


Difficulties in JavaScript: Detailed explanation of prototype and constructor binding examples
JavaScript objects and constructors
Define a JavaScript object like this
var a = { x : 1, y : 2, add : function () { return this.x + this.y; }, mul : function () { return this.x * this.y; } }
In this way, you A variable a is defined. In addition to the two public members x and y, this variable also has two functions (public methods) add and mul. However, there are two disadvantages of this definition method:
1. It is very inconvenient to generate objects in batches. If you var b=a; then every time you modify the members of b, the members of a will be changed at the same time. Because of the reference mechanism of JavaScript
2. If you need to customize some members every time you generate an object, you must write the corresponding assignment operation and increase the number of lines of code.
So, before defining a JavaScript object, we can first define a constructor.
function A(x, y) { this.x = x; this.y = y; this.add = function () { return this.x + this.y; } this.mul = function () { return this.x * this.y; } }
Then, define an object
a = new A(1, 2);
The above code looks simple, but it needs to be compared with C++ etc. To distinguish object-oriented languages, A is not the concept of "class" in the strict sense, because JavaScript does not have classes, it just calls the constructor.
Now the question comes, how do we implement inheritance? C++ clearly implements the three object-oriented features of encapsulation, inheritance, and polymorphism. However, for a relatively rough language like JavaScript, there is no strict inheritance mechanism. Instead, the following methods are used to simulate it.
JavaScript prototype
In order to explain the later apply or call function, prototype is introduced here. Prototype is only available to Function.
To use inheritance well, you must first understand why inheritance is designed? It's nothing more than "extracting the common parts" to achieve code reuse.
So in JavaScript, the public part is also placed in the prototype of Function.
Let’s compare two examples of using prototype to implement inheritance
function A(x, y) { this.x = x; this.y = y; this.add = function () { return this.x + this.y; } this.mul = function () { return this.x * this.y; } } function B(x,y){ } B.prototype=new A(1,2); console.log(new B(3,4).add()); //3
In this example, the prototype of the subclass points to a class A object
Let’s implement another example where B inherits A:
function A() { } A.prototype = { x : 1, y : 2, add : function () { return this.x + this.y; }, mul : function () { return this.x * this.y; } } A.prototype.constructor=A; function B(){ } B.prototype=A.prototype; B.prototype.constructor=B;
B’s prototype object refers to A’s prototype object. Because it is a reference, if B’s prototype is modified The prototype object and A's prototype object are also modified, because essentially they all point to a piece of memory. Therefore, every time you change the prototype of type B, you must manually change the constructor back to prevent confusion. Compared with the two examples, this problem does not occur in the previous example because there is no reference.
Create an object of type B
b=new B();
The b object has all members of type A
console.log(b.add()); //3
Because each prototype object has two important members: constructor and _proto_, constructor is essentially a function pointer, so after B.prototype=A.prototype is executed, the constructor is overwritten, so the constructor must be pointed to again later. Constructor of type B.
JavaScript constructor binding
After defining a constructor of type A, define a type B, and then inside the constructor of type B, " Embedded execution" constructor of type A.
function A(x, y) { this.x = x; this.y = y; this.add = function () { return this.x + this.y; } this.mul = function () { return this.x * this.y; } } function B(x, y, z) { A.apply(this, arguments); this.z = z; } console.log(new B(1,2,3));
The apply function is basically the same as the call function, and the A type constructor can be executed inside the B type constructor. At the same time, you can also inherit all members of A.
Display results:
Here is a formula: write A.apply(this) in the B constructor, so that the object constructed by B can Has all members in the A constructor.
