How to write high-quality JS code_Basic knowledge
I want to write an efficient JavaScript library but have no idea how to start;
I tried reading other people’s class libraries, but I seemed to understand them;
I plan to study the advanced functions of js, but the content in the authoritative book is too scattered,
Even though I remember the "usage", I don't think about the "method" when it comes to "using" it.
Maybe you are like me, there seems to be an invisible force constraining our plans, making us repeatedly think of the limitations of knowledge, causing us to stand still and find it difficult to move forward.
During this period, the pressure doubled due to various assignments, course design, and experimental reports. It's rare to squeeze out a little time, never sleep in, organize and summarize the books I read in the past, just to be closer to writing my own class library.
This article refers to "Javascript Language Essence" and "Effective JavaScript". The examples have been debugged, and after understanding them, I want to make some "profound" principles a little simpler.
1. Variable scope
Scope is like oxygen to programmers. It's everywhere, and you often don't even think about it. But when it is polluted (e.g. using global objects), you can feel suffocated (e.g. application becomes less responsive). JavaScript's core scoping rules are simple, well-designed, and powerful. Using JavaScript effectively requires mastering some basic concepts of variable scope and understanding some edge cases that can lead to elusive and nasty problems.
1.1 Use global variables as little as possible
Javascript makes it easy to create variables in the global namespace. Creating a global variable is effortless because it does not require any form of declaration and is automatically accessed by all code throughout the program.
For beginners like us, when we encounter certain needs (for example, the transmitted data is recorded, used when waiting for a certain function to be called at a certain time; or a certain function is frequently used), we do not hesitate to think of global functions, or even The C language I learned in my freshman year is too deeply rooted in process-oriented thinking, and the system is neatly full of functions. Defining global variables pollutes the shared public namespace and can lead to unexpected naming conflicts. Global variables are also detrimental to modularity because they lead to unnecessary coupling between independent components in a program. Seriously speaking, too many globals (including style sheets, directly defining the style of div or a) will become a catastrophic error when integrated into a multi-person development process. That's why all of jQuery's code is wrapped in an anonymous expression that executes immediately - a self-calling anonymous function. When the browser loads the jQuery file, the self-calling anonymous function immediately starts executing and initializes each module of jQuery to avoid damaging and contaminating global variables and affecting other codes.
(function(window,undefined){
var jQuery = ...
//...
window.jQuery = window.$ = jQuery;
})(window);
In addition, you may think that it is more convenient to "write how you want first and organize it later", but good programmers will constantly pay attention to the structure of the program, continue to classify related functions and separate irrelevant components. and include these behaviors as part of the programming process.
Since the global namespace is the only way for independent components in a JavaScript program to interact, the use of globally named controls is inevitable. Components or libraries have to define some global variables. for use by other parts of the program. Otherwise it's better to use local variables.
this.foo ;//undefined
foo = "global foo";
this.foo ;//"global foo"
var foo = "global foo";
this.foo = "changed";
foo ;//changed
The global namespace of JavaScript is also exposed to a global object accessible in the global scope of the program, which serves as the initial value of the this keyword. In web browsers, global objects are bound to the global window variable. This means that you have two ways to create a global variable: declare it using var in the global scope, or add it to the global object. The advantage of using var declaration is that it can clearly express the impact of global variables in the program scope.
Given that references to bound global variables can cause runtime errors, keeping scopes clear and concise will make it easier for users of your code to understand which global variables the program declares.
Since the global object provides a dynamic response mechanism for the global environment, you can use it to query a running environment and detect which features are available on this platform.
eg.ES5 introduces a global JSON object to read and write data in JSON format.
if(!this.JSON){
This.JSON = {
parse: ..,
stringify: ... }
}
The original design of the data structure course simulated the basic operations of strings, requiring that methods provided by the language itself could not be used. JavaScript implements the basic operations on arrays very well. If it is just for general learning needs, the idea of simulating the methods provided by the language itself is good, but if you really invest in development, there is no need to consider using JavaScript's built-in methods in the first place.
1.2 Avoid using with
The with statement provides any "convenience" that makes your application unreliable and inefficient. We need to call a series of methods on a single object in sequence. Using the with statement can easily avoid repeated references to objects:
var widget = new Widget();
with(widget){
setBackground("blue");
setForeground("white");
setText("Status : " info);
show();
}
}
with(Math){
return min(round(x),sqrt(y));//Abstract reference
}
}
Instead of with language, the simple way is to bind the object to a short variable name.
function status(info){
var w = new Widget();
w.setBackground("blue");
w.setForeground("white");
w.setText("Status : " info);
w.show();
}
In other cases, the best approach is to explicitly bind the local variable to the relevant property.
function f(x,y){
var min = Math.min,
round = Math.round,
sqrt = Math.sqrt; Return min(round(x),sqrt(y));
}
1.3 Proficient in closures
There is a single concept for understanding closures:
a) JavaScript allows you to reference variables defined outside the current function.
var magicIngredient = "peanut butter";
function make(filling){
return magicIngredient " and " filling;
}
Return make("jelly");
}
makeSandwich();// "peanut butter and jelly"
b) Even if the external function has returned, the current function can still reference the variables defined in the external function
var magicIngredient = "peanut butter";
function make(filling){
return magicIngredient " and " filling;
}
Return make;
}
var f = sandwichMaker();
f ("jelly"); // "Peanut Butter and Jelly"
f("bananas"); // "peanut butter and bananas"
f("mallows"); // "peanut butter and mallows"
The make function is a closure, and its code refers to two external variables: magicIngredient and filling. Whenever the make function is called, its code can reference these two variables because the closure stores these two variables.
A function can reference any variable within its scope, including parameters and external function variables. We can take advantage of this to write a more general sandwichMaker function.
function make(filling){
return magicIngredient " and " filling;
}
Return make;
}
var f = sandwichMaker("ham");
f("cheese"); // "ham and cheese"
f("mustard"); // "ham and mustard"
Closures are one of JavaScript’s most elegant and expressive features and are at the heart of many idioms.
c) Closures can update the value of external variables. In fact, closures store references to external variables, not copies of their values. Therefore, updates can be made for any closure that has access to these external variables.
function box(){
var val = undefined;
Return {
set: function(newval) {val = newval;},
get: function (){return val;},
type: function(){return typeof val;}
};
}
var b = box();
b.type(); //undefined
b.set(98.6);
b.get();//98.6
b.type();//number
This example produces an object containing three closures. These three closures are set, type and get properties. They all share access to the val variable. The set closure updates the value of val. Then call get and type to view the updated results.
1.4 Understanding variable declaration improvements
Javascript supports this method of scoping (the reference to the variable foo will be bound to the scope closest to the declaration of the foo variable), but does not support block-level scoping (the scope of the variable definition is not the closest enclosing scope) statement or block of code).
Not understanding this feature will lead to some subtle bugs:
function isWinner(player,others){
var highest = 0;
for(var i = 0,n = others.length ;i
If(player.score > highest){
highest = player.score; }
}
Return player.score > highest;
}
1.5 Beware of awkward scoping of named function expressions
var f = function(x){ return x*2; }
//....
Return find(tree.left, key) ||
find(tree.right,key); }
var f= function(){
Return constructor();
};
f();//{}(in ES3 environments)
This program looks like it will generate null, but it will actually generate a new object.
Because the scope of the named function variable inherits Object.prototype.constructor (that is, the constructor of Object), just like the with statement, this scope will be affected by the dynamic change of Object.prototype. The way to avoid objects polluting the scope of function expressions in your system is to avoid adding properties to Object.prototype at any time, and to avoid using any local variables with the same name as standard Object.prototype properties.
Another shortcoming in popular JavaScript engines is the promotion of declarations of named function expressions.
var f = function g(){return 17;}
g(); //17 (in nonconformat environment)
Some JavaScript environments even treat the two functions f and g as different objects, resulting in unnecessary memory allocation.
1.6 Beware of awkward scope declarations for local block functions
function f() {return "global" ; }
function test(x){
Function f(){return "local";}
var result = [];
If(x){
result.push(f());
}
result.push(f());
result result;
}
test(true); //["local","local"]
test(false); //["local"]
function f() {return "global" ; }
function test(x){
var result = [];
If(x){
function f(){return "local";}
result.push(f());
}
result.push(f());
result result;
}
test(true); //["local","local"]
test(false); //["local"]
Javascript does not have block-level scope, so the scope of internal function f should be the entire test function. This is true for some JavaScript environments, but not all JavaScript environments. JavaScript implementations report such functions as errors in strict mode (a program in strict mode with a local block function declaration will report it as a syntax error). There are Helps detect non-portable code and give more sensible and reliable semantics to local block function declarations for future versions of the standard. For this situation, you can consider declaring a local variable in the test function pointing to the global function f.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
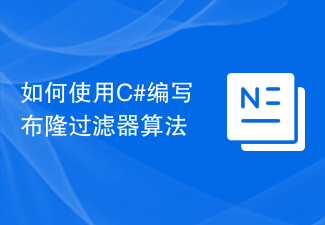
How to use C# to write a Bloom filter algorithm. The Bloom Filter (BloomFilter) is a very space-efficient data structure that can be used to determine whether an element belongs to a set. Its basic idea is to map elements into a bit array through multiple independent hash functions and mark the bits of the corresponding bit array as 1. When judging whether an element belongs to the set, you only need to judge whether the bits of the corresponding bit array are all 1. If any bit is 0, it can be judged that the element is not in the set. Bloom filters feature fast queries and
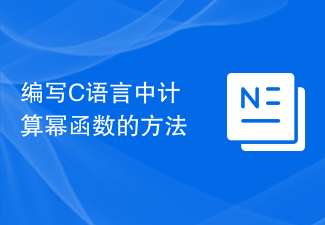
How to write exponentiation function in C language Exponentiation (exponentiation) is a commonly used operation in mathematics, which means multiplying a number by itself several times. In C language, we can implement this function by writing a power function. The following will introduce in detail how to write a power function in C language and give specific code examples. Determine the input and output of the function The input of the power function usually contains two parameters: base and exponent, and the output is the calculated result. therefore, we
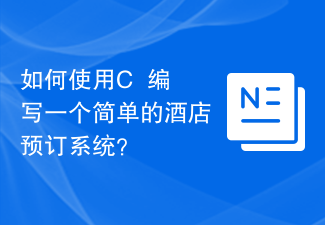
The hotel reservation system is an important information management system that can help hotels achieve more efficient management and better services. If you want to learn how to use C++ to write a simple hotel reservation system, then this article will provide you with a basic framework and detailed implementation steps. Functional Requirements of a Hotel Reservation System Before developing a hotel reservation system, we need to determine the functional requirements for its implementation. A basic hotel reservation system needs to implement at least the following functions: (1) Room information management: including room type, room number, room
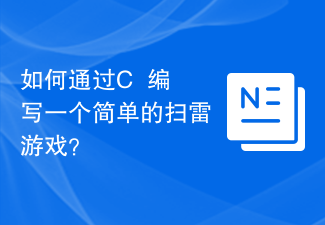
How to write a simple minesweeper game in C++? Minesweeper is a classic puzzle game that requires players to reveal all the blocks according to the known layout of the minefield without stepping on the mines. In this article, we will introduce how to write a simple minesweeper game using C++. First, we need to define a two-dimensional array to represent the map of the Minesweeper game. Each element in the array can be a structure used to store the status of the block, such as whether it is revealed, whether there are mines, etc. In addition, we also need to define
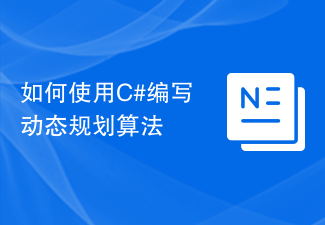
How to use C# to write dynamic programming algorithm Summary: Dynamic programming is a common algorithm for solving optimization problems and is suitable for a variety of scenarios. This article will introduce how to use C# to write dynamic programming algorithms and provide specific code examples. 1. What is a dynamic programming algorithm? Dynamic Programming (DP) is an algorithmic idea used to solve problems with overlapping subproblems and optimal substructure properties. Dynamic programming decomposes the problem into several sub-problems to solve, and records the solution to each sub-problem.
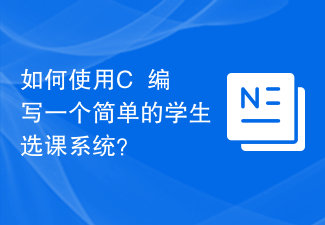
How to use C++ to write a simple student course selection system? With the continuous development of technology, computer programming has become an essential skill. In the process of learning programming, a simple student course selection system can help us better understand and apply programming languages. In this article, we will introduce how to use C++ to write a simple student course selection system. First, we need to clarify the functions and requirements of this course selection system. A basic student course selection system usually includes the following parts: student information management, course information management, selection
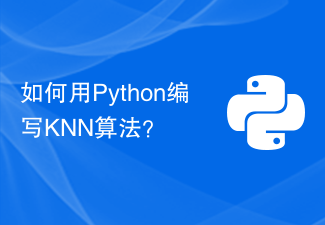
How to write KNN algorithm in Python? KNN (K-NearestNeighbors, K nearest neighbor algorithm) is a simple and commonly used classification algorithm. The idea is to classify test samples into the nearest K neighbors by measuring the distance between different samples. This article will introduce how to write and implement the KNN algorithm using Python and provide specific code examples. First, we need to prepare some data. Suppose we have a two-dimensional data set, and each sample has two features. We divide the data set into
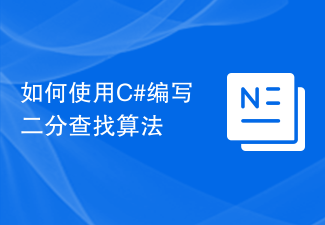
How to use C# to write a binary search algorithm. The binary search algorithm is an efficient search algorithm. It finds the position of a specific element in an ordered array with a time complexity of O(logN). In C#, we can write the binary search algorithm through the following steps. Step 1: Prepare data First, we need to prepare a sorted array as the target data for the search. Suppose we want to find the position of a specific element in an array. int[]data={1,3,5,7,9,11,13
