How to use implicit calls in javascript?
This article gives you a detailed introduction to the knowledge points related to implicit calls in JavaScript. Those who are interested in this can learn together.
Preface
I don’t know if it is accurate to use implicit calling to describe it. Its behavior is always hidden behind the scenes, and it shows its face from time to time. It doesn’t seem to have much effect, but it is still useful to understand it. Guaranteed to be useful under your use.
The so-called implicit call simply means automatically calling some methods, and these methods can be modified externally like hooks, thereby changing the established behavior.
Below I will list some implicit calls that I have seen recently. The examples are just to the point. Welcome to add
Data type conversion toSting and valueOf
var obj = { a: 1, toString: function () { console.log('toString') return '2' }, valueOf: function () { console.log('valueOf') return 3 } } console.log(obj == '2'); //依次输出 'valueOf' false console.log(String(obj));//依次输出 'toString' '2'
var obj = { a: 1, toString: function () { console.log('toString') return '2' }, valueOf: function () { console.log('valueOf') return {} //修改为对象 } } console.log(obj == '2'); //依次输出 'valueOf' 'toString' true console.log(Number(obj));//依次输出 'valueOf' 'toString' 2
In the operation of the equality operator, the object will first call valueOf. If the returned value is an object, it will call toSting, except null, and then use the returned value for comparison. The first example is equivalent to 3 == '2' Outputs false. The second example returns an object because valueOf is executed, and then toString is executed. Finally, it is equivalent to '2' == '2' and outputs true. In the Number and String methods, Number will call valueOf first, and then toString. String The method is the opposite.
In addition to the two examples above, data type conversion also exists in various other operations, such as numerical operations. When reference types are involved, the valueOf or toString method will be called, and as long as it is an object, it will be inherited. We can re-override these two methods to affect the behavior of data type conversion
handleEvent in DOM2 event
var eventObj = { a: 1, handleEvent: function (e) { console.log(this, e);//返回 eventObj 和 事件对象 alert(this.a) } } document.addEventListener('click', eventObj)
You read that right, The second parameter of addEventListener can be an object in addition to a function. After the event is triggered, the handleEvent method of the object will be executed. When the method is executed, this points to eventObj. You can bind the data you want to pass in to the eventObj object
JSON object toJSON
var Obj = { a: 10, toJSON: function () { return { a: 1, b: function () { }, c: NaN, d: -Infinity, e: Infinity, f: /\d/, g: new Error(), h: new Date(), i: undefined, } } } console.log(JSON.stringify(Obj)); //{"a":1,"c":null,"d":null,"e":null,"f":{},"g":{},"h":"2018-02-09T19:29:13.828Z"}
If the object passed in by the stringify method of JSON has a toJSON method, then the object executed by the method will be converted to the object returned after toJSON is executed. There is one thing to note. Note that, if the following code
var Obj1 = { a: 10, toJSON: function () { console.log(this === Obj1);//true return this } } console.log(JSON.stringify(Obj1));//{"a":10}
var Obj2 = { a: 10, toJSON: function () { console.log(this === Obj2);//true return { a: this } } } console.log(JSON.stringify(Obj2));//报错 Maximum call stack size exceeded
is based on the above statement, it is obvious that the error is what we expected, but when directly returning this, no error is reported at all. You might as well make a bold guess about the internal objects returned by toJSON and Compare the original objects, and if they are equal, use the then of the original object
promise object
var obj = { then: function (resolve, reject) { setTimeout(function () { resolve(1000); }, 1000) } } Promise.resolve(obj).then(function (data) { console.log(data);// 延迟1秒左右输出 1000 })
When the Promise.resolve method passes in the object, if there is a then method The then method will be executed immediately, which is equivalent to putting the method into a new Promise. In addition to Promise.resolve having this behavior, Promise.all also has this behavior
