


Detailed explanation of code spliting to optimize Vue packaging steps
This time I will bring you a detailed explanation of the steps for code spliting to optimize Vue packaging. What are the precautions for code spliting to optimize Vue packaging? The following is a practical case, let’s take a look.
In the era of http1, a common Performance optimization is to merge the number of http requests. Usually we merge many js codes together, but if a js package is particularly large in size It would be a bit overkill for performance improvement. And if we reasonably split all the code, peel off the first-screen and non-first-screen code, split the business code and basic library code, and then load a certain piece of code when it is needed, next time if If you need to use it again, read it from the cache. Firstly, you can make better use of the browser cache. Secondly, it can improve the loading speed of the first screen, which greatly improves the user experience.
Core idea
Separation of business code and basic library
This is actually easy to understand. Business code is usually updated and iterated frequently, while basic library Usually updates are slow. If you split it here, you can make full use of the browser cache to load the basic library code.
Asynchronous loading on demand
This mainly solves the problem of the first screen request size. When accessing the first screen, we only need to load the logic required for the first screen, and Not the code that loads all routes.
Practical
Recently, I used vuetify to transform an internal system. At the beginning, I used the most commonly used webpack configuration, and the functions were quickly developed. However, once I packaged it, I found that The effect is not very obvious. There are many large packages.
Here we look at the packaging distribution. The webpack-bundle-analyzer is used here. You can clearly see vue and vuetify. There are cases where modules are packaged repeatedly.
Here we will post the configuration first and use it for analysis later:
const path = require('path') const webpack = require('webpack') const CleanWebpackPlugin = require('clean-webpack-plugin') const HtmlWebpackPlugin = require('html-webpack-plugin') const BundleAnalyzerPlugin = require('webpack-bundle-analyzer').BundleAnalyzerPlugin; const generateHtml = new HtmlWebpackPlugin({ title: '逍遥系统', template: './src/index.html', minify: { removeComments: true } }) module.exports = { entry: { vendor: ['vue', 'vue-router', 'vuetify'], app: './src/main.js' }, output: { path: path.resolve(dirname, './dist'), filename: '[name].[hash].js', chunkFilename:'[id].[name].[chunkhash].js' }, resolve: { extensions: ['.js', '.vue'], alias: { 'vue$': 'vue/dist/vue.esm.js', 'public': path.resolve(dirname, './public') } }, module: { rules: [ { test: /\.vue$/, loader: 'vue-loader', options: { loaders: { } // other vue-loader options go here } }, { test: /\.js$/, loader: 'babel-loader', exclude: /node_modules/ }, { test: /\.(png|jpg|gif|svg)$/, loader: 'file-loader', options: { objectAssign: 'Object.assign' } }, { test: /\.css$/, loader: ['style-loader', 'css-loader'] }, { test: /\.styl$/, loader: ['style-loader', 'css-loader', 'stylus-loader'] } ] }, devServer: { historyApiFallback: true, noInfo: true }, performance: { hints: false }, devtool: '#eval-source-map', plugins: [ new BundleAnalyzerPlugin(), new CleanWebpackPlugin(['dist']), generateHtml, new webpack.optimize.CommonsChunkPlugin({ name: 'ventor' }), ] } if (process.env.NODE_ENV === 'production') { module.exports.devtool = '#source-map' // http://vue-loader.vuejs.org/en/workflow/production.html module.exports.plugins = (module.exports.plugins || []).concat([ new webpack.DefinePlugin({ 'process.env': { NODE_ENV: '"production"' } }), new webpack.optimize.UglifyJsPlugin({ sourceMap: true, compress: { warnings: false } }), new webpack.LoaderOptionsPlugin({ minimize: true }) ]) }
CommonChunkPlugin
ventor entrance Here we find that all the modules under the referenced node_module, such as axios, are not filtered out, so they are packaged into app.js. Here we do the separation
entry: { vendor: ['vue', 'vue-router', 'vuetify', 'axios'], app: './src/main.js' },
Then there is another problem here, I don’t We may enter the module manually. At this time, we may need to automatically separate the ventor. Here we need to introduce minChunks. In the configuration, we can package and modify the modules referenced under mode_module as follows
entry: { //vendor: ['vue', 'vue-router', 'vuetify', 'axios'], //删除 app: './src/main.js' } new webpack.optimize.CommonsChunkPlugin({ name: 'vendor', minChunks: ({ resource }) => ( resource && resource.indexOf('node_modules') >= 0 && resource.match(/\.js$/) ) }),
After the above After several steps of optimization, if we look at the file distribution, we will find that the modules under node_module have been collected under vendor.
