


JavaScript object-oriented-implementing inheritance based on function forgery
Because there are some shortcomings in the way of implementing inheritance based on the prototype chain, people have adopted another way to implement inheritance-implementing inheritance based on function forgery. The idea of this technique is to call the parent class's constructor inside the child class's constructor.
Implementation of inheritance based on function forgery
Because in JavaScript, a function is an object that executes code in a specific environment, so we can use the call() or apply() method To execute the constructor of the parent class object on the child class object. Let’s look at the following example:
/* 创建父类 */ function Parent(){ this.color = ["red","blue"]; } /* 创建子类 */ function Child(){ // 继承父类的属性 Parent.call(this); }
In the above code, we first create a parent class Parent, then create a subclass Child, and use Parent.call(this); inside the subclass Complete inheritance.
In the article Properties of Functions, we have introduced the call() and apply() methods. The function of these two methods is to call functions in a specific scope, which means that these two methods can Call a function by its name. Here we use Parent.call(this); inside Child to complete inheritance. This sentence means calling the constructor of the parent class in the child class. This at this time refers to the Child object (in Child this should be the object that executes Child), so it is equivalent to having this.color = ["red", "blue"]; in Child, which is equivalent to having this.color attribute in Child, which is also a disguised form. The inheritance is completed.
We can verify it through the following method:
var c1 = new Child(); //创建子类对象c1 c1.color.push("Green"); //为c1添加新的颜色 console.info(c1.color); //控制台输出:red,blue,Green var c2 = new Child(); //创建子类对象c2 console.info(c2.color); //控制台输出:red,blue
In the above code, we created the subclass object c1 and added a new color "Green" to it, so it will Output in the console: "red, blue, Green". Then we created object c2. Because no new color was added to it, it will only output the color inherited from the parent class in the console: "red, blue".
Every time new Child is called, it is equivalent to performing an object attribute setting. At this time, each object has a color attribute in the space, but does not exist in the prototype, so color will not be shared. . This solves the problem of reference type variables in prototype chain inheritance.
Subclass constructor
Another disadvantage of prototype chain inheritance is that the constructor of the parent class cannot be called from the subclass, so there is no way to assign attributes in the subclass to the parent class. middle. This problem can be solved well through function forgery. Let’s look at the following example:
// 创建父类 function Parent(name){ this.name = name; } //创建子类 function Student(name,age){ //使用伪造的方式就可以把子类的构造函数参数传递到父类中 Parent.call(this,name); //调用父类的属性 this.age = age; } var s1 = new Student("Leon",22); var s2 = new Student("Ada",25); console.info(s1.name + "," + s1.age); // 控制台输出:Leon,22 console.info(s2.name + "," + s2.age); // 控制台输出:Ada,25
In the above code, the subclass Student calls the name attribute of the parent class through function forgery, which actually adds a name attribute to the subclass. Here, the call() method passes the parameter name of the Student class to the parent class, and the completed operation is equivalent to this.name = name;. And this name attribute is the name attribute of the subclass, not the name attribute of the parent class.
Problems in inheritance based on function forgery
In the above discussion, we only talked about the subclass inheriting the attributes of the parent class, so how does the subclass inherit the methods of the parent class? As we said before, we usually set the method in the prototype. For example, there is a say() method in the parent class. The code is as follows:
// 创建父类 function Parent(name){ this.name = name; } // 父类的say()方法 Parent.prototype.say = function(){ console.info(this.name); } //创建子类 function Student(name,age){ Parent.call(this,name); this.age = age; }
Because the method of using function forgery will not complete the subclass Student The prototype points to the parent class Parent, so after the subclass inherits the parent class, the say() method does not exist. The way to solve this problem is to place the say() method in the Parent and use the this keyword to create it.
// 创建父类 function Parent(name){ this.name = name; // 父类的say()方法 this.say = function(){ console.info(this.name); } } //创建子类 function Student(name,age){ Parent.call(this,name); this.age = age; }
Although doing this allows the subclass to inherit the say() method of the parent class, it creates another problem: every time a subclass object is created, a say() method will be generated, which will take up a lot of memory space.
Since there are also flaws in implementing inheritance based on function forgery, we will not use this method alone to complete inheritance, but will use a combination-based method to implement inheritance. We will discuss this in the next article Introduce this inheritance method.
The above is the content of JavaScript object-oriented - inheritance based on function forgery. For more related content, please pay attention to the PHP Chinese website (www.php.cn)!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










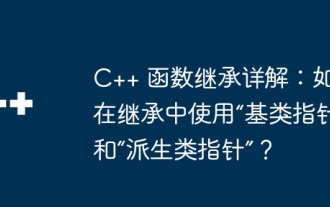
In function inheritance, use "base class pointer" and "derived class pointer" to understand the inheritance mechanism: when the base class pointer points to the derived class object, upward transformation is performed and only the base class members are accessed. When a derived class pointer points to a base class object, a downward cast is performed (unsafe) and must be used with caution.
