


How to make a highly secure PHP website, summary of security issues that need to be paid attention to when making a website
Everyone knows that PHP is currently the most popular web application programming language. But like other scripting languages, PHP also has several dangerous security holes. So in this tutorial, we'll take a look at a few practical tips to help you avoid some common PHP security issues.
Tip 1: Use appropriate error reports
Generally during the development process, many programmers always forget to make program error reports, which is extremely Big mistake, because proper error reporting is not only the best debugging tool, but also an excellent security vulnerability detection tool, which allows you to find as many problems as possible before you actually put the application online.
Of course there are many ways to enable error reporting. For example, in php.in Configuration file you can set to enable
Start error reporting at runtime
error_reporting(E_ALL);
Disable error reporting
error_reporting(0);
Tip 2: Not using PHP’s Weak attribute
There are several PHP properties that need to be set to OFF. Generally, they exist in PHP4, but their use is not recommended in PHP5. Especially in PHP6, these attributes were removed.
Register global variables
When register_globals is set to ON, it is equivalent to setting Environment, GET, POST, COOKIE or Server variables are defined as global variables. At this time, you don't need to write $_POST['username'] to get the form variable 'username'. You only need '$username' to get this variable.
Then you must be thinking that since setting register_globals to ON has such convenient benefits, why not use it? Because if you do this it will bring about a lot of security issues, and it may also conflict with local variable names.
For example, take a look at the following code:
if( !empty( $_POST[‘username'] ) && $_POST[‘username'] == ‘test123′ && !empty( $_POST[‘password'] ) && $_POST[‘password'] == “pass123″ ) { $access = true; }
If register_globals is set to ON during operation, then the user only needs to transmit access=1 in a query string to get the PHP script running Anything anymore.
Disable global variables in .htaccess
php_flag register_globals 0
Disable global variables in php.ini
register_globals = Off
Disable magic like magic_quotes_gpc, magic_quotes_runtime, magic_quotes_sybase Quotes
Set in .htaccess file
php_flag magic_quotes_gpc 0 php_flag magic_quotes_runtime 0
Set in php.ini
magic_quotes_gpc = Off magic_quotes_runtime = Off magic_quotes_sybase = Off
Tip 3: Validate user input
Of course you You can also validate user input. First you must know the data type you expect the user to input. In this way, you can be prepared on the browser side to prevent users from malicious attacks on you.
Tip 4: Avoid cross-site scripting attacks by users
In web applications, it is simple to accept user input in the form and then feedback the results. When accepting user input, it would be very dangerous to allow HTML format input, because this would also allow JavaScript to intrude in unpredictable ways and execute directly. Even if there is only one such vulnerability, cookie data may be stolen and the user's account may be stolen.
Tip 5: Prevent SQL injection attacks
PHP basically does not provide any tools to protect your database, so when you connect to the database, You can use the following mysqli_real_escape_string function.
$username = mysqli_real_escape_string( $GET[‘username'] ); mysql_query( “SELECT * FROM tbl_employee WHERE username = '”.$username.“‘”);
The above is the detailed content of How to make a highly secure PHP website, summary of security issues that need to be paid attention to when making a website. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










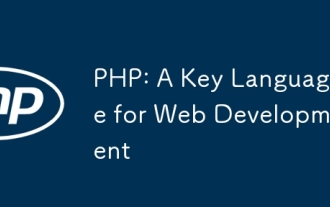
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
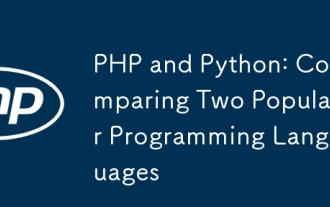
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
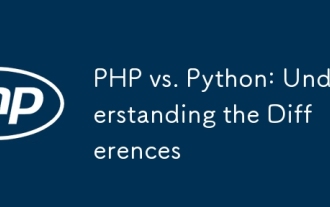
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
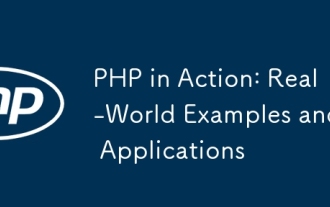
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
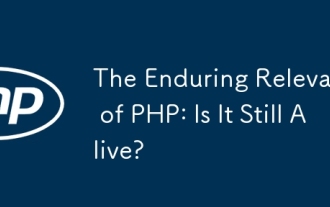
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.
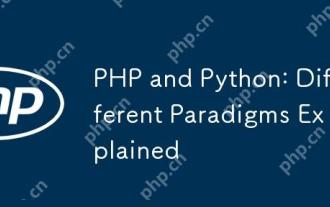
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
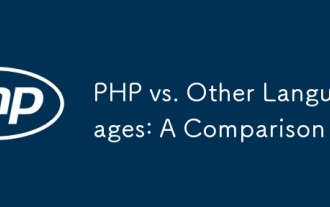
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
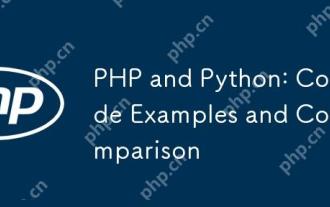
PHP and Python have their own advantages and disadvantages, and the choice depends on project needs and personal preferences. 1.PHP is suitable for rapid development and maintenance of large-scale web applications. 2. Python dominates the field of data science and machine learning.
