


Extension function example of Array array object in JavaScript program
We often add custom extension functions to the prototypes of String, Function, and Array, such as removing string spaces, sorting arrays, etc.
Today we will focus on how to extend the Array object
1. Expand directly on Array.prototype
2. Use your own method to extend the array object
Expand directly on Array.prototype, it cannot be used directly on DOM objects (such as: nodeList obtained by document.getElementsByTagName('div'));
For those with mysophobia For students, it has also broken the original ecological environment: )
Let’s first look at some methods of yui operating arrays. Here I simply stripped and changed the source code
(function(){
var YArray;
YArray = function(o,idx,arraylike){
var t = (arraylike) ? 2 : YArray.test(o),
l, a, start = idx || 0;
if (t) {
try {
return Array .prototype.slice.call(o, start); //Use Array native method to convert atoms into JS array
} catch(e) {
a = [];
l = o.length;
for (; start< ;l; start++) {
a.push(o[start]);
}
return a;
}
} else {
return [o];
}
}
YArray.test = function(o) {
var r = 0;
if (o && (typeof o == 'object' ||typeof o == 'function')) {
if (Object.prototype.toString.call(o) === "[ object Array]") {
r = 1;
} else {
try {
if (('length' in o) && !o.tagName && !o.alert && !o.apply) {
r = 2;
}
} catch(e) {}
}
}
return r;
}
YArray.each = (Array.prototype.forEach) ? //First check whether the browser supports it, and if so, call the native
function (a, f, o) {
Array.prototype.forEach.call(a || [], f, o || Y);
return YArray;
} :
function (a, f, o) {
var l = (a && a.length) || 0, i;
for (i = 0; i < l; i=i+1) {
f.call(o || Y, a[i], i, a);
}
return YArray;
};
YArray.hash = function(k, v) {
var o = {}, l = k.length, vl = v && v.length, i;
for (i=0; i
o[k[i]] = (vl && vl > i) ? v[i] : true;
}
}
return o;
};
YArray.indexOf = (Array.prototype.indexOf) ?
function(a, val) {
return Array.prototype.indexOf.call(a, val) ;
} :
function(a, val) {
for (var i=0; i
return i;
}
}
return -1; //Cannot be found
};
YArray.numericSort = function(a, b) {
return (a - b); //Sort from small to large, return (b - a); From large to small
};
YArray.some = (Array.prototype.some) ?
function (a, f, o) {
return Array.prototype.some.call(a, f, o );
} :
function (a, f, o) {
var l = a.length, i;
for (i=0; i
return true;
}
}
return false;
};
})();
Use the Array native method to convert aguments into other JS arrays Methods (Dom objects are not allowed, only traversal)
Array.apply(null,arguments);
[].slice.call(arguments,0);
[].splice.call(arguments,0,arguments .length);
[].concat.apply([],arguments);
...