We can get an experience here, that is, in a project, we can specifically package and optimize the modules under node_module. But if you are careful here, you may find that the codemirror component is also in node_module, but why is it not packaged but repeatedly packaged to other single pages? In fact, this is because using the name attribute in commonChunk actually means that only Follow the entry entrance to find the dependent packages. Since our components are loaded asynchronously, they will not be packaged here. Let’s do an experiment to verify. Now we remove the routing lazy loading of the dbmanage and system pages and change them to Directly introduce
// const dbmanage = () => import(/* webpackChunkName: "dbmanage" */'../views/dbmanage.vue') // const system = () => import(/* webpackChunkName: "system" */'../views/system.vue') import dbmanage from '../views/dbmanage.vue' import system from '../views/system.vue'
At this time, when we repackage, we can find that codemirror has been packaged, so the question is, is this good?
async
上面的问题答案是肯定的,不可以的,很明显ventor是我们的入口代码即首屏,我们完全没有必要去加载这个codemirror组件,我们先把刚才的路由修改恢复回去,但是这时又有了新问题,我们的codemirror被同时打包进了两个单页面,并且还有些自己封装的components,例如MTable或是MDataTable等也出现了重复打包。并且codemirror特别大,同时加载到两个单页面也会造成很大的性能问题,简单说就是,我们在访问第一个单页面加载了codemirror之后,在第二个页面其实就不应该再加载了。 要解决这个问题,这里我们可以使用 CommonsChunkPlugin 的 async 并在 minChunnks 里的count方法来判断数量,只要是 重用次数 超过两个包括两个的异步加载模块(即 import () 产生的chunk )我们都认为是 可以 打成公共的 ,这里我们增加一项配置。
new webpack.optimize.CommonsChunkPlugin({ async: 'used-twice', minChunks: (module, count) => ( count >= 2 ), })
再次打包,我们发现所有服用的组件被重新打到了 0.used-twice-app.js中了,这样各个单页面大小也有所下降,平均小了近10k左右
可是,这里我们发现vuetify.js和vuetify.css实在太庞大了,导致我们的打包的代码很大,这里,我们考虑把它提取出来,这里为了避免重复打包,需要使用external,并将vue以及vuetify的代码采用cdn读取的方式,首先修改index.html
//css引入 <link href='https://fonts.googleapis.com/css?family=Roboto:300,400,500,700|Material+Icons' rel="stylesheet" type="text/css"> <link href="https://unpkg.com/vuetify/dist/vuetify.min.css" rel="external nofollow" rel="stylesheet"> //js引入 <script src="https://unpkg.com/vue/dist/vue.js"></script> <script src="https://unpkg.com/vuetify/dist/vuetify.js"></script> //去掉main.js中之前对vuetifycss的引入 //import 'vuetify/dist/vuetify.css'
再修改webpack配置,新增externals
externals: { 'vue':'Vue', "vuetify":"Vuetify" }
再重新打包,可以看到vue相关的代码已经没有了,目前也只有used-twice-app.js比较大了,app.js缩小了近200kb。
但是新问题又来了,codemirror很大,而used-twice又是首屏需要的,这个打包在首屏肯定不是很好,这里我们要将system和dbmanage页面的codemirror组件改为异步加载,单独打包,修改如下:
// import MCode from "../component/MCode.vue"; //注释掉 components: { MDialog, MCode: () => import(/* webpackChunkName: "MCode" */'../component/MCode.vue') },
重新打包下,可以看到 codemirror被抽离了,首屏代码进一步得到了减少,used-twice-app.js代码缩小了近150k。
做了上面这么多的优化之后,业务测的js基本都被拆到了50kb一下(忽略map文件),算是优化成功了。
总结
可能会有朋友会问,单独分拆vue和vuetify会导致请求数增加,这里我想补充下,我们的业务现在已经切换成http2了,由于多路复用,并且加上浏览器缓存,我们分拆出的请求数其实也算是控制在合理的范畴内。
这里最后贴一下优化后的webpack配置,大家一起交流学习下哈。
const path = require('path') const webpack = require('webpack') const CleanWebpackPlugin = require('clean-webpack-plugin') const HtmlWebpackPlugin = require('html-webpack-plugin') const BundleAnalyzerPlugin = require('webpack-bundle-analyzer').BundleAnalyzerPlugin; const generateHtml = new HtmlWebpackPlugin({ title: '逍遥系统', template: './src/index.html', minify: { removeComments: true } }) module.exports = { entry: { app: './src/main.js' }, output: { path: path.resolve(dirname, './dist'), filename: '[name].[hash].js', chunkFilename:'[id].[name].[chunkhash].js' }, resolve: { extensions: ['.js', '.vue'], alias: { 'vue$': 'vue/dist/vue.esm.js', 'public': path.resolve(dirname, './public') } }, externals: { 'vue':'Vue', "vuetify":"Vuetify" }, module: { rules: [ { test: /\.vue$/, loader: 'vue-loader', options: { loaders: { } // other vue-loader options go here } }, { test: /\.js$/, loader: 'babel-loader', exclude: /node_modules/ }, { test: /\.