


Understanding and applying polymorphism in PHP [Translation]_PHP Tutorial
What is polymorphism?
Polymorphism is a long word, but it represents a very simple concept.
Polymorphism describes the object-oriented programming model in which classes have different functions while sharing a common interface.
The advantage of polymorphism is that you don't need to know which class it is using, because they all work in the same way with code from different classes.
Polymorphism can be compared to a button in the real world. Everyone knows how to use a button: you just put pressure on it. What a button "really is", however, depends on what it is connected to and the context in which it is used - but the result does not affect how it is used. If your boss tells you to press a button, you already have all the information you need to perform the task.
In the world of programming, polymorphism is used to make applications more modular and extensible. Rather than messy conditional statements describing actions in different classes, you can create interchangeable objects chosen based on your needs. This is the basic goal of polymorphism.
Interfaces
An interface is similar to a class, except that it cannot contain code. An interface can define method names and parameters, but not the content of the methods. Any class that implements an interface must implement all methods defined in the interface. A class can implement multiple interfaces.
Use the "interface" keyword to declare an interface:
interface MyInterface {
// methods
}
is attached to a class using the "implements" keyword (multiple interfaces can be separated by commas):
class MyClass implements MyInterface {
// methods
}
in the interface Methods can be defined in a class just like in a class, except that there is no method body (the part enclosed in curly braces).
interface MyInterface {
public function doThis();
public function doThat ();
public function setName($name);
}
All methods defined here must be included as described in the interface in any class that implements it middle. (Read the code comments below)
//Legal VALID
class MyClass implements MyInterface {
protected $name;
public function doThis() {
// code that does this
}
public function doThat() {
// code that does that
}
public function setName($name) {
$this->name = $name;
}
}
// Illegal INVALID
class MyClass implements MyInterface {
// missing doThis()!
private function doThat() {
// this should be public!
}
public function setName() {
// missing the name argument!
}
}
Abstract Class Abstract Class
Abstract class is a mixture of interface and class. It can define methods just like an interface. A class that inherits from an abstract class must implement all abstract methods defined in the abstract class.
Abstract classes are defined in the same way as classes, but with the abstract keyword appended in front.
abstract class MyAbstract {
// methods
}
and is attached to the class using the 'extends' keyword:
class MyClass extends MyAbstract {
// class methods
}
Just like in a normal class, normal methods as well as any abstract methods (using keyword "abstract") can be added in abstract defined in the class. An abstract method behaves like a method defined in an interface, and extending classes that inherit it must implement exactly the same definition.
abstract class MyAbstract {
public $name;
public function doThis( ) {
// do this
}
abstract public function doThat();
abstract public function setName($name);
}
We assume that you have an Article class responsible for managing articles on your website. It contains information about the article, including: title, author, date, and category.
Like this:
class poly_base_Article {
public $title;
public $author;
public $date;
public $category;
public function __construct($title, $ author, $date, $category = 0) {
$this->title = $title;
$this->author = $author;
$this->date = $date;
$this->category = $category;
}
}
Note: The example classes in this tutorial use the "package_component_Class" naming convention, which is A general method for separating class names into virtual namespaces to avoid naming conflicts.
Now you want to add a method to output information in various formats, such as XML and JSON. You might want to do something like this:
class poly_base_Article {
//. ..
public function write($type) {
$ret = '';
switch($type) {
case 'XML':
$ret = '';
$ret .= '';
$ret .= '' . $obj->author . '';
$ret .= '' . $obj->date . '';
$ret .= '' . $obj->category . '';
$ret .= '';
break;
case 'JSON':
$array = array(' article' => $obj);
$ret = json_encode($array);
break;
}
return $ret;
}
}
This solution is ugly, but it's reliable - at least for now. Ask yourself what will happen in the future, when we need to add more formats? You can keep editing this class and add more and more cases,
but now you are just diluting (FIX ME: diluting) your class.
An important principle of OOP is that a class should do one thing and do it well.
With this in mind, conditional statements should be a red flag that your class is trying to do too many different things. This is where polymorphism comes in.
In our example, two tasks are clearly presented: managing articles and formatting their data. In this tutorial, we'll refactor our formatting code into a new class, and then we'll discover how easy it is to use polymorphism.
Step 2: Define Your Interface
The first thing is that we should define the interface. It is important to try to figure out how to define your interface, because any changes to it will require changes. The code that calls it.
In our example, we will use a simple interface to define a method:
interface poly_writer_Writer {
public function write(poly_base_Article $obj);
}
It’s that simple, we have defined a public method write() which accepts a Article object as parameter. Any class that implements the Writer interface will be sure to have this method.
Tips: If you want to strictly limit the parameter types passed to your methods and functions, you can use type hints, like we have done in the write() method, which can only accept poly_base_Article object types Data
data. Unfortunately, in the current version of PHP, return type hints are not supported, so you need to be careful about the type of the return value.
Step 3: Create Your Implementation
After defining the interface, it’s time to create the class to do the real work. In our case, we need to output two formats. In this way, we need two Writer classes: XMLWriter and JSONWriter. It's entirely up to these classes to extract data from the passed
Article object and then format the information.
The following is an example of the XMLWriter class:
class poly_writer_XMLWriter implements poly_writer_Writer {
public function write(poly_base_Article $obj) {
$ret = '';
$ret .= '';
$ret .= '' . $obj->author . '';
$ret .= '' . $obj->date . '';
$ret .= '' . $obj->category . '';
$ret .= '';
return $ret;
}
}
As you can see from the class definition, we use the implements keyword to implement our interface. The write() method contains functions for formatting to XML.
Now let’s look at the JSONWriter class:
class poly_writer_JSONWriter implements poly_writer_Writer {
public function write(poly_base_Article $obj) {
$array = array('article' => $obj);
return json_encode($array) ;
}
}
Now, our code specific to each format is contained in a separate class. Each class has sole responsibility for handling a specific format and not others. No other part of your application needs to care about how these work in order to use it,
thanks to our interfaces.
Step 4: Use Your Implementation
After our new class is defined, it is time to review our Article class. All the code in the original write() method has been separated , entering our new category.
All our methods need to do now is use these new classes, like this:
class poly_base_Article {
//...
public function write(poly_writer_Writer $writer) {
return $writer->write($this);
}
}
Obtaining a Writer
You may be wondering where to get a Writer object to start, because you need to pass a Writer object to this method.
It’s all up to you, and there are many strategies. For example, you might use a factory class to get the request data and then create an object:
class poly_base_Factory {
public static function getWriter() {
// grab request variable
$format = $_REQUEST['format'];
// construct our class name and check its existence
$class = 'poly_writer_' . $format . 'Writer';
if(class_exists($class)) {
// return a new Writer object
return new $class();
}
// otherwise we fail
throw new Exception('Unsupported format');
}
}
Like I said, depending on your needs, there are There are many other strategies available. In this example, a request variable is used to select which format is to be used. It constructs a class name based on the request variable, checks whether it exists,
and returns a new Writer object. If no class of that name exists, throw an exception and let the client code decide what to do next.
Step 5: Put It All Together
When everything is in place, here is how our client code is put together:
$article = new poly_base_Article('Polymorphism', 'Steve', time(), 0);
try {
$writer = poly_base_Factory::getWriter();
}
catch (Exception $e) {
$writer = new poly_writer_XMLWriter();
}
echo $article->write($writer );
First, we create a sample Article object to work with. Then, we try to get a Factory object from the Factory and roll back to the default (XMLWriter) if an exception occurs.
Finally, we pass the Writer object to the write() method of our Article and output the result.
Conclusion
In this tutorial, I provide an introduction to polymorphism and explain interfaces in PHP. I hope you realize that I'm only showing you one potential use case for polymorphism.
Polymorphism is an elegant way to avoid ugly conditional statements in your OOP code. It follows the principle of keeping your components separate, and it's an integral part of many design patterns. If you have any questions, don’t hesitate to ask in the comments!
Translated from: http://net.tutsplus.com/tutorials/php/understanding-and-applying-polymorphism-in-php/
Original text published at: http://ihacklog.com/?p= 4703

