PHP get time difference
PHP obtains the time difference. This is a function that we often use during the development process. For example, if we want to check data for a certain time period, we will use this function.
Example 1:
/** * 功能:获取时间差 * @param int $time * @return string 时间差值 */ function tranTime($time) { $rtime = date("m-d H:i",$time); $htime = date("H:i",$time); $time = time() - $time; if ($time < 60) { $str = '刚刚'; } elseif ($time < 60 * 60) { $min = floor($time/60); $str = $min.'分钟前'; } elseif ($time < 60 * 60 * 24) { $h = floor($time/(60*60)); $str = $h.'小时前 '.$htime; } elseif ($time < 60 * 60 * 24 * 3) { $d = floor($time/(60*60*24)); if($d==1) $str = '昨天 '.$rtime; else $str = '前天 '.$rtime; } else { $str = $rtime; } return $str; }
Example 2:
function time_tran($the_time){ $now_time = date("Y-m-d H:i:s",time()+8*60*60); $now_time = strtotime($now_time); $show_time = strtotime($the_time); $dur = $now_time - $show_time; if($dur < 0){ return $the_time; }else{ if($dur < 60){ return $dur.'秒前'; }else{ if($dur < 3600){ return floor($dur/60).'分钟前'; }else{ if($dur < 86400){ return floor($dur/3600).'小时前'; }else{ if($dur < 259200){//3天内 return floor($dur/86400).'天前'; }else{ return $the_time; } } }
Example 3:
function format_date($time){ $t=time()-$time; $f=array( '31536000'=>'年', '2592000'=>'个月', '604800'=>'星期', '86400'=>'天', '3600'=>'小时', '60'=>'分钟', '1'=>'秒' ); foreach ($f as $k=>$v) { if (0 !=$c=floor($t/(int)$k)) { return $c.$v.'前'; } } }
Example 4:
function formatTime($date) { $str = ''; $timer = strtotime($date); $diff = $_SERVER['REQUEST_TIME'] - $timer; $day = floor($diff / 86400); $free = $diff % 86400; if($day > 0) { return $day."天前"; }else{ if($free>0){ $hour = floor($free / 3600); $free = $free % 3600; if($hour>0){ return $hour."小时前"; }else{ if($free>0){ $min = floor($free / 60); $free = $free % 60; if($min>0){ return $min."分钟前"; }else{ if($free>0){ return $free."秒前"; }else{ return '刚刚'; } } }else{ return '刚刚'; } } }else{ return '刚刚'; } } }
Example 5:
function time_tran($the_time){ $now_time = date("Y-m-d H:i:s",time()+8*60*60); $now_time = strtotime($now_time); $show_time = strtotime($the_time); $dur = $now_time - $show_time; if($dur < 0){ return $the_time; }else{ if($dur < 60){ return $dur.'秒前'; }else{ if($dur < 3600){ return floor($dur/60).'分钟前'; }else{ if($dur < 86400){ return floor($dur/3600).'小时前'; }else{ if($dur < 259200){//3天内 return floor($dur/86400).'天前'; }else{ return $the_time; } } } } } }
Example 6:
/* * author: Solon Ring * time: 2011-11-02 * 发博时间计算(年,月,日,时,分,秒) * $createtime 可以是当前时间 * $gettime 你要传进来的时间 */ class Mygettime{ function __construct($createtime,$gettime) { $this->createtime = $createtime; $this->gettime = $gettime; } function getSeconds() { return $this->createtime-$this->gettime; } function getMinutes() { return ($this->createtime-$this->gettime)/(60); } function getHours() { return ($this->createtime-$this->gettime)/(60*60); } function getDay() { return ($this->createtime-$this->gettime)/(60*60*24); } function getMonth() { return ($this->createtime-$this->gettime)/(60*60*24*30); } function getYear() { return ($this->createtime-$this->gettime)/(60*60*24*30*12); } function index() { if($this->getYear() > 1) { if($this->getYear() > 2) { return date("Y-m-d",$this->gettime); exit(); } return intval($this->getYear())." 年前"; exit(); } if($this->getMonth() > 1) { return intval($this->getMonth())." 月前"; exit(); } if($this->getDay() > 1) { return intval($this->getDay())." 天前"; exit(); } if($this->getHours() > 1) { return intval($this->getHours())." 小时前"; exit(); } if($this->getMinutes() > 1) { return intval($this->getMinutes())." 分钟前"; exit(); } if($this->getSeconds() > 1) { return intval($this->getSeconds()-1)." 秒前"; exit(); } } } //类的使用实例 /* * * 调用类输出方式 * * $a = new Mygettime(time(),strtotime('-25 month')); * echo iconv('utf-8', 'gb2312', $a->index())?iconv('utf-8', 'gb2312', $a->index()):iconv('utf-8', 'gb2312', '当前'); * */

