How to implement dependency injection with js
When you make some pages, many of them are implemented using ajax. When displaying, there are many add or update operations for form submission. Obviously this is very annoying. I suddenly thought of a better method. Here is what I will give you Share the idea of how to use js to implement dependency injection, and move the back-end framework idea to the front-end.
Application scenarios: One-to-one correspondence between front and back ends, saving form content, list statements, etc.
Architectural ideas: Distributor, dependency injection, etc.
Basic code statement:
j.extend({ dispatcher: (function () { var _route = {}, // default module _module = { // 授权 authenticate: true, // 验证 validation: true, // 数据转换 dataTransform: true, }, _state = { error: function () { } }, _ajax = function () { j.ajax(this) } ; function _container() { // initializer. return _route; } function _configuration(config, _tmp_route) { if (config) { config.module && (_module = $.extend(_module, config.module)) config.state && (_state = $.extend(_state, config.state)) config.post && config.post.queryString && (function () { if (!/^?/.test(config.post.queryString)) { _tmp_route += "?"; } _tmp_route += config.post.queryString; })() config.list && (function () { config.list = $.extend({ pageSize: 15, wrapped: $("#list-container"), searchForm: $("#form-post"), searchButton: $("#search-button"), post: {} }, config.list); })() } return _tmp_route; } return { ajax: new _container(), intercept: { module: function (module) { $.extend(true, _module, module); }, route: function (route) { if (!$.isEmptyObject(_route)) return; $.extend(true, _route, route); for (var i in _route) { if (_route.hasOwnProperty(i)) { var _controller = _route[i]; for (var k in _controller) { if (_controller.hasOwnProperty(k) && j.utils.isFunction(_controller[k])) { (function () { var clone = j.clone(_controller[k]), _tmp_route = "/" + i + "/" + k; _controller[k] = function (config) { var url = _configuration(config, _tmp_route); if (j.utils.isFunction(clone)) { clone.apply(_module, config); } // todo modules if (!(_module.authenticate && j.utils.isFunction(_module.authenticate)) && _module.authenticate() || _module.authenticate === true) { console.log("j.ajax." + i + "." + k + " authenticate failed."); config.state.error(); return false; } if (config.validation) { _module.validation.init(config.validation); config.validation.fireTarget.on("click", function () { if (!_module.validation || !config.validation.formTarget.valid()) return false; var data = _module.dataTransform(!config.post.data ? config.validation.formTarget.serializeJson() : config.post.data) var ajax_data = { url: url, data: data, fireTarget: config.validation.fireTarget } ajax_data = $.extend(ajax_data, config.post); _ajax.call(ajax_data); return false; }) } if (config.list) { if (!$.fn.pagination) { throw new Error("j.dispatcher.intercept.list need jQuery.pagination,please load jQuery.pagination before this file.") } config.list.onChange = function (pageIndex) { var $this = this; this.showLoading(); var formData = config.list.searchForm.serializeJson(); formData.pageIndex = pageIndex; formData.pageSize = $this.pageSize; var data = _module.dataTransform(!config.list.post.data ? formData : config.list.post.data) var ajax_data = { url: url, data: data, } $.extend(true, ajax_data, config.list.post); ajax_data.success = function () { $this.generateData(this.totalRecords, this.list); } j.jsonp(ajax_data) } j.list.table(config.list); config.list.searchButton.on("click", function () { config.list.wrapped.empty(); j.list.table(config.list); }) } } }()) } } } } } } } })() }) var global = { dataTransform: { "default": function () { if (typeof (arguments[0]) == "object" && Object.prototype.toString.call(arguments[0]).toLowerCase() == "[object object]" && !arguments[0].length) { return j.json.toKeyValString(arguments[0],true); } else if (j.utils.isString(arguments[0])) { return arguments[0]; } else { return {}; } }, "objectData": function () { if (typeof (arguments[0]) == "object" && Object.prototype.toString.call(arguments[0]).toLowerCase() == "[object object]" && !arguments[0].length) { return { data: j.json.toString(arguments[0]) } } else if (j.utils.isString(arguments[0])) { return arguments[0]; } else { return {}; } } } } j.dispatcher.intercept.module({ authenticate: function () { }, validation: (function () { var hasCongfig = false; function _config() { if (!