The essence and operation method of JavaScript DOM
Although some JavaScript frameworks, such as jQuery, Prototype and MooTools, can improve our front-end development efficiency and solve browser compatibility issues well, we still need to lay a good foundation in JavaScript technology. This article will introduce the essence of JavaScript and the Document Object Model (DOM).
JavsScript is a dynamic, loosely typed, prototype-based programming language that can be used in a variety of environments. In addition to being a popular web client programming language, it can also be used for IDE plug-ins, PDF files, or even more abstract concepts for other platforms.
JavaScript is a language based on the ECMAScript standard (ECMA-262) created by Brendan Eich from Netscape. It was originally named LiveScript, but was later changed to JavaScript, which is one of the reasons why many people confuse it with Java. Here is a detailed description of some of its features:
◆Dynamic programming languages are executed at runtime; they are not compiled. Because of this, JavaScript is sometimes considered a scripting language rather than a true programming language (obviously a misunderstanding).
◆Loosely typed languages do not require a strong type system. If you use C or Java programming (unlike JavaScript) when declaring variables you know that you must declare something like 'int' (integer). The difference with JavaScript is that you don't have to specify its type.
◆In JavaScript we use prototypes to achieve effects similar to inheritance, JavaScript does not support classes.
◆JavaScript is also a functional language, which treats functions as priority objects. It is based on the concept of lambda.
Understanding the above concepts is not very relevant to learning JavaScript technology. It just gives everyone a preliminary and correct understanding of JavaScript and the essential difference between JavaScript and other programming languages.
Document Object Model
Document Object Model (Document Object Model), usually referred to as DOM, is the interface between website content and JavaScript. Since JavaScript became the most commonly used language, JavaScript and the DOM are often treated as separate entities. The DOM interface is used to access, traverse and control HTML and XML documents.
Here are some important things to know about the DOM:
◆The Window object is a global object, you just try to use "window" to access it. The Window object contains all your JavaScript code to be executed. Just like all objects contain properties and methods.
◆Attributes are variables stored under the object. All variables created in the web page will become properties of the window object.
◆Methods are functions stored under an object. While all functions are stored under the window object, you can reference them using 'methods'.
◆DOM creates a hierarchical structure relative to the Web document structure, and the hierarchy is composed of nodes. There are many different types of DOM nodes, the most important of which are 'Element', 'Text' and 'Document'.
◆The ‘Element’ node represents the element in the page, so if you have a paragraph element (‘
’) in the page, then you can access it through the DOM node.
◆The ‘Text’ node represents all the text in the page (in the element), so if there is some text content in the paragraph of the page, then you can access it through the DOM node.
◆The ‘Document’ node represents the entire document. (It is the root node of the DOM tree)
◆ Also note that element attributes are the DOM nodes themselves.
◆Different layout engines have certain differences in the execution of DOM standards. For example, the FireFox browser using the Gecko layout engine can perform well (but it does not fully comply with the W3C specification), but IE using the Trident engine is known for its many bugs and incomplete implementation of the DOM standard. Know. This is one of the pain points in front-end development.
JavaScriptScript Elements in Web Pages
When you want to use JavaScript on your website, you need to include them in a script element:
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.php.cn"> <html xmlns="http://www.php.cn"> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8" /> <title>JavaScript!</title> </head> <body> <script type="text/javascript"> // <![CDATA[ // ]]> </script> </body> </html>
As you can see below the document there is a script element. In fact, strictly speaking, the type attribute should be set to "application/javascript", but as expected it does not work properly under IE browser, so we use "text/javascript" or not use the type attribute, if you care about code W3C specification verification If so, it is recommended that you use the former ("text/javascript").
You'll also notice that within the script element we also have a pair of commented out lines of code that tell XHTML-aware browsers that the content within the script element is character data and should not be interpreted as XHTML markup. This is necessary if you plan to use the '<' or '>' characters in your JavaScript code. Of course, if you are using ordinary HTML code, you can ignore it completely.
defer attribute
The JavaScript code in our script element will be executed during the page reading process. The only exception is when the script element has the defer attribute. By default, when the browser encounters a script element, it stops and runs the code before continuing with document parsing. The defer attribute tells the browser that the code contains non-documented code and can be executed later. The only problem with it is that it is only available under IE, so we should try not to use it and just understand it.
Link external script
If you want to connect to an external script file, then you only need to add a src attribute with the file address to your script element. Separating the script file and calling it independently is indeed a better idea than inline scripts. It means that the browser can cache the file, and you don't have to worry about CDATA nonsense.
JavaScript essentials
Before we continue to talk about DOM, it is necessary to learn the basic essentials of JavaScript. If there is something you It's a little difficult to understand, but don't worry, you'll figure it out sooner or later. There are different types of values in JavaScript, they are Numeric, String, Boolean, Object, Undefined and Null. Single-line comments use two slashes (//), and everything within this line will be understood as comment content. Multi-line comments use '/*' and '*/' to complete the comment paragraph.