The YArray function can not only operate on array objects but also on nodeList objects
YArray(document.getElementsByTagName("div "));
Traverse the DOM object and reassemble it into an array: )
a = [];
l = o.length;
for (; start
}
return a;
YArray.each
Traverse the array, if there is a function passed in, callback will be executed for each traversal
YArray.each([1,2,3] ,function(item){
alert(item);// Executed 3 times, 1,2,3
});
YArray.hash
The array is assembled into key-value pairs and can be understood as a json object
YArray.hash(["a","b"],[1,2]);
YArray.indexOf
Returns (want to find a value) the same index value of the value in the array
YArray.indexOf([ 1,2],1)
YArray.numericSort
Sort the array from small to large
[3, 1, 2].sort(YArray.numericSort);
YArray.some
Whether any elements in the array pass callBack processing? If there is one, it will return true immediately. If there is none, it will return false
YArray.some([3, 1, 2], function(el){
return el < 4;
})
Let’s look at the javascript 1.6 -1.8 extensions to arrays and learn how to implement the same functionality
every
filter
forEach
indexOf
lastIndexOf
map
some
reduce
reduceRight
Array.prototype.every
if (!Array.prototype.every)
{
Array.prototype.every = function(fun /*, thisp*/)
{
var len = this.length >>> 0;
if (typeof fun != "function")
throw new TypeError();
var thisp = arguments[1];
for (var i = 0; i < len; i++)
{
if (i in this &&
!fun.call(thisp, this[i], i, this))
return false;
}
return true;
};
}
Has every element in the array been processed by callBack? If it is, it returns true. If one of them is not, it returns false immediately. This is very similar to the YUI some function we just mentioned:) The function is exactly the opposite. Array.prototype.filter
Array. prototype.filter = function (block /*, thisp */) { //Filter, easy to add, for judgment and filtering
var values = [];
for (var i = 0; i < this.length; i++)
if (block.call(thisp, this[i]))
values.push(this[i]);
return values;
};
Usage
var val= numbers.filter(function(t){
return t < 5 ;
alert(val);
forEach and indexOf and some can refer to the yui code above, no Let’s reiterate again
lastIndexOf and indexOf codes are similar, just traverse from the end
Now let’s talk about ‘map’
Array.prototype.map = function(fun /*, thisp*/) {
var len = this. length >>> 0;
throw new TypeError();
var res = new Array(len);
var thisp = arguments[1];
for (var i = 0; i < len; i++) {
if (i in this)
res[i] = fun.call(thisp, this[i], i, this);
}
return res;
};
Traverse the array, execute the function, iterate the array, each element is used as a parameter to execute the callBack method, the callBack method processes each element, and finally returns a processed array
var numbers = [1, 4, 9 ];
Array.prototype.reduce
Array.prototype.reduce = function(fun /*, initial*/) {
var len = this.length >>> 0;
throw new TypeError();
if (len == 0 && arguments.length == 1)
throw new TypeError();
var i = 0;
if (arguments.length >= 2) {
var rv = arguments[1];
} else {
do {
if (i in this) {
rv = this[i++];
break;
}
if (++i >= len)
throw new TypeError();
} while (true);
}
for (; i < len; i++) {
if (i in this)
rv = fun.call(null, rv, this[i], i, this);
}
return rv;
};
Let the array elements call the given function in sequence , and finally returns a value. In other words, the given function must use the return value
It means the name, from right to left
Array.prototype.reduceRight = function(fun /*, initial*/) {
var len = this.length >>> 0;
throw new TypeError();
if (len == 0 && arguments.length = = 1)
throw new TypeError();
var i = len - 1;
if (arguments.length >= 2) {
var rv = arguments[1];
} else {
do {
if (i in this) {
rv = this[i--];
break;
}
if (--i < 0)
throw new TypeError();
} while (true);
}
for (; i >= 0; i--) {
if (i in this)
rv = fun.call(null, rv, this[i], i, this);
}
return rv;
};
In addition to these, any method you want to use can be added to Array.prototype
For example, the commonly used toString
Array.prototype.toString = function () {
return this.join('');
You can also add toJson, uniq, compact, reverse, etc.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