(png|jpg|gif|svg)$/, loader: 'file-loader', options: { objectAssign: 'Object.assign' } }, { test: /\.css$/, loader: ['style-loader', 'css-loader'] }, { test: /\.styl$/, loader: ['style-loader', 'css-loader', 'stylus-loader'] } ] }, devServer: { historyApiFallback: true, noInfo: true }, performance: { hints: false }, devtool: '#eval-source-map', plugins: [ new CleanWebpackPlugin(['dist']), generateHtml ] } if (process.env.NODE_ENV === 'production') { module.exports.devtool = '#source-map' module.exports.plugins = (module.exports.plugins || []).concat([ new BundleAnalyzerPlugin(), new webpack.optimize.CommonsChunkPlugin({ name: 'ventor', minChunks: ({ resource }) => ( resource && resource.indexOf('node_modules') >= 0 && resource.match(/\.js$/) ) }), new webpack.optimize.CommonsChunkPlugin({ async: 'used-twice', minChunks: (module, count) => ( count >= 2 ), }), new webpack.DefinePlugin({ 'process.env': { NODE_ENV: '"production"' } }), new webpack.optimize.UglifyJsPlugin({ sourceMap: true, compress: { warnings: false } }), new webpack.LoaderOptionsPlugin({ minimize: true }) ]) }
相信看了本文案例你已经掌握了方法,更多精彩请关注php中文网其它相关文章!
推荐阅读:
The above is the detailed content of Detailed explanation of code spliting to optimize Vue packaging steps. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










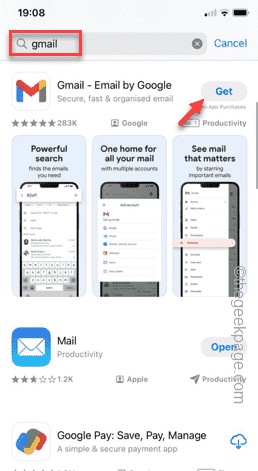
The default map on the iPhone is Maps, Apple's proprietary geolocation provider. Although the map is getting better, it doesn't work well outside the United States. It has nothing to offer compared to Google Maps. In this article, we discuss the feasible steps to use Google Maps to become the default map on your iPhone. How to Make Google Maps the Default Map in iPhone Setting Google Maps as the default map app on your phone is easier than you think. Follow the steps below – Prerequisite steps – You must have Gmail installed on your phone. Step 1 – Open the AppStore. Step 2 – Search for “Gmail”. Step 3 – Click next to Gmail app
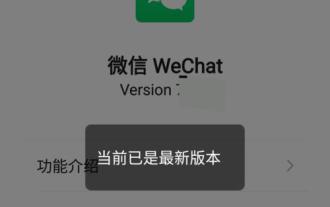
WeChat is one of the social media platforms in China that continuously launches new versions to provide a better user experience. Upgrading WeChat to the latest version is very important to keep in touch with family and colleagues, to stay in touch with friends, and to keep abreast of the latest developments. 1. Understand the features and improvements of the latest version. It is very important to understand the features and improvements of the latest version before upgrading WeChat. For performance improvements and bug fixes, you can learn about the various new features brought by the new version by checking the update notes on the WeChat official website or app store. 2. Check the current WeChat version We need to check the WeChat version currently installed on the mobile phone before upgrading WeChat. Click to open the WeChat application "Me" and then select the menu "About" where you can see the current WeChat version number. 3. Open the app
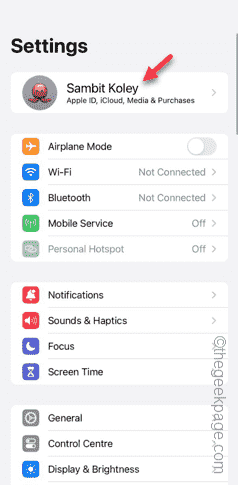
When logging into iTunesStore using AppleID, this error saying "This AppleID has not been used in iTunesStore" may be thrown on the screen. There are no error messages to worry about, you can fix them by following these solution sets. Fix 1 – Change Shipping Address The main reason why this prompt appears in iTunes Store is that you don’t have the correct address in your AppleID profile. Step 1 – First, open iPhone Settings on your iPhone. Step 2 – AppleID should be on top of all other settings. So, open it. Step 3 – Once there, open the “Payment & Shipping” option. Step 4 – Verify your access using Face ID. step
