Introduction to Javascript asynchronous programming methods
In the process of JavaScript development, we will inevitably encounter some asynchronous programming scenarios, whether it is the front-end ajax request or the various asynchronous APIs of node. The following is a summary of JavaScript asynchronous programming during the work and study process. A summary of the usage of common methods
Several methods of asynchronous programming in javascript
The asynchronous mode programming that is currently used more frequently in work has the following methods
One callback function
This is the most basic method of asynchronous programming. Suppose there are two functions f1 and f2, the latter waits for the execution result of the former
f1();f2(); 如果f1是一个很耗时的任务,可以考虑改写f1,把f2写成f1的回调函数 function f1(callback){ setTimeout(function(){ // f1的任务代码 //执行回调函数 callback() },1000)}执行代码就变成下面这样:
f1(f2);//Call
In this way, we turn synchronous operations into asynchronous operations. F1 will not block the running of the program. It is equivalent to executing the main logic of the program first and delaying the execution of time-consuming operations.
Specific Example:
Since the ajax request is asynchronous, sometimes we need to get the data after the ajax request before performing other operations. At this time, the callback function will help us solve this problem. The specific code is as follows:
import $ from 'jquery'function getData(callback){ var url="http://xxx.com/activity/v1/homepage/index"; var data={ "cityId":110100, "type":"coupon" } $.ajax({ url:url, type:'get', dataType:'jsonp', jsonp:'callback', data:data, success:function(resp){ if(resp.status==200 && resp.data){ var item = resp.data[0] && resp.data[0].coupon; if(callback){ callback(item) //执行回调函数 } } }, error:function(err){ console.log("error") } }) }function getItem(data){ if(data){ //得到数据进行处理 var url = data.moreUrl; alert(url) } } getData(getItem) //调用二 发布/订阅 模式
We assume that there is a "signal center". When a certain task is executed, it "publish" a signal to the signal center. Other tasks can "subscribe" to the signal center to know When can I start executing it myself. This is called the "publish-subscribe pattern" (publish-subscribe pattern), also known as the "observer pattern" (observer pattern).
There are many implementations of this pattern. The following is Ben Alman’s Tiny Pub/Sub, which is a plug-in for jQuery.
function PubSub(){ this.handlers = {}; } PubSub.prototype = { on:function(eventType,handler){ var self = this; if(!(eventType in self.handlers)){ self.handlers[eventType] = []; } self.handlers[eventType].push(handler); return this; }, trigger:function(eventType){ var self = this; var handlerArgs = Array.prototype.slice.call(arguments,1); for(var i=0;i<self.handlers[eventType].length;i++){ self.handlers[eventType][i].apply(self,handlerArgs) } return self; } }
Specific call:
var pubsub=new PubSub();function getData(){ var url="xxx.com/activity/v1/homepage/index"; var data={ "cityId":110100, "type":"coupon" } $.ajax({ url:url, type:'get', dataType:'jsonp', jsonp:'callback', data:data, success:function(resp){ if(resp.status==200 && resp.data){ var item = resp.data[0] && resp.data[0].coupon; pubsub.trigger('done',item) //发布事件 } }, error:function(err){ console.log("error") } }) }//订阅事件pubsub.on('done',function(data){ getItem(data) })function getItem(data){ alert('start') console.log('data='+data) if(data){ //得到数据进行处理 var url = data.moreUrl; alert(url) } } getData() //调用
Three Promise objects
The Promise object is a specification proposed by the CommonJS working group to provide a unified interface for asynchronous programming
