Detailed explanation of creating scene instances with three.js
This article mainly introduces you to the relevant information of three.js Chinese document learning and creation scenarios. The article introduces it in great detail through sample code. It has certain reference learning value for everyone to learn or use three.js. Friends who need it Let’s learn with the editor below.
What is Three.js?
If you are reading this article, you may have some understanding of Three.js, so let’s briefly introduce what Three.js is.
Three.js is a library that makes WebGL The 3D effects are easy to apply in the browser. While a simple cube in raw WebGL would turn into hundreds of lines of Javascript and shader code, a Three.js requires only a tiny bit of code.
The goal of this section is for three. js for introduction. We started by building the scene using rotating cubes. If you encounter difficulties and need help, there is source code for reference at the bottom of the page.
At least three types of components required for a scene
Camera/Deciding what will be rendered on the screen
Light source /They will have an impact on how materials are displayed and how materials are used when generating shadows
Objects/They are the main rendering formations in the camera perspective: boxes, spheres, etc.
Before you start
Save the following HTML code on your computer, include three.js in the js directory, and then open it in the browser
<html> <head> <meta charset=utf-8> <title>My first three.js app</title> <style> body { margin: 0; } canvas { width: 100%; height: 100% } </style> </head> <body> <script src="js/three.js"></script> <script> // Our Javascript will go here. </script> </body> </html>
The next code will be downloaded in the script tag
Create a sample scene
In order to use three.js for display, we need three elements: scene, camera, renderer , in order to render the scene from the camera.
var scene = new THREE.Scene(); var camera = new THREE.PerspectiveCamera( 75, window.innerWidth / window.innerHeight, 0.1, 1000 ); var renderer = new THREE.WebGLRenderer(); renderer.setSize( window.innerWidth, window.innerHeight ); document.body.appendChild( renderer.domElement );
Let's take a moment to explain what's going on. We have now created the scene, camera and renderer.
There are several types of cameras in three.js. We are temporarily using PerspectiveCamera
Its first attribute is the view angle (FOV), which is the visible view range, and its value represents the angle size.
The second attribute is the aspect ratio. Most of the time you want to use the width divided by the height, otherwise you end up with something like old movies on a widescreen TV - the image looks squashed.
The last two attributes are the near view surface and the distant view surface. Only the area between these two faces will be rendered. You don't need to worry about this for now; using these parameters can improve performance.
Next let’s talk about the renderer. This is the magic. In addition to the WebGLRenderer we use here, three.js also provides some renderers for use on older browsers that do not support WebGL.
In addition to creating a renderer instance, we also need to set the size of the application rendering. It is recommended to use a method that fills the entire width and height of the application - in this case, the width and height of the browser window. For performance-first applications, you can use setSize to set smaller values, such as window.innerHeight/2, window.innerWidth/2, which will render half the size.
If you want to render the entire size at low resolution, you can set the third parameter of setSize - uodateStyle to false if the canvas element is wide If the high value is 100%, the application will be rendered at 1/2 resolution.
Now, we need to add rendered elements to the HTML. The renderer shows us the scene through canvas.
"That's all good, but what about the cube I mentioned earlier" Let's add it now.
var geometry = new THREE.BoxGeometry( 1, 1, 1 ); var material = new THREE.MeshBasicMaterial( { color: 0x00ff00 } ); var cube = new THREE.Mesh( geometry, material ); scene.add( cube ); camera.position.z = 5;
We need BoxGeometry to create the cube. This object contains all the points (vertices) and fills (faces) of the cube. We'll discuss that later.
In addition to the geometry, we also need materials to color it. three.js provides some materials, but we will use MeshBasicMaterial for now. All materials accept and apply an object containing all properties. For simplicity, we only provide one color attribute: green - 0x00ff00 . Uses the same hexadecimal colors as in CSS and PS.
The third element we need is Mesh. A mesh is an object that applies a material to geometry, which we can then place into the scene and move around freely.
When we call scene.add(), what we add will be displayed at coordinates (0, 0, 0,) by default. This causes the camera and cube to overlap internally. To avoid this, we simply move the camera a little further out.
Rendering scene
If you copy the above code in the HTML file, nothing will be displayed on the screen. Because we haven't rendered the scene yet. So we need to call the renderer or animation loop.
function animate() { requestAnimationFrame( animate ); renderer.render( scene, camera ); } animate();
This creates a loop that has the renderer draw one frame every second. If you don't know much about web game programming, you might say "Why not write a setInterval function?" In fact, we can, but requestAnimationFrame has more benefits. The most important benefit is that requestAnimationFrame pauses rendering when the browser switches to another tab, so precious processing power and battery life are not wasted.
Let the cube move
If you insert the code we just created, you should see a green cube. Let it spin so it doesn't get boring.