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










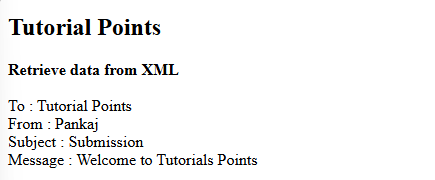
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
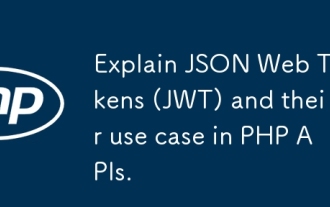
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
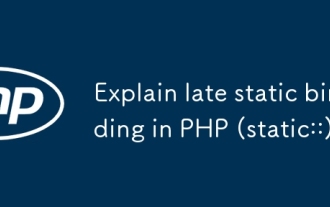
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
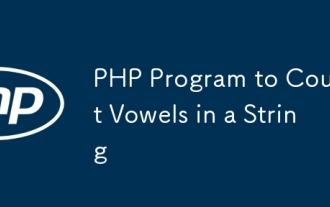
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
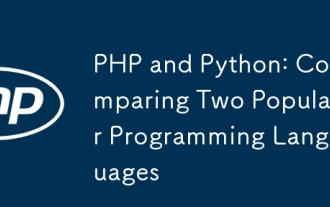
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
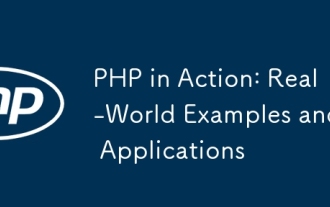
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
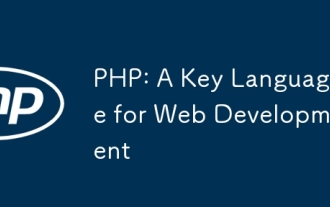
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