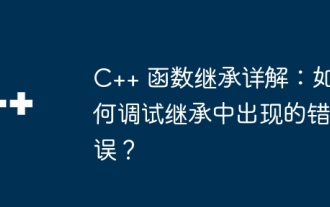
Inheritance error debugging tips: Ensure correct inheritance relationships. Use the debugger to step through the code and examine variable values. Make sure to use the virtual modifier correctly. Examine the inheritance diamond problem caused by hidden inheritance. Check for unimplemented pure virtual functions in abstract classes.
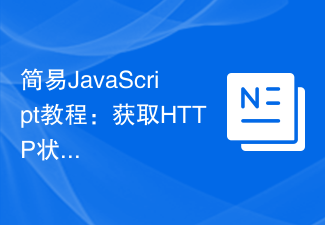
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest
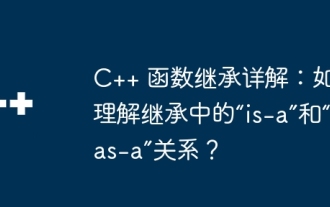
Detailed explanation of C++ function inheritance: Master the relationship between "is-a" and "has-a" What is function inheritance? Function inheritance is a technique in C++ that associates methods defined in a derived class with methods defined in a base class. It allows derived classes to access and override methods of the base class, thereby extending the functionality of the base class. "is-a" and "has-a" relationships In function inheritance, the "is-a" relationship means that the derived class is a subtype of the base class, that is, the derived class "inherits" the characteristics and behavior of the base class. The "has-a" relationship means that the derived class contains a reference or pointer to the base class object, that is, the derived class "owns" the base class object. SyntaxThe following is the syntax for how to implement function inheritance: classDerivedClass:pu
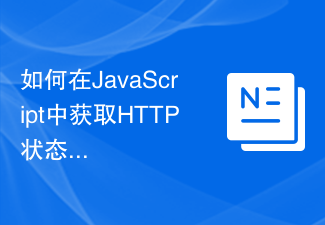
Introduction to the method of obtaining HTTP status code in JavaScript: In front-end development, we often need to deal with the interaction with the back-end interface, and HTTP status code is a very important part of it. Understanding and obtaining HTTP status codes helps us better handle the data returned by the interface. This article will introduce how to use JavaScript to obtain HTTP status codes and provide specific code examples. 1. What is HTTP status code? HTTP status code means that when the browser initiates a request to the server, the service
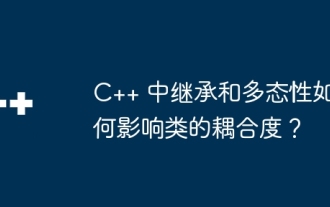
Inheritance and polymorphism affect the coupling of classes: Inheritance increases coupling because the derived class depends on the base class. Polymorphism reduces coupling because objects can respond to messages in a consistent manner through virtual functions and base class pointers. Best practices include using inheritance sparingly, defining public interfaces, avoiding adding data members to base classes, and decoupling classes through dependency injection. A practical example showing how to use polymorphism and dependency injection to reduce coupling in a bank account application.
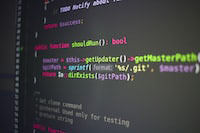
What is object-oriented programming? Object-oriented programming (OOP) is a programming paradigm that abstracts real-world entities into classes and uses objects to represent these entities. Classes define the properties and behavior of objects, and objects instantiate classes. The main advantage of OOP is that it makes code easier to understand, maintain and reuse. Basic Concepts of OOP The main concepts of OOP include classes, objects, properties and methods. A class is the blueprint of an object, which defines its properties and behavior. An object is an instance of a class and has all the properties and behaviors of the class. Properties are characteristics of an object that can store data. Methods are functions of an object that can operate on the object's data. Advantages of OOP The main advantages of OOP include: Reusability: OOP can make the code more
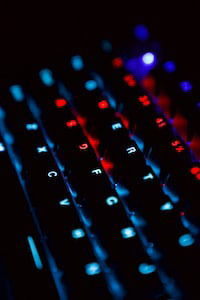
Interface: An implementationless contract interface defines a set of method signatures in Java but does not provide any concrete implementation. It acts as a contract that forces classes that implement the interface to implement its specified methods. The methods in the interface are abstract methods and have no method body. Code example: publicinterfaceAnimal{voideat();voidsleep();} Abstract class: Partially implemented blueprint An abstract class is a parent class that provides a partial implementation that can be inherited by its subclasses. Unlike interfaces, abstract classes can contain concrete implementations and abstract methods. Abstract methods are declared with the abstract keyword and must be overridden by subclasses. Code example: publicabstractcla
