


Tutorial on building React and Webpack desktop applications with Electron
Electron can use HTML, CSS, and JavaScript to build cross-platform desktop applications. However, when using React and Webpack, you will encounter some configuration problems. This article will provide a general solution for Electron configuration under React+Webpack. This article mainly introduces the method of using Electron to build React+Webpack desktop applications. The editor thinks it is quite good, so I will share it with you now and give it as a reference. Let’s follow the editor to take a look, I hope it can help everyone.
Environment configuration
##
"babel-core": "^6.26.0", "babel-loader": "^7.1.2", "babel-plugin-transform-class-properties": "^6.24.1", "babel-plugin-transform-object-rest-spread": "^6.26.0", "babel-preset-env": "^1.6.1", "babel-preset-react": "^6.24.1", "css-loader": "^0.28.7", "electron": "^1.7.9", "electron-packager": "^10.1.0", "extract-text-webpack-plugin": "^3.0.2", "node-sass": "^4.7.2", "react": "^16.2.0", "react-dom": "^16.2.0", "sass-loader": "^6.0.6", "style-loader": "^0.19.1", "webpack": "^3.10.0", "webpack-dev-server": "^2.9.7"
Configuration webpack.config.js
Due to use The default Webpack packaging will generate a large bundle file, which affects performance on the desktop. However, when debugging, the bundle needs to be generated quickly, but sourcemaps need to be used to locate bugs, so we use a function to switch between Environment:module.exports = (env)=>{ ****** const isProduction = env==='production'; ****** devtool: isProduction ? 'source-map':'inline-source-map',
"build:dev": "webpack", "build:prod":"webpack -p --env production",
const webpack = require('webpack'); const path = require('path'); const ExtractTextPlugin = require('extract-text-webpack-plugin'); module.exports = (env)=>{ const isProduction = env==='production'; const CSSExtract = new ExtractTextPlugin('styles.css'); console.log('env='+env); return { entry:'./src/app.js', target: 'electron-renderer', output:{ path:path.join(__dirname, 'public','dist'), filename:'bundle.js' }, module:{ rules:[{ loader: 'babel-loader', test: /\.js(x)?$/, exclude: /node_modules/ }, { test: /\.s?css$/, use:CSSExtract.extract({ use:[ { loader:'css-loader', options:{ sourceMap:true } }, { loader:'sass-loader', options:{ sourceMap:true } } ] }) }] }, plugins:[ CSSExtract ], devtool: isProduction ? 'source-map':'inline-source-map', devServer:{ contentBase: path.join(__dirname, 'public'), historyApiFallback:true, publicPath:'/dist/' } }; }
React
This time I wrote a simple App to display time. The React module is as follows:import React from 'react'; class Time extends React.Component{ state = { time:'' } getTime(){ let date = new Date(); let Year = date.getFullYear(); let Month = date.getMonth(); let Day = date.getDate(); let Hour = date.getHours(); let Minute = date.getMinutes(); let Seconds = date.getSeconds(); let time = Year+'年'+Month+'月'+Day+'日'+Hour+':'+Minute+':'+Seconds; return time; } componentDidMount(){ setInterval(()=>{ this.setState(()=>{ return { time:this.getTime() } }); },1000); } render(){ let timetext = this.state.time; return ( <p> <h1>{timetext}</h1> </p> ); } } export default Time;
Electron
This App does not involve complex Electron API, it is just a container for display:const electron = require('electron'); const {app,BrowserWindow} = electron; let mainWindow = electron; app.on('ready',()=>{ mainWindow = new BrowserWindow({}); mainWindow.loadURL(`file://${__dirname}/public/index.html`); });
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <title>React-Webpack-Electron</title> <link rel="stylesheet" type="text/css" href="./dist/styles.css" rel="external nofollow" > </head> <body> <p id="app"></p> <script src="./dist/bundle.js"></script> </body> </html>
Debugging
##yarn run build:prod
First we use webpack to package the file under dist/ Generate bundle.js and style.css
Start debugging:
We add the following command to the package.json file:
means to build the Mac desktop application and overwrite the files we built before using this command.
Wait for a while and you will see the built folder in the directory, which is our desktop application.
When we open the application, we will find that the navigation bar menu during debugging has disappeared, and there is only one exit option. This is because we have not set up the application Menu bar items, Electron will discard various menus for debugging when building the App.
Everyone should notice that according to the previous method, we have to reuse webpack packaging every time we modify it during debugging. Of course, we can also use webpack- dev-server to monitor changes. It’s just that we need to adjust the project:
Modify the loadURL in the index.js file to:
mainWindow.loadURL(`http://localhost:8080/index.html`);
and then run :
Because at this time we are detecting the files under webpack-dev-server, and we are in the project at this time The modifications you make can be seen in electron in real time.
If debugging and testing are completed, you only need to modify the loadURL to:
mainWindow.loadURL(`file://${__dirname}/public/index.html`);
to proceed with the next build operation.
Note that before building the final application, you should pay attention to whether the web file at this time is running under webpack-dev-server. If so, you should use webpack to generate static packaging files.
Related recommendations:
Detailed introduction to JavaScript development of cross-platform desktop applications (pictures and texts) Diagrams of XML-based desktop applications Detailed introduction to the text codeWrite desktop application with electron_html/css_WEB-ITnoseThe above is the detailed content of Tutorial on building React and Webpack desktop applications with Electron. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










