


Detailed explanation of the difference between deferred objects and extend in jQuery
This article mainly introduces the deferred object and extend method in jQuery, which has a good reference value. Let's take a look with the editor below, I hope it can help everyone.
1 deferred object
The deferred object is jQuery’s callback function solution, which is a feature introduced from jQuery 1.5.0 version
Methods of the deferred object
(1) $.Deferred() generates a deferred object.
(2) deferred.done() specifies the callback function when the operation is successful
(3) deferred.fail() specifies the callback function when the operation fails
(4 ) When deferred.promise() has no parameters, it returns a new deferred object, and the running status of the object cannot be changed; when it accepts parameters, it serves to deploy the deferred interface on the parameter object.
(5) deferred.resolve() Manually changes the running status of the deferred object to "Completed", thus triggering the done() method immediately.
(6) deferred.reject() This method is exactly the opposite of deferred.resolve(). After being called, the running status of the deferred object will be changed to "failed", thus immediately triggering the fail() method
(7) $.when() specifies callback functions for multiple operations.
In addition to these methods, the deferred object also has two important methods, which are not covered in the above tutorial.
(8) deferred.then()
Sometimes to save trouble, done() and fail() can be written together. This is the then() method.
$.when($.ajax( "/main.php" )) .then(successFunc, failureFunc );
If then() has two parameters, then the first parameter is the callback function of the done() method, and the second parameter is the callback method of the fail() method. If then() has only one parameter, it is equivalent to done().
(9)deferred.always()
This method is also used to specify the callback function. Its function is, regardless of whether deferred.resolve() or deferred.reject() is called. , is always executed in the end.
$.ajax( "test.html" ) .always( function() { alert("已执行!");} );
Let’s do something
1) The chain writing method of ajax operation
First review the traditional writing method of jQuery’s ajax operation:
$.ajax({ url: "test.html", success: function(){ alert("哈哈,成功了!"); }, error:function(){ alert("出错啦!"); } });
Above In the code, $.ajax() accepts an object parameter. This object contains two methods: the success method specifies the callback function after the operation is successful, and the error method specifies the callback function after the operation fails.
After the $.ajax() operation is completed, if you are using a jQuery version lower than 1.5.0, the XHR object will be returned, and you cannot perform chain operations; if it is higher than 1.5.0, What is returned is a deferred object, which can be chained.
After you have the deferred object, you can write it like this
$.ajax("test.html") .done(function(){ alert("哈哈,成功了!"); }) .fail(function(){ alert("出错啦!"); });
The done() in the above code is equivalent to the success method, and the fail() is equivalent to the error method. After adopting the chain writing method, the readability of the code is greatly improved.
2) Specify multiple callback functions for the same operation
One of the great benefits of the deferred object is that it allows you to add multiple callback functions freely.
Still taking the above code as an example, if after the ajax operation is successful, in addition to the original callback function, I want to run another callback function, what should I do?
It's very simple, just add it at the end.
$.ajax("test.html") .done(function(){ alert("哈哈,成功了!");} ) .fail(function(){ alert("出错啦!"); } ) .done(function(){ alert("第二个回调函数!");} );
You can add as many callback functions as you like, and they will be executed in the order they are added.
3) Specify callback functions for multiple operations
Another great benefit of the deferred object is that it allows you to specify a callback function for multiple events, which is not possible with traditional writing. .
Please look at the following code, which uses a new method $.when():
$.when($.ajax("test1.html"), $.ajax("test2.html")) .done(function(){ alert("哈哈,成功了!"); }) .fail(function(){ alert("出错啦!"); });
The meaning of this code is to perform two operations first $.ajax("test1 .html") and $.ajax("test2.html"), if both succeed, the callback function specified by done() will be executed; if one fails or both fail, the callback function specified by fail() will be executed.
4) Callback function interface for common operations (Part 1)
The biggest advantage of the deferred object is that it extends this set of callback function interfaces from ajax operations to all operations. In other words, any operation - whether it is an ajax operation or a local operation, whether it is an asynchronous operation or a synchronous operation - can use various methods of the deferred object to specify a callback function.
Let’s look at a specific example. Suppose there is a time-consuming operation wait:
var wait = function(){ var tasks = function(){ alert("执行完毕!"); }; setTimeout(tasks,5000); };
We specify a callback function for it, what should we do?
Naturally, you will think that you can use $.when():
$.when(wait()) .done(function(){ alert("哈哈,成功了!"); }) .fail(function(){ alert("出错啦!"); });
However, if you write it like this, the done() method will be executed immediately and will not function as a callback function. . The reason is that the parameters of $.when() can only be deferred objects, so wait() must be rewritten:
var dtd = $.Deferred(); // 新建一个deferred对象 var wait = function(dtd){ var tasks = function(){ alert("执行完毕!"); dtd.resolve(); // 改变deferred对象的执行状态 }; setTimeout(tasks,5000); return dtd; };
Now, the wait() function returns a deferred object, which can be chained Operated.