When it comes to apply and call, multiple inheritance can also be achieved
function IA(){ this.walk=function(){ console.log("walk"); } } function IB(){ this.run=function(){ console.log("run"); } } function Person(){ IA.apply(this); IB.apply(this); } var p=new Person(); p.walk(); //walk p.run(); //run
The above is the detailed content of Difficulties in JavaScript: Detailed explanation of prototype and constructor binding examples. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










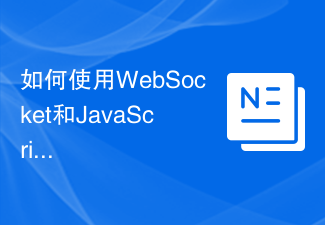
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
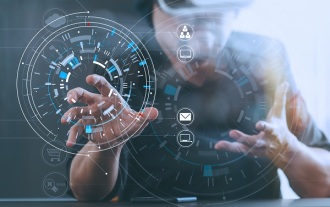
Face detection and recognition technology is already a relatively mature and widely used technology. Currently, the most widely used Internet application language is JS. Implementing face detection and recognition on the Web front-end has advantages and disadvantages compared to back-end face recognition. Advantages include reducing network interaction and real-time recognition, which greatly shortens user waiting time and improves user experience; disadvantages include: being limited by model size, the accuracy is also limited. How to use js to implement face detection on the web? In order to implement face recognition on the Web, you need to be familiar with related programming languages and technologies, such as JavaScript, HTML, CSS, WebRTC, etc. At the same time, you also need to master relevant computer vision and artificial intelligence technologies. It is worth noting that due to the design of the Web side
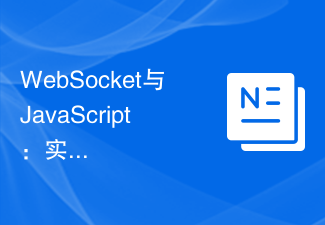
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
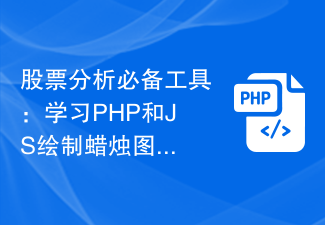
Essential tools for stock analysis: Learn the steps to draw candle charts in PHP and JS. Specific code examples are required. With the rapid development of the Internet and technology, stock trading has become one of the important ways for many investors. Stock analysis is an important part of investor decision-making, and candle charts are widely used in technical analysis. Learning how to draw candle charts using PHP and JS will provide investors with more intuitive information to help them make better decisions. A candlestick chart is a technical chart that displays stock prices in the form of candlesticks. It shows the stock price
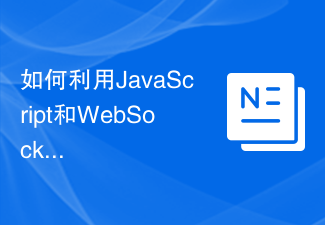
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
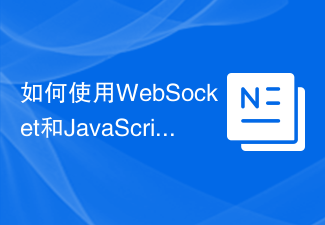
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
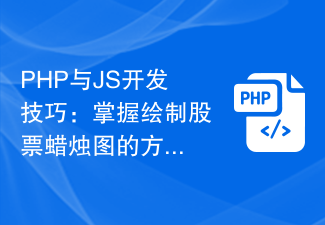
With the rapid development of Internet finance, stock investment has become the choice of more and more people. In stock trading, candle charts are a commonly used technical analysis method. It can show the changing trend of stock prices and help investors make more accurate decisions. This article will introduce the development skills of PHP and JS, lead readers to understand how to draw stock candle charts, and provide specific code examples. 1. Understanding Stock Candle Charts Before introducing how to draw stock candle charts, we first need to understand what a candle chart is. Candlestick charts were developed by the Japanese
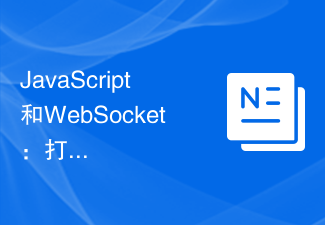
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