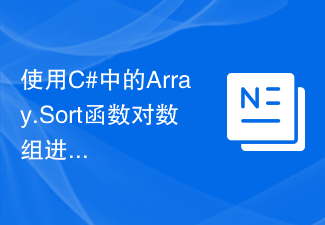
Title: Example of using the Array.Sort function to sort an array in C# Text: In C#, array is a commonly used data structure, and it is often necessary to sort the array. C# provides the Array class, which has the Sort method to conveniently sort arrays. This article will demonstrate how to use the Array.Sort function in C# to sort an array and provide specific code examples. First, we need to understand the basic usage of the Array.Sort function. Array.So
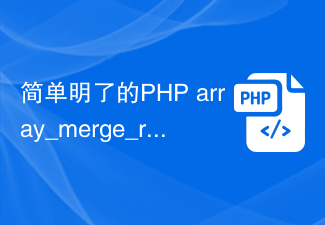
When programming in PHP, we often need to merge arrays. PHP provides the array_merge() function to complete array merging, but when the same key exists in the array, this function will overwrite the original value. In order to solve this problem, PHP also provides an array_merge_recursive() function in the language, which can merge arrays and retain the values of the same keys, making the program design more flexible. array_merge
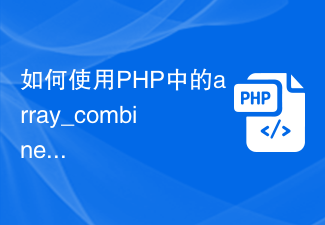
In PHP, there are many powerful array functions that can make array operations more convenient and faster. When we need to combine two arrays into an associative array, we can use PHP's array_combine function to achieve this operation. This function is actually used to combine the keys of one array as the values of another array into a new associative array. Next, we will explain how to use the array_combine function in PHP to combine two arrays into an associative array. Learn about array_comb
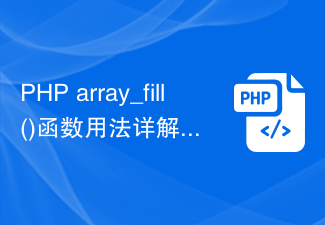
In PHP programming, array is a very important data structure that can handle large amounts of data easily. PHP provides many array-related functions, array_fill() is one of them. This article will introduce in detail the usage of the array_fill() function, as well as some tips in practical applications. 1. Overview of the array_fill() function The function of the array_fill() function is to create an array of a specified length and composed of the same values. Specifically, the syntax of this function is

In PHP programming, array is a frequently used data type. There are also quite a few array operation functions, including the array_change_key_case() function. This function can convert the case of key names in the array to facilitate our data processing. This article will introduce how to use the array_change_key_case() function in PHP. 1. Function syntax and parameters array_change_ke
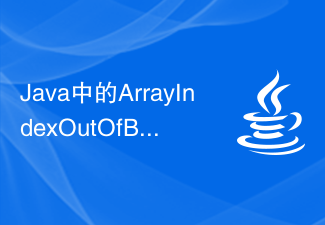
Java is a very powerful programming language that is widely used in various development fields. However, during Java programming, developers often encounter ArrayIndexOutOfBoundsException exceptions. So, what are the common causes of this anomaly? ArrayIndexOutOfBoundsException is a common runtime exception in Java. It means that when accessing data, the array subscript exceeds the range of the array. Common reasons include
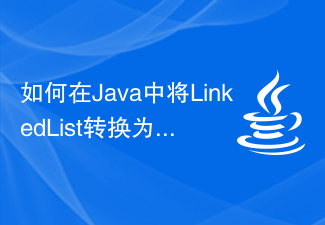
The toArray() method of the LinkedList class converts the current LinkedList object into an array of object type and returns it. This array contains all the elements in this list in correct order (from first element to last element). It acts as a bridge between array-based and collection-based APIs. So, convert LinkedList to array - instantiate LinkedList class. Populate it using the add() method. Call the toArray() method on the linked list created above and retrieve the array of objects. Converts each element of an array of objects to a string. Example Real-time demonstration of importjava.util.Arrays;importjava.uti
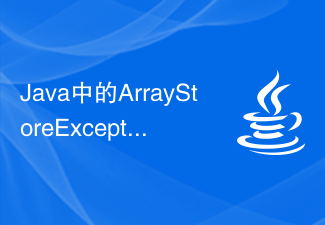
In Java development, we often use arrays to store a series of data because of the convenience and performance advantages of arrays. However, in the process of using arrays, some exceptions will occur, and one of the common exceptions is ArrayStoreException. This exception is thrown when we store incompatible data types in the array. This article will introduce what an ArrayStoreException is, why it occurs, and how to solve it. 1. Arr
