


JavaScript realizes the left and right scrolling effect of pictures [can automatically scroll, has left and right buttons]
The example in this article describes the javascript implementation of the left and right scrolling effect of images. Share it with everyone for your reference, the details are as follows:
html code:
<!doctype html> <html> <head> <meta charset="UTF-8" /> <meta http-equiv="X-UA-Compatible" content="IE=edge,chrome=1"/> <meta name="renderer" content="webkit"/> <meta name="keywords" content=""/> <meta name="description" content=""/> <title>图片滚动</title> <style> *{margin:0;padding:0;} ul{list-style:none;} img{border:0;} .scroll{width:358px;height:63px;} .scroll_left{width:23px;height:63px;background:url(images/btn_left.jpg) no-repeat;float:left;} .scroll_right{width:23px;height:63px;background:url(images/btn_right.jpg) left no-repeat;float:left;} .pic{width:312px;height:73px;float:left;} .pic ul{display:block;} .pic li{float:left;display:inline;width:104px;text-align:center;} </style> </head> <body> <p style="margin:100px auto;width:358px;"> <p> <p id="LeftArr"></p> <p id="scrollPic"> <ul> <li><a href="#" target="_blank" title=""><img src="images/pic01.png" width="100" height="63" alt="" /></a></li> <li><a href="#" target="_blank" title=""><img src="images/pic02.jpg" width="100" height="63" alt="" /></a></li> <li><a href="#" target="_blank" title=""><img src="images/pic03.jpg" width="100" height="63" alt="" /></a></li> <li><a href="#" target="_blank" title=""><img src="images/pic04.jpg" width="100" height="63" alt="" /></a></li> <li><a href="#" target="_blank" title=""><img src="images/pic05.jpg" width="100" height="63" alt="" /></a></li> <li><a href="#" target="_blank" title=""><img src="images/pic06.jpg" width="100" height="63" alt="" /></a></li> </ul> </p> <p id="RightArr"></p> </p> </p> </body> </html> <script src="scrollPic.js"></script> <script> window.onload = function(){ scrollPic(); } function scrollPic() { var scrollPic = new ScrollPic(); scrollPic.scrollContId = "scrollPic"; //内容容器ID scrollPic.arrLeftId = "LeftArr";//左箭头ID scrollPic.arrRightId = "RightArr"; //右箭头ID scrollPic.frameWidth = 312;//显示框宽度 scrollPic.pageWidth = 104; //翻页宽度 scrollPic.speed = 10; //移动速度(单位毫秒,越小越快) scrollPic.space = 10; //每次移动像素(单位px,越大越快) scrollPic.autoPlay = true; //自动播放 scrollPic.autoPlayTime = 3; //自动播放间隔时间(秒) scrollPic.initialize(); //初始化 } </script>
scrollPic.js code:
var sina = { $ : function (objName) { if (document.getElementById) { return eval('document.getElementById("' + objName + '")') } else { return eval('document.all.' + objName) } }, isIE : navigator.appVersion.indexOf("MSIE") != -1 ? true : false, addEvent : function (l, i, I) { if (l.attachEvent) { l.attachEvent("on" + i, I) } else { l.addEventListener(i, I, false) } }, delEvent : function (l, i, I) { if (l.detachEvent) { l.detachEvent("on" + i, I) } else { l.removeEventListener(i, I, false) } }, readCookie : function (O) { var o = "", l = O + "="; if (document.cookie.length > 0) { var i = document.cookie.indexOf(l); if (i != -1) { i += l.length; var I = document.cookie.indexOf(";", i); if (I == -1) I = document.cookie.length; o = unescape(document.cookie.substring(i, I)) } }; return o }, writeCookie : function (i, l, o, c) { var O = "", I = ""; if (o != null) { O = new Date((new Date).getTime() + o * 3600000); O = "; expires=" + O.toGMTString() }; if (c != null) { I = ";domain=" + c }; document.cookie = i + "=" + escape(l) + O + I }, readStyle : function (I, l) { if (I.style[l]) { return I.style[l] } else if (I.currentStyle) { return I.currentStyle[l] } else if (document.defaultView && document.defaultView.getComputedStyle) { var i = document.defaultView.getComputedStyle(I, null); return i.getPropertyValue(l) } else { return null } } }; //滚动图片构造函数 //UI&UE Dept. mengjia //080623 function ScrollPic(scrollContId, arrLeftId, arrRightId, dotListId) { this.scrollContId = scrollContId; this.arrLeftId = arrLeftId; this.arrRightId = arrRightId; this.dotListId = dotListId; this.dotClassName = "dotItem"; this.dotOnClassName = "dotItemOn"; this.dotObjArr = []; this.pageWidth = 0; this.frameWidth = 0; this.speed = 10; this.space = 10; this.pageIndex = 0; this.autoPlay = true; this.autoPlayTime = 5; var _autoTimeObj, _scrollTimeObj, _state = "ready"; this.stripDiv = document.createElement("DIV"); this.listDiv01 = document.createElement("DIV"); this.listDiv02 = document.createElement("DIV"); if (!ScrollPic.childs) { ScrollPic.childs = [] }; this.ID = ScrollPic.childs.length; ScrollPic.childs.push(this); this.initialize = function () { if (!this.scrollContId) { throw new Error("必须指定scrollContId."); return }; this.scrollContDiv = sina.