$.when(wait(dtd)) .done(function(){ alert("哈哈,成功了!"); }) .fail(function(){ alert("出错啦!"); });
After the wait() function is run, the callback function specified by the done() method will automatically run.
5) deferred.resolve() method and deferred.reject() method
jQuery stipulates that deferred objects have three execution states - unfinished, completed and failed. If the execution status is "completed" (resolved), the deferred object immediately calls the callback function specified by the done() method; if the execution status is "failed", the callback function specified by the fail() method is called; if the execution status is "unsuccessful" Completed", then
continue to wait, or call the callback function specified by the progress() method (added in jQuery 1.7 version).
前面部分的ajax操作时,deferred对象会根据返回结果,自动改变自身的执行状态;但是,在wait()函数中,这个执行状态必须由程序员手动指定。dtd.resolve()的意思是,将dtd对象的执行状态从"未完成"改为"已完成",从而触发done()方法。
类似的,还存在一个deferred.reject()方法,作用是将dtd对象的执行状态从"未完成"改为"已失败",从而触发fail()方法。
var dtd = $.Deferred(); // 新建一个Deferred对象 var wait = function(dtd){ var tasks = function(){ alert("执行完毕!"); dtd.reject(); // 改变Deferred对象的执行状态 }; setTimeout(tasks,5000); return dtd; }; $.when(wait(dtd)) .done(function(){ alert("哈哈,成功了!"); }) .fail(function(){ alert("出错啦!"); });
6)deferred.promise()方法
上面这种写法,还是有问题。那就是dtd是一个全局对象,所以它的执行状态可以从外部改变。
请看下面的代码:
var dtd = $.Deferred(); // 新建一个Deferred对象 var wait = function(dtd){ var tasks = function(){ alert("执行完毕!"); dtd.resolve(); // 改变Deferred对象的执行状态 }; setTimeout(tasks,5000); return dtd; }; $.when(wait(dtd)) .done(function(){ alert("哈哈,成功了!"); }) .fail(function(){ alert("出错啦!"); }); dtd.resolve();
我在代码的尾部加了一行dtd.resolve(),这就改变了dtd对象的执行状态,因此导致done()方法立刻执行,跳出"哈哈,成功了!"的提示框,等5秒之后再跳出"执行完毕!"的提示框。
为了避免这种情况,jQuery提供了deferred.promise()方法。它的作用是,在原来的deferred对象上返回另一个deferred对象,后者只开放与改变执行状态无关的方法(比如done()方法和fail()方法),屏蔽与改变执行状态有关的方法(比如resolve()方法和
reject()方法),从而使得执行状态不能被改变。
请看下面的代码:
var dtd = $.Deferred(); // 新建一个Deferred对象 var wait = function(dtd){ var tasks = function(){ alert("执行完毕!"); dtd.resolve(); // 改变Deferred对象的执行状态 }; setTimeout(tasks,5000); return dtd.promise(); // 返回promise对象 }; var d = wait(dtd); // 新建一个d对象,改为对这个对象进行操作 $.when(d) .done(function(){ alert("哈哈,成功了!"); }) .fail(function(){ alert("出错啦!"); }); d.resolve(); // 此时,这个语句是无效的
在上面的这段代码中,wait()函数返回的是promise对象。然后,我们把回调函数绑定在这个对象上面,而不是原来的deferred对象上面。这样的好处是,无法改变这个对象的执行状态,要想改变执行状态,只能操作原来的deferred对象。
不过,更好的写法是将dtd对象变成wait()函数的内部对象。
var wait = function(dtd){ var dtd = $.Deferred(); //在函数内部,新建一个Deferred对象 var tasks = function(){ alert("执行完毕!"); dtd.resolve(); // 改变Deferred对象的执行状态 }; setTimeout(tasks,5000); return dtd.promise(); // 返回promise对象 }; $.when(wait()) .done(function(){ alert("哈哈,成功了!"); }) .fail(function(){ alert("出错啦!"); });
