A simple example sharing of PHP interpreter mode
PHPInterpreter ModeA simple example sharing
<?php // 解释器模式 abstract class Expression { private static $keyCount = 0; private $key = NULL; abstract function interpret(InterpreterContext $ctx); /** * as array key * @return auto increment value */ public function getKey() { if(!isset($this->key)) { self::$keyCount++; $this->key = self::$keyCount; } return $this->key; } } /** * context */ class InterpreterContext { private $expressionstore = array(); /** * store value */ public function replace(Expression $exp, $value) { $this->expressionstore[$exp->getKey()] = $value; } /** * find value */ public function lookup(Expression $exp) { return $this->expressionstore[$exp->getKey()]; } } /** * literal expression */ class LiteralExpression extends Expression { private $value; public function construct($value) { $this->value = $value; } public function interpret(InterpreterContext $ctx) { $ctx->replace($this, $this->value); } } /** * var=value expression */ class VariableExpression extends Expression { private $name; private $val; public function construct($name, $val=null) { $this->name = $name; $this->val = $val; } public function interpret(InterpreterContext $ctx) { if(!is_null($this->val)) { $ctx->replace($this, $this->val); $this->val = null; } } public function setValue($value) { $this->val = $value; } /** * @override */ public function getKey() { return $this->name; } } abstract class OperatorExpression extends Expression { protected $l_op; protected $r_op; public function construct(Expression $l, Expression $r) { $this->l_op = $l; $this->r_op = $r; } /** * @param $ctx InterpreterContext * @param $result_l Expression $l's result * @param $result_r Expression $r's result */ protected abstract function doInterpret(InterpreterContext $ctx, $result_l, $result_r); public function interpret(InterpreterContext $ctx) { $this->l_op->interpret($ctx); $this->r_op->interpret($ctx); $result_l = $ctx->lookup($this->l_op); $result_r = $ctx->lookup($this->r_op); $this->doInterpret($ctx, $result_l, $result_r); } } /** * equals */ class EqualsExpression extends OperatorExpression { protected function doInterpret(InterpreterContext $ctx, $result_l, $result_r) { $ctx->replace($this, $result_l == $result_r); } } /** * or */ class BooleanOrExpression extends OperatorExpression { protected function doInterpret(InterpreterContext $ctx, $result_l, $result_r) { $ctx->replace($this, $result_l || $result_r); } } /** * and */ class BooleanAndExpression extends OperatorExpression { protected function doInterpret(InterpreterContext $ctx, $result_l, $result_r) { $ctx->replace($this, $result_l && $result_r); } } /** * not */ class BooleanNotExpression extends Expression { private $expr; public function construct(Expression $e) { $this->expr = $e; } public function interpret(InterpreterContext $ctx) { $this->expr->interpret($ctx); $ctx->replace($this, !$ctx->lookup($this->expr)); } } // test code /* $ctx = new InterpreterContext(); $literarl = new LiteralExpression('four'); $literarl->interpret($ctx); // echo $ctx->lookup($literarl); $variable = new VariableExpression('input', '444'); $variable->interpret($ctx); // echo $ctx->lookup($variable); $answer = new VariableExpression('input'); $answer->interpret($ctx); echo $ctx->lookup($answer); */ // $input equas four or $input equals 4 $ctx = new InterpreterContext(); $input = new VariableExpression('input'); $statement = new BooleanOrExpression( new EqualsExpression($input, new LiteralExpression('four')), new EqualsExpression($input, new LiteralExpression(4)) ); $input->setValue('four'); $statement->interpret($ctx); var_dump($ctx->lookup($statement)); // true $input->setValue(4); $statement->interpret($ctx); var_dump($ctx->lookup($statement)); // true $input->setValue(42); $statement->interpret($ctx); var_dump($ctx->lookup($statement)); // false $not = new BooleanNotExpression($statement); // true $not->interpret($ctx); var_dump( $ctx->lookup($not) );
The above is the detailed content of A simple example sharing of PHP interpreter mode. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










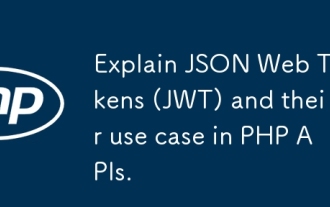
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
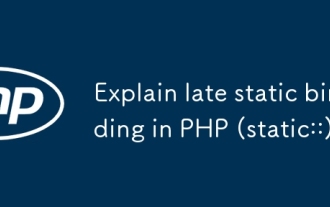
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
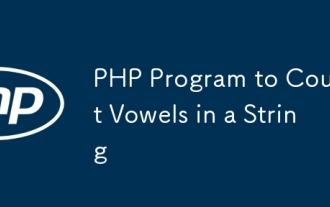
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
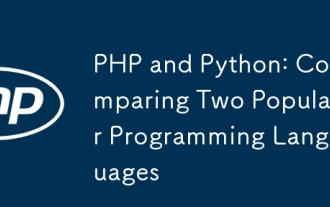
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
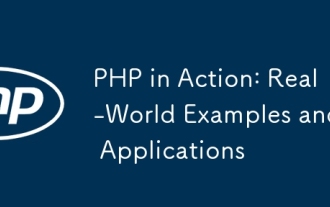
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
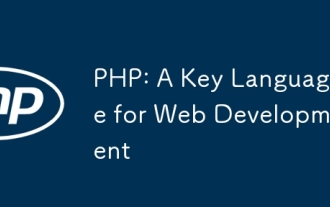
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
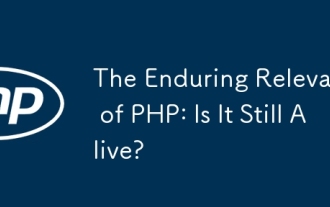
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.
