Analysis of v-model directive in Vue (with code)
The content of this article is about the analysis of the v-model instruction in Vue (with code). It has a good reference value and I hope it can help friends in need.
1. Instruction explanation
v-model creates a two-way binding on a form control or component, which is essentially responsible for monitoring user input events (onchange, onkeyup, onkeydown, etc., which one specifically , please also check the official underlying implementation documentation) to update the data and perform some special processing for some extreme scenarios.
2. Form input binding methods and methods
2.1 Input box implements two-way data binding example:
<template> <div> <p class="p1">{{title}}</p> <div class="spancss1"> <label>请输入:</label><input type="text" v-model="inputs"/><br/> <label>你在input框中输入了:</label><span>{{inputs}}</span> </div> </div> </template> <script> export default { name:"v-model", data(){ return { title: 'v-model学习', inputs: '' } } } </script> <style scoped> .p1{ text-align: left; } .spancss1{ float: left; } </style>
2.2 Textarea multiple Example of double-thinking data binding using line text:
<template> <div> <p class="p1">{{title}}</p> <div class="spancss1"> <label>请输入:</label><textarea v-model="text"></textarea><br/> <label>你在textarea框中输入了:</label><span>{{text}}</span> </div> </div> </template> <script> export default { name:"v-model", data(){ return { title: 'v-model学习', text: '' } } } </script> <style scoped> .p1{ text-align: left; } .spancss1{ float: left; } </style>
2.3 Check box data binding
Single check box data binding Example:
<template> <div> <p class="p1">{{title}}</p> <div class="spancss1"> <label>请输入:</label><input type="checkbox" id="checkbox" v-model="checked">选我<br/> <label v-if="checked">选中了:</label> <label v-if="!checked">没选中:</label> <span>{{ checked }}</span> </div> </div> </template> <script> export default { name:"v-model", data(){ return { title: 'v-model学习', checked: '' } } } </script> <style scoped> .p1{ text-align: left; } .spancss1{ float: left; } </style>
Multiple check boxes to implement data binding Example:
<template> <div> <p class="p1">{{title}}</p> <div class="spancss1"> <label>请输入:</label> <input type="checkbox" id="vue1" value="vue1" v-model="checkedValues">vue1 <input type="checkbox" id="vue2" value="vue2" v-model="checkedValues">vue2 <input type="checkbox" id="vue3" value="vue3" v-model="checkedValues">vue3 <br/> <label>选中了:</label><span>{{ checkedValues }}</span> </div> </div> </template> <script> export default { name:"v-model", data(){ return { title: 'v-model学习', checkedValues: [] } } } </script> <style scoped> .p1{ text-align: left; } .spancss1{ float: left; } </style>
2.4 Radio buttons implement data binding, and the selected radio button value is obtained and displayed:
<template> <div> <p class="p1">{{title}}</p> <div class="spancss1"> <input type="radio" id="radio1" value="1" v-model="checked">男<br/> <input type="radio" id="radio2" value="2" v-model="checked">女<br/> <label> 选中了:{{ checked }}</label> </div> </div> </template> <script> export default { name:"v-model", data(){ return { title: 'v-model学习', checked: '' } } } </script> <style scoped> .p1{ text-align: left; } .spancss1{ float: left; } </style>
2.5 Introduction to the selection box
Method 1: The selection box implements two-way data binding. First, the selection box implements a single selection. When the options in the radio button box set the disabled attribute, the options cannot be selected. When When the options in the radio button box have no value, the text of the options will be bound when the options are selected. When the options in the radio button box have a value, the value of the options will be bound when the options are selected. The example is as follows:
<template> <div> <p class="p1">{{title}}</p> <div class="spancss1"> <select v-model="selected"> <option disabled value="">请选择</option> <option>A</option> <option value="2">B</option> <option value="3">C</option> </select> <span>Selected: {{ selected }}</span> </div> </div> </template> <script> export default { name:"v-model", data(){ return { title: 'v-model学习', selected: '' } } } </script> <style scoped> .p1{ text-align: left; } .spancss1{ float: left; } </style>
Method 2 introduces the situation of multiple selections in the selection box. First, you need to add the multiple attribute to the select. When the options are in the radio button When setting the disabled attribute, this option cannot be selected. When the options in the radio button box have no value, the text of this option will be bound when this option is selected. When the options in the radio button box have a value, when this option is selected, The value of this options will be bound, then press the ctrl key and click on the options to be selected. A simple example is as follows:
<template> <div> <p class="p1">{{title}}</p> <div class="spancss1"> <select v-model="selected" multiple> <option disabled value="">请选择</option> <option>A</option> <option value="2">B</option> <option value="3">C</option> </select> <span>Selected: {{ selected }}</span> </div> </div> </template> <script> export default { name:"v-model", data(){ return { title: 'v-model学习', selected: [] } } } </script> <style scoped> .p1{ text-align: left; } .spancss1{ float: left; } </style>
3. v-model modifier
3.1 .lazy
By default, v-model synchronizes the value of the input box with the data after each input event is triggered. (Except when input method combines text). You can add the lazy modifier to switch to using onchange event is synchronized. When data is entered in the input box, the data will not change immediately. When the cursor leaves the input box, the data will be changed synchronously. The sample code is as follows:
<template> <div> <p class="p1">{{title}}</p> <div class="spancss1"> <!-- 在“change”时而非“input”时更新 --> <input type="text" id="names" v-model.lazy="msg" ><br/> <label>输入值:</label>{{msg}} </div> </div> </template> <script> export default { name:"v-model", data(){ return { title: 'v-model学习', msg: '' } } } </script> <style scoped> .p1{ text-align: left; } .spancss1{ float: left; } </style>
3.2 .number
If you want to automatically convert the user’s input value to a numerical type, define type as number type and add the number modifier to v-model , when the user enters numerical type data, v-model.number will automatically convert the input data into a numerical type. Note that if the user enters the special letter e, the number attribute cannot be recognized. A simple code example is as follows:
<template> <div> <p class="p1">{{title}}</p> <div class="spancss1"> <!-- 在“change”时而非“input”时更新 --> <input type="number" id="names" v-model.number="msg" ><br/> <label>输入值:</label>{{msg}} </div> </div> </template> <script> export default { name:"v-model", data(){ return { title: 'v-model学习', msg: '' } } } </script> <style scoped> .p1{ text-align: left; } .spancss1{ float: left; } </style>
3.3 .trim
If you want to automatically filter the leading and trailing blank characters entered by the user, you can add the trim modifier to v-model, in Add a few more spaces at the beginning of the input box. When the cursor leaves, the trim attribute will automatically filter the ending spaces. A simple code example is as follows:
<template> <div> <p class="p1">{{title}}</p> <div class="spancss1"> <label>无trim属性</label> <input type="text" id="names" v-model="msg1" ><br/> <label>输入值:</label>{{msg1}}<br/> <label>有trim属性</label> <input type="text" id="names2" v-model.trim="msg2" ><br/> <label>输入值:</label>{{msg2}} </div> </div> </template> <script> export default { name:"v-model", data(){ return { title: 'v-model学习', msg1: '', msg2: '' } } } </script> <style scoped> .p1{ text-align: left; } .spancss1{ float: left; } </style>
4. Used in components v-model
4.1 自定义组件v-model
一个组件上的 v-model 默认会利用名为 value 的 prop 和名为 input 的事件,但是像输入框、单选框、复选框等类型的输入控件可能会将 value 特性用于不同的目的。本文以自定义输入框和onchange事件为例,在输入框输入值,当光标离开以后输入值会输出绑定到置顶位置:
1. 在index中声明组件(在index声明的组件为全局组件,全局可用):
Vue.component('base-text', { model: { prop: 'value', event: 'change' }, props: { checked: Boolean }, template: `<input type="text" id="inputs" v-on:change="$emit('change', $event.target.value)">` }); =
2. 在要使用该组件的插件中声明:
<template> <div> <p class="p1">{{title}}</p> <div class="spancss1"> <base-text v-model="msg"></base-text><br/> <label>你输入了:</label>{{msg}} </div> </div> </template> <script> export default { name:"v-model", data(){ return { title: 'v-model学习', msg: '' } } } </script> <style scoped> .p1{ text-align: left; } .spancss1{ float: left; } </style>
3. 结果示例如下:
相关推荐:
The above is the detailed content of Analysis of v-model directive in Vue (with code). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










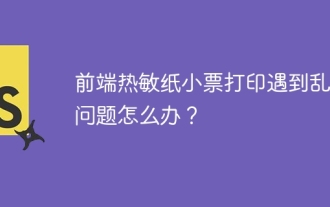
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
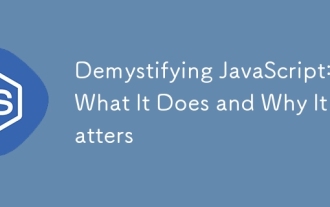
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
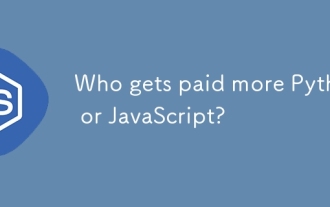
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
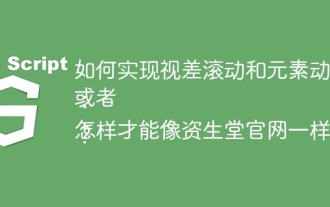
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
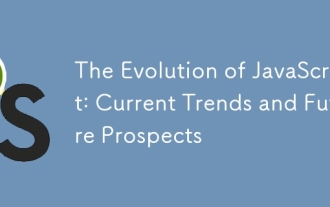
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
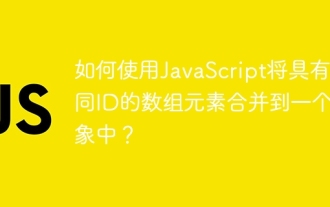
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
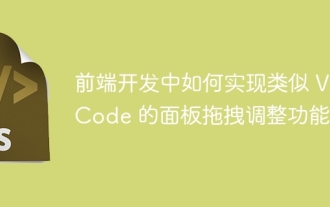
Explore the implementation of panel drag and drop adjustment function similar to VSCode in the front-end. In front-end development, how to implement VSCode similar to VSCode...
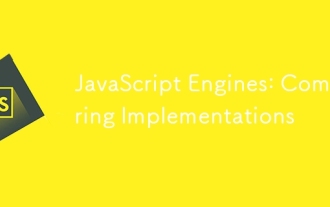
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
