


Ext.get() and Ext.query() are used in combination to achieve the most flexible way of fetching elements_jquery
May 16, 2016 pm 06:01 PMI have omitted the parameters for get() and query() written earlier. Let’s take a look at the function prototype in the document first:
Ext.get( Mixed el ) : Element
Parameters:
el : Mixed
The id of the node, a DOM Node or an existing Element.
Returns:
Element
The Element object
Ext.query( String path, [Node root] ) : Array
Parameters:
path : String
The selector/xpath query
root : Node
(optional) The start of the query (defaults to document).
Returns:
Array
The query function actually returns an array of DOM Node, and the parameter el of Ext.get can be a DOM Node, haha, do you understand? That is to say, to achieve the most flexible retrieval method, you should use query to obtain the DOM Node and then hand it to get to turn it into an Element. That is:
var x=Ext.query(QueryStr);
//Why don’t I write it as an inline function? Because x here can only be one element, and x in the above sentence is an Array, you can convert and process it yourself
var y=Ext.get(x);
Then the format of QueryStr needs to be introduced next (In fact, it is very similar to the format of the selector in jQuery). As for what you can do after obtaining the Element, you can read the description of Ext.Element in the ExtJS document for yourself. I will not extract it.
First give me an html code for demonstration purposes
<html>
<body>
<div id="bar" class="foo">
I'm a div ==> my id: bar, my class: foo
<span class="bar">I'm a span within the div with a foo class</span>
<a href="http://www.extjs.com" target ="_blank">An ExtJs link</a>
</div>
<div id="foo" class="bar">
my id: foo, my class: bar
<p>I'm a P tag within the foo div</p>
<span class="bar">I'm a span within the div with a bar class</span> ;
<a href="#">An internal link</a>
</div>
<div name="BlueLotus7">BlueLotus7@126.com</div> ;
</body>
</hmlt>
(1) Take according to the tag: // This query will return an array with two elements because the query selects the entire All span tags of the document.
Ext.query("span");
// This query will return an array with one element because the query takes into account the id foo.
Ext.query("span", "foo"); // This will return an array with one element, the content is the p tag under the div tag
Ext.query("div p");
// This will return an array with two elements, the content is the span tag under the div tag
Ext.query("div span"); (2) Based on ID: // This query will return the content containing our foo div an array of elements!
Ext.query("#foo"); //Or directly Ext.get("foo");(3) Get it according to the name of the class: Ext.query(".foo");//This query Will return an array of 5 elements.
Ext.query("*[class]"); // Result: [body#ext-gen2.ext-gecko, div#bar.foo, span.bar, div#foo.bar, span.bar] (4) Universal method to get: (You can use this method to get by id, name, class, css, etc.) // This will get all the elements whose class is equal to "bar"
Ext.query("*[class=bar" ]");
// This will get all elements whose class is not equal to "bar"
Ext.query("*[class!=bar]");
// This will get the class from " All elements starting with b"
Ext.query("*[class^=b]");
//This will get all elements whose class ends with "r"
Ext.query( "*[class$=r]");
//This will get all elements that extract the "a" character in class
Ext.query("*[class*=a]");// This will get all elements with name equal to "BlueLotus7"
Ext.query("*[name=BlueLotus7]");
Let's change the html code:
<html>
<head>
</head>
<body>
<div id="bar" class="foo" style="color:red;">
I am a div ==> My id is: bar, my class: foo
<span class="bar" style="color:pink;">I'm a span within the div with a foo class</span>
<a href="http://www .extjs.com" target="_blank" style="color:yellow;">An ExtJs link with a blank target!</a>
</div>
<div id=" foo" class="bar" style="color:fushia;">
my id: foo, my class: bar
<p>I'm a P tag within the foo div</p>
<span class="bar" style="color:brown;">I'm a span within the div with a bar class</span>
<a href="#" style= "color:green;">An internal link</a>
</div>
</body>
</html>
// Get all red elements
Ext.query("*{color=red}"); // [div#bar.foo]
// Get all pink elements with red color Elements of child elements