$(this.scrollContId); if (!this.scrollContDiv) { throw new Error("scrollContId不是正确的对象.(scrollContId = \"" + this.scrollContId + "\")"); return }; this.scrollContDiv.style.width = this.frameWidth + "px"; this.scrollContDiv.style.overflow = "hidden"; this.listDiv01.innerHTML = this.listDiv02.innerHTML = this.scrollContDiv.innerHTML; this.scrollContDiv.innerHTML = ""; this.scrollContDiv.appendChild(this.stripDiv); this.stripDiv.appendChild(this.listDiv01); this.stripDiv.appendChild(this.listDiv02); this.stripDiv.style.overflow = "hidden"; this.stripDiv.style.zoom = "1"; this.stripDiv.style.width = "32766px"; if(-[1,]){ this.listDiv01.style.cssFloat = "left"; this.listDiv02.style.cssFloat = "left"; }else{ this.listDiv01.style.styleFloat = "left"; this.listDiv02.style.styleFloat = "left"; } sina.addEvent(this.scrollContDiv, "mouseover", Function("ScrollPic.childs[" + this.ID + "].stop()")); sina.addEvent(this.scrollContDiv, "mouseout", Function("ScrollPic.childs[" + this.ID + "].play()")); if (this.arrLeftId) { this.arrLeftObj = sina.$(this.arrLeftId); if (this.arrLeftObj) { sina.addEvent(this.arrLeftObj, "mousedown", Function("ScrollPic.childs[" + this.ID + "].rightMouseDown()")); sina.addEvent(this.arrLeftObj, "mouseup", Function("ScrollPic.childs[" + this.ID + "].rightEnd()")); sina.addEvent(this.arrLeftObj, "mouseout", Function("ScrollPic.childs[" + this.ID + "].rightEnd()")) } }; if (this.arrRightId) { this.arrRightObj = sina.$(this.arrRightId); if (this.arrRightObj) { sina.addEvent(this.arrRightObj, "mousedown", Function("ScrollPic.childs[" + this.ID + "].leftMouseDown()")); sina.addEvent(this.arrRightObj, "mouseup", Function("ScrollPic.childs[" + this.ID + "].leftEnd()")); sina.addEvent(this.arrRightObj, "mouseout", Function("ScrollPic.childs[" + this.ID + "].leftEnd()")) } }; if (this.dotListId) { this.dotListObj = sina.$(this.dotListId); if (this.dotListObj) { var pages = Math.round(this.listDiv01.offsetWidth / this.frameWidth + 0.4), i, tempObj; for (i = 0; i < pages; i++) { tempObj = document.createElement("span"); this.dotListObj.appendChild(tempObj); this.dotObjArr.push(tempObj); if (i == this.pageIndex) { tempObj.className = this.dotClassName } else { tempObj.className = this.dotOnClassName }; tempObj.title = "第" + (i + 1) + "页"; sina.addEvent(tempObj, "click", Function("ScrollPic.childs[" + this.ID + "].pageTo(" + i + ")")) } } }; if (this.autoPlay) { this.play() } }; this.leftMouseDown = function () { if (_state != "ready") { return }; _state = "floating"; _scrollTimeObj = setInterval("ScrollPic.childs[" + this.ID + "].moveLeft()", this.speed) }; this.rightMouseDown = function () { if (_state != "ready") { return }; _state = "floating"; _scrollTimeObj = setInterval("ScrollPic.childs[" + this.ID + "].moveRight()", this.speed) }; this.moveLeft = function () { if (this.scrollContDiv.scrollLeft + this.space >= this.listDiv01.scrollWidth) { this.scrollContDiv.scrollLeft = this.scrollContDiv.scrollLeft + this.space - this.listDiv01.scrollWidth } else { this.scrollContDiv.scrollLeft += this.space }; this.accountPageIndex() }; this.moveRight = function () { if (this.scrollContDiv.scrollLeft - this.space <= 0) { this.scrollContDiv.scrollLeft = this.listDiv01.scrollWidth + this.scrollContDiv.scrollLeft - this.space } else { this.scrollContDiv.scrollLeft -= this.space }; this.accountPageIndex() }; this.leftEnd = function () { if (_state != "floating") { return }; _state = "stoping"; clearInterval(_scrollTimeObj); var fill = this.pageWidth - this.scrollContDiv.scrollLeft % this.pageWidth; this.move(fill) }; this.rightEnd = function () { if (_state != "floating") { return }; _state = "stoping"; clearInterval(_scrollTimeObj); var fill = -this.scrollContDiv.scrollLeft % this.pageWidth; this.move(fill) }; this.move = function (num, quick) { var thisMove = num / 5; if (!quick) { if (thisMove > this.space) { thisMove = this.space }; if (thisMove < -this.space) { thisMove = -this.space } }; if (Math.abs(thisMove) < 1 && thisMove != 0) { thisMove = thisMove >= 0 ? 