php array_walk() array function_PHP tutorial
/*Function array_walk(): Single array callback function---for each member in the array Apply user function
* 1. Syntax: bool array_walk ( array &array, callback funcname [, mixed $userdata] )
* 2. Description: Return TRUE if successful, return FALSE if failed
* 3. Notes:
* 3.1. $funcname is a callback function defined by the user. It accepts 2 parameters. The first parameter is the value of the array $array, and the second parameter is the key name of the array $array. , if the third parameter $userdata is provided, it will be passed to the callback function $funcname as the third parameter
* 3.2. Using the callback function can directly change the value of each unit of the array, but changing each key name is invalid
* 3.3. This function will not be affected by the internal array pointer of array. array_walk() will traverse the entire array regardless of the position of the pointer
*
* 3.4. The user should not change the array itself in the callback function, such as adding/deleting cells, unset cells, etc., if array_walk()
* If the array it acts on changes, the behavior of this function is undefined and unpredictable.
*/
$words=array("l"=>"lemon","o"=>"orange","b"=>"banana","a"=>" apple");
//Define a callback function to output array elements
function words_print($value,$key,$prefix){
echo "$prefix:$key=>$value
}
//Define a callback function to directly change the value of the element
function words_alter(&$value,$key){
$value=ucfirst($value);
$key=strtoupper(key);
}
//Output the value of the element
array_walk($words,'words_print','words');
//Change the value of the element
array_walk($words,'words_alter');
echo "
"; <br>print_r($words); <br>echo "";


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










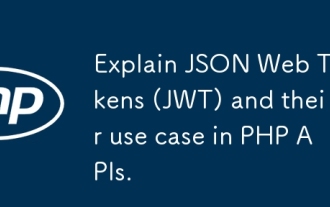
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
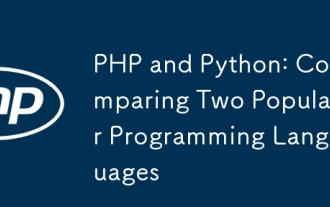
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
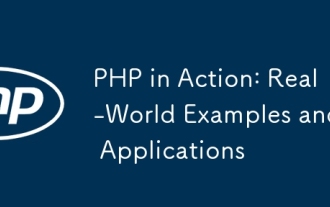
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
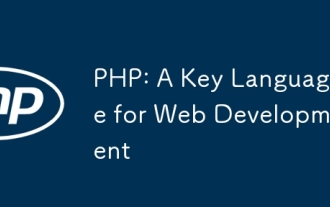
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
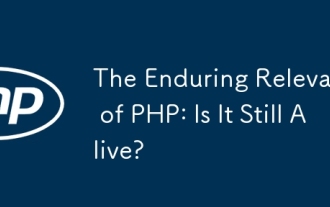
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.
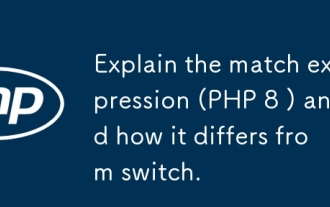
In PHP8, match expressions are a new control structure that returns different results based on the value of the expression. 1) It is similar to a switch statement, but returns a value instead of an execution statement block. 2) The match expression is strictly compared (===), which improves security. 3) It avoids possible break omissions in switch statements and enhances the simplicity and readability of the code.
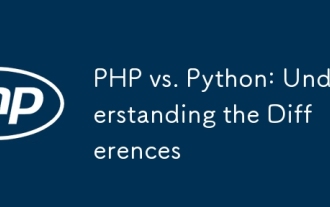
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
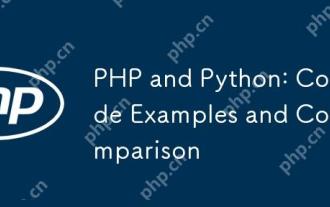
PHP and Python have their own advantages and disadvantages, and the choice depends on project needs and personal preferences. 1.PHP is suitable for rapid development and maintenance of large-scale web applications. 2. Python dominates the field of data science and machine learning.
