PHP overloaded array operator_PHP tutorial
PHP provides many interfaces to implement some very specific functions. For example, if you want to use an object as an array, you only need to implement the ArrayAccess interface. When you want to use an object in foreach When , you only need to implement the Iterator interface. Here is an example
class BtstoreRoot { /** * 根结点 * @var BtstoreElement */ static $root; } class BtstoreElement implements ArrayAccess, Iterator { /** * 当前所代表的目录 * @var string */ private $dataDir; /** * 当前所代表的数据 * @var array */ private $arrData; /** * 构造函数 * @param string $dataDir * @param array $arrData */ function __construct($dataDir, $arrData) { $this->dataDir = ''; $this->arrData = array (); if (! empty ( $dataDir ) && is_dir ( $dataDir )) { $this->dataDir = $dataDir; } if (! empty ( $arrData )) { $this->arrData = $arrData; } } function __get($key) { if (isset ( $this->arrData [$key] )) { $data = $this->arrData [$key]; if (is_array ( $data ) && ! is_object ( $data )) { $data = new BtstoreElement ( '', $data ); } return $data; } if (! empty ( $this->dataDir )) { $path = $this->dataDir . '/' . $key; if (is_dir ( $path )) { $data = new BtstoreElement ( $path, null ); $this->arrData [$key] = $data; return $data; } if (is_file ( $path )) { $content = file_get_contents ( $path ); $arrData = unserialize ( $content ); $data = new BtstoreElement ( '', $arrData ); $this->arrData [$key] = $data; return $data; } } trigger_error ( "undefined index:$key" ); } function __isset($key) { if (isset ( $this->arrData [$key] )) { return true; } if (file_exists ( $this->dataDir . '/' . $key )) { return true; } return false; } function toArray() { return $this->arrData; } /* (non-PHPdoc) * @see ArrayAccess::offsetExists() */ public function offsetExists($offset) { return $this->__isset ( $offset ); } /* (non-PHPdoc) * @see ArrayAccess::offsetGet() */ public function offsetGet($offset) { return $this->__get ( $offset ); } /* (non-PHPdoc) * @see ArrayAccess::offsetSet() */ public function offsetSet($offset, $value) { trigger_error ( 'offsetSet not implemented by BtstoreElement' ); } /* (non-PHPdoc) * @see ArrayAccess::offsetUnset() */ public function offsetUnset($offset) { trigger_error ( 'offsetUnset not implemented by BtstoreElement' ); } /* (non-PHPdoc) * @see Iterator::current() */ public function current() { return current ( $this->arrData ); } /* (non-PHPdoc) * @see Iterator::next() */ public function next() { return next ( $this->arrData ); } /* (non-PHPdoc) * @see Iterator::key() */ public function key() { return key ( $this->arrData ); } /* (non-PHPdoc) * @see Iterator::valid() */ public function valid() { $data = current ( $this->arrData ); return ! empty ( $data ); } /* (non-PHPdoc) * @see Iterator::rewind() */ public function rewind() { reset ( $this->arrData ); } } /** * 获取一个BtstoreElement对象 * @return BtstoreElement */ function btstore_get() { if (empty ( BtstoreRoot::$root )) { BtstoreRoot::$root = new BtstoreElement ( ScriptConf::BTSTORE_ROOT, null ); } return BtstoreRoot::$root; }

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










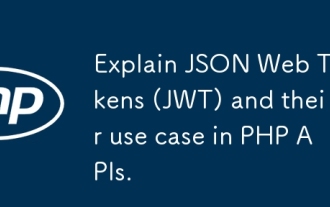
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
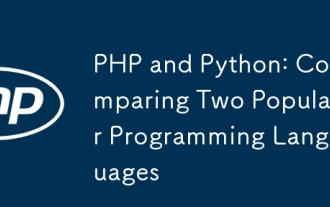
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
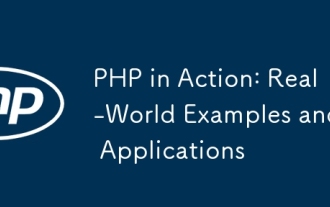
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
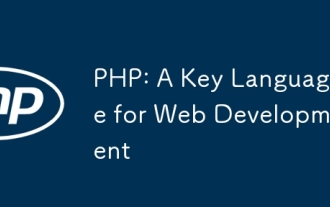
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
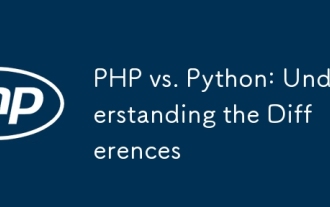
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
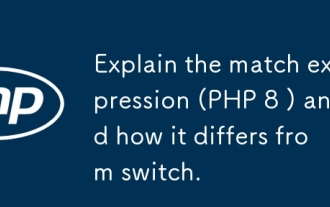
In PHP8, match expressions are a new control structure that returns different results based on the value of the expression. 1) It is similar to a switch statement, but returns a value instead of an execution statement block. 2) The match expression is strictly compared (===), which improves security. 3) It avoids possible break omissions in switch statements and enhances the simplicity and readability of the code.
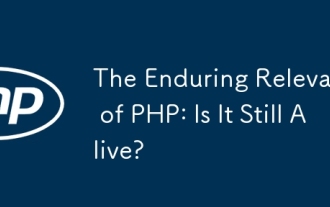
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.
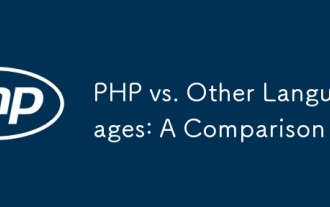
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