Add the following code to renderer.render in the animate function:
cube.rotation.x += 0.01; cube.rotation.y += 0.01;
它会按帧运行(每秒60帧),并赋予立方体优雅的动画。基本上,应用运行时,你想移动或改变任何元素,必须通过动画循环。你当然在此处能调用其他函数,以免animate函数上百行代码结尾。
结果
恭喜!你现在创建好了第一个 three.js 应用。很简单,但总得突破。
完整代码参考如下。琢磨一下并深刻理解其工作机理
<html> <head> <title>My first three.js app</title> <style> body { margin: 0; } canvas { width: 100%; height: 100% } </style> </head> <body> <script src="js/three.js"></script> <script> var scene = new THREE.Scene(); var camera = new THREE.PerspectiveCamera( 75, window.innerWidth/window.innerHeight, 0.1, 1000 ); var renderer = new THREE.WebGLRenderer(); renderer.setSize( window.innerWidth, window.innerHeight ); document.body.appendChild( renderer.domElement ); var geometry = new THREE.BoxGeometry( 1, 1, 1 ); var material = new THREE.MeshBasicMaterial( { color: 0x00ff00 } ); var cube = new THREE.Mesh( geometry, material ); scene.add( cube ); camera.position.z = 5; var animate = function () { requestAnimationFrame( animate ); cube.rotation.x += 0.1; cube.rotation.y += 0.1; renderer.render(scene, camera); }; animate(); </script> </body> </html>
相关推荐:
The above is the detailed content of Detailed explanation of creating scene instances with three.js. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


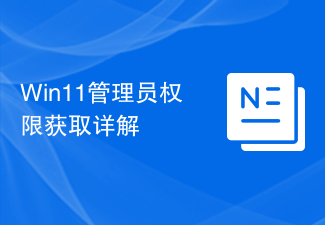
Windows operating system is one of the most popular operating systems in the world, and its new version Win11 has attracted much attention. In the Win11 system, obtaining administrator rights is an important operation. Administrator rights allow users to perform more operations and settings on the system. This article will introduce in detail how to obtain administrator permissions in Win11 system and how to effectively manage permissions. In the Win11 system, administrator rights are divided into two types: local administrator and domain administrator. A local administrator has full administrative rights to the local computer
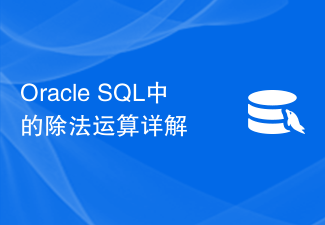
Detailed explanation of division operation in OracleSQL In OracleSQL, division operation is a common and important mathematical operation, used to calculate the result of dividing two numbers. Division is often used in database queries, so understanding the division operation and its usage in OracleSQL is one of the essential skills for database developers. This article will discuss the relevant knowledge of division operations in OracleSQL in detail and provide specific code examples for readers' reference. 1. Division operation in OracleSQL
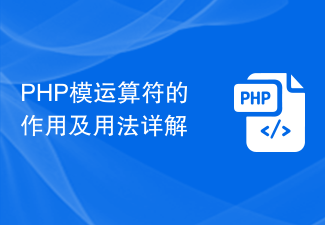
The modulo operator (%) in PHP is used to obtain the remainder of the division of two numbers. In this article, we will discuss the role and usage of the modulo operator in detail, and provide specific code examples to help readers better understand. 1. The role of the modulo operator In mathematics, when we divide an integer by another integer, we get a quotient and a remainder. For example, when we divide 10 by 3, the quotient is 3 and the remainder is 1. The modulo operator is used to obtain this remainder. 2. Usage of the modulo operator In PHP, use the % symbol to represent the modulus
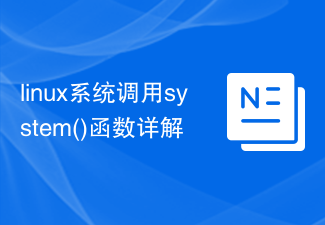
Detailed explanation of Linux system call system() function System call is a very important part of the Linux operating system. It provides a way to interact with the system kernel. Among them, the system() function is one of the commonly used system call functions. This article will introduce the use of the system() function in detail and provide corresponding code examples. Basic Concepts of System Calls System calls are a way for user programs to interact with the operating system kernel. User programs request the operating system by calling system call functions
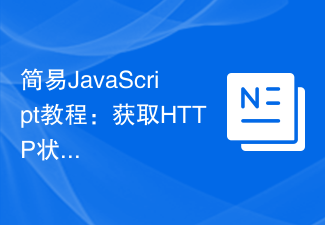
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest
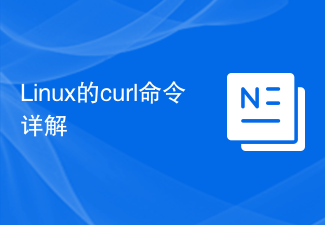
Detailed explanation of Linux's curl command Summary: curl is a powerful command line tool used for data communication with the server. This article will introduce the basic usage of the curl command and provide actual code examples to help readers better understand and apply the command. 1. What is curl? curl is a command line tool used to send and receive various network requests. It supports multiple protocols, such as HTTP, FTP, TELNET, etc., and provides rich functions, such as file upload, file download, data transmission, proxy
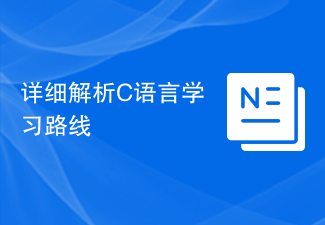
As a programming language widely used in the field of software development, C language is the first choice for many beginner programmers. Learning C language can not only help us establish the basic knowledge of programming, but also improve our problem-solving and thinking abilities. This article will introduce in detail a C language learning roadmap to help beginners better plan their learning process. 1. Learn basic grammar Before starting to learn C language, we first need to understand the basic grammar rules of C language. This includes variables and data types, operators, control statements (such as if statements,
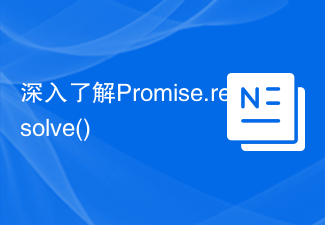
Detailed explanation of Promise.resolve() requires specific code examples. Promise is a mechanism in JavaScript for handling asynchronous operations. In actual development, it is often necessary to handle some asynchronous tasks that need to be executed in sequence, and the Promise.resolve() method is used to return a Promise object that has been fulfilled. Promise.resolve() is a static method of the Promise class, which accepts a
