Require.js usage method sharing
This article mainly brings you an article based on the usage of Require.js (summary). The editor thinks it’s pretty good, so I’ll share it with you now and give it as a reference. Let’s follow the editor to take a look, I hope it can help everyone.
1. Why use require.js
First of all, if a page loads multiple js files, the browser will stop rendering the web page. The more files are loaded, the web page will lose response time. will be longer; secondly, because there are dependencies between js files, the loading order must be strictly guaranteed. When the dependencies are complex, code writing and maintenance will become difficult.
require.js is to solve these two problems:
1. Implement asynchronous loading of js files to avoid web pages losing response;
2. Manage dependencies between modules , which facilitates code writing and maintenance.
2. Loading require.js
The first step is to download the latest version from the official website and load it directly on the page
<script src="js/require.js"></script>
Loading this file may cause the web page to be lost To respond, you can put it at the bottom of the page to load, or you can write the
<script src="js/require.js" defer async="true" ></script>
async attribute to indicate that this file needs to be loaded asynchronously to avoid the webpage losing response. IE does not support this attribute and only supports defer, so defer is also written.
After loading require.js, the next step is to load our own code, which is the entrance, which can be called the main module. If the file is named main.js, it can be written as follows:
<script src="js/require.js" data-main="js/main"></script> .js后缀可以省略
3. How to write the main module
If the main module depends on jQuery, you can write it like this
require(['jquery'], function ($){ alert($); });
4. require.config() method
require.config({ paths: { "jquery": "jquery.min", "underscore": "underscore.min", "backbone": "backbone.min" } });
The above code The file names of the three modules are given, and the path defaults to the same directory as main.js (js subdirectory). If these modules are in other directories, such as the js/lib directory, there are two ways to write them.
• One is to specify the paths one by one
require.config({ paths: { "jquery": "lib/jquery.min", "underscore": "lib/underscore.min", "backbone": "lib/backbone.min" } });
• The other is to directly change the base directory (baseUrl).
require.config({ baseUrl: "js/lib", paths: { "jquery": "jquery.min", "underscor: "underscore.min", "backbone": "backbone.min" } });
• If a module is on another host, you can also specify its URL directly, such as
require.config({ paths: { "jquery": "https://ajax.googleapis.com/ajax/libs/jquery/1.7.2/jquery.min" } });
5. How to write AMD module
Modules loaded by require.js adopt AMD specifications. In other words, the module must be written according to AMD's regulations.
Specifically, the module must be defined using a specific define() function. If a module does not depend on other modules, it can be defined directly in the define() function.
// math.js define(function (){ var add = function (x,y){ return x+y; }; return { add: add }; });
The loading method is as follows:
// main.js require(['math'], function (math){ alert(math.add(1,1)); });
If this module also depends on other modules, then the first parameter of the define() function must be an array to indicate the dependencies of the module sex.
define(['myLib'], function(myLib){ function foo(){ myLib.doSomething(); } return { //返回模块中的函数 foo : foo }; });
When the require() function loads the above module, the myLib.js file will be loaded first.
6. Loading non-standard modules (how to use shim)
// app.js function sayHello(name){ alert('Hi '+name); }
// main.js require.config({ shim: { 'app': { //这个键名为要载入的目标文件的文件名,不能随便命名。 exports: 'sayHello' //exports的值为my.js提供的 对外接口的名称 } } }); require(['app'], function(sayHello) { alert(sayHello()) })
Exporting a function means that we get a javaScript class
But if in my.js There are many functions written in it. It is a bit troublesome to integrate them into one function. Want to export them directly? The method is as follows:
// app.js function sayHi(name){ alert('Hi '+name); } function sayHello(name){ alert('Hiello '+name); }
// main.js require.config({ shim: { app: { init: function() { //这里使用init将2个接口返回 return { sayHi: sayHi, sayHello: sayHello } } } } }); require(['app'], function(a) { a.sayHi('zhangsan'); a.sayHello('lisi'); });
Ordered import of shim
require.config({ shim: { 'jquery.ui.core': ['jquery'], //表示在jquery导入之后导入 'jquery.ui.widget': ['jquery'], 'jquery.ui.mouse': ['jquery'], 'jquery.ui.slider':['jquery'] }, paths : { jquery : 'jquery-2.1.1/jquery', domReady : 'require-2.1.11/domReady', 'jquery.ui.core' : 'jquery-ui-1.10.4/development-bundle/ui/jquery.ui.core', 'jquery.ui.widget' : 'jquery-ui-1.10.4/development-bundle/ui/jquery.ui.widget', 'jquery.ui.mouse' : 'jquery-ui-1.10.4/development-bundle/ui/jquery.ui.mouse', 'jquery.ui.slider' : 'jquery-ui-1.10.4/development-bundle/ui/jquery.ui.slider' } }); require(['jquery', 'domReady','jquery.ui.core','jquery.ui.widget','jquery.ui.mouse','jquery.ui.slider'], function($) { $("#slider" ).slider({ value:0, min: 0, max: 4, step: 1, slide: function( event, ui ) {} }); });
Related recommendations:
javascript advanced modular require.js Sharing examples of specific usage methods
Detailed explanation of specific methods of using advanced modular require.js in JavaScript
Summary of how to use Require.js
The above is the detailed content of Require.js usage method sharing. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










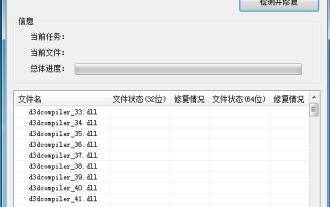
The DirectX repair tool is a professional system tool. Its main function is to detect the DirectX status of the current system. If an abnormality is found, it can be repaired directly. There may be many users who don’t know how to use the DirectX repair tool. Let’s take a look at the detailed tutorial below. 1. Use repair tool software to perform repair detection. 2. If it prompts that there is an abnormal problem in the C++ component after the repair is completed, please click the Cancel button, and then click the Tools menu bar. 3. Click the Options button, select the extension, and click the Start Extension button. 4. After the expansion is completed, re-detect and repair it. 5. If the problem is still not solved after the repair tool operation is completed, you can try to uninstall and reinstall the program that reported the error.