Numbers
In JavaScript all numeric values are represented as floating point values, remember not to use quotes when defining numeric variables.
// Note: Always use 'var' to declare variables: var leftSide = 100;
var topSide = 50;
var areaOfRectangle = leftSide * topSide; // = 5000
String
The strings you define are all It's literal, JavaScript won't handle it. A string can consist of a sequence of Unicode characters surrounded by a pair of double quotes or single quotes.
var firstPart = 'Hello'; var secondPart = 'World!';
var allOfIt = firstPart + ' ' + secondPart; // Hello World!
// + sign for string concatenation
// (It can also be used (for addition operations in mathematics)
Boolean value
The Boolean type is useful when you make conditional judgments to know whether specified criteria are met. Boolean has two possible values: true and false. The result of any comparison using logical algorithms will be a Boolean value.
5 === (3 + 2); // = true // You can declare boolean values for variables:
var veryTired = true;
// You can test like this:
if (veryTired) {
// Your code
}
The '===' you see above is a comparison operator, which we will discuss later.
Function
A function is a specialized object.
// Create a new function using function operations: function myFunctionName(arg1, arg2) {
// Here is the code of the function
}
// If you omit the function name, you create an "anonymous function" :
function(arg1, arg2) {
// Here is the code of the function
}
// To execute the function, you only need to reference it and use parentheses (with parameters):
myFunctionName(); // No parameters
myFunctionName('foo', 'bar'); // With parameters
// You can also execute the function without declaring variables
(function(){
// This is the so-called self-calling anonymous function
})();
Array
An array is also a specialized object that can contain any amount of data. To access data in an array you must use a number, which refers to its "index" in the array.
// Two different ways of declaring arrays, // Literal:
var fruit = ['apple', 'lemon', 'banana'];
// Using array constructor:
var fruit = new Array( 'apple', 'lemon', 'banana');
fruit[0]; // Access the first data item in the array (apple)
fruit[1]; // Access the second data in the array Item (lemon)
fruit[2]; // Access the third data item in the array (banana)
Object
Object is a named collection of values (key-value pairs), it is very similar to an array, unique The difference is that you can specify a name for each data value.
// Two different ways of declaring objects,
// Literal (braces):
var profile = {
name: 'Li',
age: 23,
job: 'Web Developer'
};
//Applicable object constructor:
var profile = new Object();
profile.name = 'Li';
profile.age = 23;
profile.job = 'Web Developer';
if/else statement
if The /else statement is the most common structure in JavaScript. It looks like this:
var legalDrinkingAge = 21; var yourAge = 23;
if ( yourAge >= legalDrinkingAge )

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
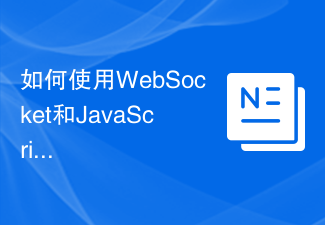
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
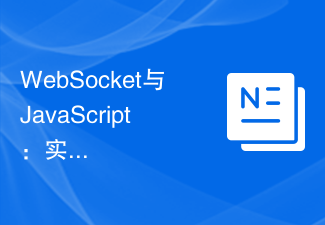
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
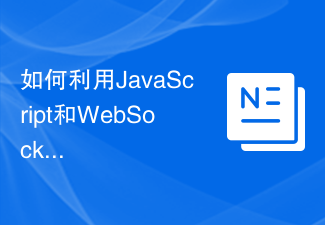
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
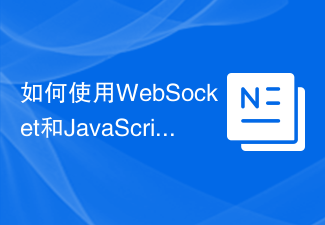
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
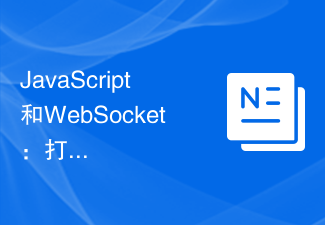
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
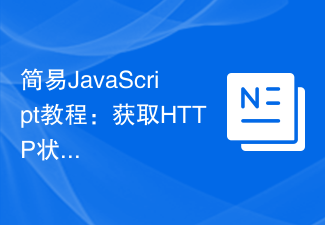
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
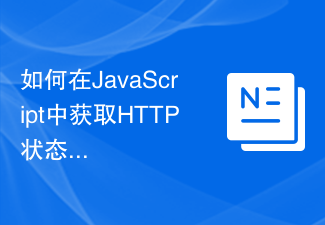
Introduction to the method of obtaining HTTP status code in JavaScript: In front-end development, we often need to deal with the interaction with the back-end interface, and HTTP status code is a very important part of it. Understanding and obtaining HTTP status codes helps us better handle the data returned by the interface. This article will introduce how to use JavaScript to obtain HTTP status codes and provide specific code examples. 1. What is HTTP status code? HTTP status code means that when the browser initiates a request to the server, the service
