


How does the singleton mode solve the access_token global cache problem?
Initially put the access_token in the database, and determine whether it has expired each time it is obtained. It can solve the problem of validity period and number of visits of access_token. However, recently it is necessary to interface the official account with the system and cancel the database of the official account. At this time, it is not appropriate to put access_token, etc. into the system database.
Benefited from this article by Xue Xilin: WeChat handles the issue of access_token global cache, implemented Use singleton mode to cache access_token.
#Remember it for future reference.
#Mark your code structure:
public class TokenSingleton { //缓存accessToken 的Map ,map中包含 一个accessToken 和 缓存的时间戳 private Map<String, String> map = new HashMap<>(); private TokenSingleton() { } private static TokenSi ngleton single = null; // 静态工厂方法 public static TokenSingleton getInstance() { if (single == null) { single = new TokenSingleton(); } return single; } public Map<String, String> getMap() { String time = map.get("time"); String accessToken = map.get("access_token"); Long nowDate = new Date().getTime(); if (accessToken != null && time != null && nowDate - Long.parseLong(time) < 6000*1000) { System.out.println("accessToken存在,且没有超时 , 返回单例"); } else { System.out.println("accessToken 超时 , 或者不存在 , 重新获取"); String access_token=JSSDKUtil.getAccessToken(); //这里是直接调用微信的API去直接获取 accessToken 和Jsapi_ticket 获取 String jsapi_token = JSSDKUtil.getTicket(access_token); //"获取jsapi_token"; map.put("time", nowDate + ""); map.put("access_token", access_token); map.put("jsapi_token", jsapi_token); } return map; } public void setMap(Map<String, String> map) { this.map = map; } public static TokenSingleton getSingle() { return single; } public static void setSingle(TokenSingleton single) { TokenSingleton.single = single; } }
Another tool class JSSDKUtil.java intercepted part:
##public static String getSignature(String accessToken,String jsapi_ticket,String noncestr,String timestamp,String url){
System.out.println("accessToken:"+accessToken+"\njsapi_ticket:"+jsapi_ticket+"\n时间戳:"+timestamp+"\n随机字符串:"+noncestr+"\nURL:"+url);
//5、将参数排序并拼接字符串
String str = "jsapi_ticket="+jsapi_ticket+"&noncestr="+noncestr+"×tamp="+timestamp+"&url="+url;
//6、将字符串进行sha1加密
String signature =SHA1(str);
System.out.println("参数:"+str+"\n签名:"+signature);
return signature;
}
public static String getAccessToken() {
String wx_appid = getProperties("wx_appid");
String wx_appsecret = getProperties("wx_appsecret");
String access_token = "";
String grant_type = "client_credential";//获取access_token填写client_credential
String AppId=wx_appid;//第三方用户唯一凭证
String secret=wx_appsecret;//第三方用户唯一凭证密钥,即appsecret
//这个url链接地址和参数皆不能变
String url = "https://api.weixin.qq.com/cgi-bin/token?grant_type="+grant_type+"&appid="+AppId+"&secret="+secret;
try {
URL urlGet = new URL(url);
HttpURLConnection http = (HttpURLConnection) urlGet.openConnection();
http.setRequestMethod("GET"); // 必须是get方式请求
http.setRequestProperty("Content-Type","application/x-www-form-urlencoded");
http.setDoOutput(true);
http.setDoInput(true);
System.setProperty("sun.net.client.defaultConnectTimeout", "30000");// 连接超时30秒
System.setProperty("sun.net.client.defaultReadTimeout", "30000"); // 读取超时30秒
http.connect();
InputStream is = http.getInputStream();
int size = is.available();
byte[] jsonBytes = new byte[size];
is.read(jsonBytes);
String message = new String(jsonBytes, "UTF-8");
JSONObject demoJson = JSONObject.fromObject(message);
System.out.println("JSON字符串:"+demoJson);
access_token = demoJson.getString("access_token");
is.close();
} catch (Exception e) {
e.printStackTrace();
}
return access_token;
}
public static String getTicket(String access_token) {
String ticket = null;
String url = "https://api.weixin.qq.com/cgi-bin/ticket/getticket?access_token="+ access_token +"&type=jsapi";//这个url链接和参数不能变
try {
URL urlGet = new URL(url);
HttpURLConnection http = (HttpURLConnection) urlGet.openConnection();
http.setRequestMethod("GET"); // 必须是get方式请求
http.setRequestProperty("Content-Type","application/x-www-form-urlencoded");
http.setDoOutput(true);
http.setDoInput(true);
System.setProperty("sun.net.client.defaultConnectTimeout", "30000");// 连接超时30秒
System.setProperty("sun.net.client.defaultReadTimeout", "30000"); // 读取超时30秒
http.connect();
InputStream is = http.getInputStream();
int size = is.available();
byte[] jsonBytes = new byte[size];
is.read(jsonBytes);
String message = new String(jsonBytes, "UTF-8");
JSONObject demoJson = JSONObject.fromObject(message);
System.out.println("JSON字符串:"+demoJson);
ticket = demoJson.getString("ticket");
is.close();
} catch (Exception e) {
e.printStackTrace();
}
return ticket;
}
public static String SHA1(String decript) {
try {
MessageDigest digest = java.security.MessageDigest.getInstance("SHA-1");
digest.update(decript.getBytes());
byte messageDigest[] = digest.digest();
// Create Hex String
StringBuffer hexString = new StringBuffer();
// 字节数组转换为 十六进制 数
for (int i = 0; i < messageDigest.length; i++) {
String shaHex = Integer.toHexString(messageDigest[i] & 0xFF);
if (shaHex.length() < 2) {
hexString.append(0);
}
hexString.append(shaHex);
}
return hexString.toString();
} catch (NoSuchAlgorithmException e) {
e.printStackTrace();
}
return "";
}
The above is the detailed content of How does the singleton mode solve the access_token global cache problem?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










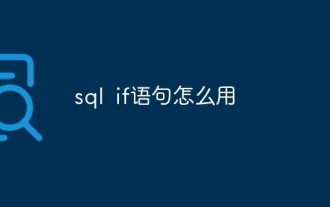
SQL IF statements are used to conditionally execute SQL statements, with the syntax as: IF (condition) THEN {statement} ELSE {statement} END IF;. The condition can be any valid SQL expression, and if the condition is true, execute the THEN clause; if the condition is false, execute the ELSE clause. IF statements can be nested, allowing for more complex conditional checks.