var timePromise = function (time) { return new Promise(function (resolve) { setTimeout(function () { resolve(time); }, time) }) } var timePromise1 = timePromise(1000); var timePromise2 = timePromise(2000); var timePromise3 = timePromise(3000); Array.prototype.then = function (resolve) { setTimeout(function () { resolve(4000); }, 4000) } Promise.all([timePromise1, timePromise2, timePromise3]).then(function (time) { console.log(time);// 等待4秒左右输出 4000 })
Object attribute accessors get and set
var obj = { _age: 100, get age() { return this._age < 18 ? this._age : 18; }, set age(value) { this._age = value; console.log(`年龄设置为${value}岁`); } } obj.age = 1000; //年龄设置为1000岁 obj.age = 200; //年龄设置为200岁 console.log(obj.age);// 18 obj.age = 2; ////年龄设置为2岁 console.log(obj.age); // 2
You can see that no matter how much the age is set, my age is 18 years old or below. When performing attribute access, the corresponding get set function of the object attribute is actually called. In addition to the above The writing method is also as follows
var input = document.createElement('input'); var span = document.createElement('span'); document.body.appendChild(input); document.body.appendChild(span); var obj = { _age:'' } var obj = Object.defineProperty(obj, 'age', { get: function () { return this._age; }, set: function (value) { this._age = value; input.value = value; span.innerHTML = value; } }); input.onkeyup = function (e) { if (e.keyCode === 37 || e.keyCode === 39) { return; } obj.age = this.value }
Now the value of input and the innerHTML value of obj.age attribute value span are bound together
Iterator interface Symbol.iterator
var arr = [ 1, 2 , 3]; arr[Symbol.iterator] = function () { const self = this; let index = 0; return { next () { if(index < self.length) { return { value: self[index] ** self[index++], done: false } } else { return { value: undefined, done: true } } } } } console.log([...arr, 4]);//返回 [1, 4, 27, 4] for(let value of arr) { console.log(value); //依次返回 1 4 27 }
You can see that whenever you call the spread operator, or use for...of to loop through an object, you will call the object's traverser interface, such as Array, String, Map, Set, TypedArray and others. Array-like objects such as arguments and NodeList natively have a traverser interface, but ordinary objects do not deploy this interface. If you want the object to be able to use the spread operator or for...of loop, you can add this method to the object, or you can add it to the original object. Overriding methods on objects with interfaces to change their behavior
The above is what I compiled for everyone. I hope it will be helpful to everyone in the future.
Related articles:
What security vulnerabilities exist when using timing-attack in node applications
Implementing one-way in the vue component delivery object Binding, how to do it?
How to use TypeScript methods in Vue components (detailed tutorial)
The above is the detailed content of How to use implicit calls in javascript?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
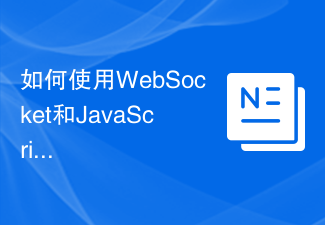
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
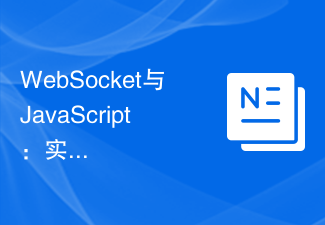
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
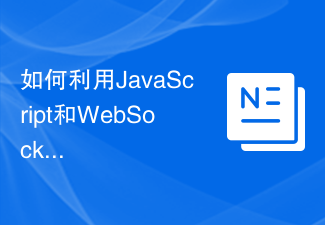
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
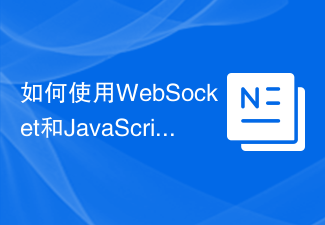
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
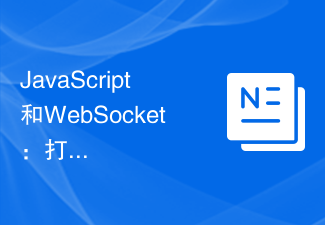
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
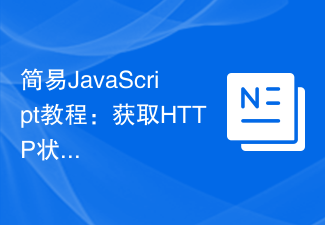
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest

Usage: In JavaScript, the insertBefore() method is used to insert a new node in the DOM tree. This method requires two parameters: the new node to be inserted and the reference node (that is, the node where the new node will be inserted).
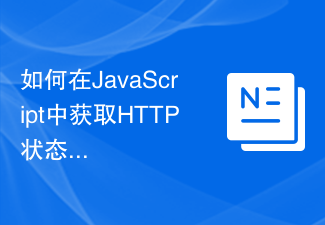
Introduction to the method of obtaining HTTP status code in JavaScript: In front-end development, we often need to deal with the interaction with the back-end interface, and HTTP status code is a very important part of it. Understanding and obtaining HTTP status codes helps us better handle the data returned by the interface. This article will introduce how to use JavaScript to obtain HTTP status codes and provide specific code examples. 1. What is HTTP status code? HTTP status code means that when the browser initiates a request to the server, the service
