PHP student management system implementation_PHP tutorial
PHP student management system implementation
The school recently opened a PHP course, so I wrote an assignment and would like to share it. . .
These are very simple things that novices can use...,,
Omit some front-end code,,,
The first is the login verification:
<?php session_start(); $user = $_POST['userName']; $pass = $_POST['passWord']; $_SESSION['user'] = $user; /*$Enter = $_POST['Login_undo']; 管理员登录的校验*/ $flag = false; if($user == "Admin"&& $pass == "root") { setcookie("userName",$user,time()+1200); setcookie("userName",$pass,time()+1200); $flag = true; header('location:adminPage.php?user=' . $user); } else header('location:Login.php?login=relog'); /* // 学生登录免校验 if($Enter) header('location:StuPage.php'); */
Then there is the registration verification:
<?php session_start(); $s_ID = $_POST['s_ID']; $Name = $_POST['Name']; $IDcard = $_POST['IDcard']; $Major = $_POST['Major']; $sex = $_POST['sex']; $_SESSION['student'][$s_ID]['s_ID'] = $s_ID; $_SESSION['student'][$s_ID]['Name'] = $Name; $_SESSION['student'][$s_ID]['IDcard'] = $IDcard; $_SESSION['student'][$s_ID]['Major'] = $Major; $_SESSION['student'][$s_ID]['sex'] = $sex; header('location:tisi.html'); /*foreach($_SESSION['student'] as $v) { if($v == $s_ID) { header("location:stu_reg.php?action=look&msg=更新&user=employee&empno=" . $empno . "&idcard=" . $idcard); } else header("location:stu_reg.php?action=look&msg=增加&user=employee&empno=" . $empno . "&idcard=" . $idcard); }*/
Graduation operation and adding history verification:
<?php session_start(); $s_ID=$_GET['s_ID']; $_SESSION['history'][$s_ID]['s_ID']=$s_ID; $_SESSION['history'][$s_ID]['Name']=$_SESSION['student'][$s_ID]['Name']; $_SESSION['history'][$s_ID]['IDcard']=$_SESSION['student'][$s_ID]['IDcard']; $_SESSION['history'][$s_ID]['sex']=$_SESSION['student'][$s_ID]['sex']; $_SESSION['history'][$s_ID]['Major']=$_SESSION['student'][$s_ID]['Major']; unset($_SESSION['student'][$s_ID]); header('location:graduate.php?user=Admin&action=delete');
Any keyword query:
<?php session_start(); $search=$_POST['search']; unset($_SESSION['search']); /*echo '<pre class="code">'; var_dump($_POST['search']); return ;*/ foreach ($_SESSION['student'] as $k1 => $value) { # code... if($search==$_SESSION['student'][$k1]['s_ID']||$search==$_SESSION['student'][$k1]['IDcard']||$search==$_SESSION['student'][$k1]['Name']||$search==$_SESSION['student'][$k1]['sex']||$search==$_SESSION['student'][$k1]['Major']){ $i = 1; $stu = $_SESSION['student'][$k1]['s_ID']; $_SESSION['search'][$stu] = $stu; } } if(isset($i)) header("location:stu_Query.php?user=Admin&action=search"); else header("location:stu_Query.php?user=Admin&action=q_error");
Traverse student information:
<!DOCTYPE HTML> <html> <head> <link href="file/Style.Css" rel="stylesheet" type="text/css" /> </head> <body> <table width="100%" border="0" cellpadding="1" cellspacing="1" class="css_table" bgcolor='#E1E1E1'> <?php session_start(); $user = isset($_SESSION['user'])?$_SESSION['user']:''; if($user =='Admin'){ if(isset($_SESSION['student'])){ foreach($_SESSION['student'] as $k1) { echo "<tr>"; foreach($k1 as $k2=>$k3) { echo "<td>" ; if($k2=='s_ID') {echo "学号:" ;} else if($k2=='IDcard'){echo "身份证号:";}else if($k2=='sex'){echo "性别:";}else if($k2=='Name'){echo "姓名:";}else if($k2 =='Major'){echo "专业:";}; echo "</td>"; echo "<td>"; if($k2=='s_ID') $s_ID=$k3; echo "$k3"; echo "</td>"; } } } } ?> </table> </body> </html>
Update data page and verification:
<!DOCTYPE HTML> <!-- 使用HTML5规范,省略多余部分 --> <html> <head> <?php session_start(); $user = isset($_SESSION['user'])?$_SESSION['user']:''; $action = isset($_GET['action'])?$_GET['action']:''; ?> <link href="file/Style.Css" rel="stylesheet" type="text/css" /> </head> <body> <?php if($user =='Admin'&&$action==''){ ?> <table width="100%" border="0" cellpadding="3" cellspacing="1" class="css_table" bgcolor='#E1E1E1'> <tr class="css_menu"> <td colspan="3"> <table width="100%" border="0" cellpadding="4" cellspacing="0" class="css_main_table"> <tr> <td class="css_main">注意</td> </tr> </table> </td> </tr> <tr> <td class="css_col11"><strong><font color=#50691B>一旦确定不可更改</font></strong></td> </tr> </table> <?php }else if ($action == 'change') {?> </div> <?php }else if ($action == 'enchange') { # code... echo "<h1 id="已经改变">已经改变</h1>"; }?> </body> </html>