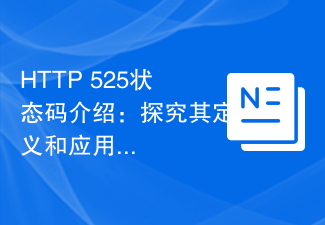
Introduction to HTTP 525 status code: Understand its definition and usage HTTP (HypertextTransferProtocol) 525 status code means that an error occurred on the server during the SSL handshake, resulting in the inability to establish a secure connection. The server returns this status code when an error occurs during the Transport Layer Security (TLS) handshake. This status code falls into the server error category and usually indicates a server configuration or setup problem. When the client tries to connect to the server via HTTPS, the server has no
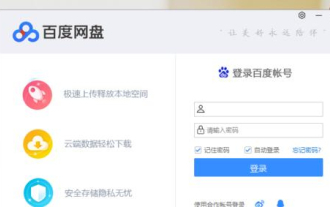
Many friends still don’t know how to use Baidu Netdisk, so the editor will explain how to use Baidu Netdisk below. If you are in need, hurry up and take a look. I believe it will be helpful to everyone. Step 1: Log in directly after installing Baidu Netdisk (as shown in the picture); Step 2: Then select "My Sharing" and "Transfer List" according to the page prompts (as shown in the picture); Step 3: In "Friend Sharing", you can share pictures and files directly with friends (as shown in the picture); Step 4: Then select "Share" and then select computer files or network disk files (as shown in the picture); Fifth Step 1: Then you can find friends (as shown in the picture); Step 6: You can also find the functions you need in the "Function Treasure Box" (as shown in the picture). The above is the editor’s opinion
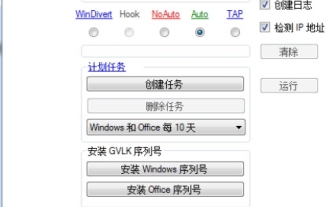
The KMS Activation Tool is a software tool used to activate Microsoft Windows and Office products. KMS is the abbreviation of KeyManagementService, which is key management service. The KMS activation tool simulates the functions of the KMS server so that the computer can connect to the virtual KMS server to activate Windows and Office products. The KMS activation tool is small in size and powerful in function. It can be permanently activated with one click. It can activate any version of the window system and any version of Office software without being connected to the Internet. It is currently the most successful and frequently updated Windows activation tool. Today I will introduce it Let me introduce to you the kms activation work
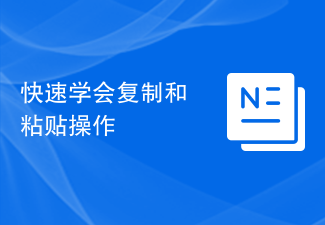
How to use the copy-paste shortcut keys Copy-paste is an operation we often encounter when using computers every day. In order to improve work efficiency, it is very important to master the copy and paste shortcut keys. This article will introduce some commonly used copy and paste shortcut keys to help readers perform copy and paste operations more conveniently. Copy shortcut key: Ctrl+CCtrl+C is the shortcut key for copying. By holding down the Ctrl key and then pressing the C key, you can copy the selected text, files, pictures, etc. to the clipboard. To use this shortcut key,
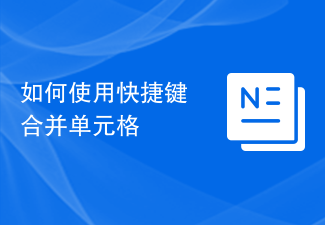
How to use the shortcut keys for merging cells In daily work, we often need to edit and format tables. Merging cells is a common operation that can merge multiple adjacent cells into one cell to improve the beauty of the table and the information display effect. In mainstream spreadsheet software such as Microsoft Excel and Google Sheets, the operation of merging cells is very simple and can be achieved through shortcut keys. The following will introduce the shortcut key usage for merging cells in these two software. exist
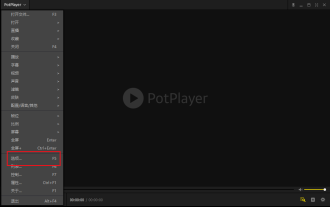
Potplayer is a very powerful media player, but many friends still don’t know how to use potplayer. Today I will introduce how to use potplayer in detail, hoping to help everyone. 1. PotPlayer shortcut keys. The default common shortcut keys for PotPlayer player are as follows: (1) Play/pause: space (2) Volume: mouse wheel, up and down arrow keys (3) forward/backward: left and right arrow keys (4) bookmark: P- Add bookmarks, H-view bookmarks (5) full screen/restore: Enter (6) multiple speeds: C-accelerate, 7) Previous/next frame: D/

PyCharm is a professional Python integrated development environment (IDE) developed by JetBrains. It provides Python developers with powerful functions and tools, making writing Python code more efficient and convenient. PyCharm supports multiple operating systems, including Windows, macOS and Linux, and also supports multiple Python versions, and provides a wealth of plug-ins and extension functions to facilitate developers to customize the IDE environment according to their own needs. P
