


Detailed explanation of JavaScript function calling and parameter passing usage examples
JavaScript function call
The difference between each method lies in the initialization of this.
Pass parameters by value
The parameters called in the function are the parameters of the function. If a function modifies the value of a parameter, it will not modify the parameter's initial value (defined outside the function). Changes to function parameters will not affect variables outside the function (local variables).
Passing parameters through objects
In JavaScript, you can reference the value of an object. Therefore, when we modify the properties of the object inside the function, its initial value will be modified. Modifying object properties can act outside the function (global variables).
this Keyword
Generally speaking, in Javascript, this points to the current object when the function is executed. Note Note that this is a reserved keyword, you cannot modify the value of this.
Calling JavaScript functions
The code in the function is executed after the function is called.
Called as a function
Instance
##
function myFunction(a, b) { return a * b; } myFunction(10, 2); // myFunction(10, 2) 返回 20
function myFunction(a, b) { return a * b; } window.myFunction(10, 2); // window.myFunction(10, 2) 返回 20
Global objectWhen the function is not called by its own object, the value of this will become the global object. In a web browser the global object is the browser window (window object). The value of this returned by this instance is the window object:
function myFunction() { return this; } myFunction(); // 返回 window 对象
Function calls as methods
In JavaScript you can define functions as methods of objects. The following example creates an object (myObject), which has two properties (firstName and lastName), and one method (fullName):var myObject = { firstName:"John", lastName: "Doe", fullName: function () { return this.firstName + " " + this.lastName; } } myObject.fullName(); // 返回 "John Doe"
This object holds JavaScript code. The value of this in the instance is the myObject object. Test the following! Modify the fullName method and return this value:
var myObject = { firstName:"John", lastName: "Doe", fullName: function () { return this; } } myObject.fullName(); // 返回 [object Object] (所有者对象)
Use the constructor to call the function
If the new keyword is used before the function call, the constructor is called.This looks like a new function is created, but in fact JavaScript functions are re-created objects:
// 构造函数: function myFunction(arg1, arg2) { this.firstName = arg1; this.lastName = arg2; } // This creates a new object var x = new myFunction("John","Doe"); x.firstName; // 返回 "John"
Calling functions as function methods
In JavaScript, functions are objects. A JavaScript function has its properties and methods.call() and apply() are predefined function methods. Two methods can be used to call functions, and the first parameter of both methods must be the object itself.
function myFunction(a, b) { return a * b; } myFunction.call(myObject, 10, 2); // 返回 20
function myFunction(a, b) { return a * b; } myArray = [10,2]; myFunction.apply(myObject, myArray); // 返回 20
In JavaScript strict mode (strict mode), the first parameter will become the value of this when calling the function, even if the parameter is not an object.
In JavaScript non-strict mode (non-strict mode), if the value of the first parameter is null or undefined, it will use the global object instead.
Note Through the call() or apply() method you can set the value of this and call it as a new method of an existing object.
JavaScript function parameters
JavaScript functions do not perform any checks on the values of parameters (arguments).Function explicit parameters and hidden parameters (arguments)In the previous tutorial, we have learned about the explicit parameters of the function:
functionName(parameter1, parameter2, parameter3) { code to be executed }
Function hidden parameters (arguments) are the real values passed to the function when the function is called.