<?php session_start(); $s_ID = $_POST['c_ID']; $Name = $_POST['Name']; $Major = $_POST['Major']; $sex = $_POST['sex']; $_SESSION['student'][$s_ID]['s_ID'] = $s_ID; $_SESSION['student'][$s_ID]['Name'] = $Name; $_SESSION['student'][$s_ID]['Major'] = $Major; $_SESSION['student'][$s_ID]['sex'] = $sex; header("location:stu_Update.php?action=enchange");
Some front-end design:
<!DOCTYPE HTML> <!-- 使用HTML5规范 --> <html> <head> <title>main</title> <link href="file/Style.Css" rel="stylesheet" type="text/css" /> </head> <body> <?php session_start(); ?> <?php $user = isset($_SESSION['user'])?$_SESSION['user']:""; ?> <?php if($user == "") { // header("location:Login.php"); die("<script> if(typeof(parent) != 'undefined'){ parent.window.location = 'Login.php'; }else{ window.location.href = 'Login.php'; } </script>"); } ?> <table width=100% border=0 cellpadding=3 cellspacing=1 class=css_table bgcolor='#E1E1E1'> <tr class=css_menu> <td colspan=3> <table width=100% border=0 cellpadding=4 cellspacing=0 class=css_main_table> <tr> <td class=css_main>欢迎<?php echo "$user";?></td> </tr> </table> </td> </tr> <tr> <td class="css_col11"><strong><font color = "#0000FF">登录cookie有效时间为1200秒</strong></td> </tr> </table> <table width="100%" border="0" cellpadding="3" cellspacing="1" class="css_table" bgcolor='#E1E1E1'> <tr class="css_menu"> <td colspan="3"> <table width="100%" border="0" cellpadding="4" cellspacing="0" class="css_main_table"> <tr> <td class="css_main">联系方式</td> </tr> </table> </td> </tr> <tr> <td class="css_col11"><strong><font color=#50691B>Blog:http://blog.csdn.net/p641290710</font></strong></td> <td class="css_col11"><strong><font color=#50691B>Email:pengjunweiright@163.com</font></strong></td> </tr> </table> <table width=100% border=0 cellpadding=3 cellspacing=1 class=css_table bgcolor='#E1E1E1'> <tr class=css_menu> <td colspan=3> <table width=100% border=0 cellpadding=4 cellspacing=0 class=css_main_table> <tr> <td class=css_main>Github</td> </tr> </table> </td> </tr> <tr> <td class="css_col11"><strong><font color=#50691B>点击进入本人Github</font></strong></td> </tr> </table> </body> </html>

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










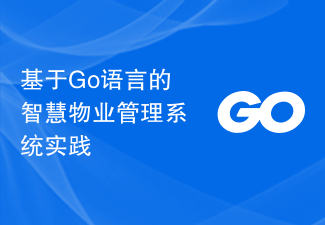
With technological advancement and social development, smart property management systems have become an indispensable part of modern urban development. In this process, the smart property management system based on Go language has attracted much attention due to its advantages such as efficiency, reliability, and speed. This article will introduce the practice of our team’s smart property management system using Go language. 1. Requirements analysis Our team mainly develops this property management system for a real estate company. Its main task is to connect property management companies and residents to facilitate the management of property management companies, and also to allow residents to
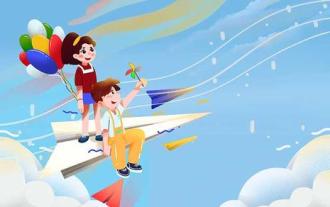
Recommended computers suitable for students majoring in geographic information science 1. Recommendation 2. Students majoring in geographic information science need to process large amounts of geographic data and conduct complex geographic information analysis, so they need a computer with strong performance. A computer with high configuration can provide faster processing speed and larger storage space, and can better meet professional needs. 3. It is recommended to choose a computer equipped with a high-performance processor and large-capacity memory, which can improve the efficiency of data processing and analysis. In addition, choosing a computer with larger storage space and a high-resolution display can better display geographic data and results. In addition, considering that students majoring in geographic information science may need to develop and program geographic information system (GIS) software, choose a computer with better graphics processing support.