7)普通操作的回调函数接口(中)
另一种防止执行状态被外部改变的方法,是使用deferred对象的建构函数$.Deferred()。
这时,wait函数还是保持不变,我们直接把它传入$.Deferred():
$.Deferred(wait) .done(function(){ alert("哈哈,成功了!"); }) .fail(function(){ alert("出错啦!"); });
jQuery规定,$.Deferred()可以接受一个函数名(注意,是函数名)作为参数,$.Deferred()所生成的deferred对象将作为这个函数的默认参数。
8)普通操作的回调函数接口(下)
除了上面两种方法以外,我们还可以直接在wait对象上部署deferred接口。
var dtd = $.Deferred(); // 生成Deferred对象 var wait = function(dtd){ var tasks = function(){ alert("执行完毕!"); dtd.resolve(); // 改变Deferred对象的执行状态 }; setTimeout(tasks,5000); }; dtd.promise(wait); wait.done(function(){ alert("哈哈,成功了!"); }) .fail(function(){ alert("出错啦!"); }); wait(dtd);
这里的关键是dtd.promise(wait)这一行,它的作用就是在wait对象上部署Deferred接口。正是因为有了这一行,后面才能直接在wait上面调用done()和fail()。
2 extend方法
JQuery的extend扩展方法:
Jquery的扩展方法extend是我们在写插件的过程中常用的方法,该方法有一些重载原型,在此,我们一起去了解了解。
1)Jquery的扩展方法原型是:
extend(dest,src1,src2,src3...);
它的含义是将src1,src2,src3...合并到dest中,返回值为合并后的dest,由此可以看出该方法合并后,是修改了dest的结构的。如果想要得到合并的结果却又不想修改dest的结构,可以如下使用:
var newSrc=$.extend({},src1,src2,src3...)//也就是将"{}"作为dest参数。
这样就可以将src1,src2,src3...进行合并,然后将合并结果返回给newSrc了。如下例:
var result=$.extend({},{name:"Tom",age:21},{name:"Jerry",sex:"Boy"})
那么合并后的结果
result={name:"Jerry",age:21,sex:"Boy"}
也就是说后面的参数如果和前面的参数存在相同的名称,那么后面的会覆盖前面的参数值。
2)省略dest参数
上述的extend方法原型中的dest参数是可以省略的,如果省略了,则该方法就只能有一个src参数,而且是将该src合并到调用extend方法的对象中去,如:
$.extend(src)
该方法就是将src合并到jquery的全局对象中去,如:
$.extend({ hello:function(){alert('hello');} });
就是将hello方法合并到jquery的全局对象中。
$.fn.extend(src)
该方法将src合并到jquery的实例对象中去,如:
$.fn.extend({ hello:function(){alert('hello');} });
就是将hello方法合并到jquery的实例对象中。
下面例举几个常用的扩展实例:
$.extend({net:{}});
这是在jquery全局对象中扩展一个net命名空间。
$.extend($.net,{ hello:function(){alert('hello');} })
这是将hello方法扩展到之前扩展的Jquery的net命名空间中去。
3)Jquery的extend方法还有一个重载原型:
extend(boolean,dest,src1,src2,src3...)
第一个参数boolean代表是否进行深度拷贝,其余参数和前面介绍的一致,什么叫深层拷贝,我们看一个例子:
var result=$.extend( true, {}, { name: "John", location: {city: "Boston",county:"USA"} }, { last: "Resig", location: {state: "MA",county:"China"} } );
我们可以看出src1中嵌套子对象location:{city:"Boston"},src2中也嵌套子对象location:{state:"MA"},第一个深度拷贝参数为true,那么合并后的结果就是:
result={name:"John",last:"Resig", location:{city:"Boston",state:"MA",county:"China"}}
也就是说它会将src中的嵌套子对象也进行合并,而如果第一个参数boolean为false,我们看看合并的结果是什么,如下:
var result=$.extend( false, {}, { name: "John", location:{city: "Boston",county:"USA"} }, { last: "Resig", location: {state: "MA",county:"China"} } );
那么合并后的结果就是:
result={name:"John",last:"Resig",location:{state:"MA",county:"China"}}
以上是网上看到的两篇分别对jQuery中的deferred对象和extend方法的介绍,感觉比较详细而且容易理解,所以把它们整理到一起与大家分享!