参数规则
JavaScript 函数定义时参数没有指定数据类型。
JavaScript 函数对隐藏参数(arguments)没有进行检测。
JavaScript 函数对隐藏参数(arguments)的个数没有进行检测。
默认参数
如果函数在调用时缺少参数,参数会默认设置为: undefined
有时这是可以接受的,但是建议最好为参数设置一个默认值:
function myFunction(x, y) { if (y === undefined) { y = 0; } }
或者,更简单的方式:
function myFunction(x, y) { y = y || 0; }
Note 如果y已经定义 , y || 返回 y, 因为 y 是 true, 否则返回 0, 因为 undefined 为 false。如果函数调用时设置了过多的参数,参数将无法被引用,因为无法找到对应的参数名。 只能使用 arguments 对象来调用。
Arguments 对象
JavaScript 函数有个内置的对象 arguments 对象.argument 对象包含了函数调用的参数数组。通过这种方式你可以很方便的找到最后一个参数的值:
x = findMax(1, 123, 500, 115, 44, 88); function findMax() { var i, max = 0; for (i = 0; i < arguments.length; i++) { if (arguments[i] > max) { max = arguments[i]; } } return max; }
或者创建一个函数用来统计所有数值的和:
x = sumAll(1, 123, 500, 115, 44, 88); function sumAll() { var i, sum = 0; for (i = 0; i < arguments.length; i++) { sum += arguments[i]; } return sum; }
The above is the detailed content of Detailed explanation of JavaScript function calling and parameter passing usage examples. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










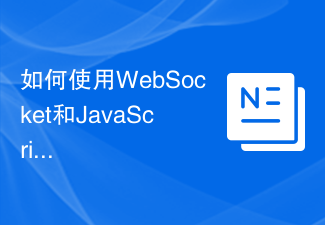
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
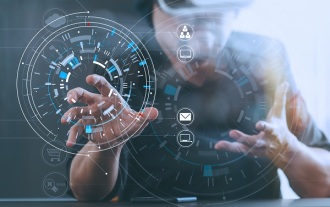
Face detection and recognition technology is already a relatively mature and widely used technology. Currently, the most widely used Internet application language is JS. Implementing face detection and recognition on the Web front-end has advantages and disadvantages compared to back-end face recognition. Advantages include reducing network interaction and real-time recognition, which greatly shortens user waiting time and improves user experience; disadvantages include: being limited by model size, the accuracy is also limited. How to use js to implement face detection on the web? In order to implement face recognition on the Web, you need to be familiar with related programming languages and technologies, such as JavaScript, HTML, CSS, WebRTC, etc. At the same time, you also need to master relevant computer vision and artificial intelligence technologies. It is worth noting that due to the design of the Web side
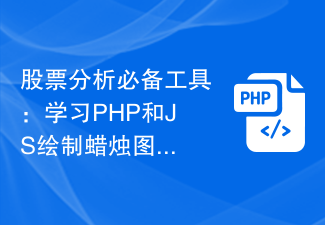
Essential tools for stock analysis: Learn the steps to draw candle charts in PHP and JS. Specific code examples are required. With the rapid development of the Internet and technology, stock trading has become one of the important ways for many investors. Stock analysis is an important part of investor decision-making, and candle charts are widely used in technical analysis. Learning how to draw candle charts using PHP and JS will provide investors with more intuitive information to help them make better decisions. A candlestick chart is a technical chart that displays stock prices in the form of candlesticks. It shows the stock price
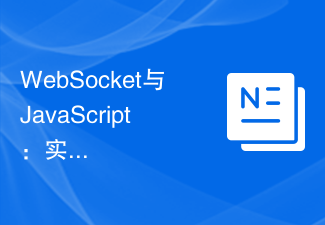
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
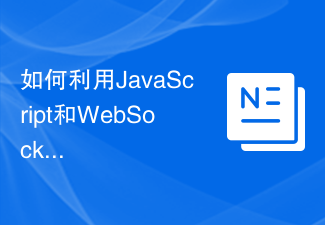
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
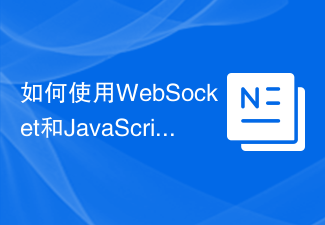
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
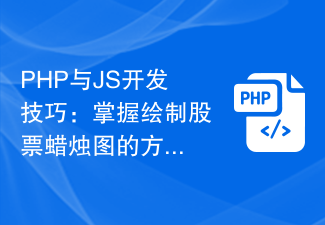
With the rapid development of Internet finance, stock investment has become the choice of more and more people. In stock trading, candle charts are a commonly used technical analysis method. It can show the changing trend of stock prices and help investors make more accurate decisions. This article will introduce the development skills of PHP and JS, lead readers to understand how to draw stock candle charts, and provide specific code examples. 1. Understanding Stock Candle Charts Before introducing how to draw stock candle charts, we first need to understand what a candle chart is. Candlestick charts were developed by the Japanese
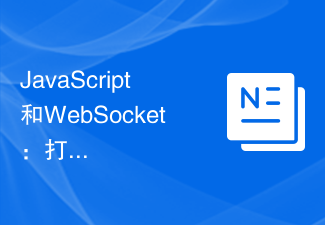
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
