


Discussion: array2xml and xml2array and the mutual conversion of xml and array_PHP tutorial
When PHP is working as a back-end server, it often encounters this situation. It needs to parse the xml file from the front-end and return the data in xml format. In this case, the conversion between xml and the associative array in PHP is Very frequent thing. For example, flex and other client programs often use this method to interact with the server. The following are two methods I have summarized, which greatly simplify the workload of converting xml and array.
/**
*
* 将简单数组转化为简单的xml
* @param string $data 要进行转化的数组
* @param string $tag 要使用的标签
* @example
* $arr = array(
'rtxAccount'=>'aaron','ipAddr'=>'192.168.0.12',
'conferenceList'=>array('conference'=>
array(
array('conferenceId'=>1212,'conferenceTitle'=>'quanshi 444','smeAccount'=>'http://www.jb51.net'),
array('conferenceId'=>454,'conferenceTitle'=>'quanshi meetting','smeAccount'=>'http://www.jb51.net'),
array('conferenceId'=>6767,'conferenceTitle'=>'quanshi meetting','smeAccount'=>'http://www.jb51.net'),
array('conferenceId'=>232323,'conferenceTitle'=>'quanshi uuu','smeAccount'=>'http://www.jb51.net'),
array('conferenceId'=>8989,'conferenceTitle'=>'quanshi meetting','smeAccount'=>'http://www.jb51.net'),
array('conferenceId'=>1234343212,'conferenceTitle'=>'quanshi meetting','smeAccount'=>'http://www.jb51.net')
)
)
);
转化为:
*/
function array2xml($data,$tag = '')
{
$xml = '';
foreach($data as $key => $value)
{
if(is_numeric($key))
{
if(is_array($value))
{
$xml .= "<$tag>";
$xml .= array2xml($value);
$xml .="$tag>";
}
else
{
$xml .= "<$tag>$value$tag>";
}
}
else
{
if(is_array($value))
{
$keys = array_keys($value);
if(is_numeric($keys[0]))
{
$xml .=array2xml($value,$key);
}
else
{
$xml .= "<$key>";
$xml .=array2xml($value);
$xml .= "$key>";
}
}
else
{
$xml .= "<$key>$value$key>";
}
}
}
return $xml;
}
}
xml2array
/**
*
* Convert simple xml into associative array
* @param string $xmlString xml string
* @example
*
Associative array after conversion:
Array
(
[conferenceTitle] => IT Exchange Meeting
[startTime] => 2011-12-19 12:00:00
[rtxAccount] => andy1111111
[ipAddr] => 192.168.1.56
[duration] => 120
[conferenceType] => 1
[invitees] => Array
(
[invitee] => Array
(> 🎜>
[1] = & gt; Array
(
[🎜> [rtxaccount] = & gt; the RTX account number of the invited person 2
[tel] = & gt; 🎜> )
)
)
*/
function xml2array($xmlString = '')
{
$targetArray = array();
$xmlObject = simplexml_load_string($xmlString);
$mixArray = (array)$xmlObject;
foreach($mixArray as $key => $value)
{
if(is_string($value))
{
$targetArray[$key] = $value;
}
if(is_object($value))
{
$targetArray[$key] = xml2array($value->asXML());
}
if(is_array($value))
{
foreach($value as $zkey => $zvalue)
{
if(is_numeric($zkey))
{
$targetArray[$key][] = xml2array($zvalue->asXML());
}
if(is_string($zkey))
{
$targetArray[$key][$zkey] = xml2array($zvalue->asXML());
}
}
}
}
return $targetArray;
}

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










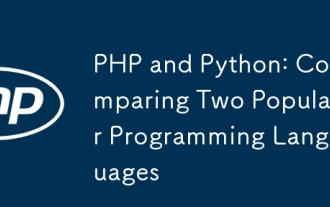
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
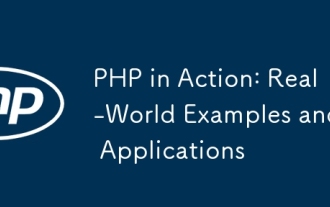
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
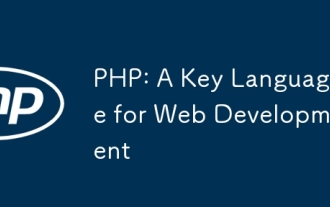
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
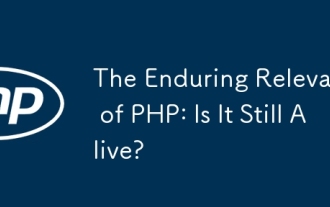
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.
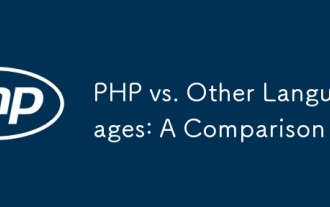
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
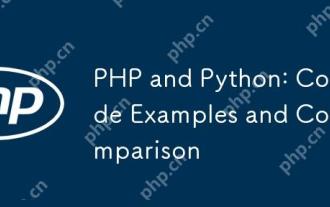
PHP and Python have their own advantages and disadvantages, and the choice depends on project needs and personal preferences. 1.PHP is suitable for rapid development and maintenance of large-scale web applications. 2. Python dominates the field of data science and machine learning.
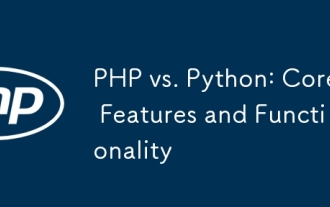
PHP and Python each have their own advantages and are suitable for different scenarios. 1.PHP is suitable for web development and provides built-in web servers and rich function libraries. 2. Python is suitable for data science and machine learning, with concise syntax and a powerful standard library. When choosing, it should be decided based on project requirements.
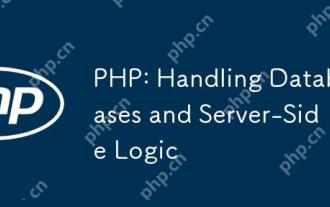
PHP uses MySQLi and PDO extensions to interact in database operations and server-side logic processing, and processes server-side logic through functions such as session management. 1) Use MySQLi or PDO to connect to the database and execute SQL queries. 2) Handle HTTP requests and user status through session management and other functions. 3) Use transactions to ensure the atomicity of database operations. 4) Prevent SQL injection, use exception handling and closing connections for debugging. 5) Optimize performance through indexing and cache, write highly readable code and perform error handling.
