JavaScript Advanced Series—Object Usage and Properties
Object as data type
Access properties
Delete properties
-
Syntax of property names
Object usage and properties
All variables in JavaScript can be used as objects, with two exceptions null and undefined .
false.toString(); // 'false' [1, 2, 3].toString(); // '1,2,3' function Foo(){} Foo.bar = 1; Foo.bar; // 1
A common misunderstanding is that numerical literals cannot be used as objects. This is due to a bug in the JavaScript parser, which attempts to parse dot operators as part of a floating-point literal value.
2.toString(); // 出错:SyntaxError
There are many workarounds to make number literals look like objects.
2..toString(); // 第二个点号可以正常解析 2 .toString(); // 注意点号前面的空格 (2).toString(); // 2先被计
Objects as data types
JavaScript objects can be used as hash tables, mainly used to save the correspondence between named keys and values.
Using the object literal syntax - {} - you can create a simple object. This newly created object inherits from Object.prototype and does not have any custom properties.
var foo = {}; // 一个空对象 // 一个新对象,拥有一个值为12的自定义属性'test' var bar = {test: 12};
Access properties
There are two ways to access the properties of an object, the dot operator or the square bracket operator.
var foo = {name: 'kitten'} foo.name; // kitten foo['name']; // kitten var get = 'name'; foo[get]; // kitten foo.1234; // SyntaxError foo['1234']; // works
The two syntaxes are equivalent, but the bracket operator is still valid in the following two situations
Dynamic setting of attributes
The attribute name is not a valid variable name (Translator's Note: For example, the attribute name contains spaces, or the attribute name is a JS keyword)
Delete attribute
The only way to delete an attribute is to use the delete operator; setting the attribute to undefined or null does not actually delete the attribute, but only removes the association between the attribute and the value.
var obj = { bar: 1, foo: 2, baz: 3 }; obj.bar = undefined; obj.foo = null; delete obj.baz; for(var i in obj) { if (obj.hasOwnProperty(i)) { console.log(i, '' + obj[i]); } }
The above output has bar undefined and foo null - only baz is actually deleted, so it disappears from the output.
Syntax of attribute names
var test = { 'case': 'I am a keyword so I must be notated as a string', delete: 'I am a keyword too so me' // 出错:SyntaxError };
The attribute names of objects can be declared using strings or ordinary characters. However, due to another incorrect design of the JavaScript parser, the second declaration method above will throw a SyntaxError before ECMAScript 5.
The reason for this error is that delete is a keyword in the JavaScript language; therefore, in order to run normally under lower versions of JavaScript engines, string literal declaration must be used.
The above is the JavaScript advanced series - object usage and attributes. For more related content, please pay attention to the PHP Chinese website (www.php.cn)!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
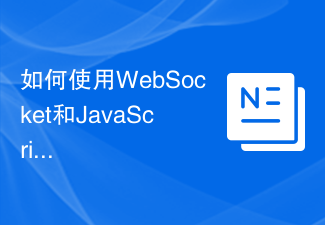
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
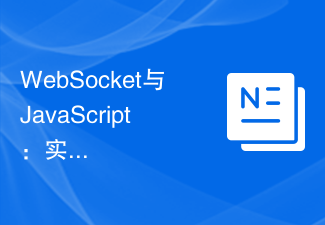
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
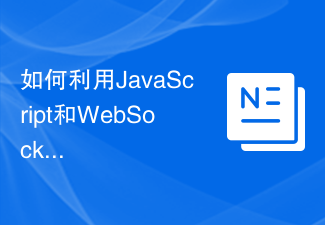
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
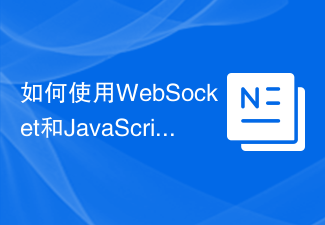
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
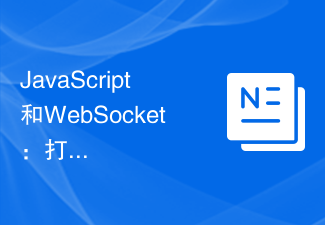
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
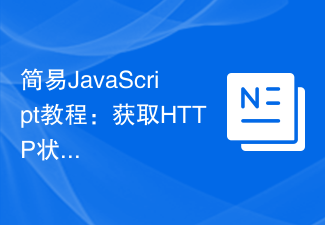
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
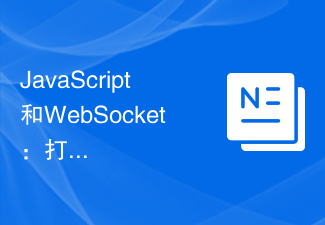
JavaScript is a programming language widely used in web development, while WebSocket is a network protocol used for real-time communication. Combining the powerful functions of the two, we can create an efficient real-time image processing system. This article will introduce how to implement this system using JavaScript and WebSocket, and provide specific code examples. First, we need to clarify the requirements and goals of the real-time image processing system. Suppose we have a camera device that can collect real-time image data