Ext.query("*{color=red} *{color=pink}"); // [span.bar]
// Get all elements that are not red text
Ext .query("*{color!=red}"); // [html, head, script firebug.js, link, body#ext-gen2.ext-gecko, script ext-base.js, script ext-core. js, span.bar, a www.extjs.com, div#foo.bar, p, span.bar, a test.html#]
// Get all elements whose color attribute starts from "yel"
Ext.query("*{color^=yel}"); // [a www.extjs.com]
// Get all elements whose color attributes end with "ow"
Ext.query( "*{color$=ow}"); // [a www.extjs.com]
// Get all elements whose color attribute contains the "ow" character
Ext.query("*{color*= ow}"); // [a www.extjs.com, span.bar]
(5) Change the pseudo operator method to html:
<html>
<head>
</head>
<body>
< ;div id="bar" class="foo" style="color:red; border: 2px dotted red; margin:5px; padding:5px;">
I am a div ==> my id It's bar, and my class is foo
<span class="bar" style="color:pink;"> Here is the span element, and the outer div element has the class attribute of foo</span>
<a href="http://www.extjs.com" target="_blank" style="color:yellow;">Set blank=target ExtJS link</a>
< /div>
<div id="foo" class="bar" style="color:fushia; border: 2px dotted black; margin:5px; padding:5px;">
The id here is : foo, the class here is the p element surrounded by bar
<p> "foo" div. </p>
<span class="bar" style="color:brown;">Here is a span element, surrounded by divs on the outer layer, and span also has a class attribute of bar. </span>
<a href="#" style="color:green;">Built-in link</a>
</div>
<div style=" border:2px dotted pink; margin:5px; padding:5px;">
<ul>
<li>Item #1</li>
<li>Item #2</ li>
<li>Item #3</li>
<li>Item #4 with <a href="#">Link</a></li>
</ul>
<table style="border:1px dotted black;">
<tr style="color:pink">
<td>First line , the first column</td>
<td>The first row, the second column</td>
</tr>
<tr style="color:brown"> ;
<td colspan="2">The second row, cells have been merged! </td>
</tr>
<tr>
<td>Third row, first column</td>
<td>Third row, Second column</td>
</tr>
</table>
</div>
<div style="border:2px dotted red; margin:5px ; padding:5px;">
<form>
<input id="chked" type="checkbox" checked/><label for="chked">clicked</ label>
<br /><br />
<input id="notChked" type="checkbox" /><label for="notChked">not me brotha! </label>
</form>
</div>
</body>
</html>
//The SPAN element is the first child element of its parent element
Ext.query("span:first-child"); // [span.bar]
//The A element is its parent The last child element of the element
Ext.query("a:last-child") // [a www.extjs.com, a test.html#]
//The SPAN element is the third of its parent element 2 child elements (number starting from 1)
Ext.query("span:nth-child(2)") // [span.bar]
//TR element is an odd number of its parent element Number of child elements
Ext.query("tr:nth-child(odd)") // [tr, tr]
//LI element is an odd number of child elements of its parent element
Ext.query("li:nth-child(even)") // [li, li]
//Returns the A element, which is the only child element of its parent element
Ext.query("a :only-child") // [a test.html#]
//Return all checked (checked) INPUT elements
Ext.query("input:checked") // [input#chked on ]
//Return the first TR element
Ext.query("tr:first") // [tr]
//Return the last INPUT element
Ext.query(" input:last") // [input#notChked on]
//Return the second TD element
Ext.query("td:nth(2)") // [td]
/ /Return each DIV containing the "within" string
Ext.query("div:contains(within)") // [div#bar.foo, div#foo.bar]
//Return None Contains those DIVs other than FORM sub-elements
Ext.query("div:not(form)") [div#bar.foo, div#foo.bar, div]
//Returns the A element Those DIV collections
Ext.query("div:has(a)") // [div#bar.foo, div#foo.bar, div]
//Return those TDs that will continue to have TDs gather. One thing in particular is that if TD with the colspan attribute is used, it will be ignored
Ext.query("td:next(td)") // [td, td]
//Return the element that precedes the INPUT Those LABEL element collections
Ext.query("label:prev(input)") //[label, label]

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
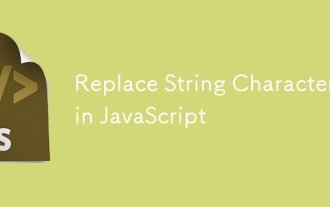
Replace String Characters in JavaScript
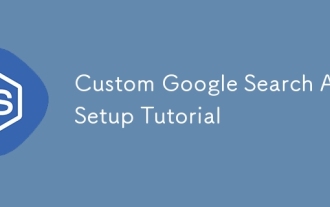
Custom Google Search API Setup Tutorial
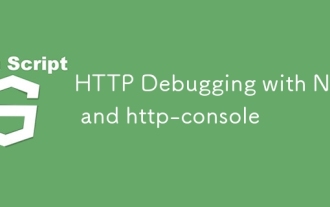
HTTP Debugging with Node and http-console