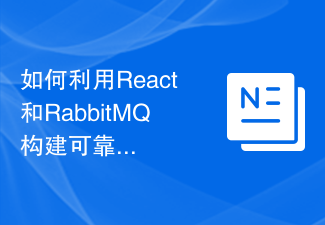
How to build a reliable messaging application with React and RabbitMQ Introduction: Modern applications need to support reliable messaging to achieve features such as real-time updates and data synchronization. React is a popular JavaScript library for building user interfaces, while RabbitMQ is a reliable messaging middleware. This article will introduce how to combine React and RabbitMQ to build a reliable messaging application, and provide specific code examples. RabbitMQ overview:
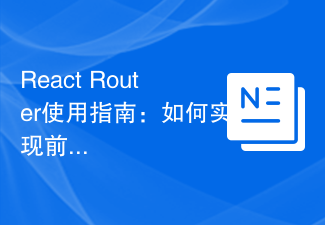
ReactRouter User Guide: How to Implement Front-End Routing Control With the popularity of single-page applications, front-end routing has become an important part that cannot be ignored. As the most popular routing library in the React ecosystem, ReactRouter provides rich functions and easy-to-use APIs, making the implementation of front-end routing very simple and flexible. This article will introduce how to use ReactRouter and provide some specific code examples. To install ReactRouter first, we need
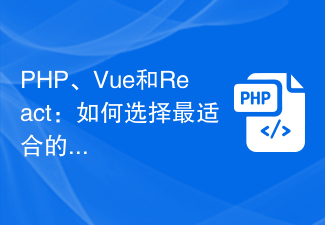
PHP, Vue and React: How to choose the most suitable front-end framework? With the continuous development of Internet technology, front-end frameworks play a vital role in Web development. PHP, Vue and React are three representative front-end frameworks, each with its own unique characteristics and advantages. When choosing which front-end framework to use, developers need to make an informed decision based on project needs, team skills, and personal preferences. This article will compare the characteristics and uses of the three front-end frameworks PHP, Vue and React.
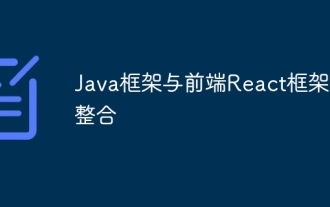
Integration of Java framework and React framework: Steps: Set up the back-end Java framework. Create project structure. Configure build tools. Create React applications. Write REST API endpoints. Configure the communication mechanism. Practical case (SpringBoot+React): Java code: Define RESTfulAPI controller. React code: Get and display the data returned by the API.
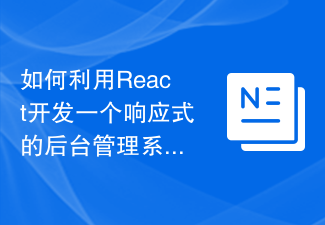
How to use React to develop a responsive backend management system. With the rapid development of the Internet, more and more companies and organizations need an efficient, flexible, and easy-to-manage backend management system to handle daily operations. As one of the most popular JavaScript libraries currently, React provides a concise, efficient and maintainable way to build user interfaces. This article will introduce how to use React to develop a responsive backend management system and give specific code examples. Create a React project first

Vue.js is suitable for small and medium-sized projects and fast iterations, while React is suitable for large and complex applications. 1) Vue.js is easy to use and is suitable for situations where the team is insufficient or the project scale is small. 2) React has a richer ecosystem and is suitable for projects with high performance and complex functional needs.
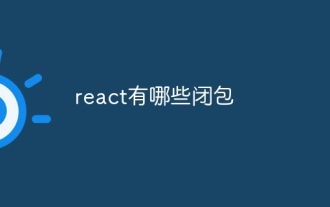
React has closures such as event handling functions, useEffect and useCallback, higher-order components, etc. Detailed introduction: 1. Event handling function closure: In React, when we define an event handling function in a component, the function will form a closure and can access the status and properties within the component scope. In this way, the state and properties of the component can be used in the event processing function to implement interactive logic; 2. Closures in useEffect and useCallback, etc.

React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.