相关推荐:
$.Deferred(),for循环内异步请求问题的解决方法
The above is the detailed content of Detailed explanation of the difference between deferred objects and extend in jQuery. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










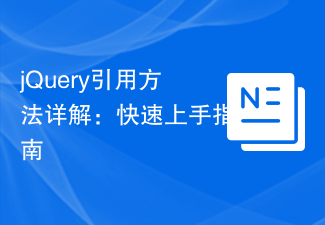
Detailed explanation of jQuery reference method: Quick start guide jQuery is a popular JavaScript library that is widely used in website development. It simplifies JavaScript programming and provides developers with rich functions and features. This article will introduce jQuery's reference method in detail and provide specific code examples to help readers get started quickly. Introducing jQuery First, we need to introduce the jQuery library into the HTML file. It can be introduced through a CDN link or downloaded
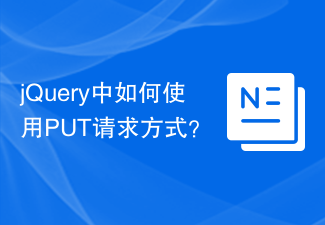
How to use PUT request method in jQuery? In jQuery, the method of sending a PUT request is similar to sending other types of requests, but you need to pay attention to some details and parameter settings. PUT requests are typically used to update resources, such as updating data in a database or updating files on the server. The following is a specific code example using the PUT request method in jQuery. First, make sure you include the jQuery library file, then you can send a PUT request via: $.ajax({u
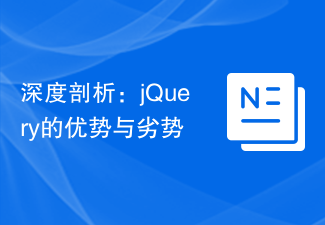
jQuery is a fast, small, feature-rich JavaScript library widely used in front-end development. Since its release in 2006, jQuery has become one of the tools of choice for many developers, but in practical applications, it also has some advantages and disadvantages. This article will deeply analyze the advantages and disadvantages of jQuery and illustrate it with specific code examples. Advantages: 1. Concise syntax jQuery's syntax design is concise and clear, which can greatly improve the readability and writing efficiency of the code. for example,
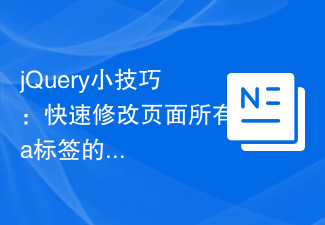
Title: jQuery Tips: Quickly modify the text of all a tags on the page In web development, we often need to modify and operate elements on the page. When using jQuery, sometimes you need to modify the text content of all a tags in the page at once, which can save time and energy. The following will introduce how to use jQuery to quickly modify the text of all a tags on the page, and give specific code examples. First, we need to introduce the jQuery library file and ensure that the following code is introduced into the page: <
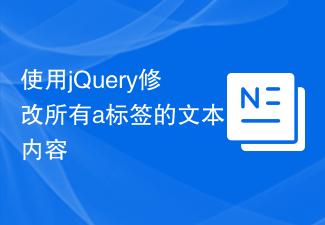
Title: Use jQuery to modify the text content of all a tags. jQuery is a popular JavaScript library that is widely used to handle DOM operations. In web development, we often encounter the need to modify the text content of the link tag (a tag) on the page. This article will explain how to use jQuery to achieve this goal, and provide specific code examples. First, we need to introduce the jQuery library into the page. Add the following code in the HTML file:
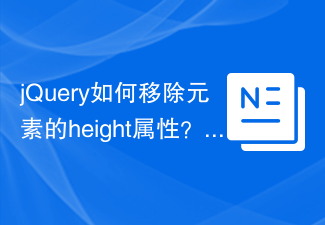
How to remove the height attribute of an element with jQuery? In front-end development, we often encounter the need to manipulate the height attributes of elements. Sometimes, we may need to dynamically change the height of an element, and sometimes we need to remove the height attribute of an element. This article will introduce how to use jQuery to remove the height attribute of an element and provide specific code examples. Before using jQuery to operate the height attribute, we first need to understand the height attribute in CSS. The height attribute is used to set the height of an element
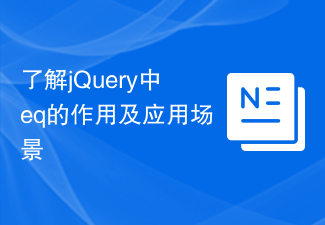
jQuery is a popular JavaScript library that is widely used to handle DOM manipulation and event handling in web pages. In jQuery, the eq() method is used to select elements at a specified index position. The specific usage and application scenarios are as follows. In jQuery, the eq() method selects the element at a specified index position. Index positions start counting from 0, i.e. the index of the first element is 0, the index of the second element is 1, and so on. The syntax of the eq() method is as follows: $("s
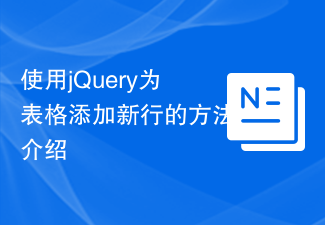
jQuery is a popular JavaScript library widely used in web development. During web development, it is often necessary to dynamically add new rows to tables through JavaScript. This article will introduce how to use jQuery to add new rows to a table, and provide specific code examples. First, we need to introduce the jQuery library into the HTML page. The jQuery library can be introduced in the tag through the following code:
