


Learn a new PHP function every day (2) array_diff()/array_diff_key()/array_diff_assoc()_PHP tutorial
Learn a new php function every day (2) array_diff()/array_diff_key()/array_diff_assoc()
array_diff( array array1, array array2 [, array …] )
Description
array_diff() returns an array containing all values in array1 that are not in any other argument array. Note that the key names remain unchanged.
Liezi
<code><!--?php $array1 = array("a" =--> "green", "red", "blue", "red"); $array2 = array("b" => "green", "yellow", "red"); $result = array_diff($array1, $array2); print_r($result); ?> </code>
The output result is
<code>Array([1] => blue) </code>
Attention
Two cells are considered identical only if (string) $elem1 === (string) $elem2. That is, when the string expressions are the same. Note that this function only checks one dimension of a multidimensional array. Of course you can use array_diff($array1[0], $array2[0]); to check deeper dimensions.
array array_diff_assoc ( array array1, array array2 [, array …] )
Description
array_diff_assoc() returns an array containing all values in array1 that are not in any other argument array. Note that unlike array_diff(), key names are also used for comparison.
Lie Zi
<code><!--?php $array1 = array("a" =--> "green", "b" => "brown", "c" => "blue", "red"); $array2 = array("a" => "green", "yellow", "red"); $result = array_diff_assoc($array1, $array2); print_r($result); ?> </code>
Output
<code>Array( [b] => brown [c] => blue [0] => red ) </code>
In the above example, you can see that the key-value pair "a" => "green" is present in both arrays, so it is not included in the output of this function. In contrast, the key-value pair 0 => "red" appears in the output because the key of "red" in the second parameter is 1.
Liezi 2
<code><!--?php $array1 = array(0, 1, 2); $array2 = array("00", "01", "2"); $result = array_diff_assoc($array1, $array2); print_r($result); ?--> </code>
Output
<code>Array( [0] => 0 [1] => 1 ) </code>
Two values in the key => value pair are considered equal only if (string)
Attention
Note: Note that this function only checks one dimension of the multi-dimensional array. Of course you can use array_diff_assoc($array1[0], $array2[0]); to check deeper dimensions.
array array_diff_key ( array $array1 , array $array2 [, array $… ] )
Description
Compare the key names in array1 with array2 and return items with different key names. This function is the same as array_diff() except that the comparison is based on keys rather than values.
Returns an array containing the values of all keys that appear in array1 but do not appear in any other parameter array.
Liezi
<code><!--?php $array1 = array('blue' =--> 1, 'red' => 2, 'green' => 3, 'purple' => 4); $array2 = array('green' => 5, 'blue' => 6, 'yellow' => 7, 'cyan' => 8); var_dump(array_diff_key($array1, $array2)); ?> </code>
Output
<code>array(2) { ["red"]=> int(2) ["purple"]=> int(4) } </code>
Two key names in a key => value pair are considered equal only if (string)$key1 === (string) $key2 . In other words, strict type checking is performed, so the string representations must be exactly the same.
array array_intersect ( array $array1 , array $array2 [, array $ … ] )
Description
array_intersect() returns an array containing all values in array1 that are also present in all other argument arrays. Note that the key names remain unchanged.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










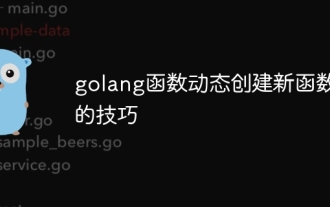
Go language provides two dynamic function creation technologies: closure and reflection. closures allow access to variables within the closure scope, and reflection can create new functions using the FuncOf function. These technologies are useful in customizing HTTP routers, implementing highly customizable systems, and building pluggable components.
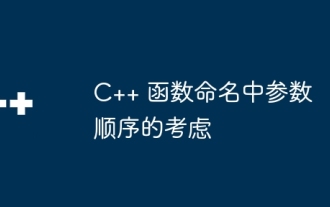
In C++ function naming, it is crucial to consider parameter order to improve readability, reduce errors, and facilitate refactoring. Common parameter order conventions include: action-object, object-action, semantic meaning, and standard library compliance. The optimal order depends on the purpose of the function, parameter types, potential confusion, and language conventions.
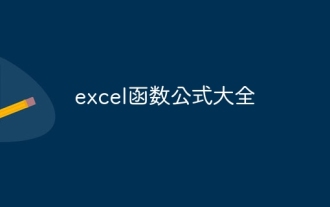
1. The SUM function is used to sum the numbers in a column or a group of cells, for example: =SUM(A1:J10). 2. The AVERAGE function is used to calculate the average of the numbers in a column or a group of cells, for example: =AVERAGE(A1:A10). 3. COUNT function, used to count the number of numbers or text in a column or a group of cells, for example: =COUNT(A1:A10) 4. IF function, used to make logical judgments based on specified conditions and return the corresponding result.
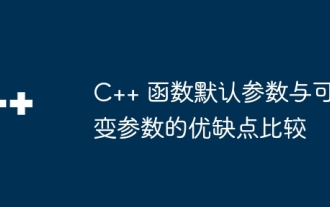
The advantages of default parameters in C++ functions include simplifying calls, enhancing readability, and avoiding errors. The disadvantages are limited flexibility and naming restrictions. Advantages of variadic parameters include unlimited flexibility and dynamic binding. Disadvantages include greater complexity, implicit type conversions, and difficulty in debugging.
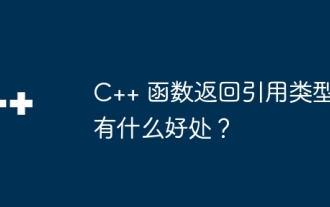
The benefits of functions returning reference types in C++ include: Performance improvements: Passing by reference avoids object copying, thus saving memory and time. Direct modification: The caller can directly modify the returned reference object without reassigning it. Code simplicity: Passing by reference simplifies the code and requires no additional assignment operations.
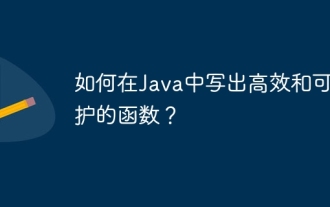
The key to writing efficient and maintainable Java functions is: keep it simple. Use meaningful naming. Handle special situations. Use appropriate visibility.
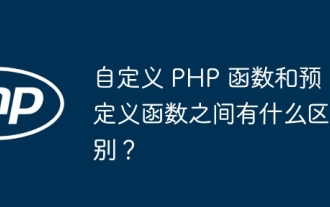
The difference between custom PHP functions and predefined functions is: Scope: Custom functions are limited to the scope of their definition, while predefined functions are accessible throughout the script. How to define: Custom functions are defined using the function keyword, while predefined functions are defined by the PHP kernel. Parameter passing: Custom functions receive parameters, while predefined functions may not require parameters. Extensibility: Custom functions can be created as needed, while predefined functions are built-in and cannot be modified.
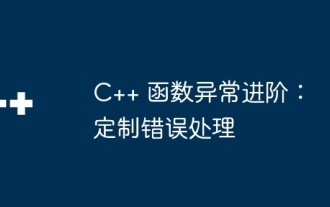
Exception handling in C++ can be enhanced through custom exception classes that provide specific error messages, contextual information, and perform custom actions based on the error type. Define an exception class inherited from std::exception to provide specific error information. Use the throw keyword to throw a custom exception. Use dynamic_cast in a try-catch block to convert the caught exception to a custom exception type. In the actual case, the open_file function throws a FileNotFoundException exception. Catching and handling the exception can provide a more specific error message.