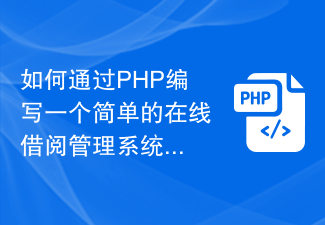
How to write a simple online lending management system through PHP requires specific code examples. Introduction: With the advent of the digital age, library management methods have also undergone tremendous changes. Traditional manual recording systems are gradually being replaced by online borrowing management systems. Online borrowing management systems greatly improve efficiency by automating the process of borrowing and returning books. This article will introduce how to use PHP to write a simple online lending management system and provide specific code examples. 1. System requirements analysis before starting to write the online borrowing management system
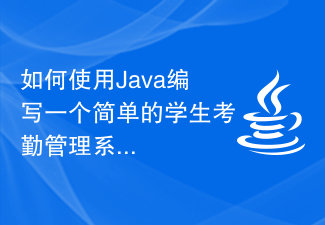
How to write a simple student attendance management system using Java? With the continuous development of technology, school management systems are also constantly updated and upgraded. The student attendance management system is an important part of it. It can help the school track students' attendance and provide data analysis and reports. This article will introduce how to write a simple student attendance management system using Java. 1. Requirements Analysis Before starting to write, we need to determine the functions and requirements of the system. Basic functions include registration and management of student information, recording of student attendance data and
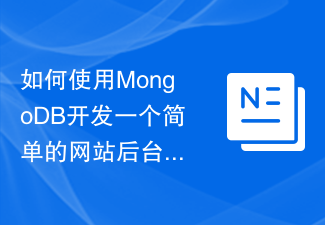
How to use MongoDB to develop a simple website backend management system. With the development of the Internet, the use and management of websites have become more and more important. In order to facilitate website administrators to manage website content in the background, it is essential to develop a simple and efficient website background management system. This article will introduce how to use MongoDB to develop a simple website backend management system, and demonstrate it through specific code examples. Preparation First, we need to ensure that the MongoDB database has been installed and configured. specific

How to create a MySQL table structure suitable for school management systems? The school management system is a complex system involving multiple modules and functions. In order to achieve its functional requirements, it is necessary to design an appropriate database table structure to store data. This article will use MySQL as an example to introduce how to create a table structure suitable for school management systems and provide relevant code examples. School information table (school_info) The school information table is used to store basic information about the school, such as school name, address, contact number, etc. CREATETABL
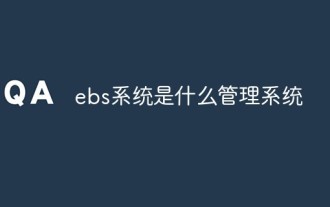
The ebs system is an electronic brake control management system. It is an electronic control system that completely uses electronically controlled pneumatic braking to improve braking comfort and safety. The components of the ebs system: 1. EBS system brake signal sensor; 2. EBS system single-channel control module; 3. EBS system dual-channel control module; 4. EBS system electronically controlled trailer control valve.
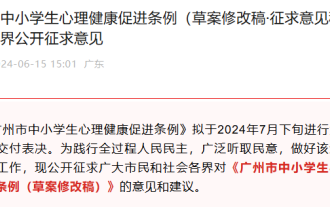
According to news on June 16, on June 15, the official website and public account of the Guangzhou Municipal People’s Congress posted the “Regulations on the Promotion of Mental Health of Primary and Secondary School Students in Guangzhou (Revised Draft and Draft for Comments)” to solicit opinions and suggestions from all walks of life. Among them, Article 14 of the "Regulations" is titled "Preventing Mobile Phones and Other Intelligent Terminal Products from Entering Campuses" and the specific provisions are as follows: Parents or other guardians of primary and secondary school students should strictly restrict their children's use of mobile phones and other intelligent terminal products. Children of products should regulate the place, time period, duration, frequency, content, functions, permissions and other matters of use, and cooperate with the school to prohibit or restrict students from using mobile phones and other smart terminal products on campus. Schools may prohibit students from bringing mobile phones and other smart terminal products into the school or using them on campus.
