Summary of commonly used instructions in Vue
The content shared with you in this article is a summary of commonly used instructions in Vue. The content is very detailed. Next, let’s take a look at the specific content. I hope it can help friends in need.
1 Commonly used commands
v-if command
v-show command
-
v-else instruction
v-for instruction
v-bind instruction
v-model
v-on command
v-text command
1.1 v-if
is a conditional rendering instruction, which deletes and inserts elements based on the true or false expression. Its basic syntax is as follows:
v-if="expression"
expression is an expression that returns a bool value. Expression The formula can be a bool attribute or an operation formula that returns bool. For example:
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title></title> <script type="text/javascript" src="https://cdn.bootcss.com/vue/2.2.2/vue.min.js"></script> </head> <body> <div id="app"> <h1>Hello, Vue.js!</h1> <h1 v-if="yes">Yes!</h1> <h1 v-if="no">No!</h1> <h1 v-if="age >= 25">Age: {{ age }}</h1> <h1 v-if="name.indexOf('jack') >= 0">Name: {{ name }}</h1> </div> </body> <script src="js/vue.js"></script> <script> var vm = new Vue({ el: '#app', data: { yes: true, no: false, age: 28, name: 'keepfool' } }) </script> </html>
The display result is as follows,
Note: v-if instruction is executed based on the value of the conditional expressionInsertion or deletion behavior of elements.
1.2 v-for directive
v-for
directive renders a list based on an array, which is similar to JavaScript’s traversal syntax :
v-for="item in items"
items is an array, item is the array element currently being traversed.
Sample code:
name age {{item.name}} {{item.age}}
1.3 The v-bind directive can take a parameter after its name, separated by a colon. This parameter is usually an attribute of the HTML element, for example :v-bind:class
v-bind:argument="expression"
1.4 v-model
v-model (value, checked, and selected will be ignored after the form element is set), commonly used in Forms and
allow form elements and data to achieve two-way binding (mapping relationship)
Sample code
<p id="app"> <p v-text="message"> </p> <input type="text" v-model="message"> </p> </body> <script type="text/javascript"> var app = new Vue({ el:"#app", data:{ message:"nice to meet you" } })</script>
1.5 The v-on directive is used to monitor DOM events. Its syntax is similar to v-bind. For example, to monitor the click event of the element:
<a v-on:click="doSomething">
There are two forms of calling methods:Bind a method (let the event point to a reference to the method), or use an inline statement.
The Greet button binds its click event directly to the greet() method, while the Hi button calls the say() method.
It is a very common requirement to call event.preventDefault()
or event.stopPropagation()
in an event handler. Vue.js provides event modifiers for v-on
. As mentioned before, modifiers are represented by instruction suffixes starting with a dot.
.stop
.prevent
.capture
.self
.once
.passive
<!-- 阻止单击事件继续传播 --> <a v-on:click.stop="doThis"></a> <!-- 提交事件不再重载页面 --> <form v-on:submit.prevent="onSubmit"></form> <!-- 修饰符可以串联 --> <a v-on:click.stop.prevent="doThat"></a> <!-- 只有修饰符 --> <form v-on:submit.prevent></form> <!-- 添加事件监听器时使用事件捕获模式 --> <!-- 即元素自身触发的事件先在此处处理,然后才交由内部元素进行处理 --> <p v-on:click.capture="doThis">...</p> <!-- 只当在 event.target 是当前元素自身时触发处理函数 --> <!-- 即事件不是从内部元素触发的 --> <p v-on:click.self="doThat">...</p>
Vue.js is the two most commonly used instructions v-bind and v-on provides an abbreviation. The v-bind instruction can be abbreviated to a colon, and the v-on instruction can be abbreviated to the @ symbol.
<!--完整语法--> <a href="javascripit:void(0)" v-bind:class="activeNumber === n + 1 ? 'active' : ''">{{ n + 1 }}</a> <!--缩写语法--> <a href="javascripit:void(0)" :class="activeNumber=== n + 1 ? 'active' : ''">{{ n + 1 }}</a> <!--完整语法--> <button v-on:click="greet">Greet</button> <!--缩写语法--> <button @click="greet">Greet</button>
1.6 The v-text directive is mainly to prevent {{}} from appearing on the page when the page is first loaded
v-text="expresstion"
Related recommendations:
ECMAScript Usage examples of typeof in
How does vue.js implement tree table encapsulation? How to implement tree table in vue.js
The above is the detailed content of Summary of commonly used instructions in Vue. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
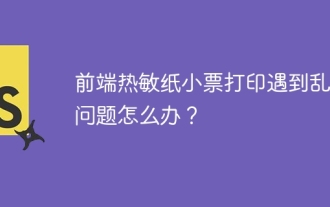
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
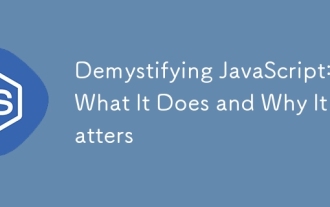
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
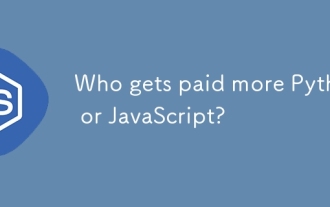
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
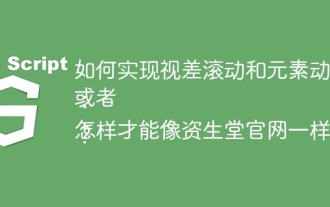
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
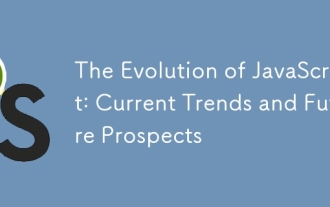
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
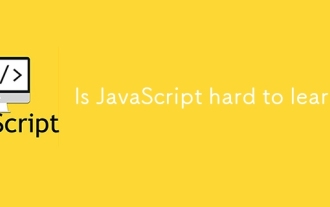
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
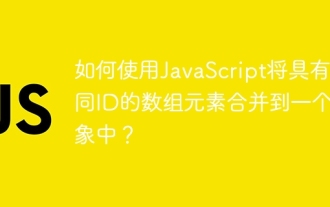
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
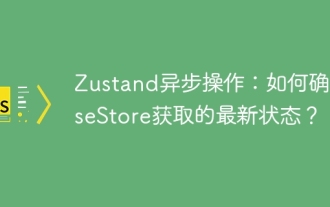
Data update problems in zustand asynchronous operations. When using the zustand state management library, you often encounter the problem of data updates that cause asynchronous operations to be untimely. �...