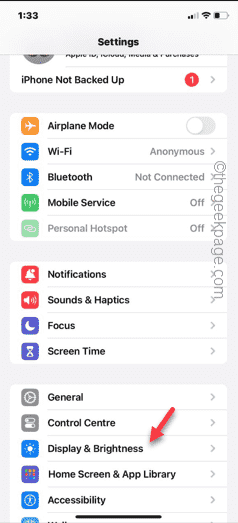
Having issues with the Shazam app on iPhone? Shazam helps you find songs by listening to them. However, if Shazam isn't working properly or doesn't recognize the song, you'll have to troubleshoot it manually. Repairing the Shazam app won't take long. So, without wasting any more time, follow the steps below to resolve issues with Shazam app. Fix 1 – Disable Bold Text Feature Bold text on iPhone may be the reason why Shazam is not working properly. Step 1 – You can only do this from your iPhone settings. So, open it. Step 2 – Next, open the “Display & Brightness” settings there. Step 3 – If you find that “Bold Text” is enabled
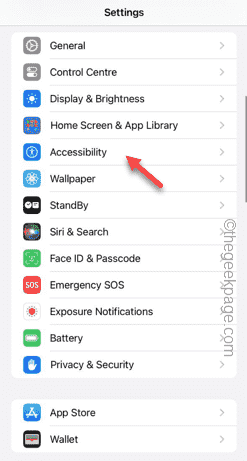
Screenshot feature not working on your iPhone? Taking a screenshot is very easy as you just need to hold down the Volume Up button and the Power button at the same time to grab your phone screen. However, there are other ways to capture frames on the device. Fix 1 – Using Assistive Touch Take a screenshot using the Assistive Touch feature. Step 1 – Go to your phone settings. Step 2 – Next, tap to open Accessibility settings. Step 3 – Open Touch settings. Step 4 – Next, open the Assistive Touch settings. Step 5 – Turn on Assistive Touch on your phone. Step 6 – Open “Customize Top Menu” to access it. Step 7 – Now you just need to link any of these functions to your screen capture. So click on the first
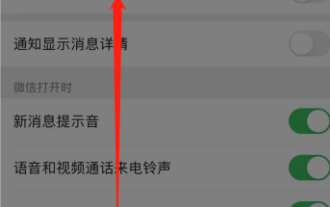
To understand how to close a WeChat video account, you first need to know that WeChat, a chat software, is very rich in functions. For different people, WeChat video accounts have different functions. For ordinary people, the WeChat video account is just a channel for sharing short videos shot by individuals, and they can share some interesting WeChat videos. For others, they use WeChat video accounts to attract traffic. Naturally, they may not be able to accept comments from trolls and trolls, so they may want to close the WeChat video account. Next, the editor of this website will introduce to you how to close the WeChat video account. Friends who are interested, come and take a look. The steps to close the WeChat video account are as follows: We first open WeChat, enter the main page, and click " I" button, which will take us to a
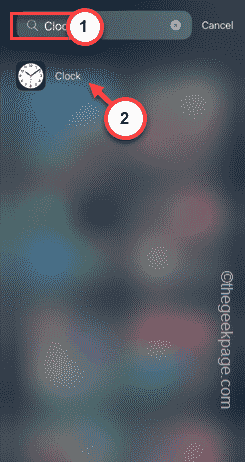
Is the clock app missing from your phone? The date and time will still appear on your iPhone's status bar. However, without the Clock app, you won’t be able to use world clock, stopwatch, alarm clock, and many other features. Therefore, fixing missing clock app should be at the top of your to-do list. These solutions can help you resolve this issue. Fix 1 – Place the Clock App If you mistakenly removed the Clock app from your home screen, you can put the Clock app back in its place. Step 1 – Unlock your iPhone and start swiping to the left until you reach the App Library page. Step 2 – Next, search for “clock” in the search box. Step 3 – When you see “Clock” below in the search results, press and hold it and
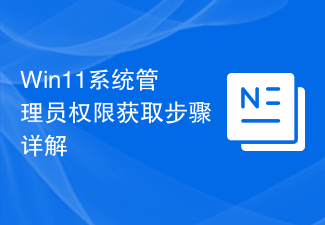
Windows 11, as the latest operating system launched by Microsoft, is deeply loved by users. In the process of using Windows 11, sometimes we need to obtain system administrator rights in order to perform some operations that require permissions. Next, we will introduce in detail the steps to obtain system administrator rights in Windows 11. The first step is to click "Start Menu". You can see the Windows icon in the lower left corner. Click the icon to open the "Start Menu". In the second step, find and click "