1 : -1 } else { thisMove = Math.round(thisMove) }; var temp = this.scrollContDiv.scrollLeft + thisMove; if (thisMove > 0) { if (this.scrollContDiv.scrollLeft + thisMove >= this.listDiv01.scrollWidth) { this.scrollContDiv.scrollLeft = this.scrollContDiv.scrollLeft + thisMove - this.listDiv01.scrollWidth } else { this.scrollContDiv.scrollLeft += thisMove } } else { if (this.scrollContDiv.scrollLeft - thisMove <= 0) { this.scrollContDiv.scrollLeft = this.listDiv01.scrollWidth + this.scrollContDiv.scrollLeft - thisMove } else { this.scrollContDiv.scrollLeft += thisMove } }; num -= thisMove; if (Math.abs(num) == 0) { _state = "ready"; if (this.autoPlay) { this.play() }; this.accountPageIndex(); return } else { this.accountPageIndex(); setTimeout("ScrollPic.childs[" + this.ID + "].move(" + num + "," + quick + ")", this.speed) } }; this.next = function () { if (_state != "ready") { return }; _state = "stoping"; this.move(this.pageWidth, true) }; this.play = function () { if (!this.autoPlay) { return }; clearInterval(_autoTimeObj); _autoTimeObj = setInterval("ScrollPic.childs[" + this.ID + "].next()", this.autoPlayTime * 1000) }; this.stop = function () { clearInterval(_autoTimeObj) }; this.pageTo = function (num) { if (_state != "ready") { return }; _state = "stoping"; var fill = num * this.frameWidth - this.scrollContDiv.scrollLeft; this.move(fill, true) }; this.accountPageIndex = function () { this.pageIndex = Math.round(this.scrollContDiv.scrollLeft / this.frameWidth); if (this.pageIndex > Math.round(this.listDiv01.offsetWidth / this.frameWidth + 0.4) - 1) { this.pageIndex = 0 }; var i; for (i = 0; i < this.dotObjArr.length; i++) { if (i == this.pageIndex) { this.dotObjArr[i].className = this.dotClassName } else { this.dotObjArr[i].className = this.dotOnClassName } } } };
Parameter description:
var scrollPic = new ScrollPic(); //Define variables and initialize methods
scrollContId //Scroll container ID
arrLeftId //Left button ID
arrRightId //Right button ID
frameWidth //Display frame width
pageWidth //Page turning width
speed //Moving speed (unit milliseconds, the smaller the faster)
space //Move pixels each time (unit px, the larger the faster)
autoPlay / /Autoplay
autoPlayTime //Autoplay interval (seconds)
initialize() //Initialization
I hope this article will be helpful to everyone in JavaScript programming.
For more javascript to achieve the left and right scrolling effect of images [can automatically scroll, with left and right buttons] please pay attention to the PHP Chinese website for related articles!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
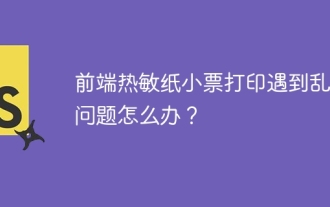
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
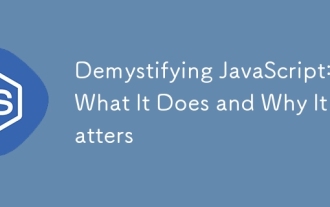
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
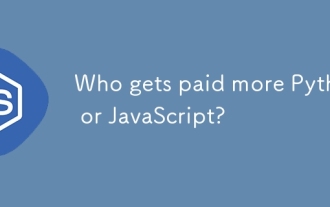
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
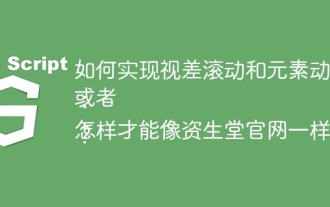
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
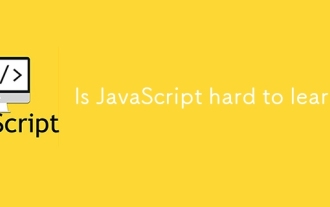
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
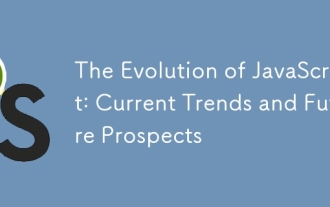
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
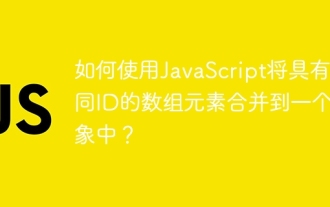
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
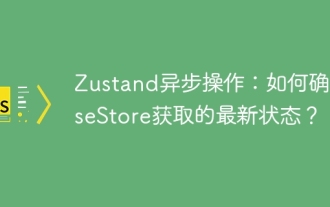
Data update problems in zustand asynchronous operations. When using the zustand state management library, you often encounter the problem of data updates that cause asynchronous operations to be untimely. �...