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










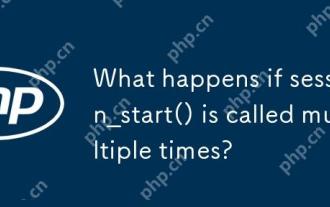
Multiple calls to session_start() will result in warning messages and possible data overwrites. 1) PHP will issue a warning, prompting that the session has been started. 2) It may cause unexpected overwriting of session data. 3) Use session_status() to check the session status to avoid repeated calls.

AI can help optimize the use of Composer. Specific methods include: 1. Dependency management optimization: AI analyzes dependencies, recommends the best version combination, and reduces conflicts. 2. Automated code generation: AI generates composer.json files that conform to best practices. 3. Improve code quality: AI detects potential problems, provides optimization suggestions, and improves code quality. These methods are implemented through machine learning and natural language processing technologies to help developers improve efficiency and code quality.
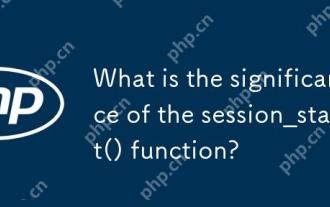
session_start()iscrucialinPHPformanagingusersessions.1)Itinitiatesanewsessionifnoneexists,2)resumesanexistingsession,and3)setsasessioncookieforcontinuityacrossrequests,enablingapplicationslikeuserauthenticationandpersonalizedcontent.
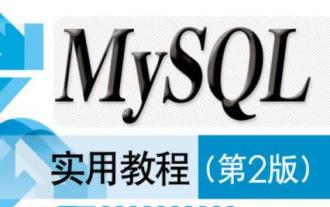
MySQL functions can be used for data processing and calculation. 1. Basic usage includes string processing, date calculation and mathematical operations. 2. Advanced usage involves combining multiple functions to implement complex operations. 3. Performance optimization requires avoiding the use of functions in the WHERE clause and using GROUPBY and temporary tables.

HTML5 brings five key improvements: 1. Semantic tags improve code clarity and SEO effects; 2. Multimedia support simplifies video and audio embedding; 3. Form enhancement simplifies verification; 4. Offline and local storage improves user experience; 5. Canvas and graphics functions enhance the visualization of web pages.

Composer is a dependency management tool for PHP, and manages project dependencies through composer.json file. 1) parse composer.json to obtain dependency information; 2) parse dependencies to form a dependency tree; 3) download and install dependencies from Packagist to the vendor directory; 4) generate composer.lock file to lock the dependency version to ensure team consistency and project maintainability.
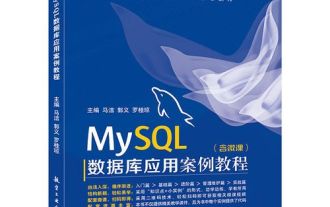
Methods for configuring character sets and collations in MySQL include: 1. Setting the character sets and collations at the server level: SETNAMES'utf8'; SETCHARACTERSETutf8; SETCOLLATION_CONNECTION='utf8_general_ci'; 2. Create a database that uses specific character sets and collations: CREATEDATABASEexample_dbCHARACTERSETutf8COLLATEutf8_general_ci; 3. Specify character sets and collations when creating a table: CREATETABLEexample_table(idINT
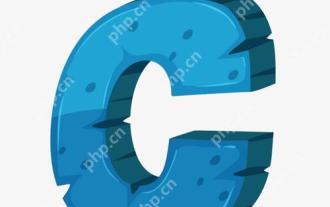
typetraits are used in C for compile-time type checking and operation, improving code flexibility and type safety. 1) Type judgment is performed through std::is_integral and std::is_floating_point to achieve efficient type checking and output. 2) Use std::is_trivially_copyable to optimize vector copy and select different copy strategies according to the type. 3) Pay attention to compile-time decision-making, type safety, performance optimization and code complexity. Reasonable use of typetraits can greatly improve code quality.