$.fn.validate) { throw new Error("j.dispatcher.intercept.module.validation need jQuery.validate,please load jQuery.validate before this file.") } jQuery.validator.addMethod("isPassword", function (value, element) { return j.config.reg_phone.test(value); }, "请输入6-20密码建议由数字、字母和符号组成!"); jQuery.validator.addMethod("isMobile", function (value, element) { return j.config.reg_phone.test(value); }, "请正确填写您的手机号码"); jQuery.validator.addMethod("isEamil", function (value, element) { return j.config.reg_email.test(value); }, "请填写正确的邮箱地址"); jQuery.validator.addMethod("isUserName", function (value, element) { return j.config.reg_login_name.test(value); }, "4-32位字符.支持汉字、字母、数字"-""_"组合"); } function _getRequired(parms, filters) { if (parms instanceof jQuery && parms.length > 0 && parms[0].tagName == "FORM") { var config = {}; parms.find("[name]").each(function () { if (!filters || filters.indexOf(this.name) == -1) { config[this.name] = { required: true }; } }) return config; } else { for (var i in parms) { if (parms[i]) { parms[i]["required"] = true; } else { parms[i] = { required: true }; } } return parms; } } function _getMessage(parms, filters) { if (parms instanceof jQuery && parms.length > 0 && parms[0].tagName == "FORM") { var config = {}; parms.find("[name]").each(function () { if (!filters || filters.indexOf(this.name) == -1) { config[this.name] = { required: $(this).attr("data-required-message") }; } }) return config; } } function _init(config) { if (!hasCongfig) { hasCongfig = true; _config(); } !config.formTarget && $("#form-post").length > 0 && (config.formTarget = $("#form-post")) !config.fireTarget && $("#post-button").length > 0 && (config.fireTarget = $("#post-button")) if (!(config.fireTarget && config.fireTarget instanceof jQuery && config.fireTarget[0].type.toUpperCase() == "SUBMIT")) throw new Error("j.validator.init needs config.submitTarget param, its type is submit"); if (!(config.formTarget && config.formTarget instanceof jQuery && config.formTarget[0].tagName == "FORM")) throw new Error("j.validator.init needs config.formTarget param, its type is form"); var rules = _getRequired(config.formTarget, config.filters), messages = _getMessage(config.formTarget, config.filters); config.rulesCallBack && config.rulesCallBack(rules); config.messagesCallBack && config.messagesCallBack(messages); config.formTarget.validate({ debug: true, rules: rules, messages: messages }); } return { init: function (config) { _init(config); }, validate: function () { return config.formTarget.valid(); } } })(), dataTransform: global.dataTransform.objectData }) j.dispatcher.intercept.route({ passport: { signin: function () { this.dataTransform = global.dataTransform.default; }, signout: function () { }, reg: function () { }, cpwd: function () { this.dataTransf
The above is the detailed content of How to implement dependency injection with js. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
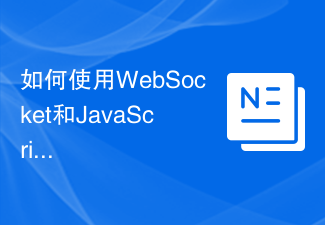
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
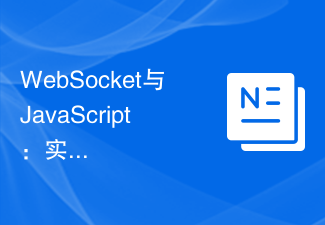
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
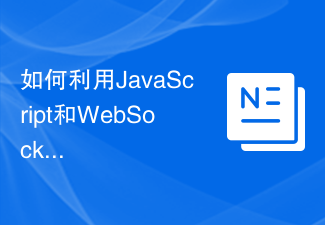
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
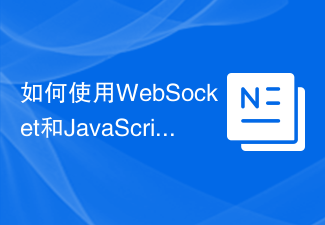
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
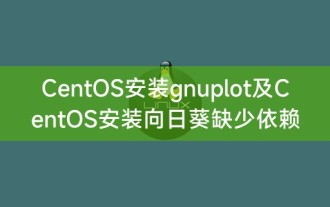
LINUX is a widely used operating system that is highly customizable and stable. CentOS is a free and open source operating system built on the Red Hat Enterprise Linux (RHEL) source code. It is widely used in servers and desktop environments. In CentOS Installing software packages on CentOS is one of the common tasks in daily use. This article will introduce how to install gnuplot on CentOS and solve the problem of missing dependencies of Sunflower software. Gnuplot is a powerful drawing tool that can generate various types of charts, including two-dimensional and three-dimensional data visualization. To install gnuplot on CentOS, you can follow the steps below: 1.
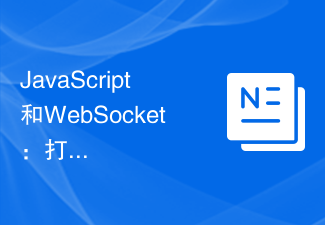
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
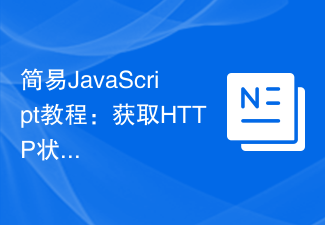
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