Used in combination with async and await provided by es7, the code is as follows:
import $ from 'jquery'function getData() { return new Promise((resolve,reject) => { var url="http://xxx.com/activity/v1/homepage/index"; var data={ "cityId":110100, "type":"coupon" } $.ajax({ url:url, type:'get', dataType:'jsonp', jsonp:'callback', data:data, success:function(resp){ if(resp.status==200 && resp.data){ var item = resp.data[0] && resp.data[0].coupon; resolve(item) } }, error:function(err){ reject("error") } }) })} function getItem(data){ if(data){ //得到数据进行处理 var url = data.moreUrl; alert(url) }} const testAsync = async () => { var data = await getData(); getItem(data); } //调用testAsync();
The above is the detailed content of Introduction to Javascript asynchronous programming methods. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
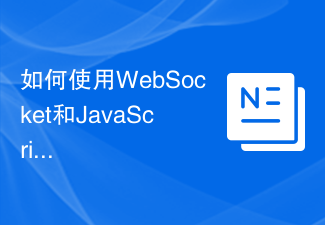
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
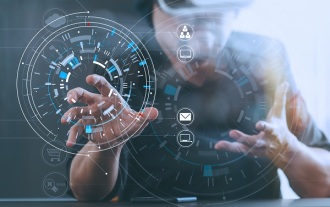
Face detection and recognition technology is already a relatively mature and widely used technology. Currently, the most widely used Internet application language is JS. Implementing face detection and recognition on the Web front-end has advantages and disadvantages compared to back-end face recognition. Advantages include reducing network interaction and real-time recognition, which greatly shortens user waiting time and improves user experience; disadvantages include: being limited by model size, the accuracy is also limited. How to use js to implement face detection on the web? In order to implement face recognition on the Web, you need to be familiar with related programming languages and technologies, such as JavaScript, HTML, CSS, WebRTC, etc. At the same time, you also need to master relevant computer vision and artificial intelligence technologies. It is worth noting that due to the design of the Web side
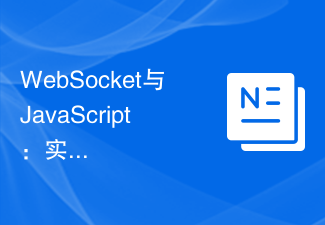
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
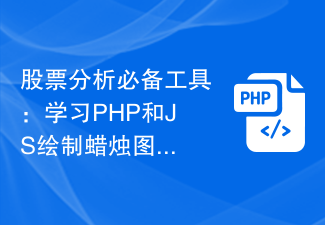
Essential tools for stock analysis: Learn the steps to draw candle charts in PHP and JS. Specific code examples are required. With the rapid development of the Internet and technology, stock trading has become one of the important ways for many investors. Stock analysis is an important part of investor decision-making, and candle charts are widely used in technical analysis. Learning how to draw candle charts using PHP and JS will provide investors with more intuitive information to help them make better decisions. A candlestick chart is a technical chart that displays stock prices in the form of candlesticks. It shows the stock price
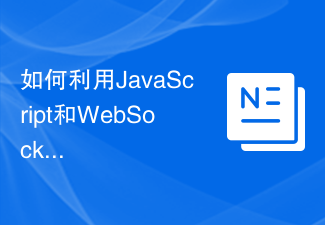
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
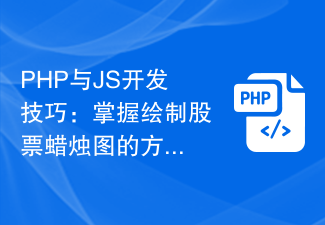
With the rapid development of Internet finance, stock investment has become the choice of more and more people. In stock trading, candle charts are a commonly used technical analysis method. It can show the changing trend of stock prices and help investors make more accurate decisions. This article will introduce the development skills of PHP and JS, lead readers to understand how to draw stock candle charts, and provide specific code examples. 1. Understanding Stock Candle Charts Before introducing how to draw stock candle charts, we first need to understand what a candle chart is. Candlestick charts were developed by the Japanese
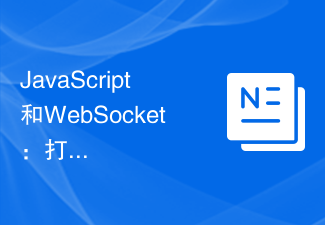
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
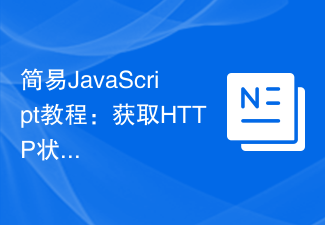
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest
