(objCloned );
console.log(JSON.stringify(objCloned));
objCloned.name = 'luopan';
console.log(obj);
console.log(JSON.stringify(obj ));
console.log(objCloned);
console.log(JSON.stringify(objCloned));
Experimental results:
From the above experimental results, it can be seen that the function of non-pure data cannot participate in the conversion, and it is "despised".
So, this one-sentence deep cloning method is only for pure data. This is something that needs to be paid attention to during development.