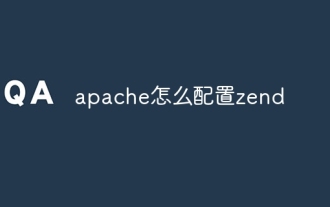
How to configure Zend in Apache? The steps to configure Zend Framework in an Apache Web Server are as follows: Install Zend Framework and extract it into the Web Server directory. Create a .htaccess file. Create the Zend application directory and add the index.php file. Configure the Zend application (application.ini). Restart the Apache Web server.
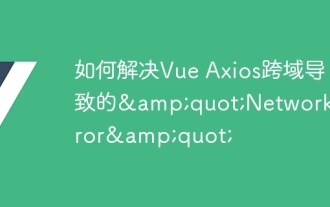
Methods to solve the cross-domain problem of Vue Axios include: Configuring the CORS header on the server side using the Axios proxy using JSONP using WebSocket using the CORS plug-in
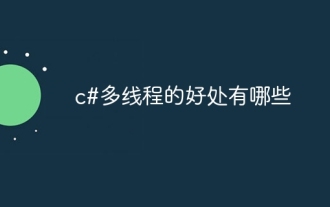
The advantage of multithreading is that it can improve performance and resource utilization, especially for processing large amounts of data or performing time-consuming operations. It allows multiple tasks to be performed simultaneously, improving efficiency. However, too many threads can lead to performance degradation, so you need to carefully select the number of threads based on the number of CPU cores and task characteristics. In addition, multi-threaded programming involves challenges such as deadlock and race conditions, which need to be solved using synchronization mechanisms, and requires solid knowledge of concurrent programming, weighing the pros and cons and using them with caution.
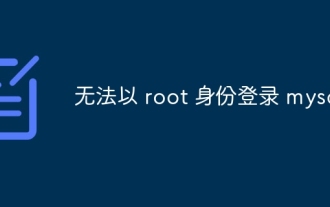
The main reasons why you cannot log in to MySQL as root are permission problems, configuration file errors, password inconsistent, socket file problems, or firewall interception. The solution includes: check whether the bind-address parameter in the configuration file is configured correctly. Check whether the root user permissions have been modified or deleted and reset. Verify that the password is accurate, including case and special characters. Check socket file permission settings and paths. Check that the firewall blocks connections to the MySQL server.
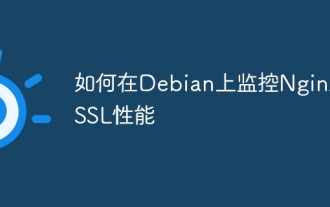
This article describes how to effectively monitor the SSL performance of Nginx servers on Debian systems. We will use NginxExporter to export Nginx status data to Prometheus and then visually display it through Grafana. Step 1: Configuring Nginx First, we need to enable the stub_status module in the Nginx configuration file to obtain the status information of Nginx. Add the following snippet in your Nginx configuration file (usually located in /etc/nginx/nginx.conf or its include file): location/nginx_status{stub_status
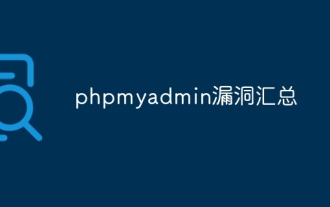
The key to PHPMyAdmin security defense strategy is: 1. Use the latest version of PHPMyAdmin and regularly update PHP and MySQL; 2. Strictly control access rights, use .htaccess or web server access control; 3. Enable strong password and two-factor authentication; 4. Back up the database regularly; 5. Carefully check the configuration files to avoid exposing sensitive information; 6. Use Web Application Firewall (WAF); 7. Carry out security audits. These measures can effectively reduce the security risks caused by PHPMyAdmin due to improper configuration, over-old version or environmental security risks, and ensure the security of the database.
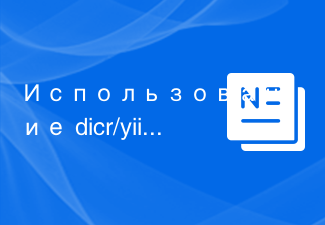
VprocesserazrabotkiveB-enclosed, Мнепришлостольностьсясзадачейтерациигооглапидляпапакробоглесхетсigootrive. LEAVALLYSUMBALLANCEFRIABLANCEFAUMDOPTOMATIFICATION, ČtookazaLovnetakProsto, Kakaožidal.Posenesko
